The ScreenGui container holds GuiObjects to display on a player's screen, including frames, labels, buttons, and more. All on‑screen UI objects and code are stored and changed on the client.
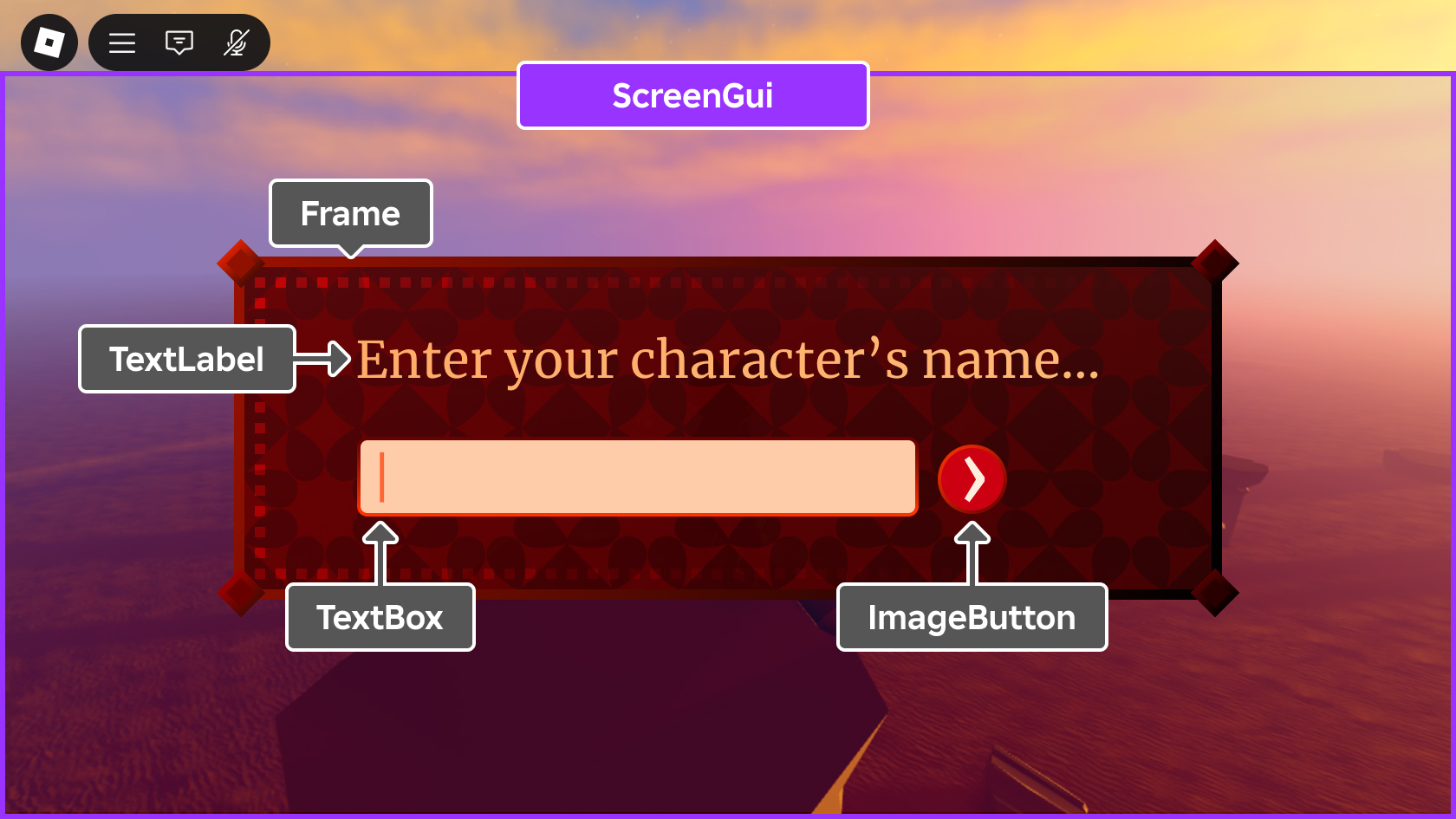
Creating and Parenting
To display a ScreenGui and its child GuiObjects to every player who joins the experience, place it inside the StarterGui container. When a player joins an experience and their character first spawns, the ScreenGui and its contents clone into the PlayerGui container for that player, located within the Players container.
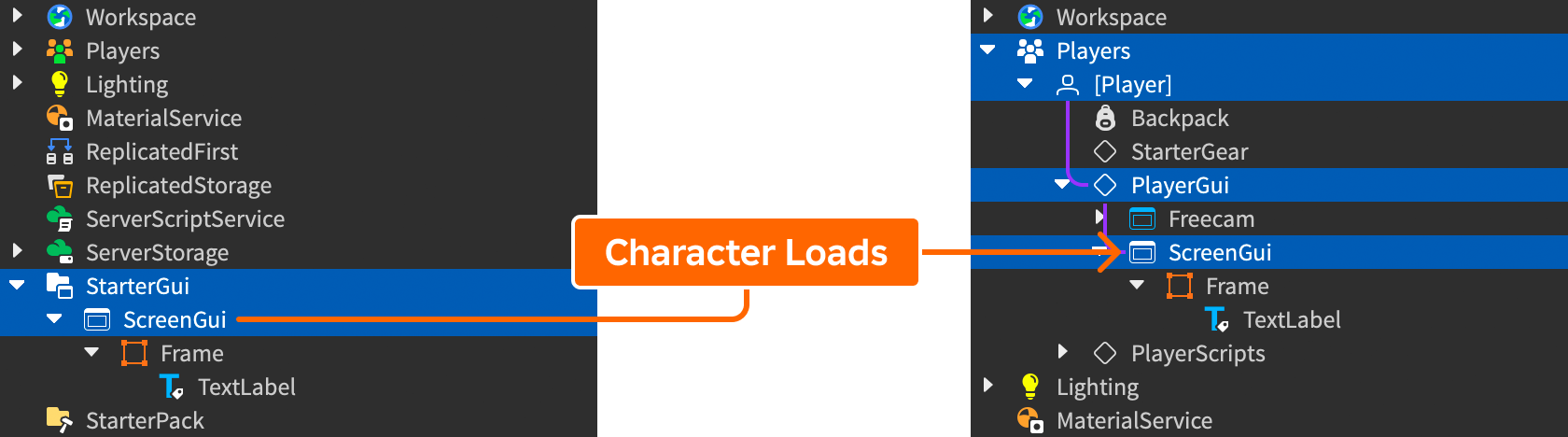
Managing Screen Containers
As an experience grows in scope, you may require multiple screen interfaces such as a title screen, settings menu, shop interface, and more. In such cases, you can place multiple unique ScreenGui containers inside StarterGui and toggle each container's Enabled property depending on whether it should be visible and active (while false, contents will not render, process user input, or update in response to changes).
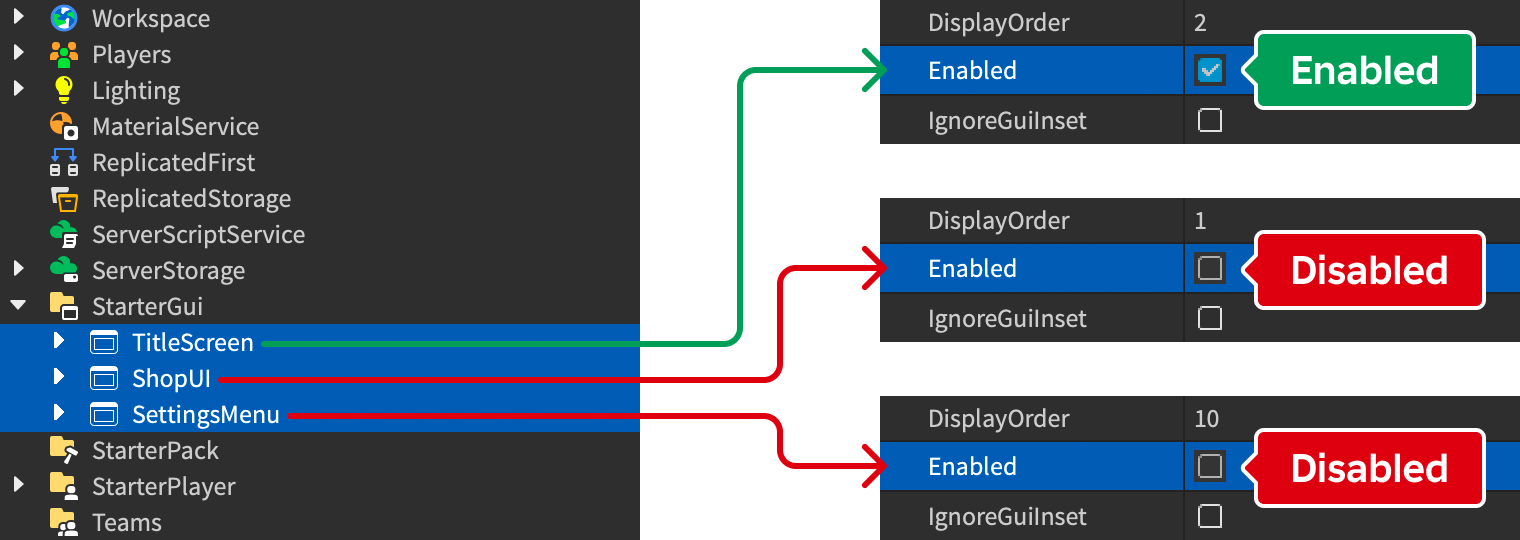
The Enabled property can be initially toggled through the Properties window and/or you can set it during playtime from a client‑side script by accessing the player's PlayerGui and setting it to true or false for the desired container(s).
Container Properties
The following properties let you customize the screen insets across multiple devices, the display order when using multiple screen containers, and more.
Screen Insets
Modern phones take advantage of the entire screen but typically include notches, cutouts, and other elements that occupy screen space. Every Roblox experience also includes the top bar controls for quick access to the main menu, chat, leaderboard, and more.
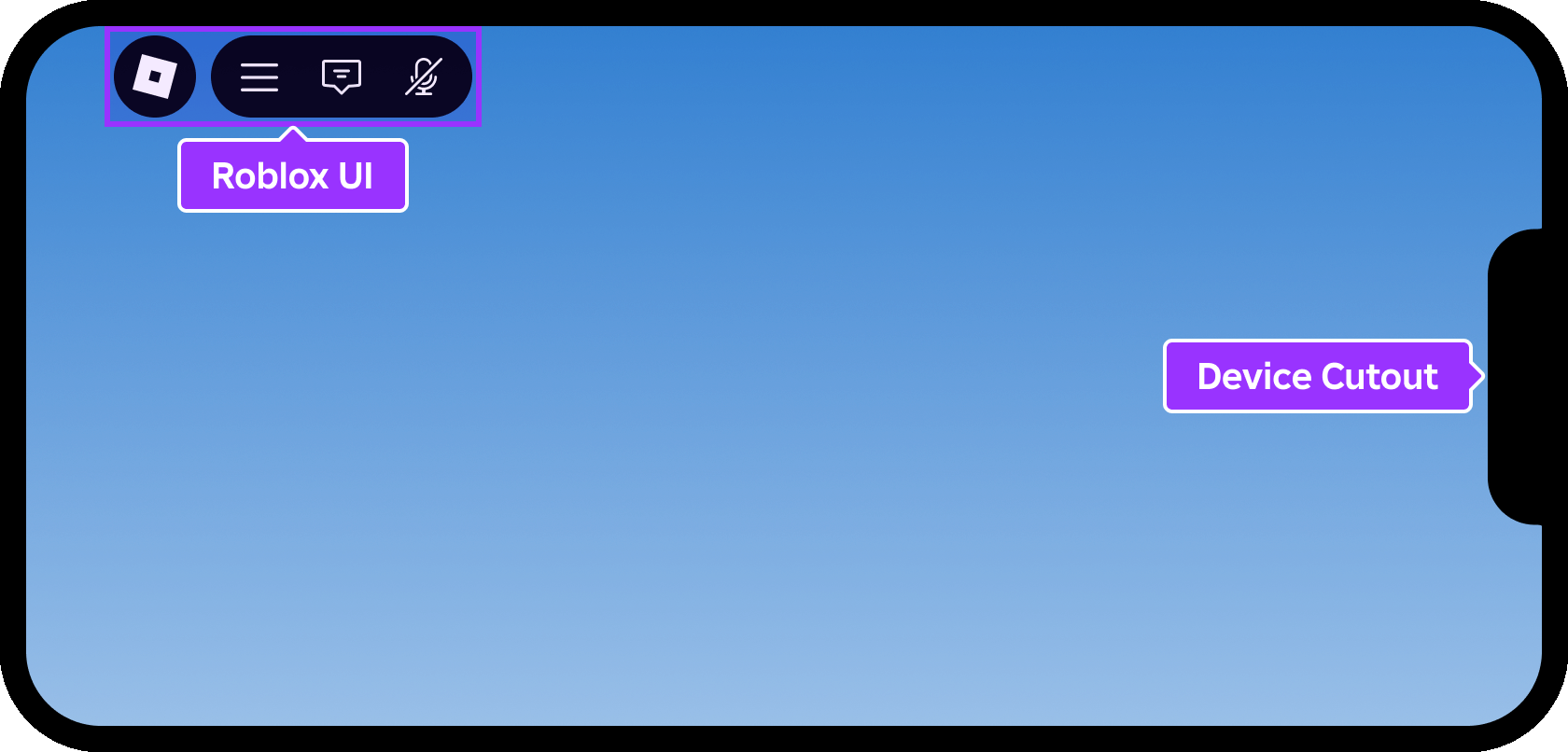
To ensure players can see and access all UI easily and without obstruction, Roblox provides the ScreenInsets property which controls the safe area insets for the contents of a ScreenGui.
The default of CoreUISafeInsets keeps all descendant GuiObjects inside the core UI safe area, clear of the top bar buttons and other screen cutouts. This setting is recommended if the ScreenGui contains interactive UI elements.
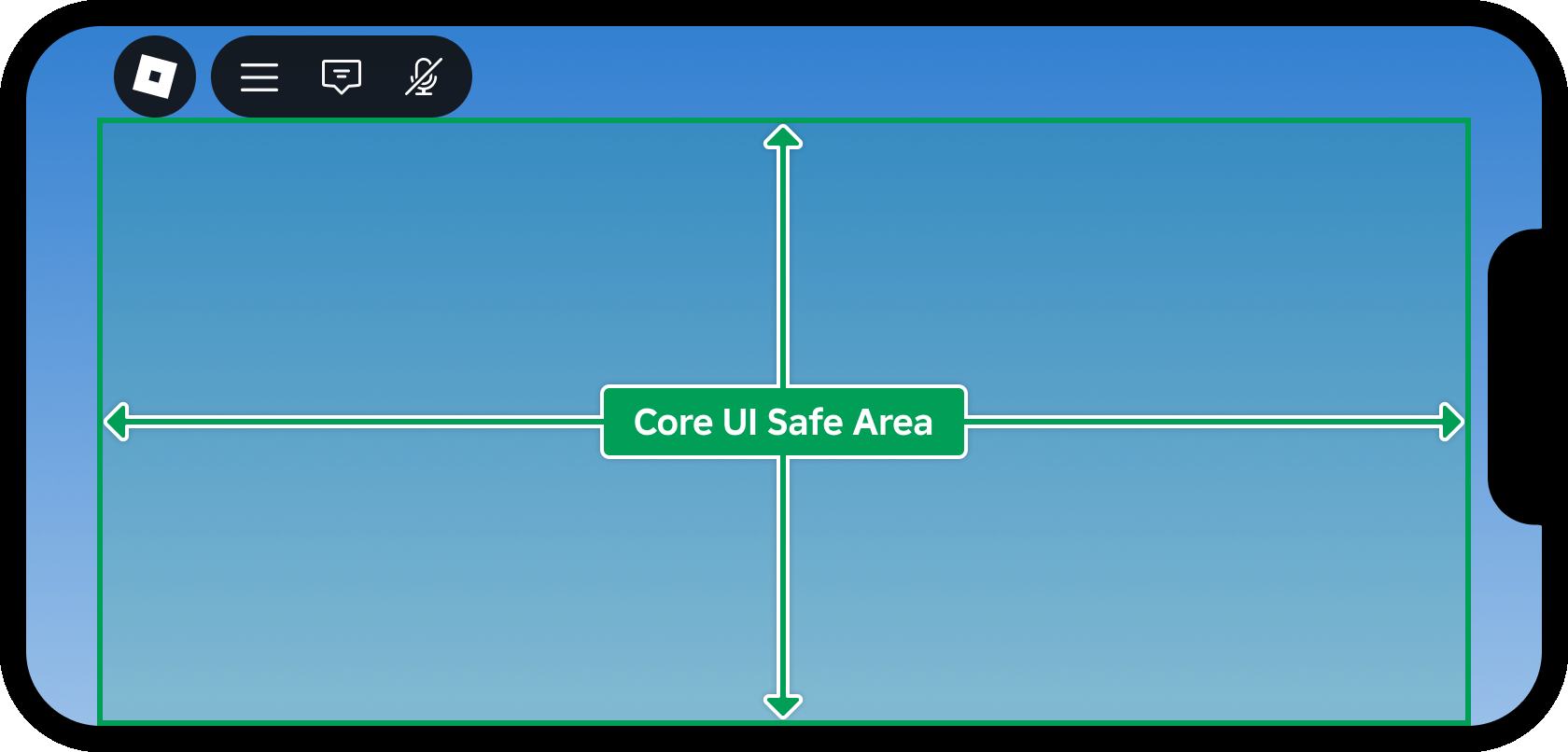
Display Order
When using multiple ScreenGui interfaces, you can layer them by Z‑index through their DisplayOrder property. For example, to display a modal settings menu on one ScreenGui in front of the experience's main user interface on another ScreenGui, assign a higher DisplayOrder to the modal's than the underlying interface's.
Reset on Spawn
The ResetOnSpawn boolean property determines if the ScreenGui resets (deletes itself and re‑clones into the player's PlayerGui) every time the player's character respawns.
Condition | Resets |
---|---|
ResetOnSpawn is true (default). | yes |
The ScreenGui is an indirect descendant of StarterGui; for example it's placed inside a Folder located within StarterGui. | yes |
ResetOnSpawn is false and the ScreenGui is a direct descendant of StarterGui. | no |
Accessing Player UI
As noted, parenting a ScreenGui to StarterGui clones it and its child GuiObjects into a player's PlayerGui container when they join the experience and their character first spawns.
If you need to control a player's UI container during playtime, for example to show/hide a specific ScreenGui or any of its children, access it as follows from a LocalScript:
LocalScript - Accessing a Player's UI
local Players = game:GetService("Players")local player = Players.LocalPlayerlocal playerGui = player.PlayerGuilocal titleScreen = playerGui:WaitForChild("TitleScreen")local settingsMenu = playerGui:WaitForChild("SettingsMenu")titleScreen.Enabled = false -- Hide title screensettingsMenu.Enabled = true -- Show settings menu
Disabling Default UI
All Roblox experiences include several UI elements that are enabled by default. If you don't need any of these elements or if you want to replace them with your own creations, you can use the SetCoreGuiEnabled() method in a client‑side script with the associated Enum.CoreGuiType option.
Default UI | Associated Enum |
---|---|
Dynamically updated Players list, commonly used as a leaderboard. | Enum.CoreGuiType.PlayerList |
The character's Health bar. Does not appear if the character's Humanoid is at full health. | Enum.CoreGuiType.Health |
The character's Backpack which contains in‑experience tools. Does not appear if there are no Tools in the backpack. | Enum.CoreGuiType.Backpack |
The text chat window. | Enum.CoreGuiType.Chat |
Popup menu of character emotes. | Enum.CoreGuiType.EmotesMenu |
A capture screenshot button along the right side of the screen. Does not appear unless the player has enabled Captures from the Roblox menu. | Enum.CoreGuiType.Captures |
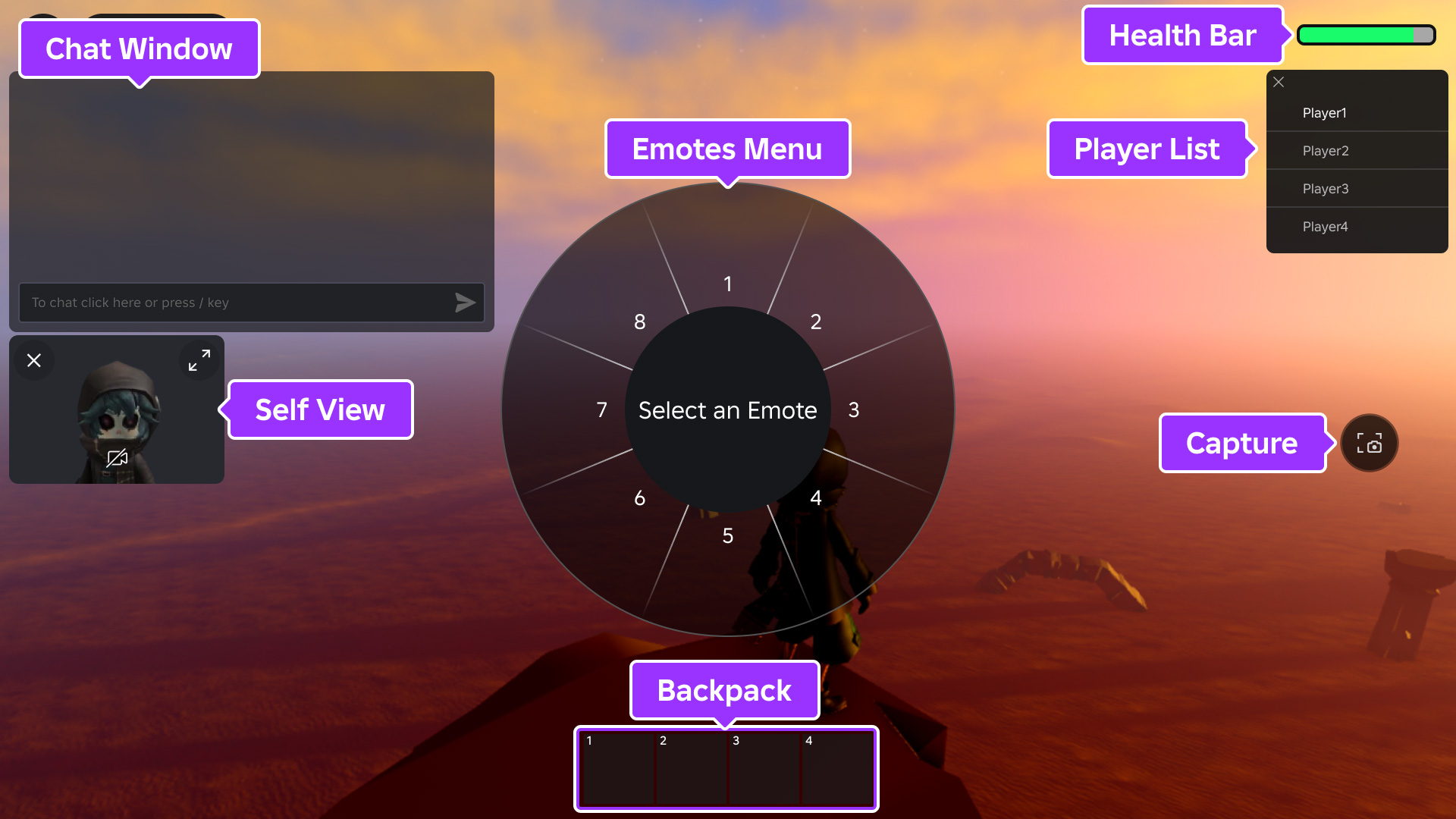
Client Script - Disable Default UI Elements
local StarterGui = game:GetService("StarterGui")-- Disable default health bar and backpackStarterGui:SetCoreGuiEnabled(Enum.CoreGuiType.Health, false)StarterGui:SetCoreGuiEnabled(Enum.CoreGuiType.Backpack, false)
Additionally, devices with touch capabilities include a virtual thumbstick and a jump button by default. If desired, you can hide these elements by setting GuiService.TouchControlsEnabled to false in a client‑side script.
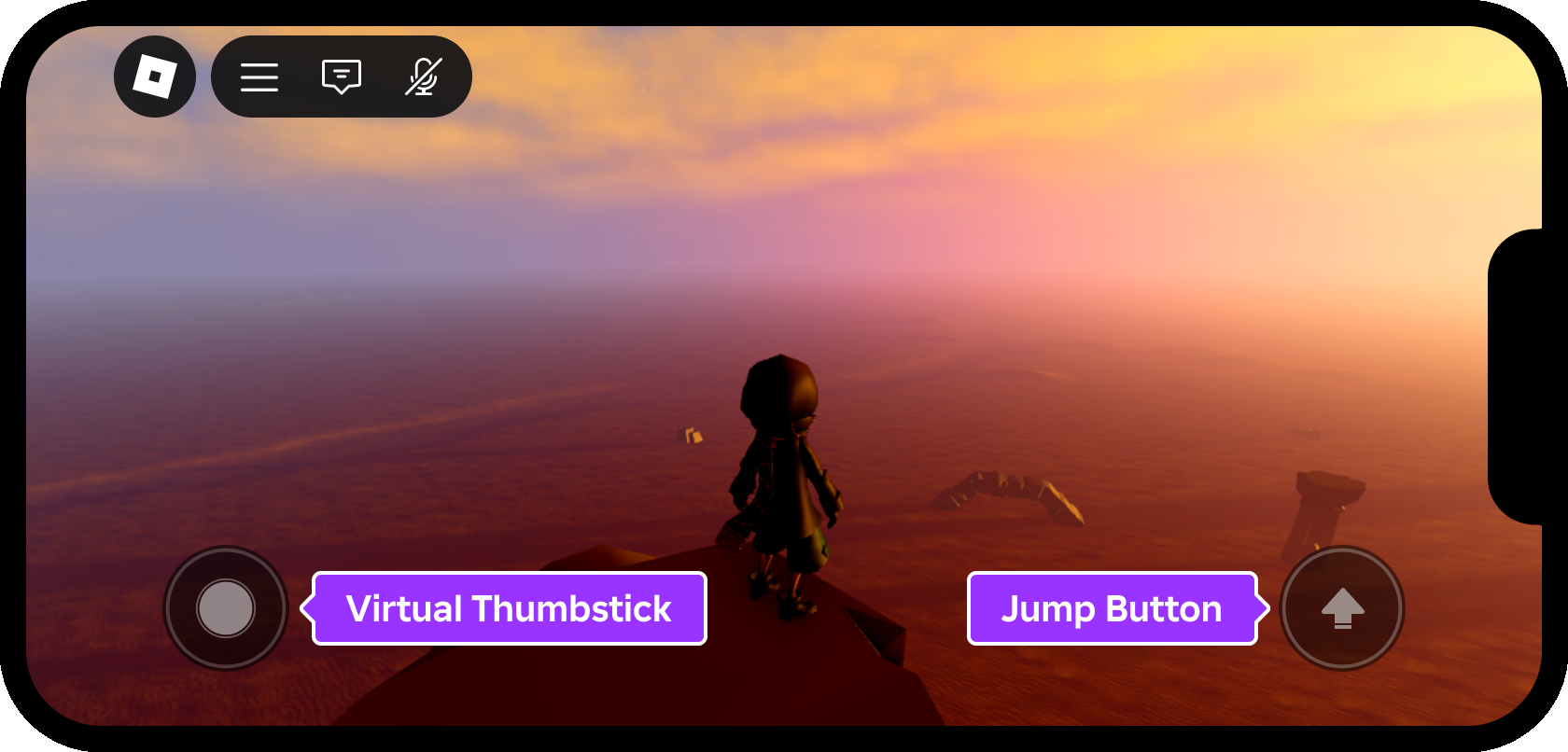
Client Script - Disable Touch Controls
local GuiService = game:GetService("GuiService")GuiService.TouchControlsEnabled = false