トレイル オブジェクトは、2つの添付ファイル間のトレイル効果を作成するために使用されます。添付ファイルが空間を移動すると、定義されたプレーンにテクスチャが描かれます。これは、プロジェクターの後ろのトレーサートレイル、足跡、タイヤトラックなどの動作を視覚化する効果を作成するのに一般的に使用されます。
詳しくは、トレイルを参照してください。
コードサンプル
This example demos the functionality of Trails by creating a BasePart to be the parent of the trail. Two Attachments are then parented to the part. The positions of these two attachments (more importantly the distance between them) determines where the trail is drawn as the part moves.
For these attachments to create a trail as described, a new Trail is parented to the part and its Attachment0 and Attachment1 are parented to attachment0 and attachment1 respectively. Finally, TweenService is used to move the part back and forth, showing how the trail is drawn as the part (and its attachments) move.
local TweenService = game:GetService("TweenService")
-- Create a parent part
local part = Instance.new("Part")
part.Material = Enum.Material.SmoothPlastic
part.Size = Vector3.new(4, 1, 2)
part.Position = Vector3.new(0, 5, 0)
part.Anchored = true
part.Parent = workspace
-- Create attachments on part
local attachment0 = Instance.new("Attachment")
attachment0.Name = "Attachment0"
attachment0.Position = Vector3.new(-2, 0, 0)
attachment0.Parent = part
local attachment1 = Instance.new("Attachment")
attachment1.Name = "Attachment1"
attachment1.Position = Vector3.new(2, 0, 0)
attachment1.Parent = part
-- Create a new trail
local trail = Instance.new("Trail")
trail.Attachment0 = attachment0
trail.Attachment1 = attachment1
trail.Parent = part
-- Tween part to display trail
local dir = 15
while true do
dir *= -1
local goal = { Position = part.Position + Vector3.new(0, 0, dir) }
local tweenInfo = TweenInfo.new(3)
local tween = TweenService:Create(part, tweenInfo, goal)
tween:Play()
task.wait(4)
end
概要
プロパティ
Attachment1 と一緒に、トレイルがどこでそのセグメントを描き始めるかを決定します。
Attachment0 と一緒に、トレイルがどこでそのセグメントを描き始めるかを決定します。
LightInfluence が 1未満のとき、トレイルから放出される光をスケールします。
寿命中のトレイルの色。
トレイルが描かれるかどうかを決定します。
方向を問わず、トレイルが常にカメラに向かうかどうかを決定します。
トレイルの各セグメントがどのくらい続くか、秒単位で決定します。
トレイルの色がどの程度背後の色とブレンドされるかを決定します。
トレイルが環境の照明に影響を受ける程度を決定します。
トレイルの最大長を設定します。
トレイルの最小長を設定します。
トレイルに表示されるテクスチャのコンテンツ ID。
トレイルのテクスチャの長さを設定し、TextureMode に依存します。
Texture スケール、重複、トレイルの添付ファイルと一緒に移動する方法を決定します。
トレイルのセグメントの透明度を Lifetime 上で設定します。
ライフサイクルの間、トレイルの幅をスケールする。
プロパティ
Attachment0
A Trail は、自分の 添付ファイル0 と Attachment1 の位置でセグメントを描画開始します。トレイルが Enabled のとき、その付属物の位置をすべてのフレームに記録し、これらの位置を前のフレームの付属物の位置に接続し、トレイルの Color と Texture によって満たされるポリゴンを作成します。
トレイルを描画中にトレイルの添付ファイルを変更すると、トレイルが既に描画したすべてのセグメントが削除されます。
Attachment1
A Trail は、自分の Attachment0 と 添付ファイル1 の位置でセグメントを描画開始します。トレイルが Enabled のとき、その付属物の位置をすべてのフレームに記録し、これらの位置を前のフレームの付属物の位置に接続し、トレイルの Color と Texture によって満たされるポリゴンを作成します。
トレイルを描画中にトレイルの添付ファイルを変更すると、トレイルが既に描画したすべてのセグメントが削除されます。
Brightness
LightInfluence が 1未満のとき、トレイルから放出される光をスケールします。このプロパティはデフォルトで 1 であり、0から10000の範囲内の任意の数に設定できます。LightInfluence の値を増加すると、このプロパティの値の効果が減少します。
Color
寿命中のトレイルの色を決定します。如果 Texture が設定する定されている場合、この色がテクスチャを着色します。
このプロパティは ColorSequence で、色をトレイルの長さに応じて変更できるように構成できます。トレイルセグメントのいくつかが描かれた後、色が変更されると、古いセグメント全体が新しい色に合わせて更新されます。
コードサンプル
This example creates a Trail with a gradient color, meaning that the color at one end of the trail is different than the color at the opposite end, and both colors blend together as they get closer to the middle of the trail.
local TweenService = game:GetService("TweenService")
-- Create a parent part
local part = Instance.new("Part")
part.Material = Enum.Material.SmoothPlastic
part.Size = Vector3.new(4, 1, 2)
part.Position = Vector3.new(0, 5, 0)
part.Anchored = true
part.Parent = workspace
-- Create attachments on part
local attachment0 = Instance.new("Attachment")
attachment0.Name = "Attachment0"
attachment0.Position = Vector3.new(-2, 0, 0)
attachment0.Parent = part
local attachment1 = Instance.new("Attachment")
attachment1.Name = "Attachment1"
attachment1.Position = Vector3.new(2, 0, 0)
attachment1.Parent = part
-- Create a new trail with color gradient
local trail = Instance.new("Trail")
trail.Attachment0 = attachment0
trail.Attachment1 = attachment1
local color1 = Color3.fromRGB(255, 0, 0)
local color2 = Color3.fromRGB(0, 0, 255)
trail.Color = ColorSequence.new(color1, color2)
trail.Parent = part
-- Tween part to display trail
local dir = 15
while true do
dir *= -1
local goal = { Position = part.Position + Vector3.new(0, 0, dir) }
local tweenInfo = TweenInfo.new(3)
local tween = TweenService:Create(part, tweenInfo, goal)
tween:Play()
task.wait(4)
end
Enabled
このプロパティは、トレイルが描かれるかどうかを決定します。
トレイルが描画されている間に に設定した場合、新しいセグメントは描画されませんが、既存のセグメントは、終了時に自然にクリーンアップされます。既存のセグメントを強制的にクリーンアップするには、同時に Clear() メソッドを呼び出します。
FaceCamera
A Trail は、3D 空間に存在する 2D プロジェクションで、すべての角度から見えない可能性があります。フェイスカメラ プロパティが に設定されると、トレイルが常に 方向を向いていることを保証し、向きが向いているかどうかに関係なく。
このプロパティをすぐに変更すると、すべての既存および将来のトレイルセグメントに影響します。
Lifetime
ライフタイム プロパティは、トレイルの各セグメントが消えるまで、秒ごとにどれほど長く続くかを決定します。デフォルトは 2 秒ですが、0.01 から 20 の間でどこでも設定できます。
トレイルの期間は、その効果の Color および Transparency プロパティによっても、それぞれのセグメントがどのように描かれるかを決定するのに使用されます。これらのプロパティの両方がシーケンスであり、セグメントのライフサイクル中に特定のキーポイントで値を定義し、セグメントが年齢となるにつれて値をインターポレートします。
トレイルのライフタイムが変更されると、既存のセグメントはすぐに新しいライフタイムを持っているように振る舞い、新しいライフタイムが存在した場合、長く存在していた場合はすぐに削除されます。
LightEmission
トレイルの色がどの程度背後の色とブレンドされるかを決定します。0から1の範囲で設定する必要があります。値 0 は通常のブレンドモードを使用し、値 1 は追加ブレンドを使用します。
このプロパティは、環境光によってトレイルが影響を受ける方法を決定する LightInfluence と混同してはならない。
このプロパティをすぐに変更すると、トレイルのすべての既存および将来のセグメントが影響を受けます。
このプロパティは、 トレイルが環境を照らすのを 阻止しません。
LightInfluence
トレイルが環境の照明に影響を受ける程度を 0 から 1 の間で決定します。0のとき、トレイルは環境の照明に影響を受けません。1のとき、照明に完全に影響を受けることになります。BasePart としても。
このプロパティをすぐに変更すると、トレイルのすべての既存および将来のセグメントが影響を受けます。
また、LightEmission によって、トレイルの色が後ろの色とどの程度ブレンドされるかが指定されています。
LocalTransparencyModifier
MaxLength
このプロパティは、スタッドでのトレイルの最大長を決定します。その値は 0 にデフォルトで設定され、トレイルは最大の長さを持たず、トレイルセグメントは Lifetime で期限切れします。
このプロパティは、描画する前にトレイルが持つ最小長さを決定する MinLength プロパティと一緒に使用できます。
MinLength
このプロパティは、スタッドでのトレイルの最小長を決定します。トレイルの添付ファイルのどちらも、この値以上移動しない場合、新しいセグメントは作成されず、現在のセグメントの端点は添付ファイルの現在の位置に移動されます。
このプロパティを変更すると、描画された新しい セグメント のみに影響し、既に描画されたすべての古いセグメントは現在の長さを維持します。
このプロパティは、最も古いセグメントが削除される前に最大トレイル長を決定する MaxLength プロパティと一緒に使用できます。
Texture
トレイルに表示されるテクスチャのコンテンツ ID。このプロパティが設設定するされていない場合、トレイルは厚い平面として表示されます;これは、テクスチャのテクスチャが無効なコンテンツIDに設定されているか、テクスチャに関連する画像がまだロードされていない場合も同様です。
テクスチャの外観は、Color や Transparency などの他のトレイルプロパティによってさらに修正できます。
テクスチャのスケーリングは、Attachment0 と Attachment1 の距離、および TextureMode、TextureLength、および WidthScale プロパティによって決定されます。
コードサンプル
This example adds a paw prints texture to a trail object. In order for the paw prints to remain "stamped" in place after rendering, TextureMode is set to Enum.TextureMode.Static.
local TweenService = game:GetService("TweenService")
-- Create a parent part
local part = Instance.new("Part")
part.Material = Enum.Material.SmoothPlastic
part.Size = Vector3.new(2, 1, 2)
part.Position = Vector3.new(0, 5, 0)
part.Anchored = true
part.Parent = workspace
-- Create attachments on part
local attachment0 = Instance.new("Attachment")
attachment0.Name = "Attachment0"
attachment0.Position = Vector3.new(-1, 0, 0)
attachment0.Parent = part
local attachment1 = Instance.new("Attachment")
attachment1.Name = "Attachment1"
attachment1.Position = Vector3.new(1, 0, 0)
attachment1.Parent = part
-- Create a new trail with color gradient
local trail = Instance.new("Trail")
trail.Attachment0 = attachment0
trail.Attachment1 = attachment1
trail.Texture = "rbxassetid://16178262222"
trail.TextureMode = Enum.TextureMode.Static
trail.TextureLength = 2
trail.Parent = part
-- Tween part to display trail
local dir = 15
while true do
dir *= -1
local goal = { Position = part.Position + Vector3.new(0, 0, dir) }
local tweenInfo = TweenInfo.new(3)
local tween = TweenService:Create(part, tweenInfo, goal)
tween:Play()
task.wait(4)
end
TextureMode
このプロパティは、TextureLength とともに、トレイルの Texture がどのようにスケールし、繰り返し、トレイルの添付ファイルと一緒に移動するかを決定します。このプロパティをすぐに変更すると、すべての既存および将来のトレイルセグメントに影響します。
スケールと反復
When テクスチャモード が Enum.TextureMode.Wrap または Enum.TextureMode.Static に設定されると、TextureLength プロパティは、トレイル全体で繰り返されるときのテクスチャの長さを設定します。
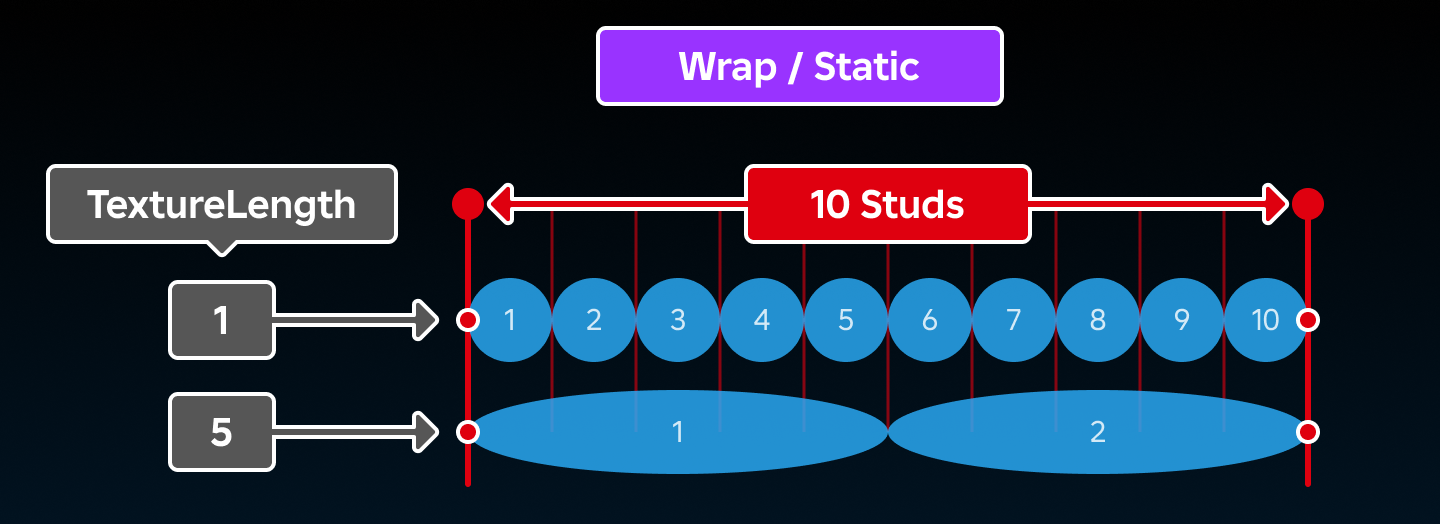
When テクスチャモード が Enum.TextureMode.Stretch に設定されると、テクスチャはトレイル全体の長さにわたって TextureLength 回繰り返されます。
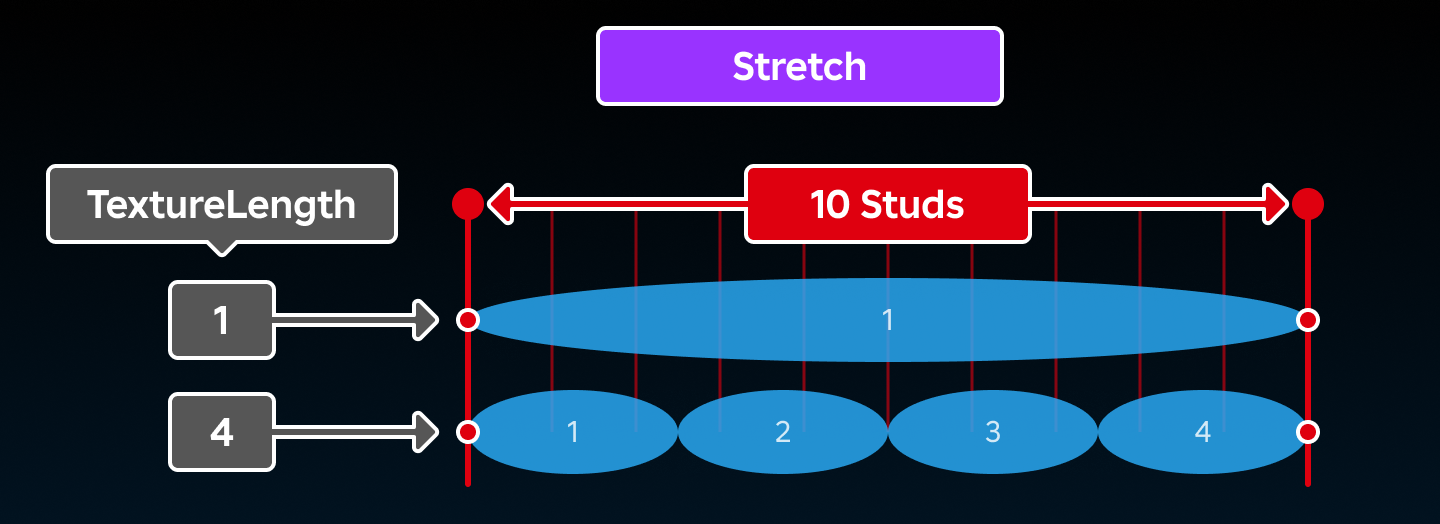
移動
テクスチャモード プロパティは、トレイルのテクスチャの 移動 にも以下のように影響します:
Enum.TextureMode.Stretch に設定すると、テクスチャはトレイルの寿命に基づいて伸び、トレイルの添付ファイルが移動を停止すると縮小します。
Enum.TextureMode.Wrap に設定すると、テクスチャはトレイルの長さが変化するに従ってタイル化されますが、テクスチャは付属物に対して静止します。
Enum.TextureMode.Static に設定すると、テクスチャは添付ファイルが移動すると巻き出され、寿命が達するまで残ります。この設定は、描画された場所で「スタンプ」として表示されるべきトレイルテクスチャに最適です、例えば、爪印やタイヤトラックです。
Transparency
トレイルのセグメントの透明度を Lifetime 上で設定します。この値は NumberSequence で、静的値であるか、トレイルセグメントのライフタイム中に変更できる意味があります。
WidthScale
このプロパティは、ライフサイクルの間にトレイルの幅を拡大する NumberSequence です。値は 0 から 1 の間にあり、トレイルの付属物の間の距離の乗数として機能します。たとえば、トレイルの添付ファイルが 2スタッド離れており、このプロパティの値が 0.5 である場合、トレイルの幅は 1スタッドであり、2つの添付ファイルの間にトレイルが中央に配置されます。
方法
Clear
このメソッドは、すぐにトレイルのすべてのセグメントをクリアし、より長いライフタイムを持つトレイルをクリーンアップするか、特定のアクションによりトレイルが削除される必操作がある場合に便利です。
このメソッドを呼び出すと、既存のセグメントにのみ影響が及びます。既存のトレイルセグメントをクリアするには、 と を一時的に新しいセグメントが描かれないようにし、トレイルの Enabled プロパティを同時に false に切り替えます。