AudioChannelMixer
*Ce contenu est traduit en utilisant l'IA (Beta) et peut contenir des erreurs. Pour consulter cette page en anglais, clique ici.
AudioChannelMixer mélange plusieurs flux audio en un seul flux multicanal.Il fournit un connecteur combiné entrée pin, un connecteur sortie pin, ainsi que les pins d'entrée secondaire suivants, tous lesquels peuvent être connectés à/de Wires : Gauche , Droite , Centre , SurroundLeft , SurroundRight , Sub , ArrièreGauche , ArrièreDroite , HautGauche , HautDroite , HautGaucheSud , et HautDroiteSud .
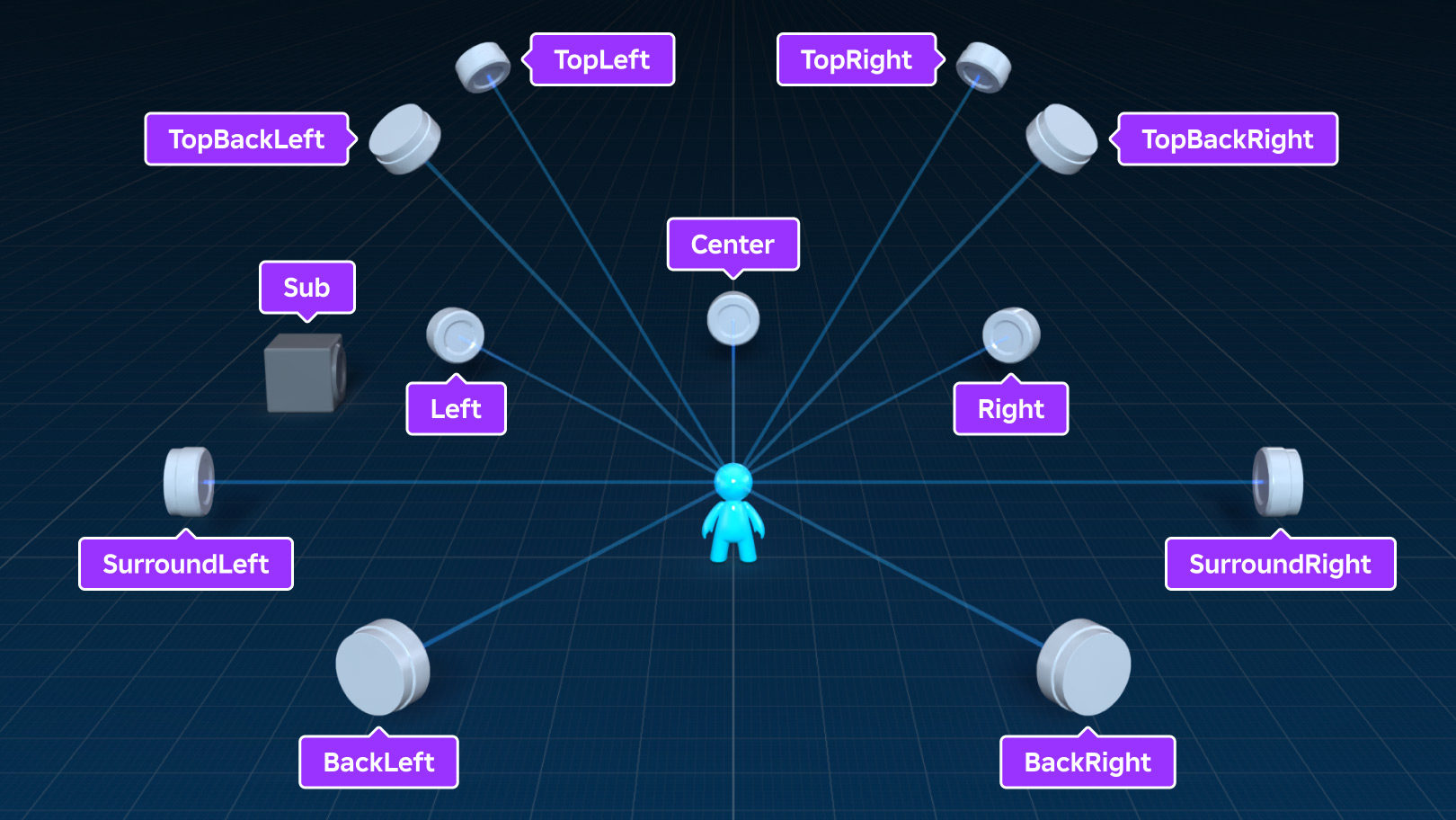
Échantillons de code
local Workspace = game:GetService("Workspace")
local function wireUp(source : Instance, target : Instance, sourceName : string?, targetName : string?)
local wire = Instance.new("Wire", source)
wire.SourceInstance = source
wire.TargetInstance = target
if sourceName then wire.SourceName = sourceName end
if targetName then wire.TargetName = targetName end
return wire
end
local listener = Instance.new("AudioListener")
listener.Parent = Workspace.CurrentCamera
local output = Instance.new("AudioDeviceOutput")
output.Parent = Workspace
local splitter = Instance.new("AudioChannelSplitter")
splitter.Parent = Workspace
local mixer = Instance.new("AudioChannelMixer")
mixer.Parent = Workspace
-- Send what the listener hears to a splitter and send a mix to the final output
wireUp(listener, splitter)
wireUp(mixer, output)
-- Set up both the splitter and mixer to use a quadrophonic layout
splitter.Layout = Enum.AudioChannelLayout.Quad
mixer.Layout = Enum.AudioChannelLayout.Quad
-- Give each of the four channels its own pitch shifter
local frontLeft = Instance.new("AudioPitchShifter")
frontLeft.Name = "Front Left"
frontLeft.Pitch = 1.25
frontLeft.Parent = Workspace
local backLeft = Instance.new("AudioPitchShifter")
backLeft.Name = "Back Left"
backLeft.Pitch = 0.5
backLeft.Parent = Workspace
local frontRight = Instance.new("AudioPitchShifter")
frontRight.Name = "Front Right"
frontRight.Pitch = 1.5
frontRight.Parent = Workspace
local backRight = Instance.new("AudioPitchShifter")
backRight.Name = "Back Right"
backRight.Pitch = 0.75
backRight.Parent = Workspace
wireUp(splitter, frontLeft, "Left")
wireUp(splitter, backLeft, "BackLeft")
wireUp(splitter, frontRight, "Right")
wireUp(splitter, backRight, "BackRight")
wireUp(frontLeft, mixer, nil, "Left")
wireUp(backLeft, mixer, nil, "BackLeft")
wireUp(frontRight, mixer, nil, "Right")
wireUp(backRight, mixer, nil, "BackRight")
-- Configure a part to emit audio
local part = Instance.new("Part")
part.Shape = Enum.PartType.Ball
part.Size = Vector3.new(4, 4, 4)
part.Material = Enum.Material.SmoothPlastic
part.CastShadow = false
part.Position = Vector3.new(0, 4, -12)
part.Anchored = true
part.Parent = Workspace
local analyzer = Instance.new("AudioAnalyzer")
analyzer.Parent = part
local emitter = Instance.new("AudioEmitter")
emitter.Parent = part
local assetPlayer = Instance.new("AudioPlayer")
assetPlayer.Looping = true
assetPlayer.Asset = "rbxassetid://97799489309320"
assetPlayer.Parent = emitter
wireUp(assetPlayer, emitter)
wireUp(assetPlayer, analyzer)
-- Start playing the audio
assetPlayer:Play()
-- Adjust the part's color as the audio plays
while true do
local peak = math.sqrt(analyzer.PeakLevel)
part.Color = Color3.new(peak, peak, peak)
task.wait()
end
Résumé
Propriétés
Contrôle la disposition du canal de sortie à mélanger.
Méthodes
Renvoie un tableau de Wires qui sont connectés au pin spécifié.
Retourne les broches d'entrée qui peuvent être sélectionnées par Wire.TargetName.
Renvoie l'épingle de sortie disponible pour Wire.SourceName .
Évènements
Se déclenche lorsqu'une autre instance est connectée ou déconnectée du AudioChannelMixer via un Wire .
Propriétés
Layout
Contrôle la disposition du canal de sortie à mélanger.La broche d'entrée de est toujours envoyée "telle quelle" au sortie , mais en fonction de la valeur de >:
- Pour Mono, l'épingle du centre consomme des flux audio.
- Pour Quad , le Gauche , Droite , ArrièreGauche et ArrièreDroite les épingles consomment des flux audio.
- Surround_7_1 est identique à Surround_5_1 , plus SurroundLeft et SurroundRight consomment des flux audio.
- Pour Surround_7_1_4, toutes les broches d'entrée secondaire consomment des flux audio.
Méthodes
GetConnectedWires
Renvoie un tableau de Wires qui sont connectés au pin spécifié.
Paramètres
Retours
GetInputPins
Renvoie une table de chaînes indiquant quelles épingles d'entrée sont disponibles pour Wire.TargetName :
- "Input"
- "Left"
- "Right"
- "Center"
- "SurroundLeft"
- "SurroundRight"
- "BackLeft"
- "BackRight"
- "Sub"
- "TopLeft"
- "TopRight"
- "TopBackLeft"
- "TopBackRight"
Retours
GetOutputPins
Renvoie une table contenant une chaîne, "Output" , indiquant l'épingle de sortie disponible pour Wire.SourceName .
Retours
Évènements
WiringChanged
Événement qui se déclenche après qu'un Wire devienne connecté ou déconnecté, et que Wire soit maintenant ou ait été précédemment connecté à un bouton sur le AudioChannelMixer et à une autre instance wirable.
Paramètres
Si l'instance s'est connectée ou déconnectée.
L'épingle sur le AudioChannelMixer que les cibles Wire .
Le Wire entre la AudioChannelMixer et l'autre instance.