Lighting
The Lighting service controls global lighting in an experience. It includes a range of adjustable properties that you can use to change how lighting appears and interacts with other objects, as summarized in Lighting Properties.
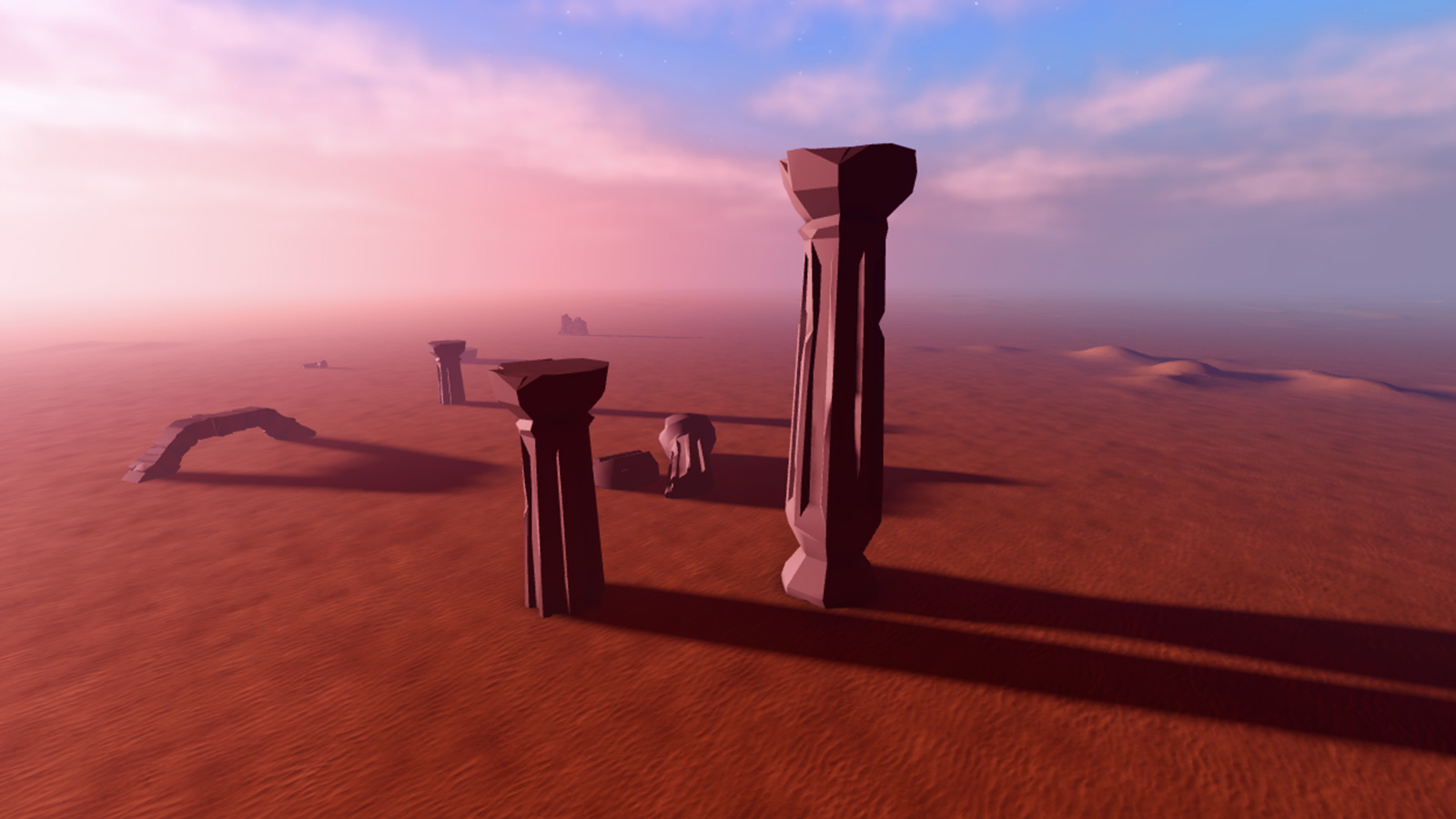
Lighting may also contain an Atmosphere object to render realistic atmospheric effects, including particle density, haze, glare, and color. See Atmospheric Effects for details.
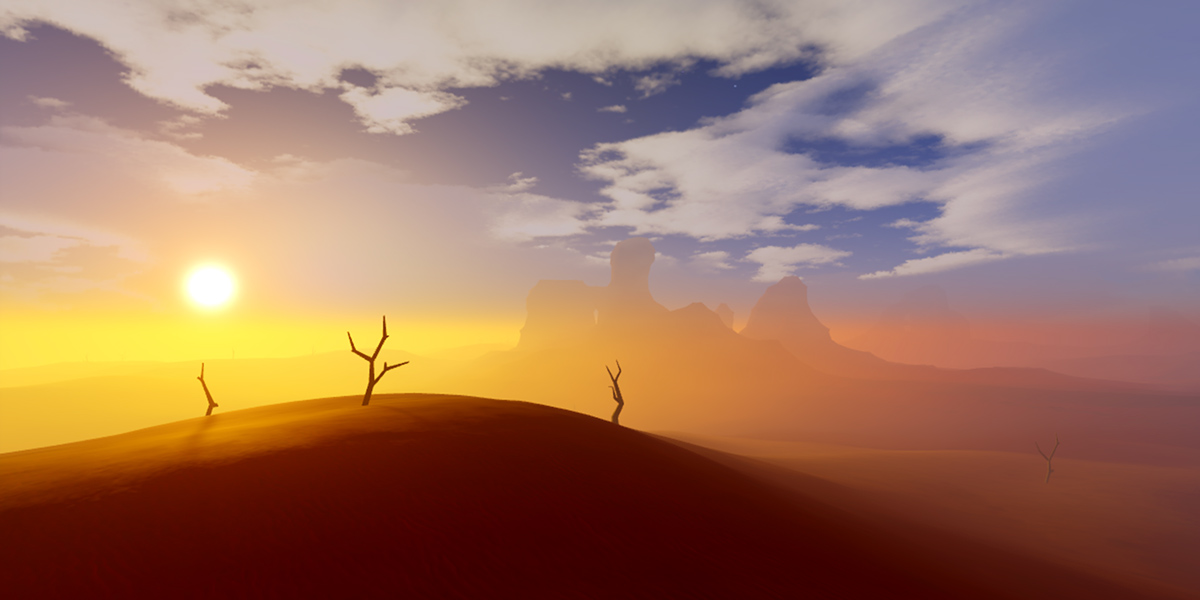
In addition, Lighting (along with Workspace.CurrentCamera) may contain post‑processing effects such as SunRaysEffect and BlurEffect.
Summary
Properties
The lighting hue applied to areas that are occluded from the sky, such as indoor areas.
The intensity of illumination in the place.
A numerical representation (in hours) of the current time of day used by Lighting.
The hue represented in light reflected in the opposite surfaces to those facing the sun or moon.
The hue represented in light reflected from surfaces facing the sun or moon.
Ambient light that is derived from the environment.
Specular light derived from environment.
The exposure compensation value.
A Color3 value giving the hue of Lighting fog.
The depth from the Workspace.CurrentCamera, in studs, at which fog will be completely opaque.
The depth from the Workspace.CurrentCamera, in studs, at which fog begins to show.
The geographic latitude, in degrees, of the scene, influencing the result of Lighting time on the position of the sun and moon.
Toggles voxel-based dynamic lighting for the place.
The lighting hue applied to outdoor areas.
Controls how blurry the shadows are.
Determines the lighting system for rendering the 3D world. Non-scriptable. .
A 24-hour string representation of the current time of day used by Lighting.
Methods
Returns the number of minutes that have passed after midnight for the purposes of lighting.
Returns a Vector3 representing the direction of the moon.
Returns the moon's current phase.
Returns a Vector3 representing the direction of the sun.
Sets TimeOfDay and ClockTime to the given number of minutes after midnight.
Events
This event fires when a Lighting property is changed or a Sky is added or removed from Lighting.
Properties
Ambient
Ambient is the lighting hue applied to areas that are occluded from the sky, such as indoor areas.
Ambient defaults to [0, 0, 0] (black). As long as the red, green, and blue channels of this property do not exceed the corresponding channels in OutdoorAmbient, the change in hue will be reserved for areas occluded from the sun/moon.
Note that when GlobalShadows is disabled, there is no distinction between areas occluded from the sky and non‑occluded areas. In this case, OutdoorAmbient will be ignored and the hue from the Ambient property will be applied everywhere.
Brightness
The intensity of illumination in the place.
Changing this value will influence the impact of the light source (sun or
moon) on the place's lighting. Note that Ambient
and OutdoorAmbient can also be used to
influence how bright a place appears. For example, setting
OutdoorAmbient to
ClockTime
A numerical representation (in hours) of the current time of day used by Lighting. Note that this property does not correspond with the actual time of day and will not change during gameplay unless it has been changed by a script.
For a measure of Lighting time formatted as a 24-hour string, use TimeOfDay. Changing TimeOfDay or using SetMinutesAfterMidnight() will also change this property.
ColorShift_Bottom
The hue represented in light reflected in the opposite surfaces to those facing the sun or moon.
The surfaces of a BasePart influenced by ColorShift_Bottom depends on the position and orientation of the BasePart relative to the sun or moon's position. Where the sun is directly overhead a BasePart, the shift in color will only apply to the bottom surface.
This effect can be increased or reduced by altering Brightness.
Note that ColorShift_Top and
ColorShift_Bottom will interact with the
Ambient and
OutdoorAmbient properties if they are
greater than
ColorShift_Top
The hue represented in light reflected from surfaces facing the sun or moon.
The surfaces of a BasePart influenced by ColorShift_Top depends on the position and orientation of the BasePart relative to the sun or moon's position. Where the sun is directly overhead a BasePart, the shift in color will only apply to the top surface.
This effect can be increased or reduced by altering Brightness.
Note that ColorShift_Top and
ColorShift_Bottom will interact with
the Ambient and
OutdoorAmbient properties if they are
greater than
EnvironmentDiffuseScale
Ambient light that is derived from the environment with a default of 0. This property is similar to Ambient and OutdoorAmbient but it's dynamic and can change according to the sky and time of day. When this property is increased, it's recommended to decrease Ambient and OutdoorAmbient accordingly.
EnvironmentSpecularScale
Specular light derived from environment with a default of 0. This property will make smooth objects reflect the environment and it is especially important to make metal look more realistic.
ExposureCompensation
This property determines the exposure compensation amount which applies a bias to the exposure level of the scene prior to the tonemap step. Defaults to 0 (no exposure compensation) and has a range from -5 to 5. A value of 1 indicates twice as much exposure and -1 means half as much exposure.
FogColor
A Color3 value giving the hue of Lighting fog. Note that fog properties are hidden when Lighting contains an Atmosphere object.
FogEnd
The depth from the Workspace.CurrentCamera, in studs, at which fog will be completely opaque. Note that fog properties are hidden when Lighting contains an Atmosphere object.
FogStart
The depth from the Workspace.CurrentCamera, in studs, at which fog begins to show. Note that fog properties are hidden when Lighting contains an Atmosphere object.
GeographicLatitude
The geographic latitude, in degrees, of the scene, influencing the result of Lighting time on the position of the sun and moon. When calculating the position of the sun, the earth's tilt is also taken into account.
Changing GeographicLatitude will alter the position of the sun at every TimeOfDay. If you're looking to obtain the sun or moon's position, use GetSunDirection() or GetMoonDirection().
GlobalShadows
Toggles voxel-based dynamic lighting in the place. When set to true, shadows are rendered in sheltered areas depending on the position of the sun and moon. The lighting hue applied to these sheltered areas is determined by the Ambient property while the lighting hue in all other areas is determined by the OutdoorAmbient property.
When false, shadows are not drawn and no distinction is made between indoor and outdoor areas. As a result, the Ambient property determines the lighting hue and OutdoorAmbient will do nothing.
Shadows are calculated using a voxel system and each lighting voxel is 4×4×4 studs. This means objects need to be larger than 4×4×4 studs to display a realistic shadow. Shadows are also recalculated when BaseParts are moving.
LightingStyle
OutdoorAmbient
OutdoorAmbient is the lighting hue applied to outdoor areas.
OutdoorAmbient defaults to [127, 127, 127]. As long as the red, green, and blue channels of Ambient do not exceed the corresponding channels in OutdoorAmbient, the hue of the lighting in outdoor areas will be determined by this property.
The effective OutdoorAmbient value is clamped to be greater than or equal to Ambient in all channels, meaning that if a channel of Ambient exceeds its corresponding OutdoorAmbient channel, the hue of Ambient will begin to apply to outdoor areas.
Note that when GlobalShadows is disabled, there is no distinction between areas occluded from the sky and non‑occluded areas. In this case, OutdoorAmbient will be ignored and the hue from the Ambient property will be applied everywhere.
PrioritizeLightingQuality
ShadowSoftness
Controls how blurry the shadows are with a default of 0.2. This property only works when Technology mode is ShadowMap or Future and the device is capable of rendering shadow maps.
Technology
Determines the lighting system for rendering the 3D world. This property is non‑scriptable and only modifiable in Studio. See Enum.Technology for available options and Lighting Technology for detailed descriptions and visual effects of each option.
TimeOfDay
A 24-hour string representation of the current time of day used by Lighting. Note that this property does not correspond with the actual time of day and will not change during gameplay unless it has been changed by a script.
For a numeric measure of Lighting time, use ClockTime. Changing ClockTime or using SetMinutesAfterMidnight() will also change this property.
Methods
GetMinutesAfterMidnight
Returns the number of minutes that have passed after midnight for the purposes of lighting. This number will be nearly identical to ClockTime multiplied by 60.
Note that this number will not always be equal to the value given in SetMinutesAfterMidnight() as it returns minutes after midnight in the current day.
Returns
The number of minutes after midnight.
GetMoonDirection
Returns a Vector3 representing the direction of the moon from
the position
GetSunDirection() is a variant of this method for obtaining the direction of the sun.
Returns
GetMoonPhase
Returns the moon's current phase. There is no way to change the moon's phase so this will always return 0.75.
Returns
GetSunDirection
Returns a Vector3 representing the direction of the sun from
the position
GetMoonDirection() is a variant of this method for obtaining the direction of the moon.
Returns
SetMinutesAfterMidnight
Sets TimeOfDay and ClockTime to the given number of minutes after midnight.
This method allows a numerical value to be used, for example in a day/night cycle Script, without the need to convert to a string in the format required by TimeOfDay. It also allows values greater than 24 hours to be given that correspond to times in the next day.
The following code sample includes a simple day/night cycle script. The speed of time and the initial time can be changed using the TIME_SPEED and START_TIME parameters.
local Lighting = game:GetService("Lighting")local TIME_SPEED = 60 -- 1 min = 1 hourlocal START_TIME = 9 -- 9 AMlocal minutesAfterMidnight = START_TIME * 60local waitTime = 60 / TIME_SPEEDwhile true dominutesAfterMidnight = minutesAfterMidnight + 1Lighting:SetMinutesAfterMidnight(minutesAfterMidnight)task.wait(waitTime)end
Parameters
The number of minutes after midnight.
Returns
Events
LightingChanged
This event fires when a Lighting property is changed or a Sky is added or removed from Lighting, with some exceptions:
- Changing GlobalShadows will not fire this event.
In cases where this behavior is not desired, the Object.Changed event or Object:GetPropertyChangedSignal() method can be used.