근접 프롬프트 인스턴스를 사용하면 플레이어에게 3D 세계의 개체와 상호작용하도록 요청할 수 있습니다(예: 문 열기 또는 아이템 집기).부모로 지정된 A ProximityPrompt 개체는 작업 영역에서 BasePart , Attachment 또는 Model (PrimaryPart 설정)에 대해 작동합니다.플레이어의 캐릭터가 접근하면 UI가 나타나서 입력을 요청합니다.
프롬프트는 주요 요소 3개로 구성되며 각각 지정된 속성으로 제어할 수 있습니다.기본 UI는 Style에 설명된 대로 사용자 지정 모양으로 교체할 수 있습니다.
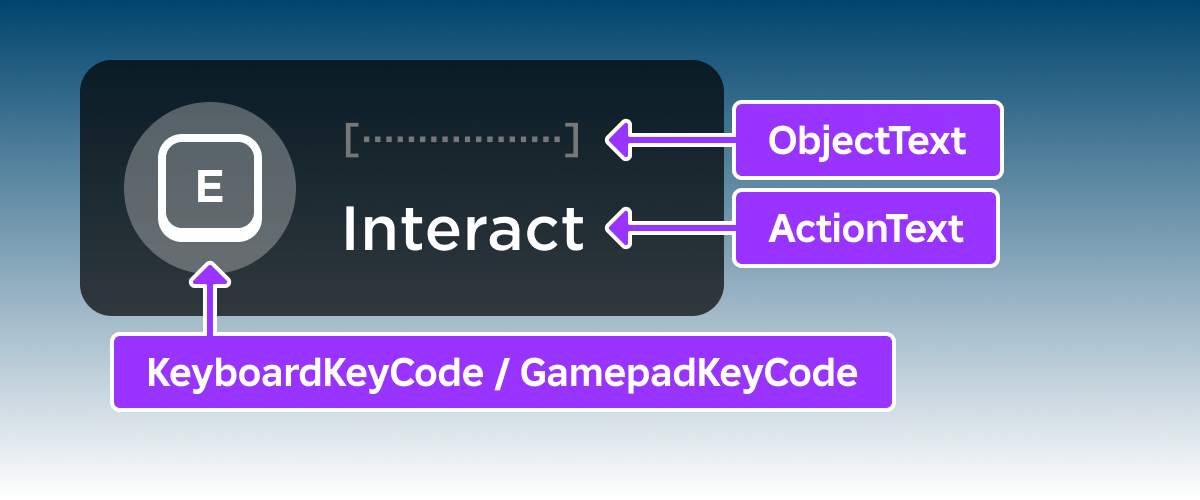
<td>설명</td><td>기본</td></tr></thead><tbody><tr><td><code>Class.ProximityPrompt.ObjectText|ObjectText</code></td><td>상호 작용하는 개체의 선택적 이름입니다.</td><td /></tr><tr><td><code>Class.ProximityPrompt.ActionText|액션텍스트</code></td><td>플레이어에게 표시되는 선택적 작업 이름입니다.</td><td>상호작용</td></tr><tr><td><code>Class.ProximityPrompt.KeyboardKeyCode|KeyboardKeyCode</code></td><td>프롬프트를 트리거할 키보드 키.</td><td>E</td></tr><tr><td><code>Class.ProximityPrompt.GamepadKeyCode|게임패드키코드</code></td><td>프롬프트를 트리거할 게임패드 버튼.</td><td>버튼X</td></tr></tbody>
속성 |
---|
근접 프롬프트 이벤트에 연결할 수 있는 방법은 다음과 같습니다. ProximityPrompt 개체 자체 또는 전역적으로 ProximityPromptService을 통해 연결할 수 있습니다.ProximityPromptService 는 모든 근접 프롬프트 동작을 한 위치에서 관리할 수 있으므로 경험에서 중복 코드가 필요하지 않습니다.
근접 프롬프트에 대한 자세한 정보는 근접 프롬프트 가이드를 참조하십시오.
코드 샘플
In the example below, a user must interact with a chair to sit in it. Paste this into a Script that is a child of a ProximityPrompt, which is itself a sibling of a Seat object named Seat.
local proximityPrompt = script.Parent
local seat = proximityPrompt.Parent.Seat
seat:GetPropertyChangedSignal("Occupant"):Connect(function()
if seat.Occupant then
proximityPrompt.Enabled = false
else
proximityPrompt.Enabled = true
end
end)
proximityPrompt.Triggered:Connect(function(player)
seat:Sit(player.Character.Humanoid)
end)
The example below generates a custom ProximityPrompt UI similar to the default style, which you may use as a starting point. It should be used in a LocalScript.
local UserInputService = game:GetService("UserInputService")
local ProximityPromptService = game:GetService("ProximityPromptService")
local TweenService = game:GetService("TweenService")
local TextService = game:GetService("TextService")
local Players = game:GetService("Players")
local LocalPlayer = Players.LocalPlayer
local PlayerGui = LocalPlayer:WaitForChild("PlayerGui")
local GamepadButtonImage = {
[Enum.KeyCode.ButtonX] = "rbxasset://textures/ui/Controls/xboxX.png",
[Enum.KeyCode.ButtonY] = "rbxasset://textures/ui/Controls/xboxY.png",
[Enum.KeyCode.ButtonA] = "rbxasset://textures/ui/Controls/xboxA.png",
[Enum.KeyCode.ButtonB] = "rbxasset://textures/ui/Controls/xboxB.png",
[Enum.KeyCode.DPadLeft] = "rbxasset://textures/ui/Controls/dpadLeft.png",
[Enum.KeyCode.DPadRight] = "rbxasset://textures/ui/Controls/dpadRight.png",
[Enum.KeyCode.DPadUp] = "rbxasset://textures/ui/Controls/dpadUp.png",
[Enum.KeyCode.DPadDown] = "rbxasset://textures/ui/Controls/dpadDown.png",
[Enum.KeyCode.ButtonSelect] = "rbxasset://textures/ui/Controls/xboxView.png",
[Enum.KeyCode.ButtonStart] = "rbxasset://textures/ui/Controls/xboxmenu.png",
[Enum.KeyCode.ButtonL1] = "rbxasset://textures/ui/Controls/xboxLB.png",
[Enum.KeyCode.ButtonR1] = "rbxasset://textures/ui/Controls/xboxRB.png",
[Enum.KeyCode.ButtonL2] = "rbxasset://textures/ui/Controls/xboxLT.png",
[Enum.KeyCode.ButtonR2] = "rbxasset://textures/ui/Controls/xboxRT.png",
[Enum.KeyCode.ButtonL3] = "rbxasset://textures/ui/Controls/xboxLS.png",
[Enum.KeyCode.ButtonR3] = "rbxasset://textures/ui/Controls/xboxRS.png",
[Enum.KeyCode.Thumbstick1] = "rbxasset://textures/ui/Controls/xboxLSDirectional.png",
[Enum.KeyCode.Thumbstick2] = "rbxasset://textures/ui/Controls/xboxRSDirectional.png",
}
local KeyboardButtonImage = {
[Enum.KeyCode.Backspace] = "rbxasset://textures/ui/Controls/backspace.png",
[Enum.KeyCode.Return] = "rbxasset://textures/ui/Controls/return.png",
[Enum.KeyCode.LeftShift] = "rbxasset://textures/ui/Controls/shift.png",
[Enum.KeyCode.RightShift] = "rbxasset://textures/ui/Controls/shift.png",
[Enum.KeyCode.Tab] = "rbxasset://textures/ui/Controls/tab.png",
}
local KeyboardButtonIconMapping = {
["'"] = "rbxasset://textures/ui/Controls/apostrophe.png",
[","] = "rbxasset://textures/ui/Controls/comma.png",
["`"] = "rbxasset://textures/ui/Controls/graveaccent.png",
["."] = "rbxasset://textures/ui/Controls/period.png",
[" "] = "rbxasset://textures/ui/Controls/spacebar.png",
}
local KeyCodeToTextMapping = {
[Enum.KeyCode.LeftControl] = "Ctrl",
[Enum.KeyCode.RightControl] = "Ctrl",
[Enum.KeyCode.LeftAlt] = "Alt",
[Enum.KeyCode.RightAlt] = "Alt",
[Enum.KeyCode.F1] = "F1",
[Enum.KeyCode.F2] = "F2",
[Enum.KeyCode.F3] = "F3",
[Enum.KeyCode.F4] = "F4",
[Enum.KeyCode.F5] = "F5",
[Enum.KeyCode.F6] = "F6",
[Enum.KeyCode.F7] = "F7",
[Enum.KeyCode.F8] = "F8",
[Enum.KeyCode.F9] = "F9",
[Enum.KeyCode.F10] = "F10",
[Enum.KeyCode.F11] = "F11",
[Enum.KeyCode.F12] = "F12",
}
local function getScreenGui()
local screenGui = PlayerGui:FindFirstChild("ProximityPrompts")
if screenGui == nil then
screenGui = Instance.new("ScreenGui")
screenGui.Name = "ProximityPrompts"
screenGui.ResetOnSpawn = false
screenGui.Parent = PlayerGui
end
return screenGui
end
local function createProgressBarGradient(parent, leftSide)
local frame = Instance.new("Frame")
frame.Size = UDim2.fromScale(0.5, 1)
frame.Position = UDim2.fromScale(leftSide and 0 or 0.5, 0)
frame.BackgroundTransparency = 1
frame.ClipsDescendants = true
frame.Parent = parent
local image = Instance.new("ImageLabel")
image.BackgroundTransparency = 1
image.Size = UDim2.fromScale(2, 1)
image.Position = UDim2.fromScale(leftSide and 0 or -1, 0)
image.Image = "rbxasset://textures/ui/Controls/RadialFill.png"
image.Parent = frame
local gradient = Instance.new("UIGradient")
gradient.Transparency = NumberSequence.new({
NumberSequenceKeypoint.new(0, 0),
NumberSequenceKeypoint.new(0.4999, 0),
NumberSequenceKeypoint.new(0.5, 1),
NumberSequenceKeypoint.new(1, 1),
})
gradient.Rotation = leftSide and 180 or 0
gradient.Parent = image
return gradient
end
local function createCircularProgressBar()
local bar = Instance.new("Frame")
bar.Name = "CircularProgressBar"
bar.Size = UDim2.fromOffset(58, 58)
bar.AnchorPoint = Vector2.new(0.5, 0.5)
bar.Position = UDim2.fromScale(0.5, 0.5)
bar.BackgroundTransparency = 1
local gradient1 = createProgressBarGradient(bar, true)
local gradient2 = createProgressBarGradient(bar, false)
local progress = Instance.new("NumberValue")
progress.Name = "Progress"
progress.Parent = bar
progress.Changed:Connect(function(value)
local angle = math.clamp(value * 360, 0, 360)
gradient1.Rotation = math.clamp(angle, 180, 360)
gradient2.Rotation = math.clamp(angle, 0, 180)
end)
return bar
end
local function createPrompt(prompt, inputType, gui)
local tweensForButtonHoldBegin = {}
local tweensForButtonHoldEnd = {}
local tweensForFadeOut = {}
local tweensForFadeIn = {}
local tweenInfoInFullDuration =
TweenInfo.new(prompt.HoldDuration, Enum.EasingStyle.Linear, Enum.EasingDirection.Out)
local tweenInfoOutHalfSecond = TweenInfo.new(0.5, Enum.EasingStyle.Quad, Enum.EasingDirection.Out)
local tweenInfoFast = TweenInfo.new(0.2, Enum.EasingStyle.Quad, Enum.EasingDirection.Out)
local tweenInfoQuick = TweenInfo.new(0.06, Enum.EasingStyle.Linear, Enum.EasingDirection.Out)
local promptUI = Instance.new("BillboardGui")
promptUI.Name = "Prompt"
promptUI.AlwaysOnTop = true
local frame = Instance.new("Frame")
frame.Size = UDim2.fromScale(0.5, 1)
frame.BackgroundTransparency = 1
frame.BackgroundColor3 = Color3.new(0.07, 0.07, 0.07)
frame.Parent = promptUI
local roundedCorner = Instance.new("UICorner")
roundedCorner.Parent = frame
local inputFrame = Instance.new("Frame")
inputFrame.Name = "InputFrame"
inputFrame.Size = UDim2.fromScale(1, 1)
inputFrame.BackgroundTransparency = 1
inputFrame.SizeConstraint = Enum.SizeConstraint.RelativeYY
inputFrame.Parent = frame
local resizeableInputFrame = Instance.new("Frame")
resizeableInputFrame.Size = UDim2.fromScale(1, 1)
resizeableInputFrame.Position = UDim2.fromScale(0.5, 0.5)
resizeableInputFrame.AnchorPoint = Vector2.new(0.5, 0.5)
resizeableInputFrame.BackgroundTransparency = 1
resizeableInputFrame.Parent = inputFrame
local inputFrameScaler = Instance.new("UIScale")
inputFrameScaler.Parent = resizeableInputFrame
local inputFrameScaleFactor = inputType == Enum.ProximityPromptInputType.Touch and 1.6 or 1.33
table.insert(
tweensForButtonHoldBegin,
TweenService:Create(inputFrameScaler, tweenInfoFast, { Scale = inputFrameScaleFactor })
)
table.insert(tweensForButtonHoldEnd, TweenService:Create(inputFrameScaler, tweenInfoFast, { Scale = 1 }))
local actionText = Instance.new("TextLabel")
actionText.Name = "ActionText"
actionText.Size = UDim2.fromScale(1, 1)
actionText.Font = Enum.Font.GothamMedium
actionText.TextSize = 19
actionText.BackgroundTransparency = 1
actionText.TextTransparency = 1
actionText.TextColor3 = Color3.new(1, 1, 1)
actionText.TextXAlignment = Enum.TextXAlignment.Left
actionText.Parent = frame
table.insert(tweensForButtonHoldBegin, TweenService:Create(actionText, tweenInfoFast, { TextTransparency = 1 }))
table.insert(tweensForButtonHoldEnd, TweenService:Create(actionText, tweenInfoFast, { TextTransparency = 0 }))
table.insert(tweensForFadeOut, TweenService:Create(actionText, tweenInfoFast, { TextTransparency = 1 }))
table.insert(tweensForFadeIn, TweenService:Create(actionText, tweenInfoFast, { TextTransparency = 0 }))
local objectText = Instance.new("TextLabel")
objectText.Name = "ObjectText"
objectText.Size = UDim2.fromScale(1, 1)
objectText.Font = Enum.Font.GothamMedium
objectText.TextSize = 14
objectText.BackgroundTransparency = 1
objectText.TextTransparency = 1
objectText.TextColor3 = Color3.new(0.7, 0.7, 0.7)
objectText.TextXAlignment = Enum.TextXAlignment.Left
objectText.Parent = frame
table.insert(tweensForButtonHoldBegin, TweenService:Create(objectText, tweenInfoFast, { TextTransparency = 1 }))
table.insert(tweensForButtonHoldEnd, TweenService:Create(objectText, tweenInfoFast, { TextTransparency = 0 }))
table.insert(tweensForFadeOut, TweenService:Create(objectText, tweenInfoFast, { TextTransparency = 1 }))
table.insert(tweensForFadeIn, TweenService:Create(objectText, tweenInfoFast, { TextTransparency = 0 }))
table.insert(
tweensForButtonHoldBegin,
TweenService:Create(frame, tweenInfoFast, { Size = UDim2.fromScale(0.5, 1), BackgroundTransparency = 1 })
)
table.insert(
tweensForButtonHoldEnd,
TweenService:Create(frame, tweenInfoFast, { Size = UDim2.fromScale(1, 1), BackgroundTransparency = 0.2 })
)
table.insert(
tweensForFadeOut,
TweenService:Create(frame, tweenInfoFast, { Size = UDim2.fromScale(0.5, 1), BackgroundTransparency = 1 })
)
table.insert(
tweensForFadeIn,
TweenService:Create(frame, tweenInfoFast, { Size = UDim2.fromScale(1, 1), BackgroundTransparency = 0.2 })
)
local roundFrame = Instance.new("Frame")
roundFrame.Name = "RoundFrame"
roundFrame.Size = UDim2.fromOffset(48, 48)
roundFrame.AnchorPoint = Vector2.new(0.5, 0.5)
roundFrame.Position = UDim2.fromScale(0.5, 0.5)
roundFrame.BackgroundTransparency = 1
roundFrame.Parent = resizeableInputFrame
local roundedFrameCorner = Instance.new("UICorner")
roundedFrameCorner.CornerRadius = UDim.new(0.5, 0)
roundedFrameCorner.Parent = roundFrame
table.insert(tweensForFadeOut, TweenService:Create(roundFrame, tweenInfoQuick, { BackgroundTransparency = 1 }))
table.insert(tweensForFadeIn, TweenService:Create(roundFrame, tweenInfoQuick, { BackgroundTransparency = 0.5 }))
if inputType == Enum.ProximityPromptInputType.Gamepad then
if GamepadButtonImage[prompt.GamepadKeyCode] then
local icon = Instance.new("ImageLabel")
icon.Name = "ButtonImage"
icon.AnchorPoint = Vector2.new(0.5, 0.5)
icon.Size = UDim2.fromOffset(24, 24)
icon.Position = UDim2.fromScale(0.5, 0.5)
icon.BackgroundTransparency = 1
icon.ImageTransparency = 1
icon.Image = GamepadButtonImage[prompt.GamepadKeyCode]
icon.Parent = resizeableInputFrame
table.insert(tweensForFadeOut, TweenService:Create(icon, tweenInfoQuick, { ImageTransparency = 1 }))
table.insert(tweensForFadeIn, TweenService:Create(icon, tweenInfoQuick, { ImageTransparency = 0 }))
end
elseif inputType == Enum.ProximityPromptInputType.Touch then
local buttonImage = Instance.new("ImageLabel")
buttonImage.Name = "ButtonImage"
buttonImage.BackgroundTransparency = 1
buttonImage.ImageTransparency = 1
buttonImage.Size = UDim2.fromOffset(25, 31)
buttonImage.AnchorPoint = Vector2.new(0.5, 0.5)
buttonImage.Position = UDim2.fromScale(0.5, 0.5)
buttonImage.Image = "rbxasset://textures/ui/Controls/TouchTapIcon.png"
buttonImage.Parent = resizeableInputFrame
table.insert(tweensForFadeOut, TweenService:Create(buttonImage, tweenInfoQuick, { ImageTransparency = 1 }))
table.insert(tweensForFadeIn, TweenService:Create(buttonImage, tweenInfoQuick, { ImageTransparency = 0 }))
else
local buttonImage = Instance.new("ImageLabel")
buttonImage.Name = "ButtonImage"
buttonImage.BackgroundTransparency = 1
buttonImage.ImageTransparency = 1
buttonImage.Size = UDim2.fromOffset(28, 30)
buttonImage.AnchorPoint = Vector2.new(0.5, 0.5)
buttonImage.Position = UDim2.fromScale(0.5, 0.5)
buttonImage.Image = "rbxasset://textures/ui/Controls/key_single.png"
buttonImage.Parent = resizeableInputFrame
table.insert(tweensForFadeOut, TweenService:Create(buttonImage, tweenInfoQuick, { ImageTransparency = 1 }))
table.insert(tweensForFadeIn, TweenService:Create(buttonImage, tweenInfoQuick, { ImageTransparency = 0 }))
local buttonTextString = UserInputService:GetStringForKeyCode(prompt.KeyboardKeyCode)
local buttonTextImage = KeyboardButtonImage[prompt.KeyboardKeyCode]
if buttonTextImage == nil then
buttonTextImage = KeyboardButtonIconMapping[buttonTextString]
end
if buttonTextImage == nil then
local keyCodeMappedText = KeyCodeToTextMapping[prompt.KeyboardKeyCode]
if keyCodeMappedText then
buttonTextString = keyCodeMappedText
end
end
if buttonTextImage then
local icon = Instance.new("ImageLabel")
icon.Name = "ButtonImage"
icon.AnchorPoint = Vector2.new(0.5, 0.5)
icon.Size = UDim2.fromOffset(36, 36)
icon.Position = UDim2.fromScale(0.5, 0.5)
icon.BackgroundTransparency = 1
icon.ImageTransparency = 1
icon.Image = buttonTextImage
icon.Parent = resizeableInputFrame
table.insert(tweensForFadeOut, TweenService:Create(icon, tweenInfoQuick, { ImageTransparency = 1 }))
table.insert(tweensForFadeIn, TweenService:Create(icon, tweenInfoQuick, { ImageTransparency = 0 }))
elseif buttonTextString ~= nil and buttonTextString ~= "" then
local buttonText = Instance.new("TextLabel")
buttonText.Name = "ButtonText"
buttonText.Position = UDim2.fromOffset(0, -1)
buttonText.Size = UDim2.fromScale(1, 1)
buttonText.Font = Enum.Font.GothamMedium
buttonText.TextSize = 14
if string.len(buttonTextString) > 2 then
buttonText.TextSize = 12
end
buttonText.BackgroundTransparency = 1
buttonText.TextTransparency = 1
buttonText.TextColor3 = Color3.new(1, 1, 1)
buttonText.TextXAlignment = Enum.TextXAlignment.Center
buttonText.Text = buttonTextString
buttonText.Parent = resizeableInputFrame
table.insert(tweensForFadeOut, TweenService:Create(buttonText, tweenInfoQuick, { TextTransparency = 1 }))
table.insert(tweensForFadeIn, TweenService:Create(buttonText, tweenInfoQuick, { TextTransparency = 0 }))
else
error(
"ProximityPrompt '"
.. prompt.Name
.. "' has an unsupported keycode for rendering UI: "
.. tostring(prompt.KeyboardKeyCode)
)
end
end
if inputType == Enum.ProximityPromptInputType.Touch or prompt.ClickablePrompt then
local button = Instance.new("TextButton")
button.BackgroundTransparency = 1
button.TextTransparency = 1
button.Size = UDim2.fromScale(1, 1)
button.Parent = promptUI
local buttonDown = false
button.InputBegan:Connect(function(input)
if
(
input.UserInputType == Enum.UserInputType.Touch
or input.UserInputType == Enum.UserInputType.MouseButton1
) and input.UserInputState ~= Enum.UserInputState.Change
then
prompt:InputHoldBegin()
buttonDown = true
end
end)
button.InputEnded:Connect(function(input)
if
input.UserInputType == Enum.UserInputType.Touch
or input.UserInputType == Enum.UserInputType.MouseButton1
then
if buttonDown then
buttonDown = false
prompt:InputHoldEnd()
end
end
end)
promptUI.Active = true
end
if prompt.HoldDuration > 0 then
local circleBar = createCircularProgressBar()
circleBar.Parent = resizeableInputFrame
table.insert(
tweensForButtonHoldBegin,
TweenService:Create(circleBar.Progress, tweenInfoInFullDuration, { Value = 1 })
)
table.insert(
tweensForButtonHoldEnd,
TweenService:Create(circleBar.Progress, tweenInfoOutHalfSecond, { Value = 0 })
)
end
local holdBeganConnection
local holdEndedConnection
local triggeredConnection
local triggerEndedConnection
if prompt.HoldDuration > 0 then
holdBeganConnection = prompt.PromptButtonHoldBegan:Connect(function()
for _, tween in ipairs(tweensForButtonHoldBegin) do
tween:Play()
end
end)
holdEndedConnection = prompt.PromptButtonHoldEnded:Connect(function()
for _, tween in ipairs(tweensForButtonHoldEnd) do
tween:Play()
end
end)
end
triggeredConnection = prompt.Triggered:Connect(function()
for _, tween in ipairs(tweensForFadeOut) do
tween:Play()
end
end)
triggerEndedConnection = prompt.TriggerEnded:Connect(function()
for _, tween in ipairs(tweensForFadeIn) do
tween:Play()
end
end)
local function updateUIFromPrompt()
-- todo: Use AutomaticSize instead of GetTextSize when that feature becomes available
local actionTextSize =
TextService:GetTextSize(prompt.ActionText, 19, Enum.Font.GothamMedium, Vector2.new(1000, 1000))
local objectTextSize =
TextService:GetTextSize(prompt.ObjectText, 14, Enum.Font.GothamMedium, Vector2.new(1000, 1000))
local maxTextWidth = math.max(actionTextSize.X, objectTextSize.X)
local promptHeight = 72
local promptWidth = 72
local textPaddingLeft = 72
if
(prompt.ActionText ~= nil and prompt.ActionText ~= "")
or (prompt.ObjectText ~= nil and prompt.ObjectText ~= "")
then
promptWidth = maxTextWidth + textPaddingLeft + 24
end
local actionTextYOffset = 0
if prompt.ObjectText ~= nil and prompt.ObjectText ~= "" then
actionTextYOffset = 9
end
actionText.Position = UDim2.new(0.5, textPaddingLeft - promptWidth / 2, 0, actionTextYOffset)
objectText.Position = UDim2.new(0.5, textPaddingLeft - promptWidth / 2, 0, -10)
actionText.Text = prompt.ActionText
objectText.Text = prompt.ObjectText
actionText.AutoLocalize = prompt.AutoLocalize
actionText.RootLocalizationTable = prompt.RootLocalizationTable
objectText.AutoLocalize = prompt.AutoLocalize
objectText.RootLocalizationTable = prompt.RootLocalizationTable
promptUI.Size = UDim2.fromOffset(promptWidth, promptHeight)
promptUI.SizeOffset =
Vector2.new(prompt.UIOffset.X / promptUI.Size.Width.Offset, prompt.UIOffset.Y / promptUI.Size.Height.Offset)
end
local changedConnection = prompt.Changed:Connect(updateUIFromPrompt)
updateUIFromPrompt()
promptUI.Adornee = prompt.Parent
promptUI.Parent = gui
for _, tween in ipairs(tweensForFadeIn) do
tween:Play()
end
local function cleanup()
if holdBeganConnection then
holdBeganConnection:Disconnect()
end
if holdEndedConnection then
holdEndedConnection:Disconnect()
end
triggeredConnection:Disconnect()
triggerEndedConnection:Disconnect()
changedConnection:Disconnect()
for _, tween in ipairs(tweensForFadeOut) do
tween:Play()
end
task.wait(0.2)
promptUI.Parent = nil
end
return cleanup
end
local function onLoad()
ProximityPromptService.PromptShown:Connect(function(prompt, inputType)
if prompt.Style == Enum.ProximityPromptStyle.Default then
return
end
local gui = getScreenGui()
local cleanupFunction = createPrompt(prompt, inputType, gui)
prompt.PromptHidden:Wait()
cleanupFunction()
end)
end
onLoad()
The example below illustrates a prompt that requires the user to hold the button down in order to heal their character. This should be used in a Script that is a child of a ProximityPrompt.
local RunService = game:GetService("RunService")
local prompt = script.Parent
local playersHealing = {}
RunService.Stepped:Connect(function(_currentTime, deltaTime)
for player, _value in pairs(playersHealing) do
local humanoid = player.Character:FindFirstChildWhichIsA("Humanoid")
if humanoid then
humanoid.Health = humanoid.Health + 30 * deltaTime
end
end
end)
prompt.Triggered:Connect(function(player)
playersHealing[player] = true
end)
prompt.TriggerEnded:Connect(function(player)
playersHealing[player] = nil
end)
요약
속성
사용자에게 표시되는 작업 텍스트.
프롬프트의 ProximityPrompt.ActionText 및 ProximityPrompt.ObjectText 가 ProximityPrompt.RootLocalizationTable 에 따라 지역화되는지 여부
프롬프트를 클릭하거나 탭하여 프롬프트 UI를 활성화할 수 있는지 여부.
이 메시지가 표시되어야 하는지 여부.
동시에 표시할 수 있는 프롬프트를 사용자 지정하는 데 사용됩니다.
플레이어가 프롬프트를 트리거하기 위해 누르는 게임패드 버튼.
플레이어가 버튼/키를 길게 누르고 프롬프트를 트리거해야 하는 시간(초)입니다.
플레이어가 프롬프트를 트리거하기 위해 누르는 키.
플레이어의 character 가 나타나기 위해 최대 거리는 다음과 같습니다.The maximum distance a Player's can be from the ProximityPrompt for the prompt to appear.
사용자에게 표시되는 개체 이름 텍스트를 결정하는 선택적 속성입니다.
플레이어의 Camera 와 개체 부모가 ProximityPrompt 에 차단되는 경우 프롬프트가 숨겨지는지 여부.
이 프롬프트의 LocalizationTable 및 ProximityPrompt.ActionText에 자동 번역을 적용하기 위해 사용할 ProximityPrompt.ObjectText 참조.
프롬프트의 UI 스타일.
프롬프트의 UI에 적용된 픽셀 오프셋.
메서드
사용자가 빠른 GUI 버튼을 누르기 시작했음을 나타내는 신호를 발사합니다.
사용자가 빠른 대화형 GUI 버튼을 누르는 것을 종료했음을 나타내는 신호를 발사합니다.
이벤트
플레이어가 0보다 큰 key /버튼이 연결된 프롬프트에서 길게 누르기 시작할 때 트리거됩니다.Triggered when a player begins holding down the /button connected to a prompt with a non-zero ProximityPrompt.HoldDuration .
플레이어가 0보다 큰 ProximityPrompt.HoldDuration 버튼을 길게 누르는 프롬프트에서 트리거가 발생합니다.
프롬프트가 숨겨지면 트리거됩니다.
prompt가 표시되면 트리거됩니다.
사용자가 버튼을 길게 누르는 더 긴 이벤트에서 /button이 릴리스되면 트리거됩니다.
프롬프트 key/버튼이 누르거나 특정 시간 동안 버튼을 누른 후, ProximityPrompt.HoldDuration가 사용되면 트리거됩니다.
속성
AutoLocalize
이 속성은 프롬프트의 ProximityPrompt.ActionText 및 ProximityPrompt.ObjectText가 ProximityPrompt.RootLocalizationTable에 따라 지역화되는지 여부를 결정합니다.설정을 true로 설정하면 지역화가 적용됩니다.
ClickablePrompt
이 속성은 프롬프트의 UI를 클릭하거나 탭하여 프롬프트를 활성화할 수 있는지 여부를 결정합니다.거짓으로 설정되면 모바일에서만 클릭/탭으로 프롬프트를 활성화할 수 없습니다.
Enabled
이 속성은 이 속성이 표시되어야 하는지 여부를 나타냅니다.This property indicates whether or this ProximityPrompt should be shown.
MaxActivationDistance
이 속성은 플레이어의 character 가 나타나기 위해 최대 거리가 될 수 있는 ProximityPrompt 에 대한 프롬프트가 표시될 수 있는 거리를 결정합니다.
RequiresLineOfSight
이 속성은 플레이어의 Camera 와 개체 부모가 ProximityPrompt 에 차단되는 경우 프롬프트가 표시되는지 여부를 나타냅니다.참이면 카메라에서 개체로 명확한 경로가 있는 경우에만 이 메시지가 표시됩니다.
RootLocalizationTable
이 속성은 자동 번역을 적용하기 위해 사용된 LocalizationTable 및 ProximityPrompt.ActionText 및 ProximityPrompt.ObjectText에 대한 참조로 사용됩니다.이것이 적용되려면 ProximityPrompt.AutoLocalize 가 설정되어야 합니다.
개발자는 이를 DataModel 로캘리제이션 테이블을 참조하도록 설정할 수 있습니다.LocalizationService의 자식이 되어야 하지 않습니다.참조된 테이블에 번역이 없으면 해당 테이블의 부모에서 번역을 찾으며, 이 또한 지역화 테이블이면 계속됩니다.
이 속성은 프롬프트의 스타일을 나타냅니다. 기본 UI가 제공되지 않도록 설정되면 사용자 지정으로 설정됩니다.
제공된 UI를 사용자 지정 UI로 교체할 수 있습니다.이를 수행하려면 스타일을 사용자 지정으로 설정합니다.그런 다음, 개발자가 UI를 만들고 철거해야 하는 ProximityPrompt.PromptShown 및 ProximityPrompt.PromptHidden 이벤트를 들어 LocalScript 에서 수신하십시오.
개발자들은 또한 및 를 사용하여 진행 상태 애니메이션 기능을 활용할 수 있습니다.
메서드
InputHoldBegin
이 함수는 사용자가 ProximityPrompt 프롬프트 버튼을 누르기 시작했음을 나타내는 신호를 트리거합니다.프롬프트를 사용자 지정하고 프롬프트 GUI 버튼 누르다트리거하려는 개발자가 사용해야 합니다.
반환
이벤트
PromptButtonHoldBegan
이 이벤트는 플레이어가 0보다 큰 key/버튼을 누르기 시작할 때 트리거됩니다.This event triggers when a player begins holding down the /button on a prompt with a non-zero ProximityPrompt.HoldDuration .가능한 사용 중 하나는 보유 진행률 바를 애니메이션하는 것입니다.
매개 변수
PromptButtonHoldEnded
이 이벤트는 플레이어가 0보다 큰 ProximityPrompt.HoldDuration 버튼을 길게 누르는 프롬프트에서 종료될 때 트리거됩니다.가능한 사용 중 하나는 보유 진행률 바를 애니메이션하는 것입니다.
매개 변수
입력 대기를 종료한 플레이어.
PromptShown
이 이벤트는 보기 가능한 prompt가 되면 트리거됩니다. 이 이벤트는 클라이언트 측에서 LocalScripts에 대해 트리거됩니다.
매개 변수
프롬프트를 트리거하는 입력.
Triggered
이 이벤트는 프롬프트 key/버튼이 누르거나 특정 시간 동안 버튼을 누른 후 발생하며, ProximityPrompt.HoldDuration가 사용되는 경우에는 시간이 지나면 발생합니다.