BasePart
BasePart is an abstract base class for in-world objects that render and are physically simulated while in the Workspace. There are several implementations of BasePart, the most common being Part and MeshPart. Others include WedgePart, SpawnLocation, and the singleton Terrain object. Generally, when documentation refers to a "part," most BasePart implementations will work and not just Part.
For information on how BaseParts are grouped into simulated rigid bodies, see Assemblies.
There are many different objects that interact with BasePart (other than Terrain), including:
- Several BaseParts may be grouped within a Model and moved at the same time using PVInstance:PivotTo(). See Models.
- A Decal applies a stretched image texture to the faces of a BasePart, while a Texture applies a tiled image texture to the faces. See Textures and Decals.
- Attachments can be added to a BasePart to specify CFrames relative to the part. These are often used by physical Constraint objects as outlined in Mechanical Constraints and Mover Constraints.
- ParticleEmitter objects emit particles uniformly in the volume of the BasePart to which they are parented. See Particle Emitters.
- Light objects like PointLight emit light from the center of a BasePart as illustrated in Light Sources.
- If parented to a Tool and given the name Handle, a BasePart can be held by characters. See In-Experience Tools.
Summary
Properties
Determines whether a part is immovable by physics.
The angular velocity of the part's assembly.
The center of mass of the part's assembly in world space.
The linear velocity of the part's assembly.
The total mass of the part's assembly.
A reference to the root part of the assembly.
Determines the type of surface for the Back face of a part (+Z direction).
Determines the type of surface for the Bottom face of a part (-Y direction).
Determines the color of a part.
Determines the position and orientation of the BasePart in the world.
Determines whether a part may collide with other parts.
Determines whether the part is considered during spatial query operations.
Determines if Touched and TouchEnded events fire on the part.
Determines whether or not a part casts a shadow.
Describes the world position in which a part's center of mass is located.
Describes the name of a part's collision group.
Determines the color of a part.
Indicates the current physical properties of the part.
Determines several physical properties of a part.
Used to enable or disable aerodynamic forces on parts and assemblies.
The actual physical size of the BasePart as regarded by the physics engine.
Determines the type of surface for the Front face of a part (-Z direction).
Determines the type of surface for the Left face of a part (-X direction).
Determines a multiplier for BasePart.Transparency that is only visible to the local client.
Determines whether a part is selectable in Studio.
Describes the mass of the part, the product of its density and volume.
Determines whether the part contributes to the total mass or inertia of its rigid body.
Determines the texture and default physical properties of a part.
The name of MaterialVariant.
Describes the rotation of the part in the world.
Specifies the offset of the part's pivot from its CFrame.
Describes the position of the part in the world.
Time since last recorded physics update.
Determines how much a part reflects the skybox.
Describes the smallest change in size allowable by the Resize method.
Describes the faces on which a part may be resized.
Determines the type of surface for the Right face of a part (+X direction).
The main rule in determining the root part of an assembly.
The rotation of the part in degrees for the three axes.
Determines the dimensions of a part (length, width, height).
Determines the type of surface for the Top face of a part (+Y direction).
Determines how much a part can be seen through (the inverse of part opacity).
Methods
Apply an angular impulse to the assembly.
Apply an impulse to the assembly at the assembly's center of mass.
Apply an impulse to the assembly at specified position.
Returns whether the parts can collide with each other.
Checks whether you can set a part's network ownership.
Returns a table of parts connected to the object by any kind of rigid joint.
Return all Joints or Constraints that is connected to this Part.
Returns the value of the Mass property.
Returns the current player who is the network owner of this part, or nil in case of the server.
Returns true if the game engine automatically decides the network owner for this part.
Returns the base part of an assembly of parts.
Returns a table of all BasePart.CanCollide true parts that intersect with this part.
Returns the linear velocity of the part's assembly at the given position relative to this part.
Returns true if the object is connected to a part that will hold it in place (eg an Anchored part), otherwise returns false.
Changes the size of an object just like using the Studio resize tool.
Sets the given player as network owner for this and all connected parts.
Lets the game engine dynamically decide who will handle the part's physics (one of the clients or the server).
- IntersectAsync(parts : Instances,collisionfidelity : Enum.CollisionFidelity,renderFidelity : Enum.RenderFidelity):Instance
Creates a new IntersectOperation from the overlapping geometry of the part and the other parts in the given array.
- SubtractAsync(parts : Instances,collisionfidelity : Enum.CollisionFidelity,renderFidelity : Enum.RenderFidelity):Instance
Creates a new UnionOperation from the part, minus the geometry occupied by the parts in the given array.
- UnionAsync(parts : Instances,collisionfidelity : Enum.CollisionFidelity,renderFidelity : Enum.RenderFidelity):Instance
Creates a new UnionOperation from the part, plus the geometry occupied by the parts in the given array.
Gets the pivot of a PVInstance.
Transforms the PVInstance along with all of its descendant PVInstances such that the pivot is now located at the specified CFrame.
Events
Fires when a part stops touching another part as a result of physical movement.
Fires when a part touches another part as a result of physical movement.
Properties
Anchored
The Anchored property determines whether the part will be immovable by physics. When enabled, a part will never change position due to gravity, other part collisions, overlapping other parts, or any other physics-related causes. As a result, two anchored parts will never fire the BasePart.Touched event on each other.
An anchored part may still be moved by changing its CFrame or Position, and it still may have a nonzero AssemblyLinearVelocity and AssemblyAngularVelocity.
Finally, if an unanchored part is joined with an anchored part through an object like a Weld, it too will act anchored. If such a joint breaks, the part may be affected by physics again. See Assemblies for more details.
Network ownership cannot be set on anchored parts. If a part's anchored status changes on the server, the network ownership of that part will be affected.
Code Samples
local part = script.Parent
-- Create a ClickDetector so we can tell when the part is clicked
local cd = Instance.new("ClickDetector", part)
-- This function updates how the part looks based on its Anchored state
local function updateVisuals()
if part.Anchored then
-- When the part is anchored...
part.BrickColor = BrickColor.new("Bright red")
part.Material = Enum.Material.DiamondPlate
else
-- When the part is unanchored...
part.BrickColor = BrickColor.new("Bright yellow")
part.Material = Enum.Material.Wood
end
end
local function onToggle()
-- Toggle the anchored property
part.Anchored = not part.Anchored
-- Update visual state of the brick
updateVisuals()
end
-- Update, then start listening for clicks
updateVisuals()
cd.MouseClick:Connect(onToggle)
AssemblyAngularVelocity
The angular velocity vector of this part's assembly. It's the rate of change of orientation in radians per second.
Angular velocity is the same at every point of the assembly.
Setting the velocity directly may lead to unrealistic motion. Using Torque or AngularVelocity constraint is preferred, or use BasePart:ApplyAngularImpulse() if you want instantaneous change in velocity.
If the part is owned by the server, this property must be changed from a server Script (not from a LocalScript or a Script with RunContext set to Enum.RunContext.Client). If the part is owned by a client through automatic ownership, this property can be changed from either a client script or a server script; changing it from a client script for a server-owned part will have no effect.
AssemblyCenterOfMass
A position calculated via the mass and position of all the parts in the assembly.
If the assembly has an anchored part, that part's center of mass will be the assembly's center of mass, and the assembly will have infinite mass.
Knowing the center of mass can help the assembly maintain stability. A force applied to the center of mass will not cause angular acceleration, only linear. An assembly with a low center of mass will have a better time staying upright under the effect of gravity.
AssemblyLinearVelocity
The linear velocity vector of this part's assembly. It's the rate of change in position of the assembly's center of mass in studs per second.
If you want to know the velocity at a point other than the assembly's center of mass, use BasePart:GetVelocityAtPosition().
Setting the velocity directly may lead to unrealistic motion. Using a VectorForce constraint is preferred, or use BasePart:ApplyImpulse() if you want instantaneous change in velocity.
If the part is owned by the server, this property must be changed from a server Script (not from a LocalScript or a Script with RunContext set to Enum.RunContext.Client). If the part is owned by a client through automatic ownership, this property can be changed from either a client script or a server script; changing it from a client script for a server-owned part will have no effect.
AssemblyMass
The sum of the mass of all the parts in this part's assembly. Parts that are Massless and are not the assembly's root part will not contribute to the AssemblyMass.
If the assembly has an anchored part, the assembly's mass is considered infinite. Constraints and other physical interactions between unanchored assemblies with a large difference in mass may cause instabilities.
AssemblyRootPart
This property indicates the BasePart automatically chosen to represent the assembly's root part. It is the same part that's returned when developers call GetRootPart().
The root part can be changed by changing the RootPriority of the parts in the assembly.
Parts that all share the same AssemblyRootPart are in the same assembly.
For more information on root parts, see Assemblies.
AudioCanCollide
BackSurface
The BackSurface property determines the type of surface used for the +Z direction of a part. When two parts' faces are placed next to each other, they may create a joint between them. If set to Motor, the BasePart.BackSurfaceInput determines how a motor joint should behave.
Most SurfaceTypes render a texture on the part face if the BasePart.Material is set to Plastic. Some SurfaceTypes - Hinge, Motor and SteppingMotor - will render a 3D adornment instead. If this property is selected in the Properties window, it will be highlighted in the game world similar to that of a SurfaceSelection.
Code Samples
local demoPart = script.Parent
-- Create a billboard gui to display what the current surface type is
local billboard = Instance.new("BillboardGui")
billboard.AlwaysOnTop = true
billboard.Size = UDim2.new(0, 200, 0, 50)
billboard.Adornee = demoPart
local textLabel = Instance.new("TextLabel")
textLabel.Size = UDim2.new(0, 200, 0, 50)
textLabel.BackgroundTransparency = 1
textLabel.TextStrokeTransparency = 0
textLabel.TextColor3 = Color3.new(1, 1, 1) -- White
textLabel.Parent = billboard
billboard.Parent = demoPart
local function setAllSurfaces(part, surfaceType)
part.TopSurface = surfaceType
part.BottomSurface = surfaceType
part.LeftSurface = surfaceType
part.RightSurface = surfaceType
part.FrontSurface = surfaceType
part.BackSurface = surfaceType
end
while true do
-- Iterate through the different SurfaceTypes
for _, enum in pairs(Enum.SurfaceType:GetEnumItems()) do
textLabel.Text = enum.Name
setAllSurfaces(demoPart, enum)
task.wait(1)
end
end
BottomSurface
The BottomSurface property determines the type of surface used for the -Y direction of a part. When two parts' faces are placed next to each other, they may create a joint between them. If set to Motor, the BasePart.BottomSurfaceInput determines how a motor joint should behave.
Most SurfaceTypes render a texture on the part face if the BasePart.Material is set to Plastic. Some SurfaceTypes - Hinge, Motor and SteppingMotor - will render a 3D adornment instead. If this property is selected in the Properties window, it will be highlighted in the game world similar to that of a SurfaceSelection.
Code Samples
local demoPart = script.Parent
-- Create a billboard gui to display what the current surface type is
local billboard = Instance.new("BillboardGui")
billboard.AlwaysOnTop = true
billboard.Size = UDim2.new(0, 200, 0, 50)
billboard.Adornee = demoPart
local textLabel = Instance.new("TextLabel")
textLabel.Size = UDim2.new(0, 200, 0, 50)
textLabel.BackgroundTransparency = 1
textLabel.TextStrokeTransparency = 0
textLabel.TextColor3 = Color3.new(1, 1, 1) -- White
textLabel.Parent = billboard
billboard.Parent = demoPart
local function setAllSurfaces(part, surfaceType)
part.TopSurface = surfaceType
part.BottomSurface = surfaceType
part.LeftSurface = surfaceType
part.RightSurface = surfaceType
part.FrontSurface = surfaceType
part.BackSurface = surfaceType
end
while true do
-- Iterate through the different SurfaceTypes
for _, enum in pairs(Enum.SurfaceType:GetEnumItems()) do
textLabel.Text = enum.Name
setAllSurfaces(demoPart, enum)
task.wait(1)
end
end
BrickColor
The BrickColor property determines the color of a part. If the part has a BasePart.Material, this also determines the color used when rendering the material texture. For more control over the color, the BasePart.Color property can be used (it is a Color3 variant of this property). If Color set, this property will use the closest BrickColor.
Other visual properties of a part are determined by BasePart.Transparency and BasePart.Reflectance.
Code Samples
local part = script.Parent
-- Create a ClickDetector so we can tell when the part is clicked
local cd = Instance.new("ClickDetector", part)
-- This function updates how the part looks based on its Anchored state
local function updateVisuals()
if part.Anchored then
-- When the part is anchored...
part.BrickColor = BrickColor.new("Bright red")
part.Material = Enum.Material.DiamondPlate
else
-- When the part is unanchored...
part.BrickColor = BrickColor.new("Bright yellow")
part.Material = Enum.Material.Wood
end
end
local function onToggle()
-- Toggle the anchored property
part.Anchored = not part.Anchored
-- Update visual state of the brick
updateVisuals()
end
-- Update, then start listening for clicks
updateVisuals()
cd.MouseClick:Connect(onToggle)
CFrame
The CFrame property determines both the position and orientation of the BasePart in the world. It acts as an arbitrary reference location on the geometry, but ExtentsCFrame represents the actual CFrame of its physical center.
When setting CFrame on a part, other joined parts are also moved relative to the part, but it is recommended that you use PVInstance:PivotTo() to move an entire model, such as when teleporting a player's character.
Unlike setting BasePart.Position, setting BasePart.CFrame will always move the part to the exact given CFrame; in other words: no overlap checking is done and the physics solver will attempt to resolve any overlap unless both parts are Anchored.
For keeping track of positions relative to a part's CFrame, an Attachment may be useful.
Code Samples
local part = script.Parent:WaitForChild("Part")
local otherPart = script.Parent:WaitForChild("OtherPart")
-- Reset the part's CFrame to (0, 0, 0) with no rotation.
-- This is sometimes called the "identity" CFrame
part.CFrame = CFrame.new()
-- Set to a specific position (X, Y, Z)
part.CFrame = CFrame.new(0, 25, 10)
-- Same as above, but use a Vector3 instead
local point = Vector3.new(0, 25, 10)
part.CFrame = CFrame.new(point)
-- Set the part's CFrame to be at one point, looking at another
local lookAtPoint = Vector3.new(0, 20, 15)
part.CFrame = CFrame.lookAt(point, lookAtPoint)
-- Rotate the part's CFrame by pi/2 radians on local X axis
part.CFrame = part.CFrame * CFrame.Angles(math.pi / 2, 0, 0)
-- Rotate the part's CFrame by 45 degrees on local Y axis
part.CFrame = part.CFrame * CFrame.Angles(0, math.rad(45), 0)
-- Rotate the part's CFrame by 180 degrees on global Z axis (note the order!)
part.CFrame = CFrame.Angles(0, 0, math.pi) * part.CFrame -- Pi radians is equal to 180 degrees
-- Composing two CFrames is done using * (the multiplication operator)
part.CFrame = CFrame.new(2, 3, 4) * CFrame.new(4, 5, 6) --> equal to CFrame.new(6, 8, 10)
-- Unlike algebraic multiplication, CFrame composition is NOT communitative: a * b is not necessarily b * a!
-- Imagine * as an ORDERED series of actions. For example, the following lines produce different CFrames:
-- 1) Slide the part 5 units on X.
-- 2) Rotate the part 45 degrees around its Y axis.
part.CFrame = CFrame.new(5, 0, 0) * CFrame.Angles(0, math.rad(45), 0)
-- 1) Rotate the part 45 degrees around its Y axis.
-- 2) Slide the part 5 units on X.
part.CFrame = CFrame.Angles(0, math.rad(45), 0) * CFrame.new(5, 0, 0)
-- There is no "CFrame division", but instead simply "doing the inverse operation".
part.CFrame = CFrame.new(4, 5, 6) * CFrame.new(4, 5, 6):Inverse() --> is equal to CFrame.new(0, 0, 0)
part.CFrame = CFrame.Angles(0, 0, math.pi) * CFrame.Angles(0, 0, math.pi):Inverse() --> equal to CFrame.Angles(0, 0, 0)
-- Position a part relative to another (in this case, put our part on top of otherPart)
part.CFrame = otherPart.CFrame * CFrame.new(0, part.Size.Y / 2 + otherPart.Size.Y / 2, 0)
CanCollide
CanCollide determines whether a part will physically interact with other parts. When disabled, other parts can pass through the brick uninterrupted. Parts used for decoration usually have CanCollide disabled, as they need not be considered by the physics engine.
If a part is not BasePart.Anchored and has CanCollide disabled, it may fall out of the world to be eventually destroyed by Workspace.FallenPartsDestroyHeight.
When CanCollide is disabled, parts may still fire the BasePart.Touched event (as well the other parts touching them). You can disable this with BasePart.CanTouch.
For more information on collisions, see Collisions.
Code Samples
-- Paste into a Script inside a tall part
local part = script.Parent
local OPEN_TIME = 1
-- Can the door be opened at the moment?
local debounce = false
local function open()
part.CanCollide = false
part.Transparency = 0.7
part.BrickColor = BrickColor.new("Black")
end
local function close()
part.CanCollide = true
part.Transparency = 0
part.BrickColor = BrickColor.new("Bright blue")
end
local function onTouch(otherPart)
-- If the door was already open, do nothing
if debounce then
print("D")
return
end
-- Check if touched by a Humanoid
local human = otherPart.Parent:FindFirstChildOfClass("Humanoid")
if not human then
print("not human")
return
end
-- Perform the door opening sequence
debounce = true
open()
task.wait(OPEN_TIME)
close()
debounce = false
end
part.Touched:Connect(onTouch)
close()
CanQuery
CanQuery determines whether the part is considered during spatial query operations, such as GetPartBoundsInBox or Raycast. CanCollide must also be disabled when disabling CanQuery. These functions will never include parts whose CanQuery and CanCollide is false.
Beyond this property, it is also possible to exclude parts which are descendants of a given list of parts using an OverlapParams or RaycastParams object when calling the spatial query functions.
CanTouch
This property determines if Touched and TouchEnded events fire on the part. If true, other touching parts must also have CanTouch set to true for touch events to fire. If false, touch events cannot be set up for the part and attempting to do so will throw an error. Similarly, if the property is set to false after a touch event is connected, the event will be disconnected and the TouchTransmitter removed.
Note that this collision logic can be set to respect collision groups through the Workspace.TouchesUseCollisionGroups property. If true, parts in non-colliding groups will ignore both collisions and touch events, thereby making this property irrelevant.
Performance
There is a small performance gain on parts that have both CanTouch and CanCollide set to false, as these parts will never need to compute any kind of part to part collisions. However, they can still be hit by Raycasts and OverlapParams queries.
CastShadow
Determines whether or not a part casts a shadow.
Note that this feature is not designed for performance enhancement. It should only be disabled on parts where you want to hide the shadows the part casts. Disabling this property for a given part may cause visual artifacts on the shadows cast upon that part.
CenterOfMass
The CenterOfMass property describes the local position of a part's center of mass. If this is a single part assembly, this is the AssemblyCenterOfMass converted from world space to local. On simple Parts, the center of mass is always (0,0,0). It can vary for WedgePart or MeshPart however.
CollisionGroup
The CollisionGroup property describes the name of the part's collision group (maximum of 100 characters). Parts start off in the default group whose name is "Default". This value cannot be empty.
Although this property itself is non-replicated, the engine internally replicates the value through another private property to solve backward compatibility issues.
Code Samples
local PhysicsService = game:GetService("PhysicsService")
local collisionGroupBall = "CollisionGroupBall"
local collisionGroupDoor = "CollisionGroupDoor"
-- Register collision groups
PhysicsService:RegisterCollisionGroup(collisionGroupBall)
PhysicsService:RegisterCollisionGroup(collisionGroupDoor)
-- Assign parts to collision groups
script.Parent.BallPart.CollisionGroup = collisionGroupBall
script.Parent.DoorPart.CollisionGroup = collisionGroupDoor
-- Set groups as non-collidable with each other and check the result
PhysicsService:CollisionGroupSetCollidable(collisionGroupBall, collisionGroupDoor, false)
print(PhysicsService:CollisionGroupsAreCollidable(collisionGroupBall, collisionGroupDoor)) --> false
Color
The Color property determines the color of a part. If the part has a BasePart.Material, this also determines the color used when rendering the material texture. If this property is set, BasePart.BrickColor will use the closest BrickColor to the Color3 value.
Other visual properties of a part are determined by BasePart.Transparency and BasePart.Reflectance.
Code Samples
-- Paste into a Script within StarterCharacterScripts
-- Then play the game, and fiddle with your character's health
local char = script.Parent
local human = char.Humanoid
local colorHealthy = Color3.new(0.4, 1, 0.2)
local colorUnhealthy = Color3.new(1, 0.4, 0.2)
local function setColor(color)
for _, child in pairs(char:GetChildren()) do
if child:IsA("BasePart") then
child.Color = color
while child:FindFirstChildOfClass("Decal") do
child:FindFirstChildOfClass("Decal"):Destroy()
end
elseif child:IsA("Accessory") then
child.Handle.Color = color
local mesh = child.Handle:FindFirstChildOfClass("SpecialMesh")
if mesh then
mesh.TextureId = ""
end
elseif child:IsA("Shirt") or child:IsA("Pants") then
child:Destroy()
end
end
end
local function update()
local percentage = human.Health / human.MaxHealth
-- Create a color by tweening based on the percentage of your health
-- The color goes from colorHealthy (100%) ----- > colorUnhealthy (0%)
local color = Color3.new(
colorHealthy.R * percentage + colorUnhealthy.r * (1 - percentage),
colorHealthy.G * percentage + colorUnhealthy.g * (1 - percentage),
colorHealthy.B * percentage + colorUnhealthy.b * (1 - percentage)
)
setColor(color)
end
update()
human.HealthChanged:Connect(update)
CurrentPhysicalProperties
CurrentPhysicalProperties indicates the current physical properties of the part. You can set custom values for the physical properties per part, custom material, and material override. The Engine prioritizes more granular definitions when determining the effective physical properties of a part. The values in the following list are in order from highest to lowest priority:
- Custom physical properties of the part
- Custom physical properties of the part's custom material
- Custom physical properties of the material override of the part's material
- Default physical properties of the part's material
CustomPhysicalProperties
CustomPhysicalProperties lets you customize various physical aspects of a Part, such as its density, friction, and elasticity.
If enabled, this property let's you configure these physical properties. If disabled, these physical properties are determined by the BasePart.Material of the part. The page for Enum.Material contains list of the various part materials.
Code Samples
local part = script.Parent
-- This will make the part light and bouncy!
local DENSITY = 0.3
local FRICTION = 0.1
local ELASTICITY = 1
local FRICTION_WEIGHT = 1
local ELASTICITY_WEIGHT = 1
local physProperties = PhysicalProperties.new(DENSITY, FRICTION, ELASTICITY, FRICTION_WEIGHT, ELASTICITY_WEIGHT)
part.CustomPhysicalProperties = physProperties
EnableFluidForces
When true, and when Workspace.FluidForces is enabled, causes the physics engine to compute aerodynamic forces on this BasePart.
ExtentsSize
The actual physical size of the BasePart as regarded by the physics engine, for example in collision detection.
FrontSurface
The FrontSurface property determines the type of surface used for the -Z direction of a part. When two parts' faces are placed next to each other, they may create a joint between them. If set to Motor, the BasePart.FrontSurfaceInput determines how a motor joint should behave.
Most SurfaceTypes render a texture on the part face if the BasePart.Material is set to Plastic. Some SurfaceTypes including Hinge, Motor, and SteppingMotor render a 3D adornment instead. If this property is selected in the Properties window, it will be highlighted in the game world similar to that of a SurfaceSelection.
Code Samples
local demoPart = script.Parent
-- Create a billboard gui to display what the current surface type is
local billboard = Instance.new("BillboardGui")
billboard.AlwaysOnTop = true
billboard.Size = UDim2.new(0, 200, 0, 50)
billboard.Adornee = demoPart
local textLabel = Instance.new("TextLabel")
textLabel.Size = UDim2.new(0, 200, 0, 50)
textLabel.BackgroundTransparency = 1
textLabel.TextStrokeTransparency = 0
textLabel.TextColor3 = Color3.new(1, 1, 1) -- White
textLabel.Parent = billboard
billboard.Parent = demoPart
local function setAllSurfaces(part, surfaceType)
part.TopSurface = surfaceType
part.BottomSurface = surfaceType
part.LeftSurface = surfaceType
part.RightSurface = surfaceType
part.FrontSurface = surfaceType
part.BackSurface = surfaceType
end
while true do
-- Iterate through the different SurfaceTypes
for _, enum in pairs(Enum.SurfaceType:GetEnumItems()) do
textLabel.Text = enum.Name
setAllSurfaces(demoPart, enum)
task.wait(1)
end
end
LeftSurface
The LeftSurface property determines the type of surface used for the -X direction of a part. When two parts' faces are placed next to each other, they may create a joint between them. If set to Motor, the BasePart.LeftSurfaceInput determines how a motor joint should behave.
Most SurfaceTypes render a texture on the part face if the BasePart.Material is set to Plastic. Some SurfaceTypes including Hinge, Motor, and SteppingMotor render a 3D adornment instead. If this property is selected in the Properties window, it will be highlighted in the game world similar to that of a SurfaceSelection.
Code Samples
local demoPart = script.Parent
-- Create a billboard gui to display what the current surface type is
local billboard = Instance.new("BillboardGui")
billboard.AlwaysOnTop = true
billboard.Size = UDim2.new(0, 200, 0, 50)
billboard.Adornee = demoPart
local textLabel = Instance.new("TextLabel")
textLabel.Size = UDim2.new(0, 200, 0, 50)
textLabel.BackgroundTransparency = 1
textLabel.TextStrokeTransparency = 0
textLabel.TextColor3 = Color3.new(1, 1, 1) -- White
textLabel.Parent = billboard
billboard.Parent = demoPart
local function setAllSurfaces(part, surfaceType)
part.TopSurface = surfaceType
part.BottomSurface = surfaceType
part.LeftSurface = surfaceType
part.RightSurface = surfaceType
part.FrontSurface = surfaceType
part.BackSurface = surfaceType
end
while true do
-- Iterate through the different SurfaceTypes
for _, enum in pairs(Enum.SurfaceType:GetEnumItems()) do
textLabel.Text = enum.Name
setAllSurfaces(demoPart, enum)
task.wait(1)
end
end
LocalTransparencyModifier
The LocalTransparencyModifier property is a multiplier to BasePart.Transparency that is only visible to the local client. It does not replicate from client to server and is useful for when a part should not render for a specific client, such as how the player does not see their character's body parts when they zoom into first person mode.
This property modifies the local part's transparency through the following formula, with resulting values clamped between 0 and 1.
clientTransparency = 1 - ((1 - part.Transparency) * (1 - part.LocalTransparencyModifier))
Transparency | LocalTransparencyModifier | Server-Side Transparency | Client-Side Transparency |
---|---|---|---|
0.5 | 0 | 0.5 | 0.5 |
0.5 | 0.25 | 0.5 | 0.625 |
0.5 | 0.5 | 0.5 | 0.75 |
0.5 | 0.75 | 0.5 | 0.875 |
0.5 | 1 | 0.5 | 1 |
Locked
The Locked property determines whether a part (or a model it is contained within) may be selected in Roblox Studio by clicking on it. This property is most often enabled on parts within environment models that aren't being edited at the moment. Roblox Studio has a Lock/Unlock All tool that can toggle the Locked state of every part descendant in a model at once.
Code Samples
-- Paste into a Script within a Model you want to unlock
local model = script.Parent
-- This function recurses through a model's heirarchy and unlocks
-- every part that it encounters.
local function recursiveUnlock(object)
if object:IsA("BasePart") then
object.Locked = false
end
-- Call the same function on the children of the object
-- The recursive process stops if an object has no children
for _, child in pairs(object:GetChildren()) do
recursiveUnlock(child)
end
end
recursiveUnlock(model)
Mass
Mass is a read-only property that describes the product of a part's volume and density. It is returned by the GetMass function.
- The density of a part is determined by its Material or CustomPhysicalProperties, if specified.
Massless
If this property is enabled, the BasePart will not contribute to the total mass or inertia of its assembly as long as it is welded to another part that has mass.
If the part is its own root part according to AssemblyRootPart, this will be ignored for that part, and it will still contribute mass and inertia to its assembly like a normal part. Parts that are massless should never become an assembly root part unless all other parts in the assembly are also massless.
This might be useful for things like optional accessories on vehicles that you don't want to affect the handling of the car or a massless render mesh welded to a simpler collision mesh.
See also Assemblies, an article documenting what root parts are and how to use them.
Material
The Material property allows a builder to set a part's texture and default physical properties (in the case that BasePart.CustomPhysicalProperties is unset). The default Plastic material has a very light texture, and the SmoothPlastic material has no texture at all. Some material textures like DiamondPlate and Granite have very visible textures. Each material's texture reflects sunlight differently, especially Foil.
Setting this property then enabling BasePart.CustomPhysicalProperties will use the default physical properties of a material. For instance, DiamondPlate is a very dense material while Wood is very light. A part's density determines whether it will float in terrain water.
The Glass material changes rendering behavior on moderate graphics settings. It applies a bit of reflectiveness (similar to BasePart.Reflectance) and perspective distortion. The effect is especially pronounced on sphere-shaped parts (set BasePart.Shape to Ball). Semitransparent objects and Glass parts behind Glass are not visible.
Code Samples
local part = script.Parent
-- Create a ClickDetector so we can tell when the part is clicked
local cd = Instance.new("ClickDetector", part)
-- This function updates how the part looks based on its Anchored state
local function updateVisuals()
if part.Anchored then
-- When the part is anchored...
part.BrickColor = BrickColor.new("Bright red")
part.Material = Enum.Material.DiamondPlate
else
-- When the part is unanchored...
part.BrickColor = BrickColor.new("Bright yellow")
part.Material = Enum.Material.Wood
end
end
local function onToggle()
-- Toggle the anchored property
part.Anchored = not part.Anchored
-- Update visual state of the brick
updateVisuals()
end
-- Update, then start listening for clicks
updateVisuals()
cd.MouseClick:Connect(onToggle)
MaterialVariant
The system searches the MaterialVariant instance with specified MaterialVariant name and BasePart.Material type. If it successfully finds a matching MaterialVariant instance, it uses this MaterialVariant instance to replace the default material. Default material can be the built-in material or an override MaterialVariant specified in MaterialService.
Orientation
The Orientation property describes the part's rotation in degrees around the X, Y and Z axes using a Vector3. The rotations are applied in Y → X → Z order. This differs from proper Euler angles and is instead Tait-Bryan angles, which describe yaw, pitch and roll. It is also worth noting how this property differs from the CFrame.Angles() constructor, which applies rotations in a different order (Z → Y → X). For better control over the rotation of a part, it is recommended that BasePart.CFrame is set instead.
When setting this property any Welds or Motor6Ds connected to this part will have the matching C0 or C1 property updated and to allow the part to move relative to any other parts it is joined to.
WeldConstraints will also be temporarily disabled and re-enabled during the move.
Code Samples
local part = script.Parent
local INCREMENT = 360 / 20
-- Rotate the part continually
while true do
for degrees = 0, 360, INCREMENT do
-- Set only the Y axis rotation
part.Rotation = Vector3.new(0, degrees, 0)
-- A better way to do this would be setting CFrame
--part.CFrame = CFrame.new(part.Position) * CFrame.Angles(0, math.rad(degrees), 0)
task.wait()
end
end
PivotOffset
This property specifies the offset of the part's pivot from its CFrame, that is part:GetPivot() is the same as part.CFrame * part.PivotOffset.
This is convenient for setting the pivot to a location in local space, but setting a part's pivot to a location in world space can be done as follows:
local part = workspace.BluePartlocal desiredPivotCFrameInWorldSpace = CFrame.new(0, 10, 0)part.PivotOffset = part.CFrame:ToObjectSpace(desiredPivotCFrameInWorldSpace)
Code Samples
local function resetPivot(model)
local boundsCFrame = model:GetBoundingBox()
if model.PrimaryPart then
model.PrimaryPart.PivotOffset = model.PrimaryPart.CFrame:ToObjectSpace(boundsCFrame)
else
model.WorldPivot = boundsCFrame
end
end
resetPivot(script.Parent)
local function createHand(length, width, yOffset)
local part = Instance.new("Part")
part.Size = Vector3.new(width, 0.1, length)
part.Material = Enum.Material.Neon
part.PivotOffset = CFrame.new(0, -(yOffset + 0.1), length / 2)
part.Anchored = true
part.Parent = workspace
return part
end
local function positionHand(hand, fraction)
hand:PivotTo(CFrame.fromEulerAnglesXYZ(0, -fraction * 2 * math.pi, 0))
end
-- Create dial
for i = 0, 11 do
local dialPart = Instance.new("Part")
dialPart.Size = Vector3.new(0.2, 0.2, 1)
dialPart.TopSurface = Enum.SurfaceType.Smooth
if i == 0 then
dialPart.Size = Vector3.new(0.2, 0.2, 2)
dialPart.Color = Color3.new(1, 0, 0)
end
dialPart.PivotOffset = CFrame.new(0, -0.1, 10.5)
dialPart.Anchored = true
dialPart:PivotTo(CFrame.fromEulerAnglesXYZ(0, (i / 12) * 2 * math.pi, 0))
dialPart.Parent = workspace
end
-- Create hands
local hourHand = createHand(7, 1, 0)
local minuteHand = createHand(10, 0.6, 0.1)
local secondHand = createHand(11, 0.2, 0.2)
-- Run clock
while true do
local components = os.date("*t")
positionHand(hourHand, (components.hour + components.min / 60) / 12)
positionHand(minuteHand, (components.min + components.sec / 60) / 60)
positionHand(secondHand, components.sec / 60)
task.wait()
end
Position
The Position property describes the coordinates of a part using a Vector3. It reflects the position of the part's BasePart.CFrame, however it can also be set.
When setting this property any Welds or Motor6Ds connected to this part will have the matching C0 or C1 property updated and to allow the part to move relative to any other parts it is joined to.
WeldConstraints will also be temporarily disabled and re-enabled during the move.
ReceiveAge
This returns the time in seconds since the part's physics got last updated on the local client (or the server). Returns 0 when the part has no physics (Anchored)
Reflectance
The Reflectance property determines how much a part reflects the skybox. A value of 0 indicates the part is not reflective at all, and a value of 1 indicates the part should fully reflect.
Reflectance is not affected by BasePart.Transparency, unless the part is fully transparent, in which case reflectance will not render at all. Reflectance may or may not be ignored depending on the BasePart.Material of the part.
Code Samples
local part = script.Parent
local pointLight = Instance.new("PointLight")
pointLight.Brightness = 0
pointLight.Range = 12
pointLight.Parent = part
local touchNo = 0
local function blink()
-- Advance touchNo to tell other blink() calls to stop early
touchNo = touchNo + 1
-- Save touchNo locally so we can tell when it changes globally
local myTouchNo = touchNo
for i = 1, 0, -0.1 do
-- Stop early if another blink started
if touchNo ~= myTouchNo then
break
end
-- Update the blink animation
part.Reflectance = i
pointLight.Brightness = i * 2
task.wait(0.05)
end
end
part.Touched:Connect(blink)
ResizeIncrement
The ResizeIncrement property is a read-only property that describes the smallest change in size allowable by the BasePart:Resize() method. It differs between implementations of the BasePart abstract class. For instance, Part has this set to 1 and TrussPart has this set to 2 (since individual truss sections are 2x2x2 in size).
Code Samples
-- Put this Script in several kinds of BasePart, like
-- Part, TrussPart, WedgePart, CornerWedgePart, etc.
local part = script.Parent
-- Create a handles object for this part
local handles = Instance.new("Handles")
handles.Adornee = part
handles.Parent = part
-- Manually specify the faces applicable for this handle
handles.Faces = Faces.new(Enum.NormalId.Top, Enum.NormalId.Front, Enum.NormalId.Left)
-- Alternatively, use the faces on which the part can be resized.
-- If part is a TrussPart with only two Size dimensions
-- of length 2, then ResizeableFaces will only have two
-- enabled faces. For other parts, all faces will be enabled.
handles.Faces = part.ResizeableFaces
ResizeableFaces
The ResizeableFaces property (with an e, not ResizableFaces) describes using a Faces object the different faces on which a part may be resized. For most implementations of BasePart, such as Part and WedgePart, this property includes all faces. However, TrussPart will set its ResizeableFaces set to only two faces since those kinds of parts must have two BasePart.Size dimensions of length 2. This property is most commonly used with tools used for building and manipulating parts and has little use outside of that context. The Handles class, which has the Handles.Faces property, can be used in conjunction with this property to display only the handles on faces that can be resized on a part.
Code Samples
-- Put this Script in several kinds of BasePart, like
-- Part, TrussPart, WedgePart, CornerWedgePart, etc.
local part = script.Parent
-- Create a handles object for this part
local handles = Instance.new("Handles")
handles.Adornee = part
handles.Parent = part
-- Manually specify the faces applicable for this handle
handles.Faces = Faces.new(Enum.NormalId.Top, Enum.NormalId.Front, Enum.NormalId.Left)
-- Alternatively, use the faces on which the part can be resized.
-- If part is a TrussPart with only two Size dimensions
-- of length 2, then ResizeableFaces will only have two
-- enabled faces. For other parts, all faces will be enabled.
handles.Faces = part.ResizeableFaces
RightSurface
The RightSurface property determines the type of surface used for the +X direction of a part. When two parts' faces are placed next to each other, they may create a joint between them. If set to Motor, the BasePart.RightSurfaceInput determines how a motor joint should behave.
Most SurfaceTypes render a texture on the part face if the BasePart.Material is set to Plastic. Some SurfaceTypes including Hinge, Motor, and SteppingMotor will render a 3D adornment instead. If this property is selected in the Properties window, it will be highlighted in the game world similar to that of a SurfaceSelection.
Code Samples
local demoPart = script.Parent
-- Create a billboard gui to display what the current surface type is
local billboard = Instance.new("BillboardGui")
billboard.AlwaysOnTop = true
billboard.Size = UDim2.new(0, 200, 0, 50)
billboard.Adornee = demoPart
local textLabel = Instance.new("TextLabel")
textLabel.Size = UDim2.new(0, 200, 0, 50)
textLabel.BackgroundTransparency = 1
textLabel.TextStrokeTransparency = 0
textLabel.TextColor3 = Color3.new(1, 1, 1) -- White
textLabel.Parent = billboard
billboard.Parent = demoPart
local function setAllSurfaces(part, surfaceType)
part.TopSurface = surfaceType
part.BottomSurface = surfaceType
part.LeftSurface = surfaceType
part.RightSurface = surfaceType
part.FrontSurface = surfaceType
part.BackSurface = surfaceType
end
while true do
-- Iterate through the different SurfaceTypes
for _, enum in pairs(Enum.SurfaceType:GetEnumItems()) do
textLabel.Text = enum.Name
setAllSurfaces(demoPart, enum)
task.wait(1)
end
end
RootPriority
This property is an integer between -127 and 127 that takes precedence over all other rules for root part sort. When considering multiple parts that are not Anchored and which share the same Massless value, a part with a higher RootPriority will take priority over those with lower RootPriority.
You can use this property to control which part of an assembly is the root part and keep the root part stable if size changes.
See also Assemblies, an article documenting what root parts are and how to use them.
Rotation
The rotation of the part in degrees for the three axes.
When setting this property any Welds or Motor6Ds connected to this part will have the matching C0 or C1 property updated and to allow the part to move relative to any other parts it is joined to.
WeldConstraints will also be temporarily disabled and re-enabled during the move.
Size
A part's Size property determines its visual dimensions, while ExtentsSize represents the actual size used by the physics engine, such as in collision detection. The individual dimensions (length, width, height) can be as low as 0.001 and as high as 2048. Size dimensions below 0.05 will be visually represented as if the part's dimensions are 0.05.
The size of the part determines its mass which is given by BasePart:GetMass(). A part's Size is used by a variety of other objects:
- ParticleEmitter to determine the area from which particles are spawned.
- BlockMesh to partially determine the rendered rectangular prism.
- SpecialMesh for certain MeshTypes, to determine the size of the rendered mesh.
- SurfaceLight to determine the space to illuminate.
Code Samples
local TOWER_BASE_SIZE = 30
local position = Vector3.new(50, 50, 50)
local hue = math.random()
local color0 = Color3.fromHSV(hue, 1, 1)
local color1 = Color3.fromHSV((hue + 0.35) % 1, 1, 1)
local model = Instance.new("Model")
model.Name = "Tower"
for i = TOWER_BASE_SIZE, 1, -2 do
local part = Instance.new("Part")
part.Size = Vector3.new(i, 2, i)
part.Position = position
part.Anchored = true
part.Parent = model
-- Tween from color0 and color1
local perc = i / TOWER_BASE_SIZE
part.Color = Color3.new(
color0.R * perc + color1.R * (1 - perc),
color0.G * perc + color1.G * (1 - perc),
color0.B * perc + color1.B * (1 - perc)
)
position = position + Vector3.new(0, part.Size.Y, 0)
end
model.Parent = workspace
TopSurface
The TopSurface property determines the type of surface used for the +Y direction of a part. When two parts' faces are placed next to each other, they may create a joint between them. If set to Motor, the BasePart.TopSurfaceInput determines how a motor joint should behave.
Most SurfaceTypes render a texture on the part face if the BasePart.Material is set to Plastic. Some SurfaceTypes - Hinge, Motor and SteppingMotor - will render a 3D adornment instead. If this property is selected in the Properties window, it will be highlighted in the game world similar to that of a SurfaceSelection.
Code Samples
local demoPart = script.Parent
-- Create a billboard gui to display what the current surface type is
local billboard = Instance.new("BillboardGui")
billboard.AlwaysOnTop = true
billboard.Size = UDim2.new(0, 200, 0, 50)
billboard.Adornee = demoPart
local textLabel = Instance.new("TextLabel")
textLabel.Size = UDim2.new(0, 200, 0, 50)
textLabel.BackgroundTransparency = 1
textLabel.TextStrokeTransparency = 0
textLabel.TextColor3 = Color3.new(1, 1, 1) -- White
textLabel.Parent = billboard
billboard.Parent = demoPart
local function setAllSurfaces(part, surfaceType)
part.TopSurface = surfaceType
part.BottomSurface = surfaceType
part.LeftSurface = surfaceType
part.RightSurface = surfaceType
part.FrontSurface = surfaceType
part.BackSurface = surfaceType
end
while true do
-- Iterate through the different SurfaceTypes
for _, enum in pairs(Enum.SurfaceType:GetEnumItems()) do
textLabel.Text = enum.Name
setAllSurfaces(demoPart, enum)
task.wait(1)
end
end
Transparency
The Transparency property controls the visibility of a part on a scale of 0 to 1, where 0 is completely visible (opaque), and a value of 1 is completely invisible (not rendered at all).
BasePart.Reflectance can reduce the overall transparency of a brick if set to a value close to 1.
While fully transparent parts are not rendered at all, partially transparent objects have some significant rendering costs. Having many translucent parts may slow down the game's performance.
When transparent parts overlap, render order can act unpredictable - try to keep semi-transparent parts from overlapping to avoid this.
The BasePart.LocalTransparencyModifier is a multiplier to Transparency that is only visible to the local client.
Code Samples
-- Paste into a Script inside a tall part
local part = script.Parent
local OPEN_TIME = 1
-- Can the door be opened at the moment?
local debounce = false
local function open()
part.CanCollide = false
part.Transparency = 0.7
part.BrickColor = BrickColor.new("Black")
end
local function close()
part.CanCollide = true
part.Transparency = 0
part.BrickColor = BrickColor.new("Bright blue")
end
local function onTouch(otherPart)
-- If the door was already open, do nothing
if debounce then
print("D")
return
end
-- Check if touched by a Humanoid
local human = otherPart.Parent:FindFirstChildOfClass("Humanoid")
if not human then
print("not human")
return
end
-- Perform the door opening sequence
debounce = true
open()
task.wait(OPEN_TIME)
close()
debounce = false
end
part.Touched:Connect(onTouch)
close()
local function makeXRayPart(part)
-- LocalTransparencyModifier will make parts see-through but only for the local
-- client, and it won't replicate to the server
part.LocalTransparencyModifier = 0.5
end
-- This function uses recursion to search for parts in the game
local function recurseForParts(object)
if object:IsA("BasePart") then
makeXRayPart(object)
end
-- Stop if this object has a Humanoid - we don't want to see-through players!
if object:FindFirstChildOfClass("Humanoid") then
return
end
-- Check the object's children for more parts
for _, child in pairs(object:GetChildren()) do
recurseForParts(child)
end
end
recurseForParts(workspace)
Methods
AngularAccelerationToTorque
Parameters
Returns
ApplyAngularImpulse
Applies an instant angular force impulse to this part's assembly, causing the assembly to spin.
The resulting angular velocity from the impulse relies on the assembly's mass. So a higher impulse is required to move more massive assemblies. Impulses are useful for cases where you want a force applied instantly, such as an explosion or collision.
If the part is owned by the server, this function must be called from a server Script (not from a LocalScript or a Script with RunContext set to Enum.RunContext.Client). If the part is owned by a client through automatic ownership, this function can be called from either a client script or a server script; calling it from a client script for a server-owned part will have no effect.
Parameters
A force vector to be applied to the assembly as an impulse.
Returns
ApplyImpulse
This function applies an instant force impulse to this part's assembly.
The force is applied at the assembly's center of mass, so the resulting movement will only be linear.
The resulting velocity from the impulse relies on the assembly's mass. So a higher impulse is required to move more massive assemblies. Impulses are useful for cases where you want a force applied instantly, such as an explosion or collision.
If the part is owned by the server, this function must be called from a server Script (not from a LocalScript or a Script with RunContext set to Enum.RunContext.Client). If the part is owned by a client through automatic ownership, this function can be called from either a client script or a server script; calling it from a client script for a server-owned part will have no effect.
Parameters
A force vector to be applied to the assembly as an impulse.
Returns
ApplyImpulseAtPosition
This function applies an instant force impulse to this part's assembly, at the specified position in world space.
If the position is not at the assembly's center of mass, the impulse will cause a positional and rotational movement.
The resulting velocity from the impulse relies on the assembly's mass. So a higher impulse is required to move more massive assemblies. Impulses are useful for cases where developers want a force applied instantly, such as an explosion or collision.
If the part is owned by the server, this function must be called from a server Script (not from a LocalScript or a Script with RunContext set to Enum.RunContext.Client). If the part is owned by a client through automatic ownership, this function can be called from either a client script or a server script; calling it from a client script for a server-owned part will have no effect.
Parameters
A force vector to be applied to the assembly as an impulse.
The position, in world space, to apply the impulse.
Returns
CanCollideWith
Returns whether the parts can collide with each other or not. This function takes into account the collision groups of the two parts. This function will error if the specified part is not a BasePart.
Parameters
The specified part being checked for collidability.
Returns
Whether the parts can collide with each other.
CanSetNetworkOwnership
The CanSetNetworkOwnership function checks whether you can set a part's network ownership.
The function's return value verifies whether or not you can call BasePart:SetNetworkOwner() or BasePart:SetNetworkOwnershipAuto() without encountering an error. It returns true if you can modify/read the network ownership, or returns false and the reason you can't, as a string.
Returns
Whether you can modify or read the network ownership and the reason.
Code Samples
local part = workspace:FindFirstChild("Part")
if part and part:IsA("BasePart") then
local canSet, errorReason = part:CanSetNetworkOwnership()
if canSet then
print(part:GetFullName() .. "'s Network Ownership can be changed!")
else
warn("Cannot change the Network Ownership of " .. part:GetFullName() .. " because: " .. errorReason)
end
end
GetConnectedParts
Returns a table of parts connected to the object by any kind of rigid joint.
If recursive is true this function will return all of the parts in the assembly rigidly connected to the BasePart.
Rigid Joints
When a joint connects two parts together (Part0 → Part1), a joint is rigid if the physics of Part1 are completely locked down by Part0. This only applies to the following joint types:
Parameters
Returns
GetJoints
Return all Joints or Constraints that is connected to this Part.
Returns
An array of all Joints or Constraints connected to the Part.
GetMass
GetMass returns the value of the read-only Mass property.
This function predates the Mass property. It remains supported for backward-compatibility; you should use the Mass property directly.
Returns
The part's mass.
Code Samples
local myPart = Instance.new("Part")
myPart.Size = Vector3.new(4, 6, 4)
myPart.Anchored = true
myPart.Parent = workspace
local myMass = myPart:GetMass()
print("My part's mass is " .. myMass)
GetNetworkOwner
Returns the current player who is the network owner of this part, or nil in case of the server.
Returns
The current player who is the network owner of this part, or nil in case of the server.
GetNetworkOwnershipAuto
Returns true if the game engine automatically decides the network owner for this part.
Returns
Whether the game engine automatically decides the network owner for this part.
GetNoCollisionConstraints
Returns
GetRootPart
Returns the base part of an assembly. When moving an assembly of parts using a CFrame. it is important to move this base part (this will move all other parts connected to it accordingly). More information is available in the Assemblies article.
This function predates the AssemblyRootPart property. It remains supported for backwards compatibility, but you should use AssemblyRootPart directly.
Returns
The base part of an assembly (a collection of parts connected together).
GetTouchingParts
Returns a table of all parts that are physically interacting with this part. If the part itself has CanCollide set to false, then this function returns an empty table unless the part has a TouchInterest object parented to it (meaning something is connected to its Touched event). Parts that are adjacent but not intersecting are not considered touching. This function predates the WorldRoot:GetPartsInPart() function, which provides more flexibility and avoids the special TouchInterest rules described above. Use WorldRoot:GetPartsInPart() instead.
Returns
A table of all parts that intersect and can collide with this part.
GetVelocityAtPosition
Returns the linear velocity of the part's assembly at the given position relative to this part. It can be used to identify the linear velocity of parts in an assembly other than the root part. If the assembly has no angular velocity, than the linear velocity will always be the same for every position.
Parameters
Returns
IsGrounded
Returns true if the object is connected to a part that will hold it in place (eg an Anchored part), otherwise returns false. In an assembly that has an Anchored part, every other part is grounded.
Returns
Whether the object is connected to a part that will hold it in place.
Resize
Changes the size of an object just like using the Studio resize tool.
Parameters
The side to resize.
How much to grow/shrink on the specified side.
Returns
Whether the part is resized.
SetNetworkOwner
Sets the given player as network owner for this and all connected parts. When playerInstance is nil, the server will be the owner instead of a player.
Parameters
The player being given network ownership of the part.
Returns
SetNetworkOwnershipAuto
Lets the game engine dynamically decide who will handle the part's physics (one of the clients or the server).
Returns
TorqueToAngularAcceleration
Parameters
Returns
IntersectAsync
Creates a new IntersectOperation from the intersecting geometry of the part and the other parts in the given array. Only Parts are supported, not Terrain or MeshParts. Similar to Clone(), the returned object has no set Parent.
The following properties from the calling part are applied to the resulting IntersectOperation:
In the following image comparison, IntersectAsync() is called on the purple block using a table containing the blue block. The resulting IntersectOperation resolves into a shape of the intersecting geometry of both parts.
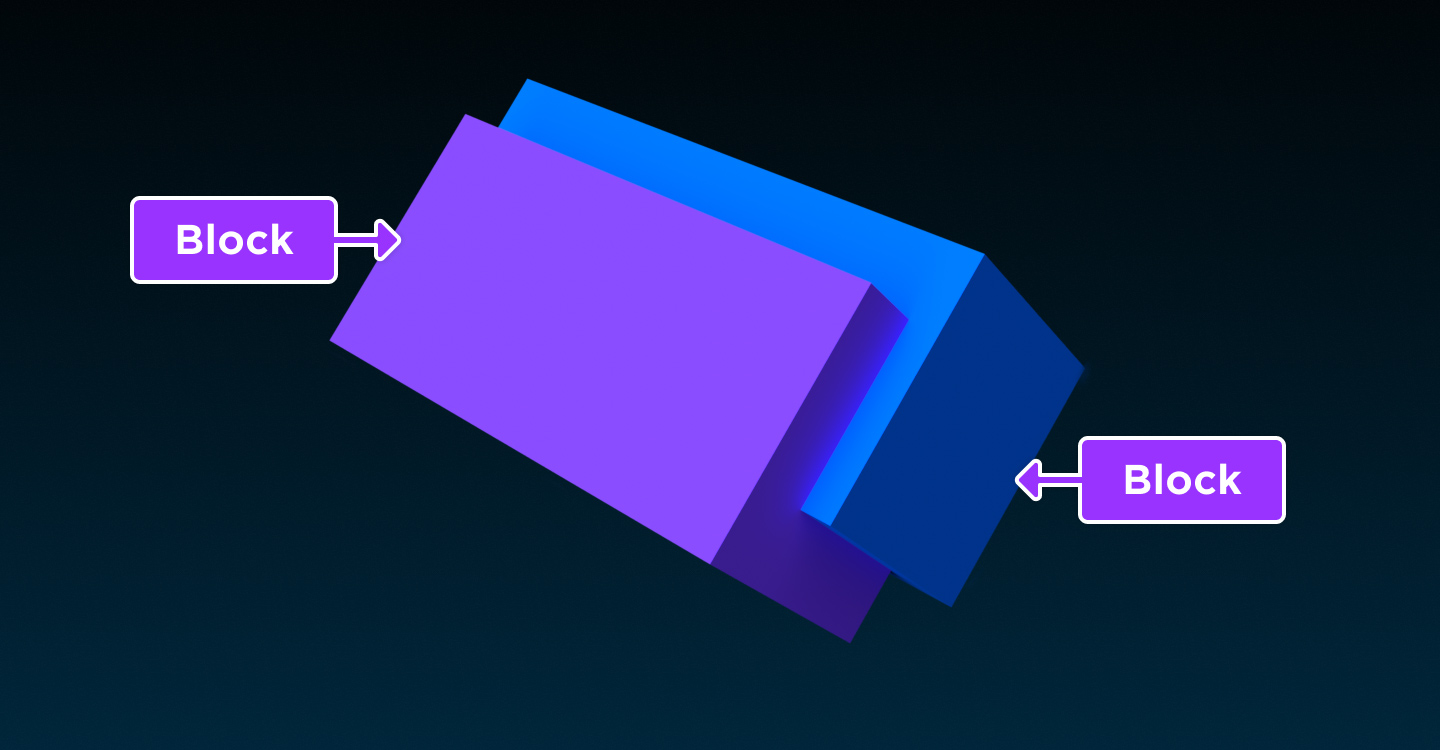
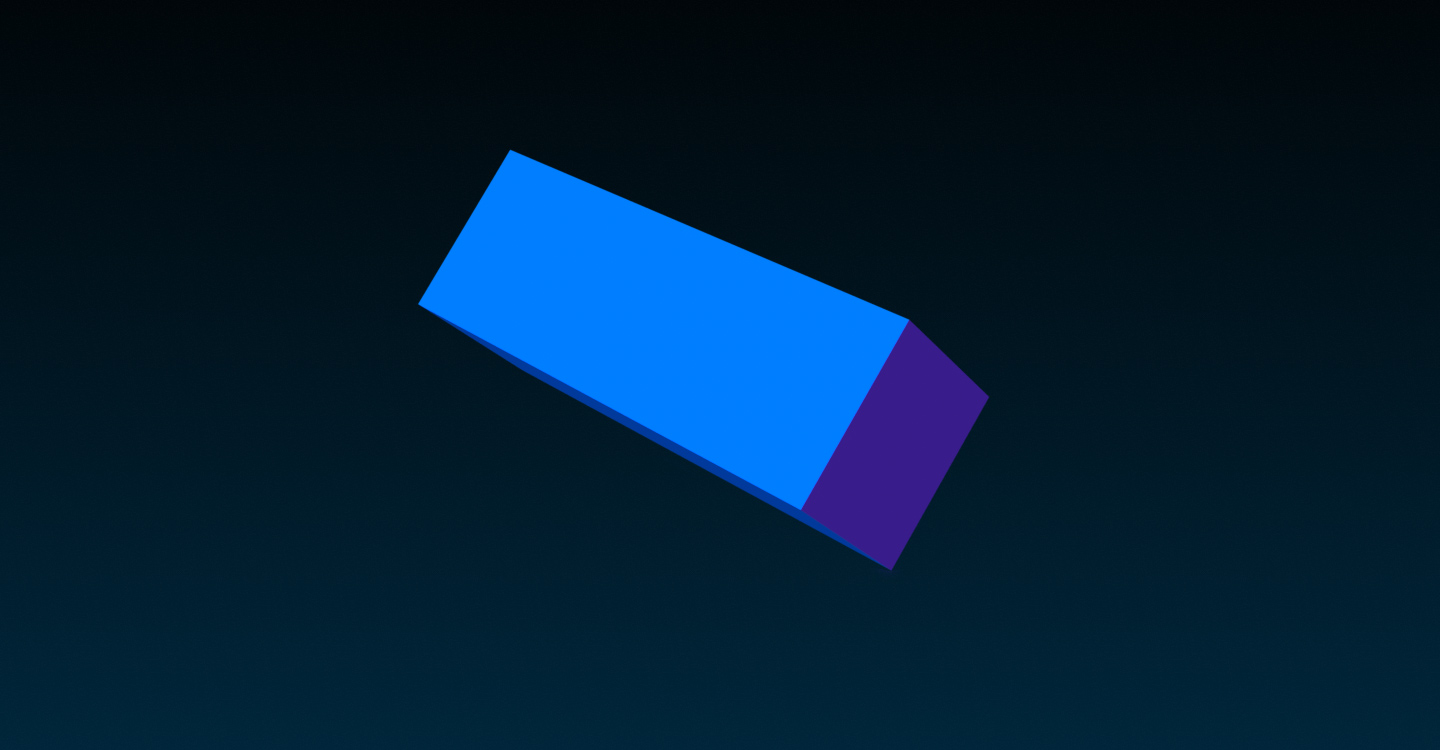
Notes
- The original parts remain intact following a successful intersect operation. In most cases, you should Destroy() all of the original parts and parent the returned IntersectOperation to the same place as the calling BasePart.
- By default, the face colors of the resulting intersection are borrowed from the Color property of the original parts. To change the entire intersection to a specific color, set its UsePartColor property to true.
- If an intersect operation would result in a part with more than 20,000 triangles, it will be simplified to 20,000 triangles.
Parameters
The objects taking part in the intersection.
The Enum.CollisionFidelity value for the resulting IntersectOperation.
The Enum.RenderFidelity value of the resulting PartOperation.
Returns
Resulting IntersectOperation with default name Intersect.
SubtractAsync
Creates a new UnionOperation from the part, minus the geometry occupied by the parts in the given array. Only Parts are supported, not Terrain or MeshParts. Similar to Clone(), the returned object has no set Parent.
Note that the resulting union cannot be empty due to subtractions. If the operation would result in completely empty geometry, it will fail.
In the following image comparison, SubtractAsync() is called on the blue cylinder using a table containing the purple block. The resulting UnionOperation resolves into a shape that omits the block's geometry from that of the cylinder.
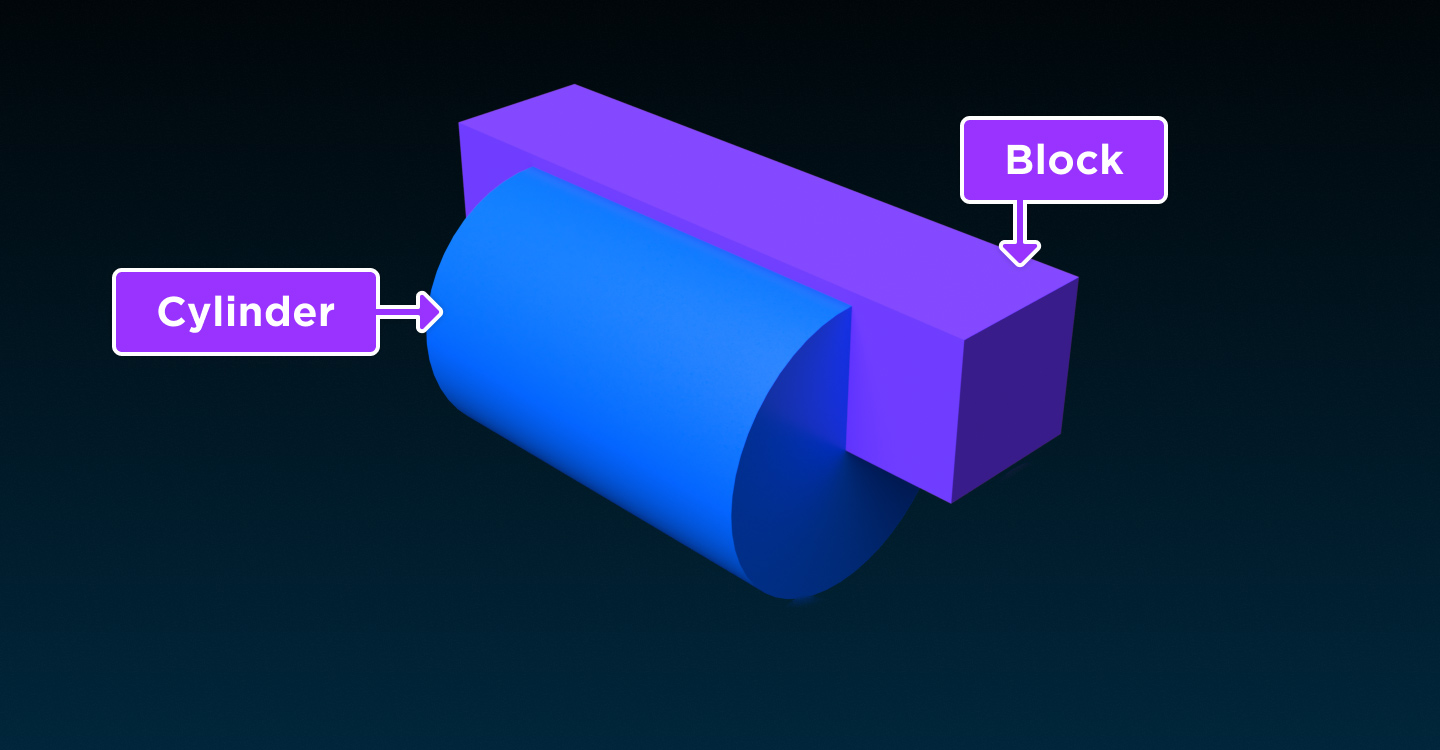
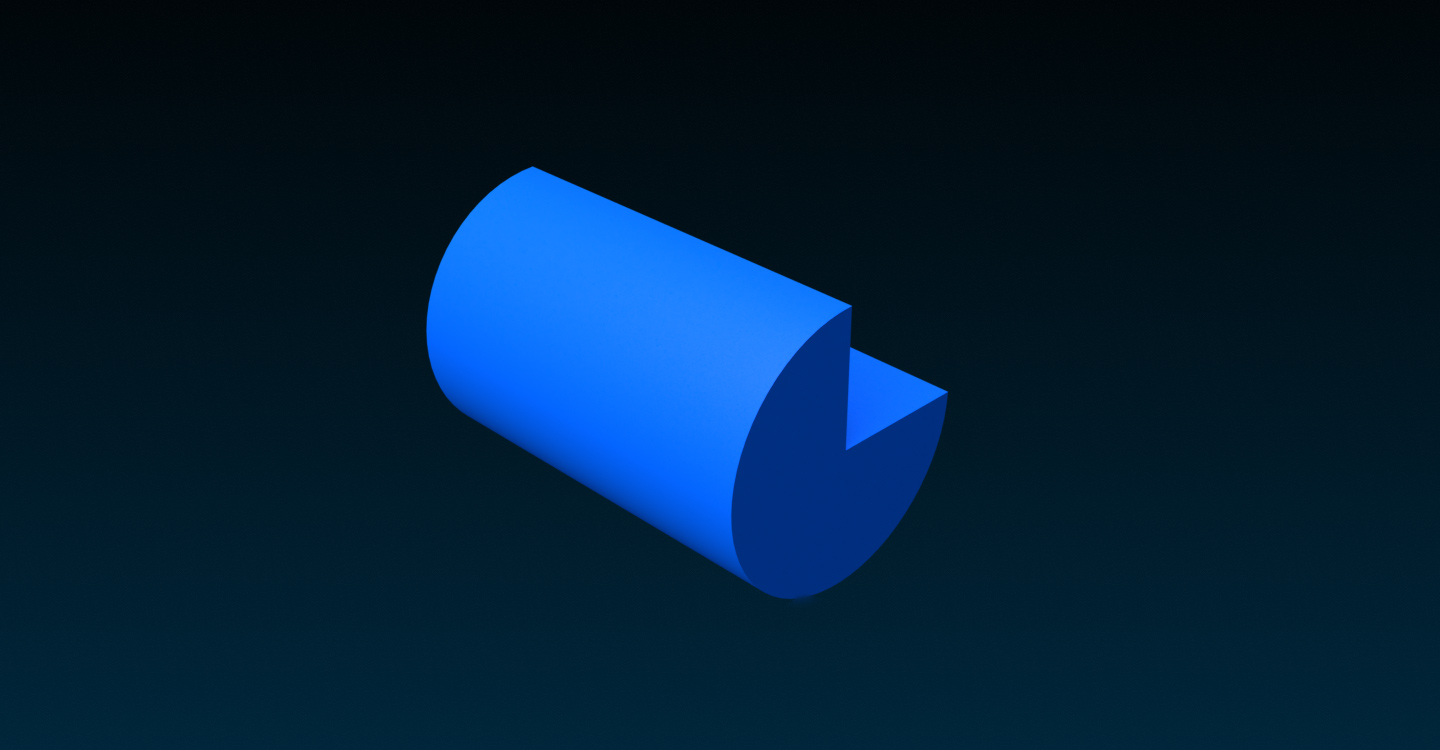
Parameters
The objects taking part in the subtraction.
The Enum.CollisionFidelity value for the resulting UnionOperation.
The Enum.RenderFidelity value of the resulting PartOperation.
Returns
Resulting UnionOperation with default name Union.
Code Samples
local Workspace = game:GetService("Workspace")
local mainPart = script.Parent.PartA
local otherParts = { script.Parent.PartB, script.Parent.PartC }
-- Perform subtract operation
local success, newSubtract = pcall(function()
return mainPart:SubtractAsync(otherParts)
end)
-- If operation succeeds, position it at the same location and parent it to the workspace
if success and newSubtract then
newSubtract.Position = mainPart.Position
newSubtract.Parent = Workspace
end
-- Destroy original parts which remain intact after operation
mainPart:Destroy()
for _, part in otherParts do
part:Destroy()
end
UnionAsync
Creates a new UnionOperation from the part, plus the geometry occupied by the parts in the given array. Only Parts are supported, not Terrain or MeshParts. Similar to Clone(), the returned object has no set Parent.
The following properties from the calling part are applied to the resulting UnionOperation:
In the following image comparison, UnionAsync() is called on the blue block using a table containing the purple cylinder. The resulting UnionOperation resolves into a shape of the combined geometry of both parts.
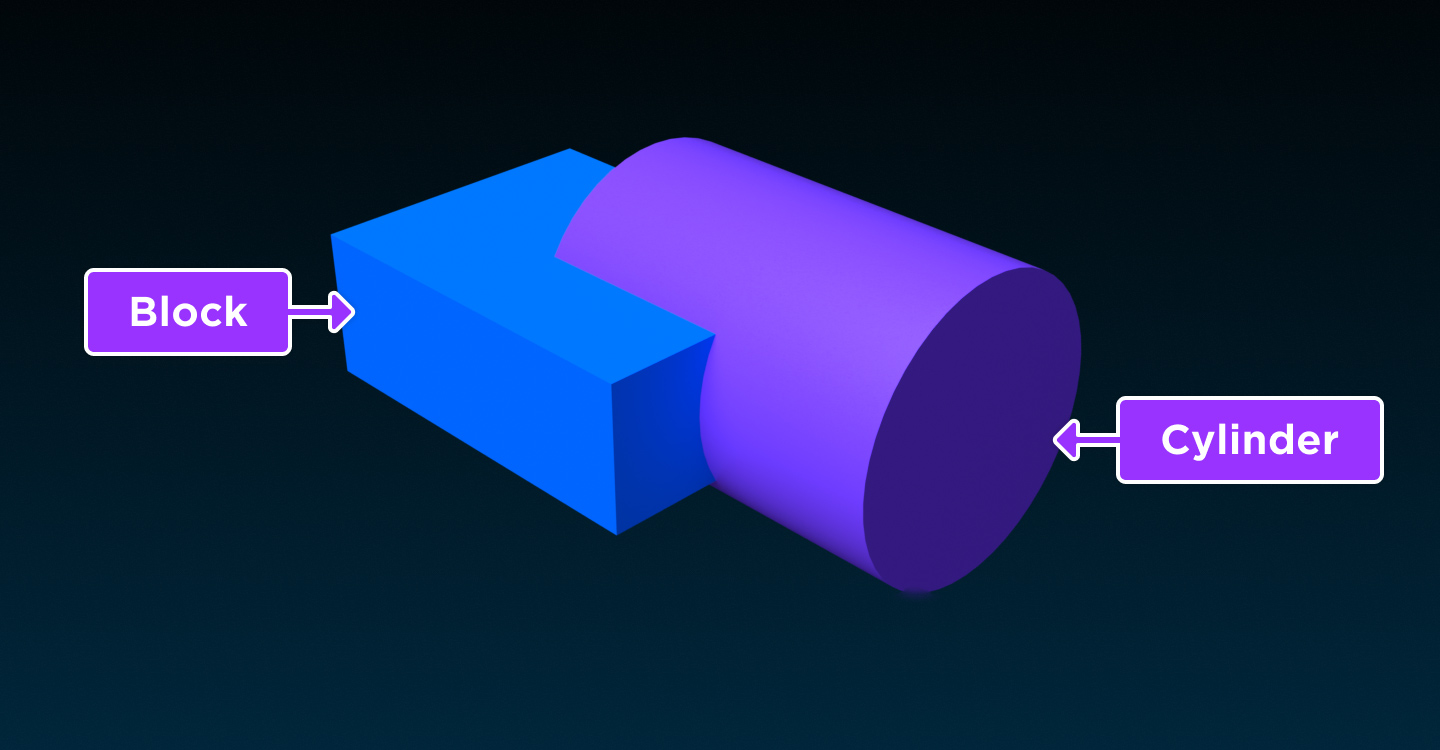
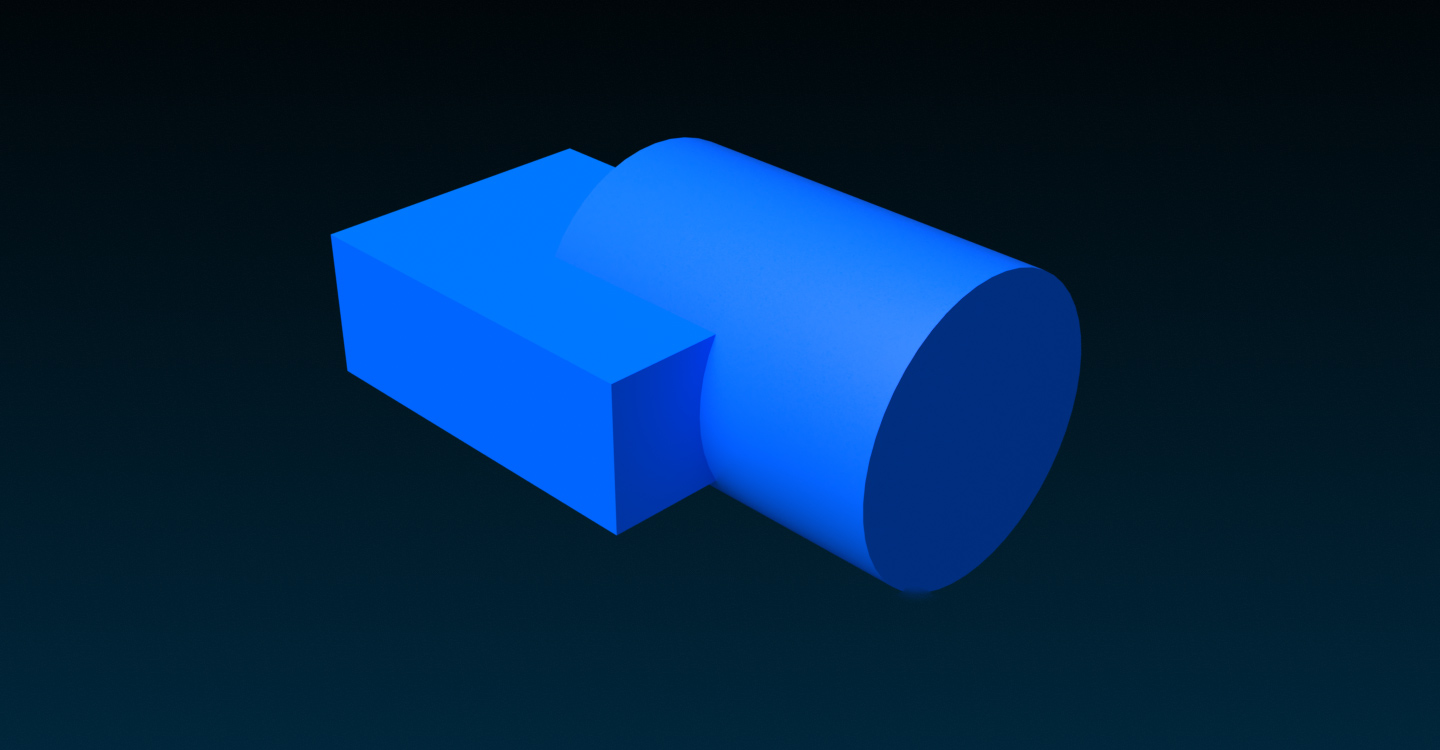
Notes
- The original parts remain intact following a successful union operation. In most cases, you should Destroy() all of the original parts and parent the returned UnionOperation to the same place as the calling BasePart.
- By default, the resulting union respects the Color property of each of its parts. To change the entire union to a specific color, set its UsePartColor property to true.
- If a union operation would result in a part with more than 20,000 triangles, it will be simplified to 20,000 triangles.
Parameters
The objects taking part in the union with the calling part.
The Enum.CollisionFidelity value for the resulting UnionOperation.
The Enum.RenderFidelity value of the resulting PartOperation.
Returns
Resulting UnionOperation with default name Union.
Code Samples
local Workspace = game:GetService("Workspace")
local mainPart = script.Parent.PartA
local otherParts = { script.Parent.PartB, script.Parent.PartC }
-- Perform union operation
local success, newUnion = pcall(function()
return mainPart:UnionAsync(otherParts)
end)
-- If operation succeeds, position it at the same location and parent it to the workspace
if success and newUnion then
newUnion.Position = mainPart.Position
newUnion.Parent = Workspace
end
-- Destroy original parts which remain intact after operation
mainPart:Destroy()
for _, part in otherParts do
part:Destroy()
end
Events
TouchEnded
Fires when a part stops touching another part under similar conditions to those of BasePart.Touched.
This event works in conjunction with Workspace.TouchesUseCollisionGroups to specify whether collision groups are acknowledged for detection.
Parameters
Code Samples
local part = script.Parent
local billboardGui = Instance.new("BillboardGui")
billboardGui.Size = UDim2.new(0, 200, 0, 50)
billboardGui.Adornee = part
billboardGui.AlwaysOnTop = true
billboardGui.Parent = part
local tl = Instance.new("TextLabel")
tl.Size = UDim2.new(1, 0, 1, 0)
tl.BackgroundTransparency = 1
tl.Parent = billboardGui
local numTouchingParts = 0
local function onTouch(otherPart)
print("Touch started: " .. otherPart.Name)
numTouchingParts = numTouchingParts + 1
tl.Text = numTouchingParts
end
local function onTouchEnded(otherPart)
print("Touch ended: " .. otherPart.Name)
numTouchingParts = numTouchingParts - 1
tl.Text = numTouchingParts
end
part.Touched:Connect(onTouch)
part.TouchEnded:Connect(onTouchEnded)
Touched
The Touched event fires when a part comes in contact with another part. For instance, if PartA bumps into PartB, then PartA.Touched fires with PartB, and PartB.Touched fires with PartA.
This event only fires as a result of physical movement, so it will not fire if the CFrame property was changed such that the part overlaps another part. This also means that at least one of the parts involved must not be Anchored at the time of the collision.
This event works in conjunction with Workspace.TouchesUseCollisionGroups to specify whether collision groups are acknowledged for detection.
Parameters
The other part that came in contact with the given part.
Code Samples
local part = script.Parent
local billboardGui = Instance.new("BillboardGui")
billboardGui.Size = UDim2.new(0, 200, 0, 50)
billboardGui.Adornee = part
billboardGui.AlwaysOnTop = true
billboardGui.Parent = part
local tl = Instance.new("TextLabel")
tl.Size = UDim2.new(1, 0, 1, 0)
tl.BackgroundTransparency = 1
tl.Parent = billboardGui
local numTouchingParts = 0
local function onTouch(otherPart)
print("Touch started: " .. otherPart.Name)
numTouchingParts = numTouchingParts + 1
tl.Text = numTouchingParts
end
local function onTouchEnded(otherPart)
print("Touch ended: " .. otherPart.Name)
numTouchingParts = numTouchingParts - 1
tl.Text = numTouchingParts
end
part.Touched:Connect(onTouch)
part.TouchEnded:Connect(onTouchEnded)
local model = script.Parent
local function onTouched(otherPart)
-- Ignore instances of the model coming in contact with itself
if otherPart:IsDescendantOf(model) then return end
print(model.Name .. " collided with " .. otherPart.Name)
end
for _, child in pairs(model:GetChildren()) do
if child:IsA("BasePart") then
child.Touched:Connect(onTouched)
end
end