Beam
A Beam object connects two Attachments by drawing a texture between them.
To display, a beam must be a descendant of the Workspace with its Attachment0 and Attachment1 properties set to Attachments also descending from the Workspace.
The beam's appearance can be customized using the range of properties outlined below. Also see the Beams guide for visual examples.
Beam Curvature
Beams are configured to use a cubic Bézier curve formed by four control points. This means they are not constrained to straight lines and the curve of the beam can be modified by changing CurveSize0, CurveSize1, and the orientation of the beam's Attachments.
- P0 — The start of the beam; position of Attachment0.
- P3 — The end of the beam; position of Attachment1
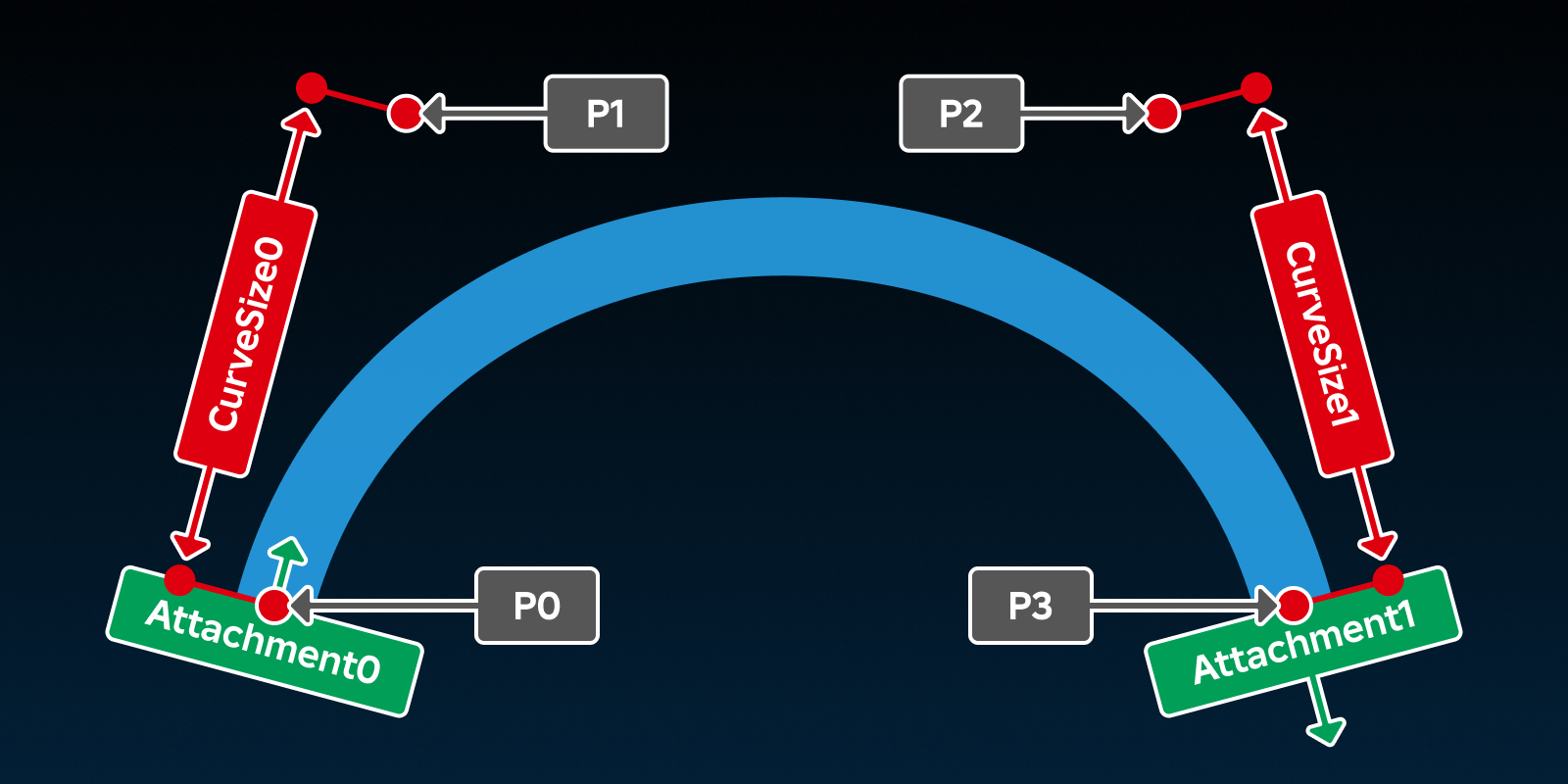
Code Samples
This code sample demonstrates how a Beam effect can be created from scratch by creating a Beam, setting all of its properties and configuring it's Attachments.
-- create attachments
local att0 = Instance.new("Attachment")
local att1 = Instance.new("Attachment")
-- parent to terrain (can be part instead)
att0.Parent = workspace.Terrain
att1.Parent = workspace.Terrain
-- position attachments
att0.Position = Vector3.new(0, 10, 0)
att1.Position = Vector3.new(0, 10, 10)
-- create beam
local beam = Instance.new("Beam")
beam.Attachment0 = att0
beam.Attachment1 = att1
-- appearance properties
beam.Color = ColorSequence.new({ -- a color sequence shifting from white to blue
ColorSequenceKeypoint.new(0, Color3.fromRGB(255, 255, 255)),
ColorSequenceKeypoint.new(1, Color3.fromRGB(0, 255, 255)),
})
beam.LightEmission = 1 -- use additive blending
beam.LightInfluence = 0 -- beam not influenced by light
beam.Texture = "rbxasset://textures/particles/sparkles_main.dds" -- a built in sparkle texture
beam.TextureMode = Enum.TextureMode.Wrap -- wrap so length can be set by TextureLength
beam.TextureLength = 1 -- repeating texture is 1 stud long
beam.TextureSpeed = 1 -- slow texture speed
beam.Transparency = NumberSequence.new({ -- beam fades out at the end
NumberSequenceKeypoint.new(0, 0),
NumberSequenceKeypoint.new(0.8, 0),
NumberSequenceKeypoint.new(1, 1),
})
beam.ZOffset = 0 -- render at the position of the beam without offset
-- shape properties
beam.CurveSize0 = 2 -- create a curved beam
beam.CurveSize1 = -2 -- create a curved beam
beam.FaceCamera = true -- beam is visible from every angle
beam.Segments = 10 -- default curve resolution
beam.Width0 = 0.2 -- starts small
beam.Width1 = 2 -- ends big
-- parent beam
beam.Enabled = true
beam.Parent = att0
Summary
Properties
The Attachment the beam originates from.
The Attachment the beam ends at.
Scales the light emitted from the beam when LightInfluence is less than 1.
Determines the color of the beam across its Segments.
Determines, along with Attachment0, the position of the second control point in the beam's Bézier curve.
Determines, along with Attachment1, the position of the third control point in the beam's Bézier curve.
Determines whether the beam is visible or not.
Determines whether the Segments of the beam will always face the camera, regardless of its orientation.
Determines to what degree the colors of the beam are blended with the colors behind it.
Determines the degree to which the beam is influenced by the environment's lighting.
Sets how many straight segments the beam is made up of.
The content ID of the texture to be displayed on the beam.
Sets the length of the beam's texture, dependent on TextureMode.
Determines the manner in which the Texture scales and repeats.
Determines the speed at which the Texture image moves along the beam.
Determines the transparency of the beam across its segments.
The width of the beam at its origin (Attachment0), in studs.
The width of the beam at its end (Attachment1), in studs.
The distance, in studs, the beam display is offset relative to the CurrentCamera.
Methods
Sets the current offset of the beam's texture cycle.
Properties
Attachment0
The Attachment the beam originates from. This attachment is the first control point on the beam's cubic Bézier curve; its orientation, alongside the CurveSize0 property, determines the position of the second control point. See Beams for more details.
For the Attachment where the beam ends, see Attachment1.
Attachment1
The Attachment the beam ends at. This attachment is the fourth and final control point on the beam's cubic Bézier curve; its orientation, alongside the CurveSize1 property, determines the position of the third control point. See Beams for more details.
For the Attachment where the beam originates from, see Attachment0.
Brightness
Scales the light emitted from the beam when LightInfluence is less than 1. This property is 1 by default and can set to any number within the range of 0 to 10000. Increasing the value of LightInfluence decreases the effect of this property's value.
Color
Determines the color of the beam across its Segments. If Texture is set, this color will be applied to the beam's texture. If no Texture is set, the Beam will appear as a solid line colored in accordance with this property.
This property is a ColorSequence, allowing the color to be configured to vary across the length of the beam. Consider the following ColorSequence which, when applied to a beam, would yield the pictured result.
local colorSequence = ColorSequence.new({ColorSequenceKeypoint.new(0, Color3.fromRGB(255, 0, 0)), -- RedColorSequenceKeypoint.new(0.5, Color3.fromRGB(0, 188, 203)), -- CyanColorSequenceKeypoint.new(1, Color3.fromRGB(196, 0, 255)), -- Purple})
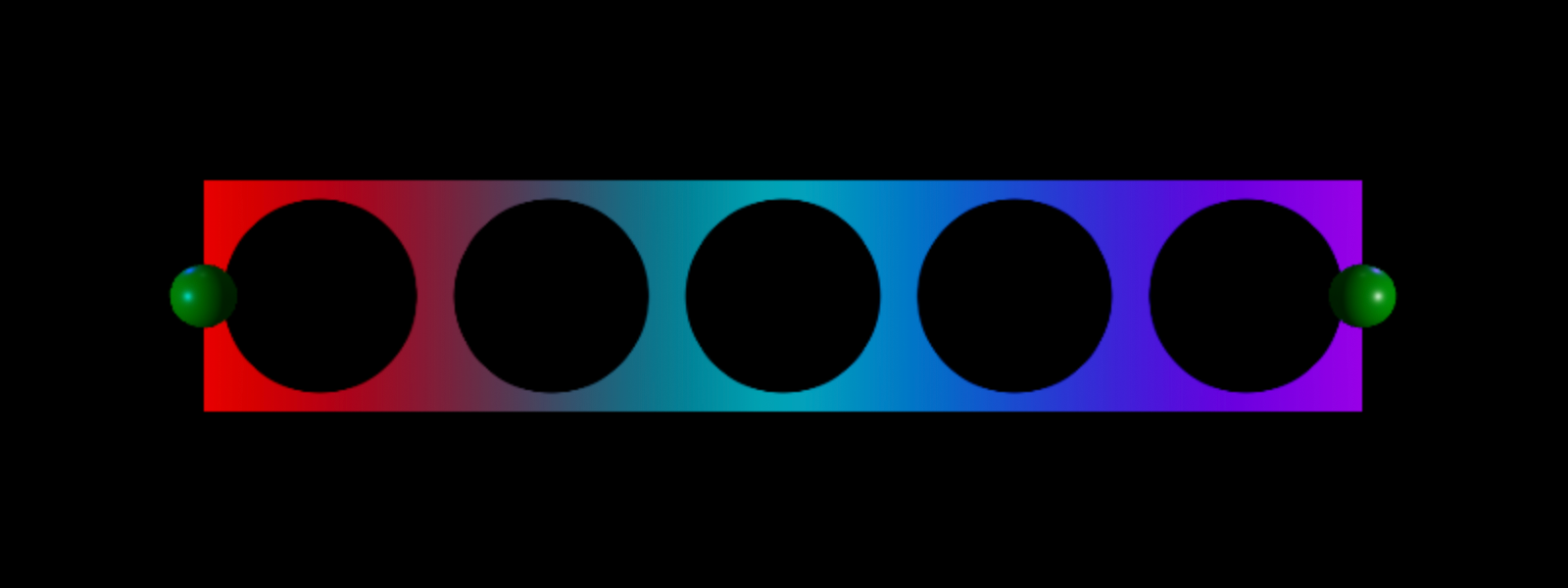
Note the beam's coloration also depends on the number of Segments the Beam has. Each segment of the beam can only show a transition between two colors. Therefore a Beam will need to have at least n-1 segments in order for the color to display correctly, where n is the number of ColorSequenceKeypoints in the ColorSequence.
CurveSize0
Determines, along with Attachment0, the position of the second control point in the beam's Bézier curve. See Beams for more details.
The position of this point can be determined by the following equation:
local controlPoint2 = Beam.Attachment0.WorldPosition + (Beam.Attachment0.CFrame.RightVector * Beam.CurveSize0)
CurveSize1
Determines, along with Attachment1, the position of the third control point in the beam's Bézier curve. See Beams for more details.
The position of this point can be determined by the following equation:
local controlPoint3 = Beam.Attachment1.WorldPosition - (Beam.Attachment1.CFrame.RightVector * Beam.CurveSize1)
Enabled
Determines whether the beam is visible or not.
When this property is set to false, the beam's Segments will not be displayed.
FaceCamera
A Beam is a 2D projection existing in 3D space, meaning that it may not be visible from every angle. The FaceCamera property, when set to true, ensures that the beam always faces the CurrentCamera, regardless of its orientation.
LightEmission
Determines to what degree the colors of the beam are blended with the colors behind it. It should be set in the range of 0 to 1. A value of 0 uses normal blending modes and a value of 1 uses additive blending.
This property should not be confused with LightInfluence which determines how the beam is affected by environmental light.
This property does not cause the beam to light the environment.
LightInfluence
Determines the degree to which the beam is influenced by the environment's lighting, clamped between 0 and 1. When 0, the beam will be unaffected by the environment's lighting. When 1, it will be fully affected by lighting as a BasePart would be.
See also LightEmission which specifies to what degree the colors of the beam are blended with the colors behind it.
LocalTransparencyModifier
Segments
Rather than being a perfect curve, a beam is made up of straight segments. The more segments, the higher the resolution of the curve. The Segments property sets how many straight segments the beam is made up of, with a default value of 10.
Note that the Color and Transparency properties require a certain number of segments to display correctly. This is because each segment can only show a transition between two colors or transparencies. Therefore a Beam requires at least n-1 segments to display correctly, where n is the number of keypoint associated with the beam's Color and Transparency.
Texture
The content ID of the texture to be displayed on the beam. If this property is not set, the beam will be displayed as a solid line; this also occurs when the texture is set to an invalid content ID or the image associated with the texture has not yet loaded.
The appearance of the texture can be further modified by other beam properties including Color and Transparency.
Scaling of the texture is determined by the TextureMode, TextureLength, Width0, and Width1 properties.
TextureMode
This property, alongside TextureLength, determines how a beam's Texture repeats.
When set to Enum.TextureMode.Wrap or Enum.TextureMode.Static, the texture repetitions will equal the beam's overall length (in studs) divided by its TextureLength.
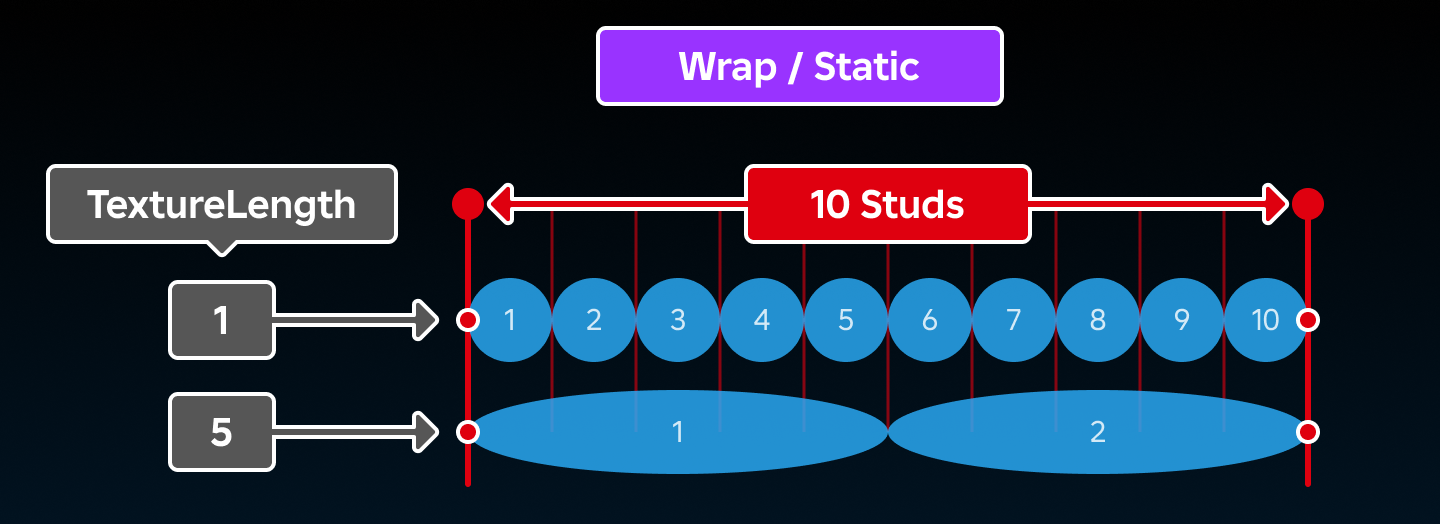
When set to Enum.TextureMode.Stretch, the texture will repeat TextureLength times across the beam's overall length.
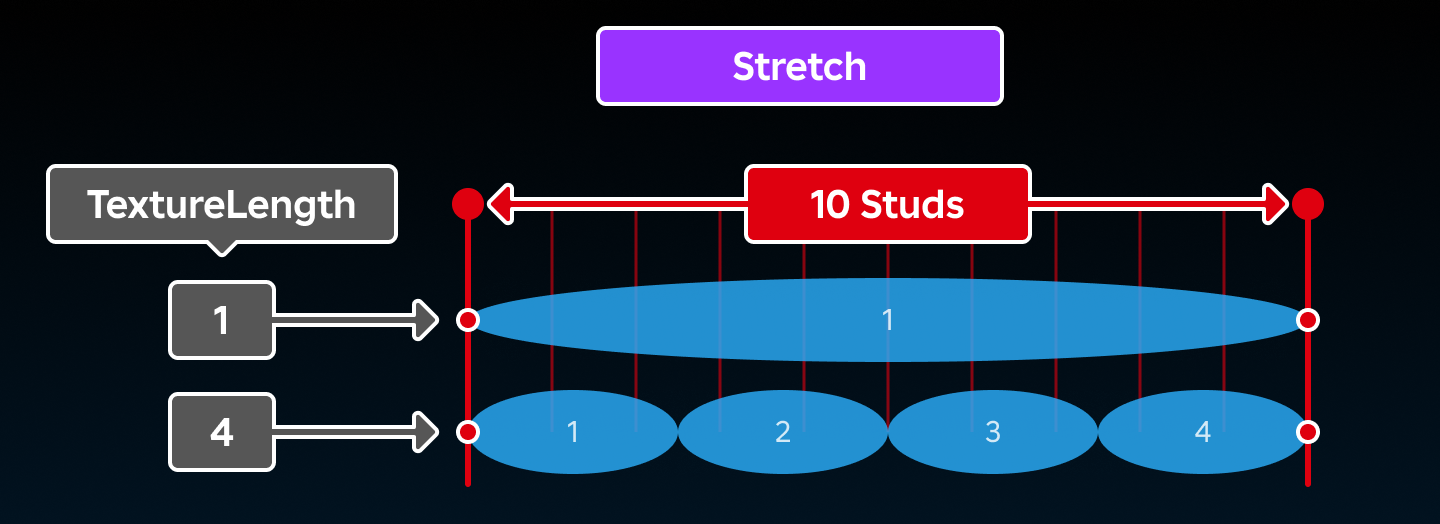
TextureSpeed
Determines the speed at which the Texture image moves along the beam. When this property is a positive value, the beam's texture will move from Attachment0 to Attachment1. This direction can be inverted by setting this property to a negative number.
Transparency
Determines the transparency of the beam across its segments. This property is a NumberSequence, allowing the transparency to be configured to vary across the length of the beam.
Consider the following NumberSequence which, when applied to a beam, would yield the pictured result.
local numberSequence = NumberSequence.new({NumberSequenceKeypoint.new(0, 0), -- OpaqueNumberSequenceKeypoint.new(0.5, 1), -- TransparentNumberSequenceKeypoint.new(1, 0), -- Opaque})
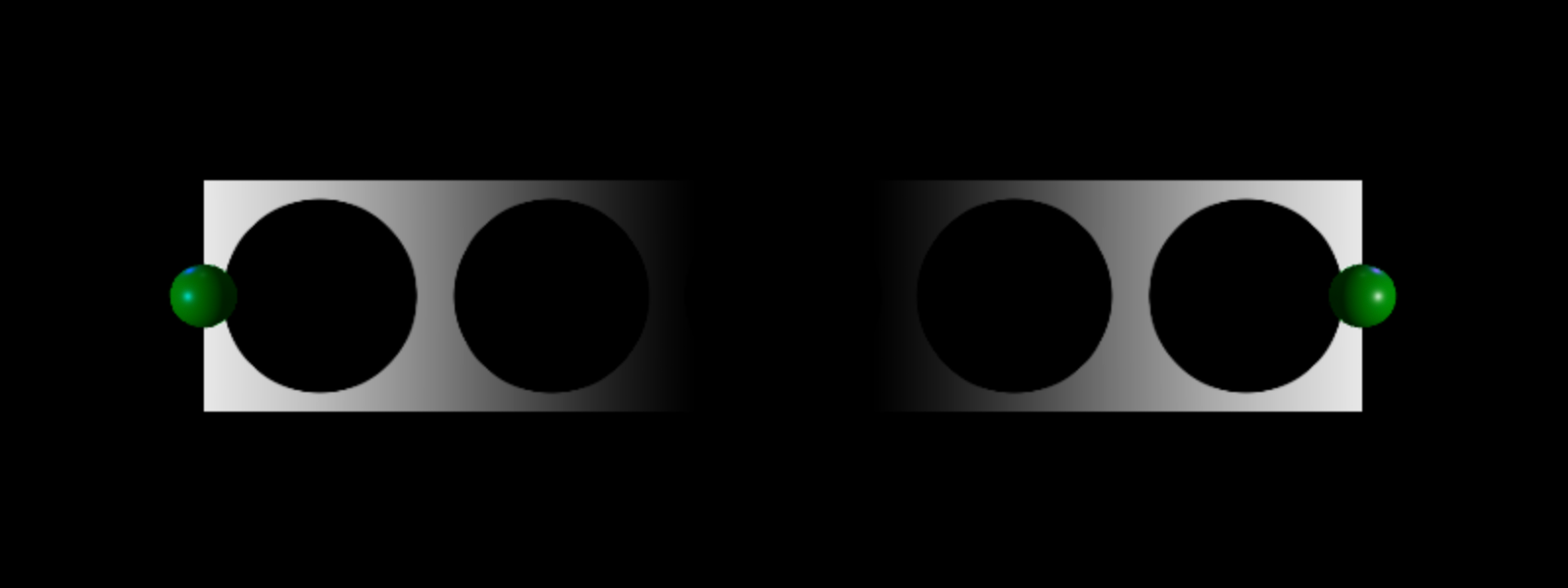
Note that the beam's transparency also depends on the number of Segments. Each segment of the beam can only show a transition between two transparencies. Therefore a beam will need to have at least n-1 segments in order to display correctly, where n is the number of NumberSequenceKeypoints in the NumberSequence.
Width0
The width of the beam at its origin (Attachment0), in studs. The beam's width will change linearly to Width1 studs at its end (Attachment1).
Width1
The width of the beam at its end (Attachment1), in studs. The beam's width will change linearly from Width0 studs at its origin (Attachment0).
ZOffset
The distance, in studs, the beam display is offset relative to the CurrentCamera. When 0, the beam will be displayed in its standard position between Attachment0 and Attachment1. ZOffset can be either positive or negative.
This property is particularly useful to avoid "Z‑fighting" when using multiple Beams between the same Attachments.
Code Samples
This code sample uses the Beam.ZOffset property to layer multiple beams between the same attachments.
-- Create beams
local beam1 = Instance.new("Beam")
beam1.Color = ColorSequence.new(Color3.new(1, 0, 0))
beam1.FaceCamera = true
beam1.Width0 = 3
beam1.Width1 = 3
local beam2 = Instance.new("Beam")
beam2.Color = ColorSequence.new(Color3.new(0, 1, 0))
beam2.FaceCamera = true
beam2.Width0 = 2
beam2.Width1 = 2
local beam3 = Instance.new("Beam")
beam3.Color = ColorSequence.new(Color3.new(0, 0, 1))
beam3.FaceCamera = true
beam3.Width0 = 1
beam3.Width1 = 1
-- Layer beams
beam1.ZOffset = 0
beam2.ZOffset = 0.01
beam3.ZOffset = 0.02
-- Create attachments
local attachment0 = Instance.new("Attachment")
attachment0.Position = Vector3.new(0, -10, 0)
attachment0.Parent = workspace.Terrain
local attachment1 = Instance.new("Attachment")
attachment1.Position = Vector3.new(0, 10, 0)
attachment1.Parent = workspace.Terrain
-- Connect beams
beam1.Attachment0 = attachment0
beam1.Attachment1 = attachment1
beam2.Attachment0 = attachment0
beam2.Attachment1 = attachment1
beam3.Attachment0 = attachment0
beam3.Attachment1 = attachment1
-- Parent beams
beam1.Parent = workspace
beam2.Parent = workspace
beam3.Parent = workspace
Methods
SetTextureOffset
The offset of a beam's texture cycle represents the progress of its texture animation. This method sets the current offset of the beam's texture cycle; hence, it can be used to reset the cycle by passing 0 as the offset parameter.
Notes
- The given offset parameter is expected to be a value between 0 and 1, but greater values can be used.
- The texture cycle wraps at 0 and 1, meaning the texture is in the same position when the offset is at 0 or 1.
- If the Texture property is not set, this method does nothing.
- Increasing the offset will act in the inverse direction to the TextureSpeed property, meaning it will move the texture in the opposite direction to the direction the texture animates when TextureSpeed is greater than 0.
Parameters
The desired offset of the texture cycle.