GeometryService
*このコンテンツは、ベータ版のAI(人工知能)を使用して翻訳されており、エラーが含まれている可能性があります。このページを英語で表示するには、 こちら をクリックしてください。
特定のオブジェクトに関連しない幾何学オペレーションを含むサービス。
概要
方法
Class.Constraint|Constraints と Attachments を保持するテーブルを返します。それぞれの親と一緒に保持することを選択できます。
1つまたは複数の PartOperations を作成します。
別のパーツからのジオメトリの使用を削減するために、1つまたは複数の PartOperations を作成します。
別のパーツとのグラデーションを含めた複数のパーツから、PartOperations を作成します。
プロパティ
方法
CalculateConstraintsToPreserve
Class.Constraint|Constraints と Attachments の テーブルを返します。これらのテーブルを選択して保持することを選択できます。このテーブルをイテレートすると、Class.Limit|限定 と 1>Class.Attachments|アタッチメント1> の両方の親を含む、おすすめの制限とアタッチメントを保持できます。
注:Options テーブルには、tolerance 値 (番号) と/または WeldConstraintPreserve 値 ( 1>EnumerateWeldConstraintsPreserve1> ) が含まれる可能性があります。
- tolerance – 接続の距離の耐久性に関連して、Attachmentの保存と最も近いポイントの間の距離の耐久性について、接続と最も近いポイントの表面の間の距離の耐久性が Class.Instance.Parent|Parent</
パラメータ
ソリッドモデリングオペレーションが実行されたオリジナルオブジェクト、たとえば part の UnionAsync()。
メソッドのオプションの表:
- tolerance – 接続の距離の耐久性に関連して、Attachmentの保存と最も近いポイントの間の距離の耐久性について、接続と最も近いポイントの表面の間の距離の耐久性が Class.Instance.Parent|Parent</
戻り値
一般的な場合の情報を含むテーブル Constraints 、 NoCollisionConstraints 、および WeldConstraints 。1> Class.Attachment1> または 4> Class.Constructor4> をドロップす
For general case Constraints such as HingeConstraint :
<tbody><tr><td>アタッチメント</td><td><code>Class.Attachment</code></td></tr><tr><td>制限</td><td><code>Class.Constraint</code></td></tr><tr><td>アタッチメント親</td><td><code>Class.BasePart</code> または <code>nil</code></td></tr><tr><td>親コントラクト</td><td><code>Class.BasePart</code> または <code>nil</code></td></tr></tbody>
キー | タイプ |
---|
For WeldConstraints :
<tbody><tr><td>Welドクストランク</td><td><code>Class.WeldConstraint</code></td></tr><tr><td>親コントリンクター</td><td><code>Class.BasePart</code> または <code>nil</code></td></tr><tr><td>WeldConstraintPart0 部品</td><td><code>Class.BasePart</code></td></tr><tr><td>WeldConstraintPart1 パーツ</td><td><code>Class.BasePart</code></td></tr></tbody>
キー | タイプ |
---|
For NoCollisionConstraints :
<tbody><tr><td>当たり判定無効</td><td><code>Class.NoCollisionConstraint</code></td></tr><tr><td>NoCollisionReasonorParent</td><td><code>Class.BasePart</code> または <code>nil</code></td></tr><tr><td>NoCollisionConstraintPart0</td><td><code>Class.BasePart</code></td></tr><tr><td>NoCollisionConstraintPart1</td><td><code>Class.BasePart</code></td></tr></tbody>
キー | タイプ |
---|
コードサンプル
local GeometryService = game:GetService("GeometryService")
local mainPart = workspace.PurpleBlock
local otherParts = {workspace.BlueBlock}
local options = {
CollisionFidelity = Enum.CollisionFidelity.Default,
RenderFidelity = Enum.RenderFidelity.Automatic,
SplitApart = true
}
local constraintOptions = {
tolerance = 0.1,
weldConstraintPreserve = Enum.WeldConstraintPreserve.All,
dropAttachmentsWithoutConstraints = false
}
-- Perform subtract operation in pcall() since it's asyncronous
local success, newParts = pcall(function()
return GeometryService:SubtractAsync(mainPart, otherParts, options)
end)
if success and newParts then
-- Loop through resulting parts to reparent/reposition
for _, newPart in pairs(newParts) do
newPart.Parent = mainPart.Parent
newPart.CFrame = mainPart.CFrame
newPart.Anchored = mainPart.Anchored
end
-- Calculate constraints/attachments to either preserve or drop
local recommendedTable = GeometryService:CalculateConstraintsToPreserve(mainPart, newParts, constraintOptions)
-- Preserve constraints/attachments based on recommended table
for _, item in pairs(recommendedTable) do
if item.Attachment then
item.Attachment.Parent = item.AttachmentParent
if item.Constraint then
item.Constraint.Parent = item.ConstraintParent
end
elseif item.NoCollisionConstraint then
local newNoCollision = Instance.new("NoCollisionConstraint")
newNoCollision.Part0 = item.NoCollisionPart0
newNoCollision.Part1 = item.NoCollisionPart1
newNoCollision.Parent = item.NoCollisionParent
elseif item.WeldConstraint then
local newWeldConstraint = Instance.new("WeldConstraint")
newWeldConstraint.Part0 = item.WeldConstraintPart0
newWeldConstraint.Part1 = item.WeldConstraintPart1
newWeldConstraint.Parent = item.WeldConstraintParent
end
end
-- Destroy original parts
mainPart.Parent = nil
mainPart:Destroy()
for _, otherPart in pairs(otherParts) do
otherPart.Parent = nil
otherPart:Destroy()
end
end
IntersectAsync
主要部と他のパーツの交差点のジオメトリから、PartOperations を作成します。Parts および Class.PartOperation|PartOperations
メイン部分の次のプロパティ ( part ) は、結果となる PartOperations に適用されます:
- Class.BasePart.Color|Color , Material , MaterialVariant , 0> Class.BasePart.Reflectance|Reflectance0> , Color3>
- Class.BasePart.Anchored|Anchored , Density , Elasticity , 0> Class.BasePart.ElasticityWeight|Elasticity0> , 3> Class.BasePart.Fr
次のイメージ比較では、 IntersectAsync() は、パープルブロックとブルーブロックのアレイを使用して呼び出されます。結果として、 PartOperation は、両方のパーツの交差点の形状を表します。
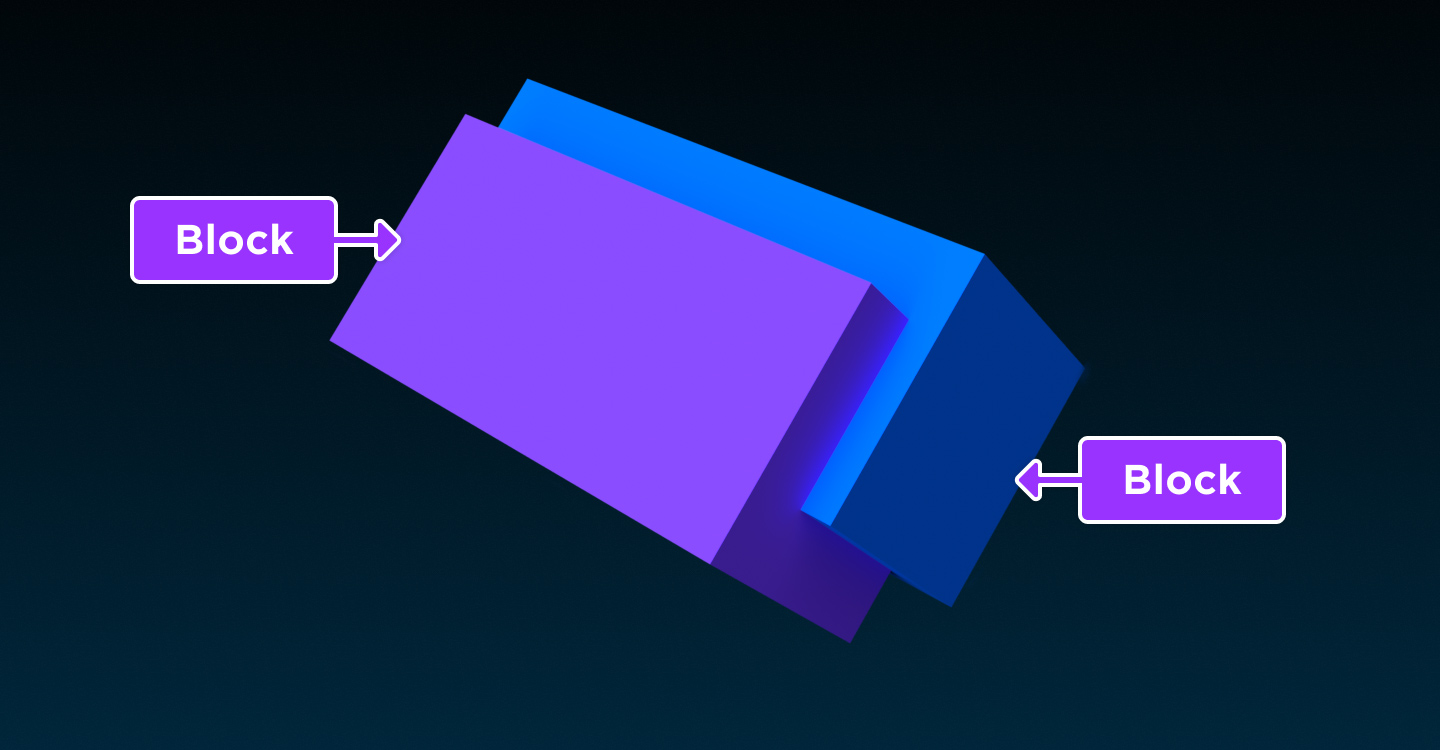
<figcaption>パーツを別々にする</figcaption>
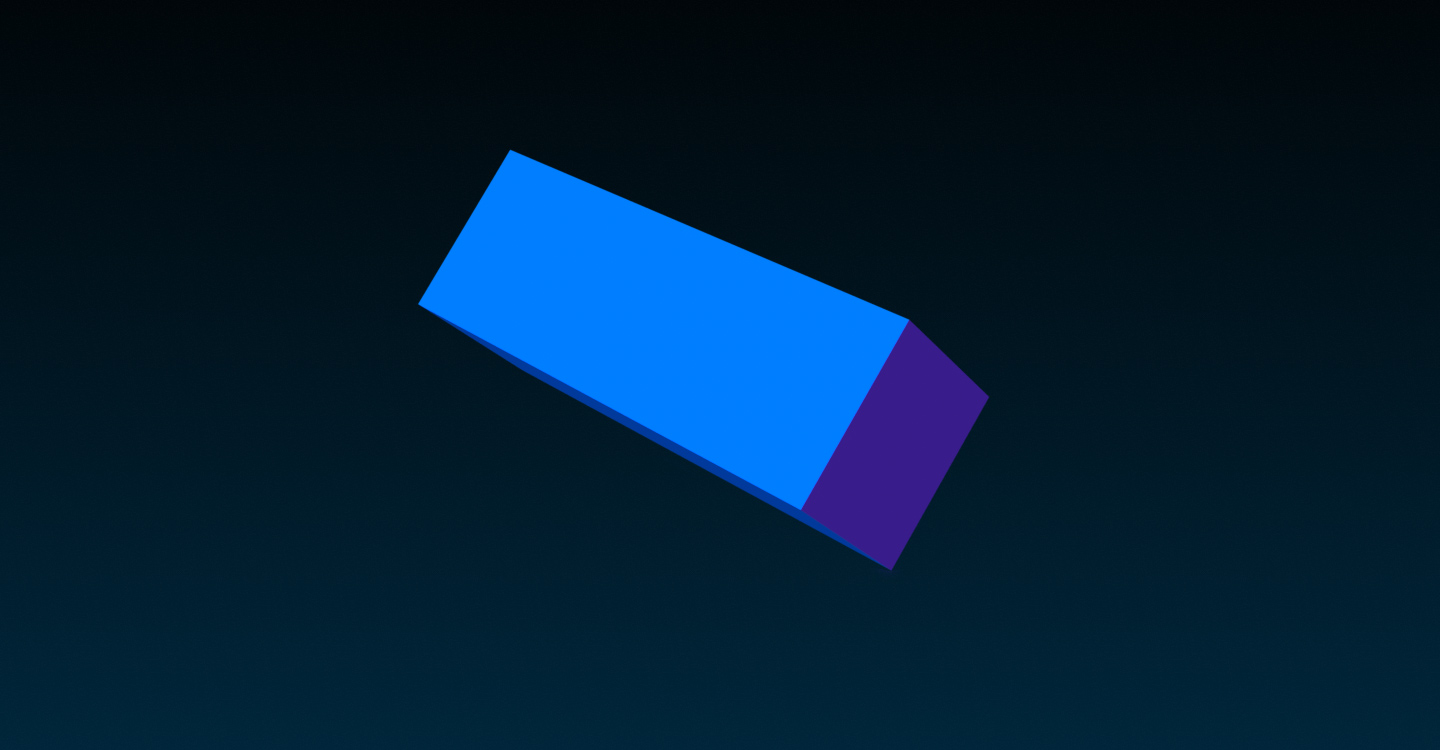
<figcaption>結果 <code>Class.PartOperation</code> ></figcaption>
注意
Class.BasePart:IntersectAsync() と比較して、このメソッドは以下のように異なります:
- 入力部分はスケーンに親化される必要がありません。これにより、バックグラウンドオペレーションが可能になります。
- SplitApart オプションが true (デフォルト) に設定されると、それぞれの異なるボディは自分の PartOperation に戻ります。
- 返された各パーツは、メインパーツのコーディネートスペースにあります。これは、返された任意のパーツの (0, 0, 0) が、メインパーツのボディの中心ではないことを意味します。
- クライアントでこのメソッドを呼び出すことはできますが、いくつかの制限があります。まず、現在クライアントにはオブジェクトが作成されている 上で 呼び出す必要があります。第二に、クライアントからサーバーへのレプリケーションは使用できません。
オリジナルのパーツは、成功したオペレーションの後に完全に残ります。ほとんどの場合、PartOperations をメインパーツと同じ場所に親にする必要があり、Class.Instance.Destroy|Destroy() すべてのオリジナルのパーツを破壊します。
デフォルトで、結果の PartOperations の顔の色は、元のパーツの Color プロパティから借りられますが、UsePartColor プロパティを有効にすると、特定の色に変更できます。
交差操作が 20,000 以上の三角形を含む場合、それらは PartOperations に単純化されます。これにより、コード -14 でエラーが発生します。
メイン部分がオペレーションの計算中に移動する場合は、返された部分をメイン部分の更新された CFrame に設定できます。メイン部分と同じコーディネートスペースにあるため、返された部分はメイン部分と同じコーディネートスペースにあります。
このメソッドをメインパーツとして使用する場合、Class.PartOperation
パラメータ
メイン Part または PartOperation を操作します。
メイン部分とインターセクトするパーツのアレイ。
メソッドのコントロールが含まれるオプションのテーブル:
- CollisionFidelity – 結果のパーツで CollisionFidelity の値。
- RenderFidelity – 結果のパーツで RenderFidelity の値。
- FluidFidelity – 結果のパーツで FluidFidelity の値。
- SplitApart – オブジェクトがすべて一緒に保持されるかどうかを制御します。デフォルトは true (スプリット) です。
戻り値
主要部分 ( PartOperations ) と他の部分の交差点の中の 1つまたは複数の part 。
コードサンプル
local GeometryService = game:GetService("GeometryService")
local mainPart = workspace.PurpleBlock
local otherParts = {workspace.BlueBlock}
local options = {
CollisionFidelity = Enum.CollisionFidelity.Default,
RenderFidelity = Enum.RenderFidelity.Automatic,
SplitApart = true
}
-- Perform intersect operation in pcall() since it's asyncronous
local success, newParts = pcall(function()
return GeometryService:IntersectAsync(mainPart, otherParts, options)
end)
if success and newParts then
-- Loop through resulting parts to reparent/reposition
for _, newPart in pairs(newParts) do
newPart.Parent = mainPart.Parent
newPart.CFrame = mainPart.CFrame
newPart.Anchored = mainPart.Anchored
end
-- Destroy original parts
mainPart.Parent = nil
mainPart:Destroy()
for _, otherPart in pairs(otherParts) do
otherPart.Parent = nil
otherPart:Destroy()
end
end
SubtractAsync
主要なパーツのミナスにある他のパーツのジオメトリを含む、PartOperations を 1 つまたは複数作成します。Parts
メイン部分の次のプロパティ ( part ) は、結果となる PartOperations に適用されます:
- Class.BasePart.Color|Color , Material , MaterialVariant , 0> Class.BasePart.Reflectance|Reflectance0> , Color3>
- Class.BasePart.Anchored|Anchored , Density , Elasticity , 0> Class.BasePart.ElasticityWeight|Elasticity0> , 3> Class.BasePart.Fr
次の画像比較では、 SubtractAsync() は、青いシリンダーとパープルブロックを含むアレイを使用して呼び出されます。結果として、 PartOperation は、シリンダーのジオメトリからブロックのジオメトリを削除する形式に変換されます。
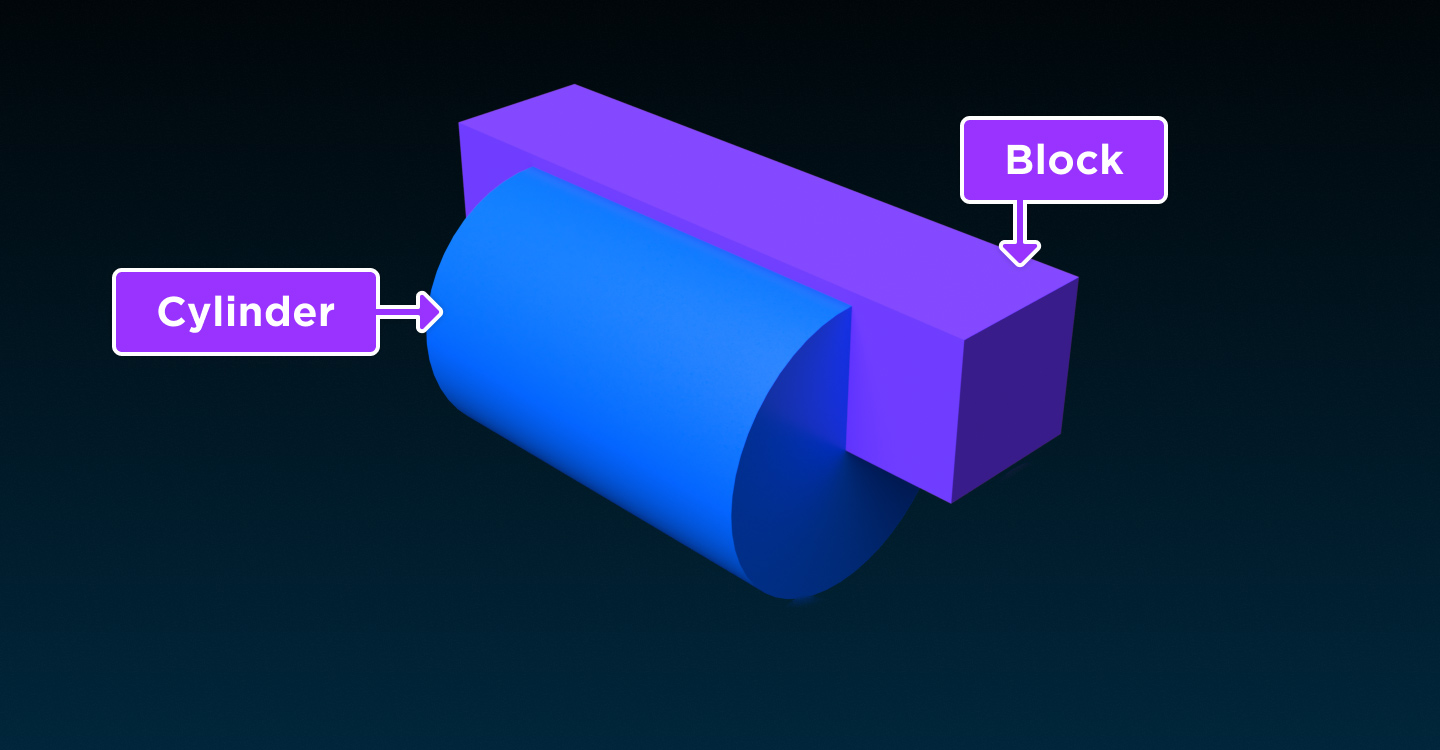
<figcaption>パーツを別々にする</figcaption>
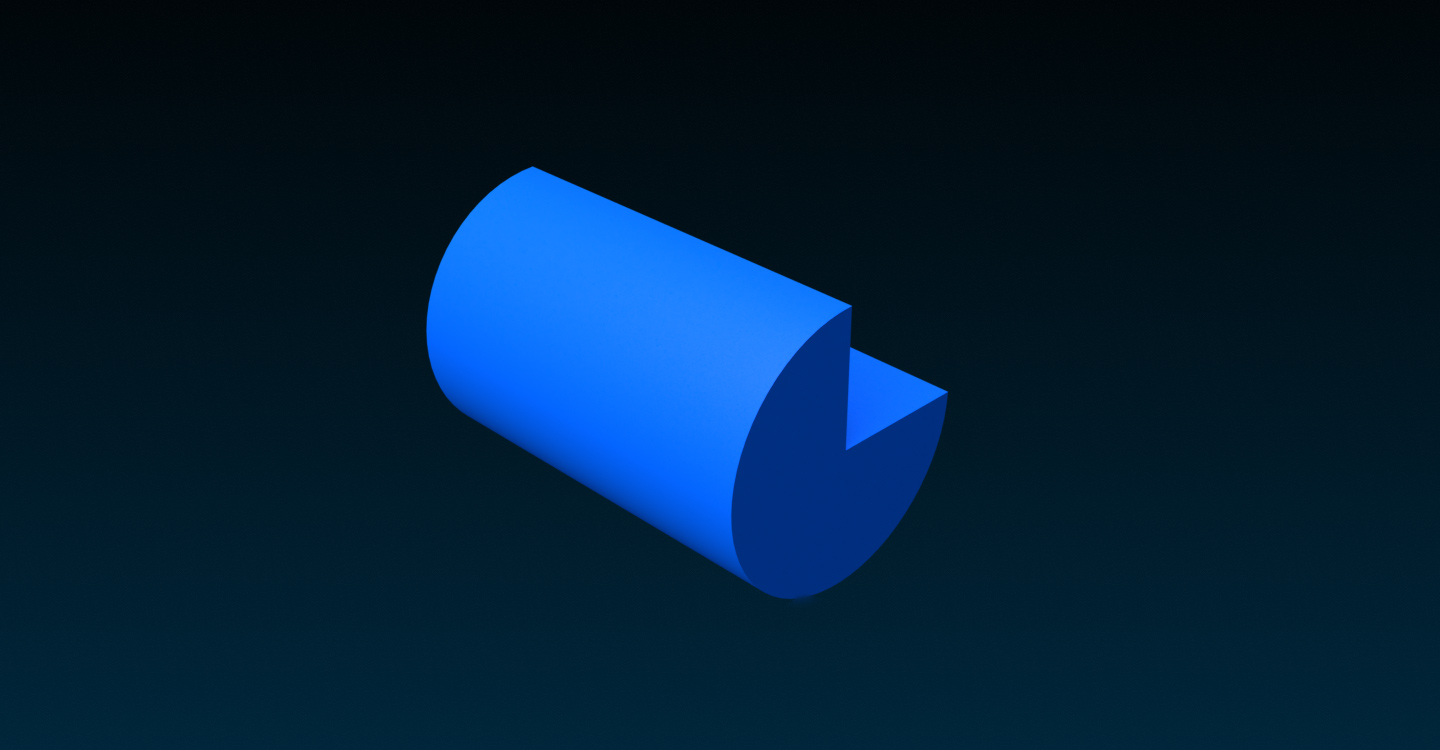
<figcaption>結果 <code>Class.PartOperation</code> ></figcaption>
注意
Class.BasePart:SubtractAsync() と比較して、このメソッドは以下のように異なります:
- 入力部分はスケーンに親化される必要がありません。これにより、バックグラウンドオペレーションが可能になります。
- SplitApart オプションが true (デフォルト) に設定されると、それぞれの異なるボディは自分の PartOperation に戻ります。
- 返された各パーツは、メインパーツのコーディネートスペースにあります。これは、返された任意のパーツの (0, 0, 0) が、メインパーツのボディの中心ではないことを意味します。
- クライアントでこのメソッドを呼び出すことはできますが、いくつかの制限があります。まず、現在クライアントにはオブジェクトが作成されている 上で 呼び出す必要があります。第二に、クライアントからサーバーへのレプリケーションは使用できません。
オリジナルのパーツは、成功したオペレーションの後に完全に残ります。ほとんどの場合、PartOperations をメインパーツと同じ場所に親にする必要があり、Class.Instance.Destroy|Destroy() すべてのオリジナルのパーツを破壊します。
デフォルトで、結果の PartOperations の顔の色は、元のパーツの Color プロパティから借りられますが、UsePartColor プロパティを有効にすると、特定の色に変更できます。
Class.PartOperation|PartOperations 以上のトライアングルを含む サブトラクトオペレーションが結果として 20,000 以上のトライアングルを含む場合、-14 コードでエラーが発生します。これにより、コード -14 のエラーが発生します。
メイン部分がオペレーションの計算中に移動する場合は、返された部分をメイン部分の更新された CFrame に設定できます。メイン部分と同じコーディネートスペースにあるため、返された部分はメイン部分と同じコーディネートスペースにあります。
このメソッドをメインパーツとして使用する場合、Class.PartOperation
パラメータ
メイン Part または PartOperation を操作します。
メイン部分から控除するパーツの配列。
メソッドのコントロールが含まれるオプションのテーブル:
- CollisionFidelity – 結果のパーツで CollisionFidelity の値。
- RenderFidelity – 結果のパーツで RenderFidelity の値。
- FluidFidelity – 結果のパーツで FluidFidelity の値。
- SplitApart – オブジェクトがすべて一緒に保持されるかどうかを制御します。デフォルトは true (スプリット) です。
戻り値
メイン部分のジオメトリから PartOperations を 1つまたは複数、他のパーツのジオメトリを控除してください。
コードサンプル
local GeometryService = game:GetService("GeometryService")
local mainPart = workspace.BlueCylinder
local otherParts = {workspace.PurpleBlock}
local options = {
CollisionFidelity = Enum.CollisionFidelity.Default,
RenderFidelity = Enum.RenderFidelity.Automatic,
SplitApart = true
}
-- Perform subtract operation in pcall() since it's asyncronous
local success, newParts = pcall(function()
return GeometryService:SubtractAsync(mainPart, otherParts, options)
end)
if success and newParts then
-- Loop through resulting parts to reparent/reposition
for _, newPart in pairs(newParts) do
newPart.Parent = mainPart.Parent
newPart.CFrame = mainPart.CFrame
newPart.Anchored = mainPart.Anchored
end
-- Destroy original parts
mainPart.Parent = nil
mainPart:Destroy()
for _, otherPart in pairs(otherParts) do
otherPart.Parent = nil
otherPart:Destroy()
end
end
UnionAsync
主要部分とその他のパーツのジオメトリを含むグループ化されたパーツを 1つまたは複数作成します。 主要部分のみがサポートされ、 Class.Terr
メイン部分の次のプロパティ ( part ) は、結果となる PartOperations に適用されます:
- Class.BasePart.Color|Color , Material , MaterialVariant , 0> Class.BasePart.Reflectance|Reflectance0> , Color3>
- Class.BasePart.Anchored|Anchored , Density , Elasticity , 0> Class.BasePart.ElasticityWeight|Elasticity0> , 3> Class.BasePart.Fr
次の画像比較では、 UnionAsync() は、青いブロックと紫のシリンダーを含むアレイを使用して呼び出されます。結果として、 PartOperation は、両方のパーツの共同ギオメトリの形式に変換されます。
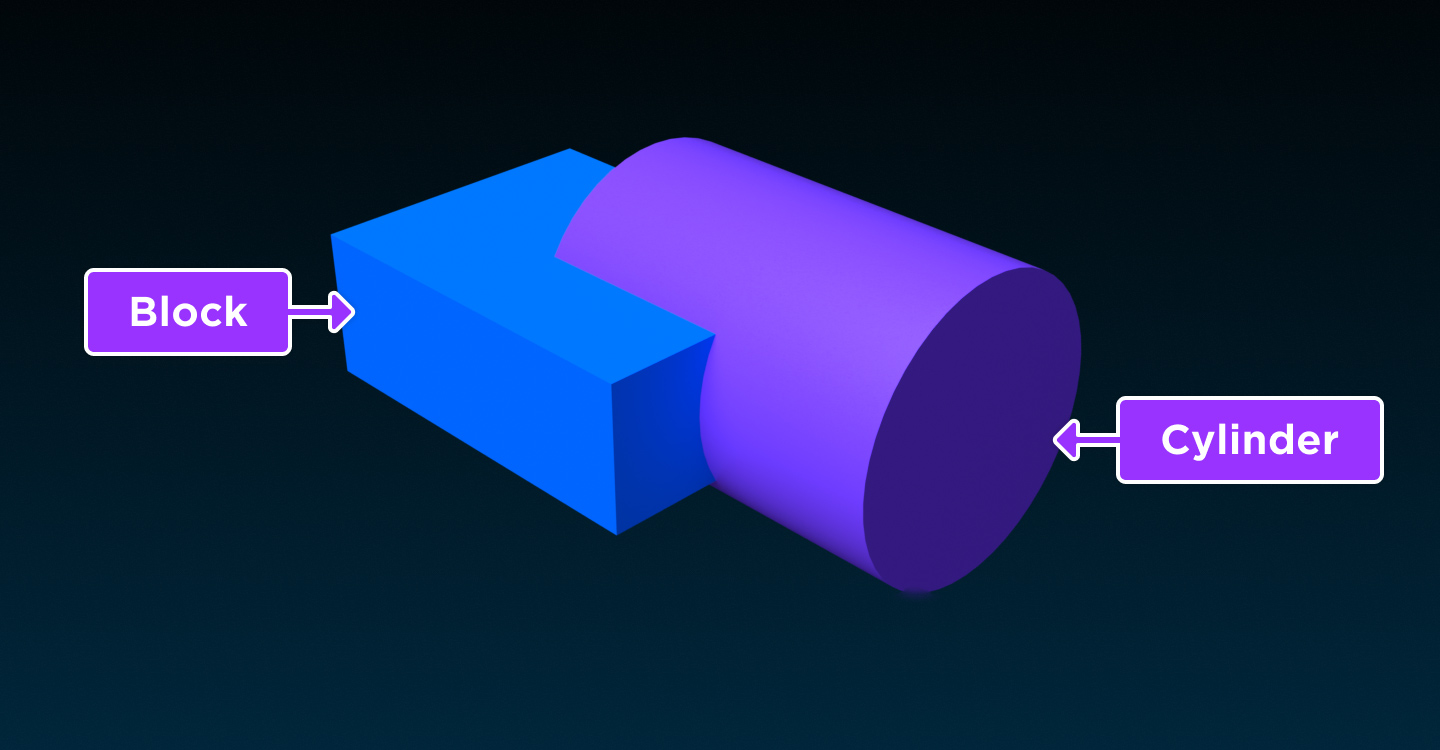
<figcaption>パーツを別々にする</figcaption>
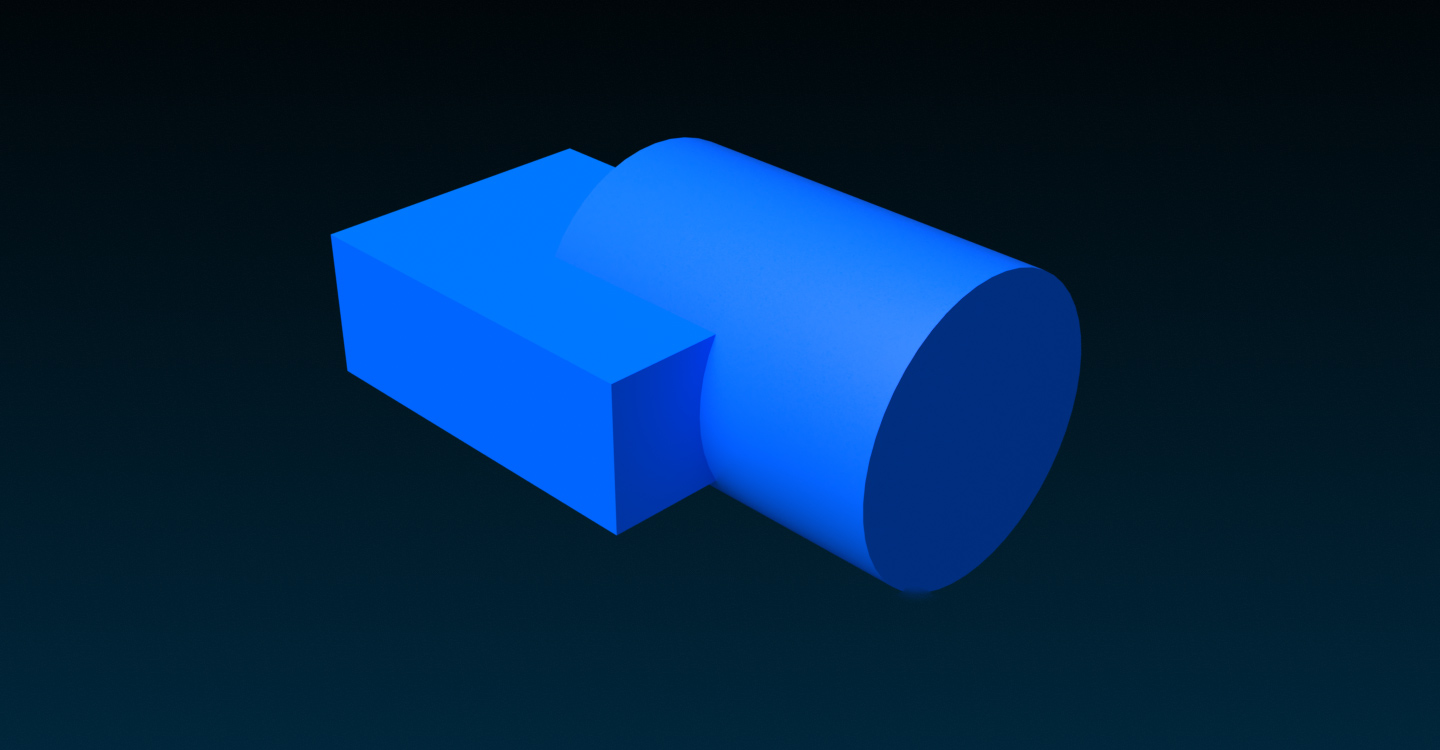
<figcaption>結果 <code>Class.PartOperation</code> ></figcaption>
注意
Class.BasePart:UnionAsync() と比較して、このメソッドは以下のように異なります:
- 入力部分はスケーンに親化される必要がありません。これにより、バックグラウンドオペレーションが可能になります。
- SplitApart オプションが true (デフォルト) に設定されると、それぞれの異なるボディは自分の PartOperation に戻ります。
- 返された各パーツは、メインパーツのコーディネートスペースにあります。これは、返された任意のパーツの (0, 0, 0) が、メインパーツのボディの中心ではないことを意味します。
- クライアントでこのメソッドを呼び出すことはできますが、いくつかの制限があります。まず、現在クライアントにはオブジェクトが作成されている 上で 呼び出す必要があります。第二に、クライアントからサーバーへのレプリケーションは使用できません。
オリジナルのパーツは、成功したオペレーションの後に完全に残ります。ほとんどの場合、PartOperations をメインパーツと同じ場所に親にする必要があり、Class.Instance.Destroy|Destroy() すべてのオリジナルのパーツを破壊します。
デフォルトでは、結果の PartOperations の色は、元のパーツの Color プロパティから借りられますが、UsePartColor プロパティを有効にすると、特定の色に変更できます。
ユニオンオペレーションが 20,000 以上の三角形を含む <a href="/reference/engine/databases">データベース</a> に結果を示す場合、それらは 20,000 に単純化されます。これにより、コード <a href="/reference/engine/databases">-14</a> でエラーが発生します。
メイン部分がオペレーションの計算中に移動する場合は、返された部分をメイン部分の更新された CFrame に設定できます。メイン部分と同じコーディネートスペースにあるため、返された部分はメイン部分と同じコーディネートスペースにあります。
このメソッドをメインパーツとして使用する場合、Class.PartOperation
パラメータ
メイン Part または PartOperation を操作します。
メイン部分とユニオンするパーツの配列。
メソッドのコントロールが含まれるオプションのテーブル:
- CollisionFidelity – 結果のパーツで CollisionFidelity の値。
- RenderFidelity – 結果のパーツで RenderFidelity の値。
- FluidFidelity – 結果のパーツで FluidFidelity の値。
- SplitApart – オブジェクトがすべて一緒に保持されるかどうかを制御します。デフォルトは true (スプリット) です。
戻り値
メイン部分のジオメトリと他のパーツのジオメトリの 1つまたは複数の PartOperations 。
コードサンプル
local GeometryService = game:GetService("GeometryService")
local mainPart = workspace.BlueBlock
local otherParts = {workspace.PurpleCylinder}
local options = {
CollisionFidelity = Enum.CollisionFidelity.Default,
RenderFidelity = Enum.RenderFidelity.Automatic,
SplitApart = false
}
-- Perform union operation in pcall() since it's asyncronous
local success, newParts = pcall(function()
return GeometryService:UnionAsync(mainPart, otherParts, options)
end)
if success and newParts then
-- Loop through resulting parts to reparent/reposition
for _, newPart in pairs(newParts) do
newPart.Parent = mainPart.Parent
newPart.CFrame = mainPart.CFrame
newPart.Anchored = mainPart.Anchored
end
-- Destroy original parts
mainPart.Parent = nil
mainPart:Destroy()
for _, otherPart in pairs(otherParts) do
otherPart.Parent = nil
otherPart:Destroy()
end
end