Class.Path2D インスタンス、API メソッド、プロパティを含む、2D スプラインと 2D カーブドライブを実装することができます。これは、パスベースのアニメーションやグラフィックエディタなどの UI エフェクトに便利です。
2D パスを作成する
画面に追加する Path2D またはエクスペリエンスオブジェクトに追加する:
新しい Path2D を選択してビューポートツールウィジェットを表示します。デフォルトでは、 追加ポイント ツールが選択されます。
画面をクリックして [コントロールポイント] シリーズを追加して、パスを形成します。最初のパスは不正確ですが、後でコントロールポイントの位置を調整できます。
終了したら、ウィジェットの 完了 ボタンをクリックするか、Enter を押します。
コントロールポイントを変更する
Class.Path2D を選択した、Explorer 階層の下で、個々のコントロールポイントとその tangents を変更できます。
移動ポイント
パス上の個々のコントロールポイントを移動するには、 Select ツール (V) を有効にし、それからクリックして新しい位置にドラッグします。

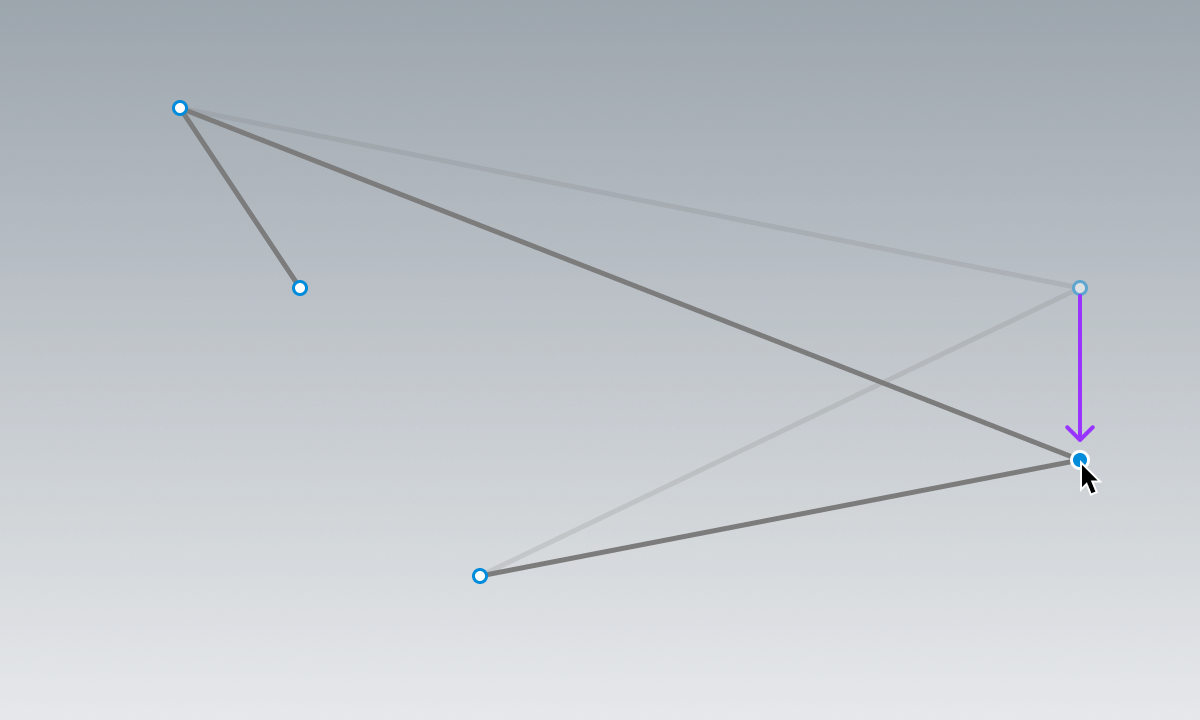
非常に特定の位置については、コントロールポイントを選択し、プロパティ ウィンドウで、ポイントの SelectedControlPointData プロパティの新しい位置を設定します (UDim2)。
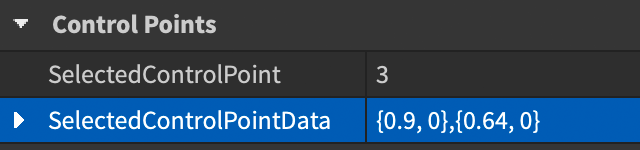
注:ポイントの位置は絶対ではなく、 rather 相対 をパスの親コンテナに置きます。たとえば、 ScreenGui 内のコントロールポイントを 20% から左に比較して、 Frame を上隅右隅に配置
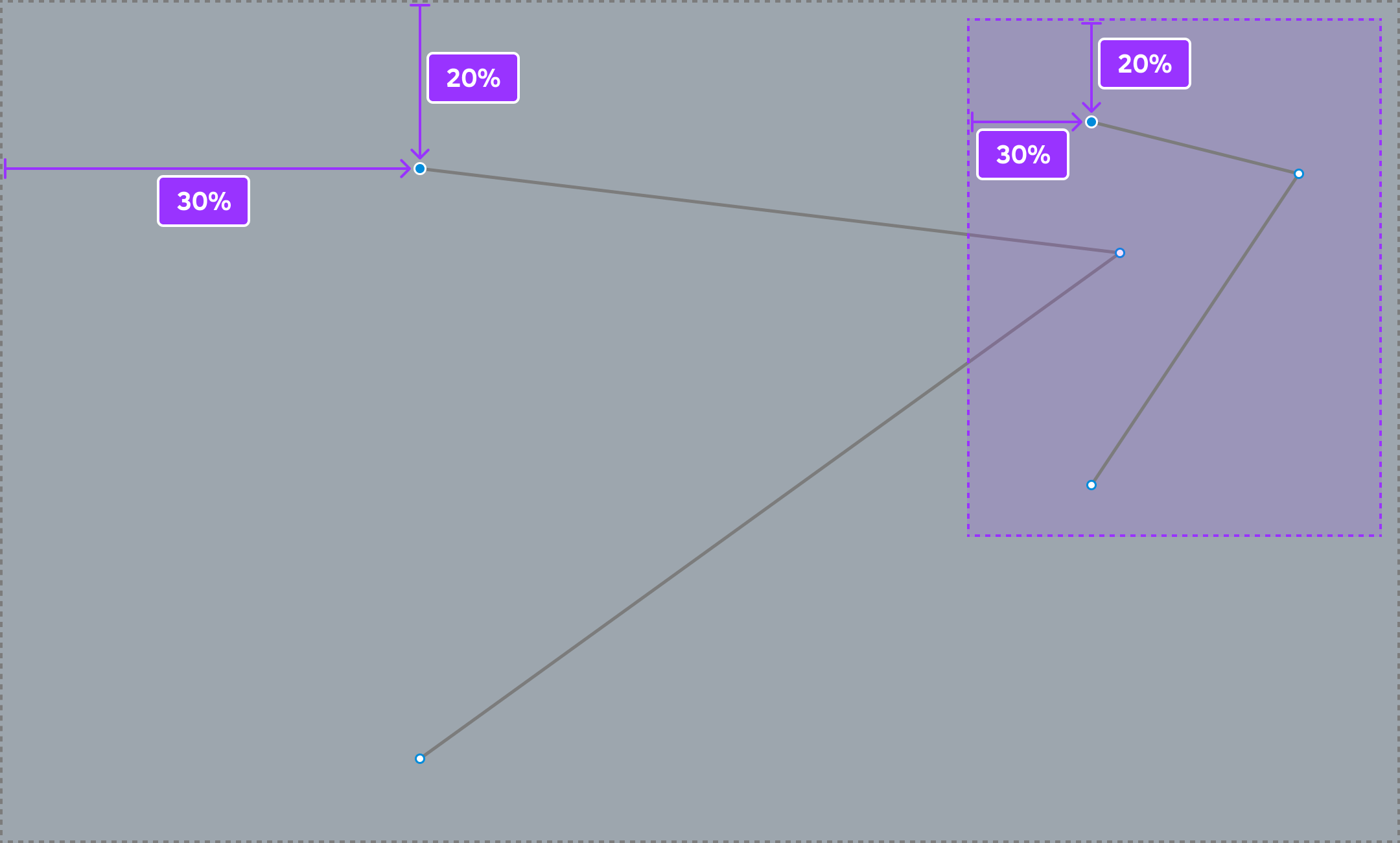
ポイントを追加する
新しいコントロールポイントは、2つの既存のポイント間または Path2D ツールを使用して、P の端点から追加できます。

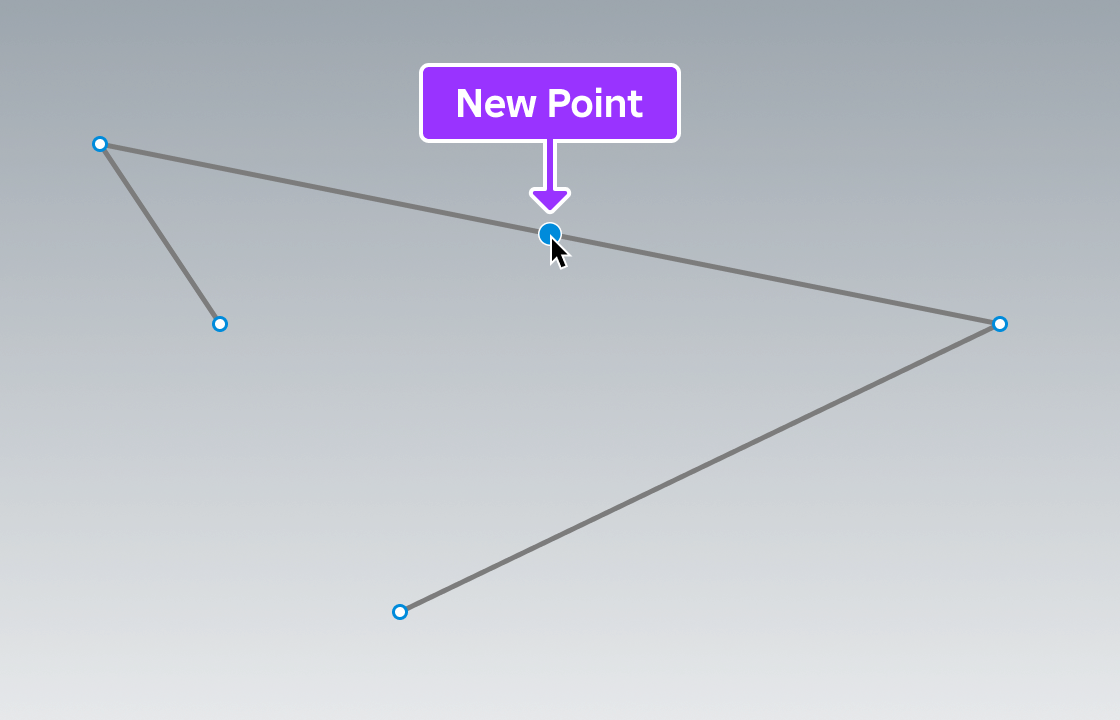
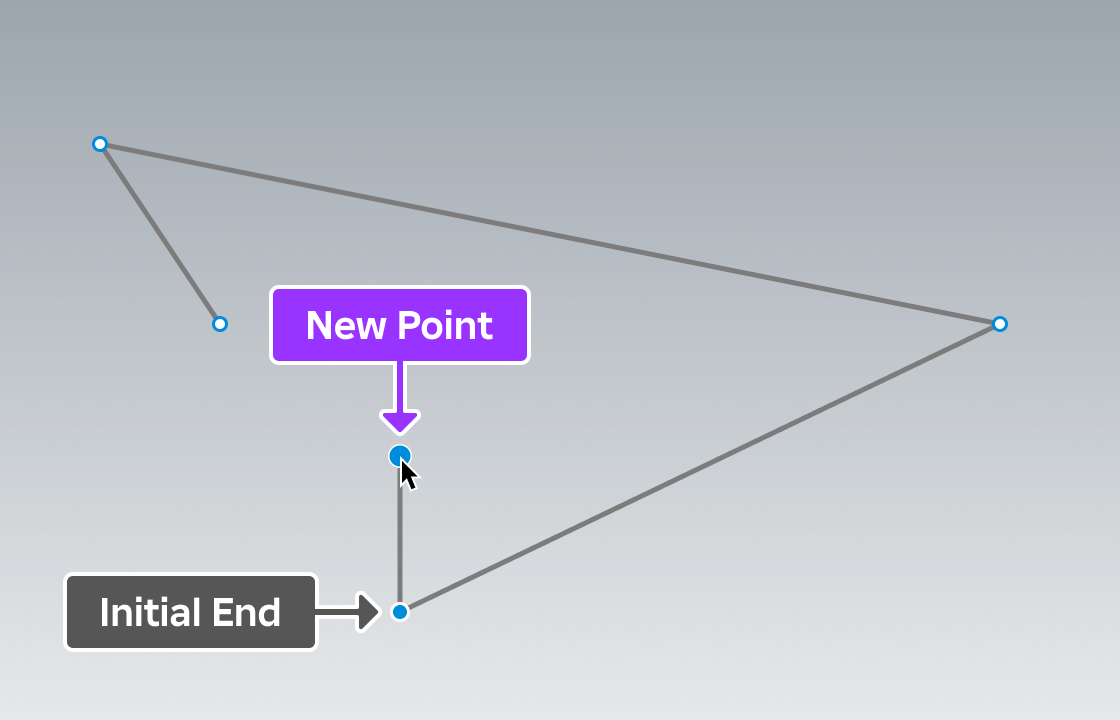
ポイントを削除する
コントロールポイントを削除するには、マウスオーバーして右クリックし、コンテキストポップアップメニューから ポイントを削除 を選択します。
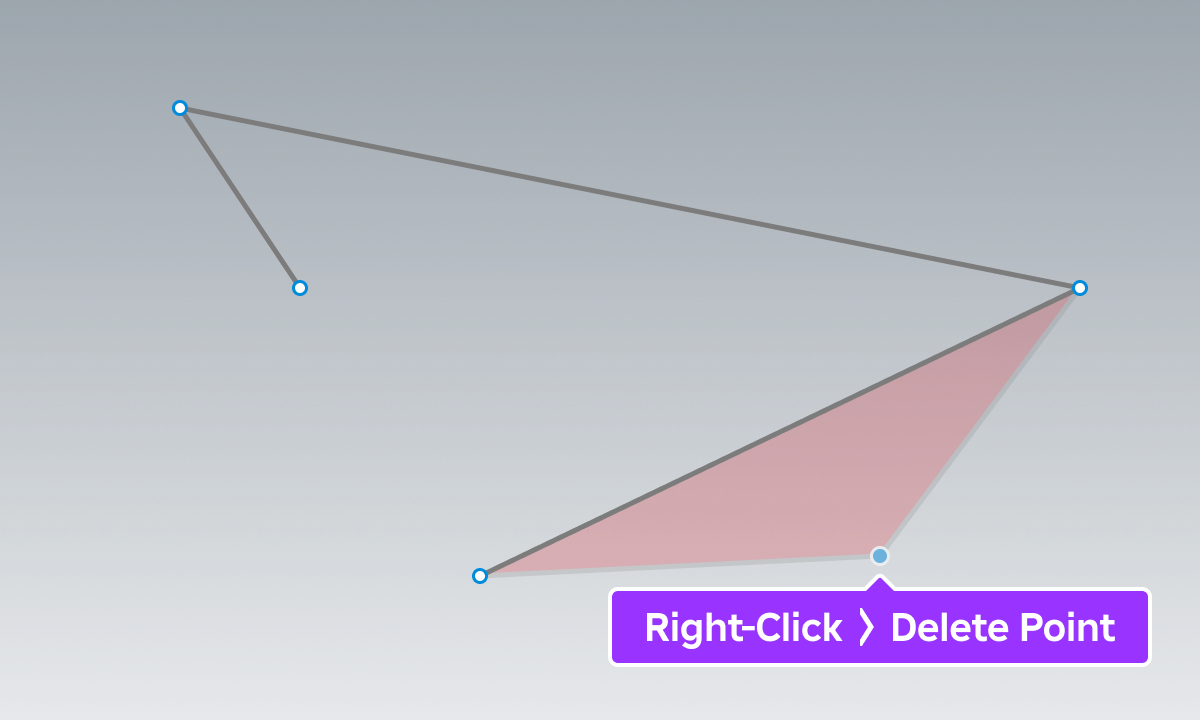
コントロールポイントのタンジェント
コントロールポイント タンジェント を使用すると、パス上の曲線を作成および調整できます。
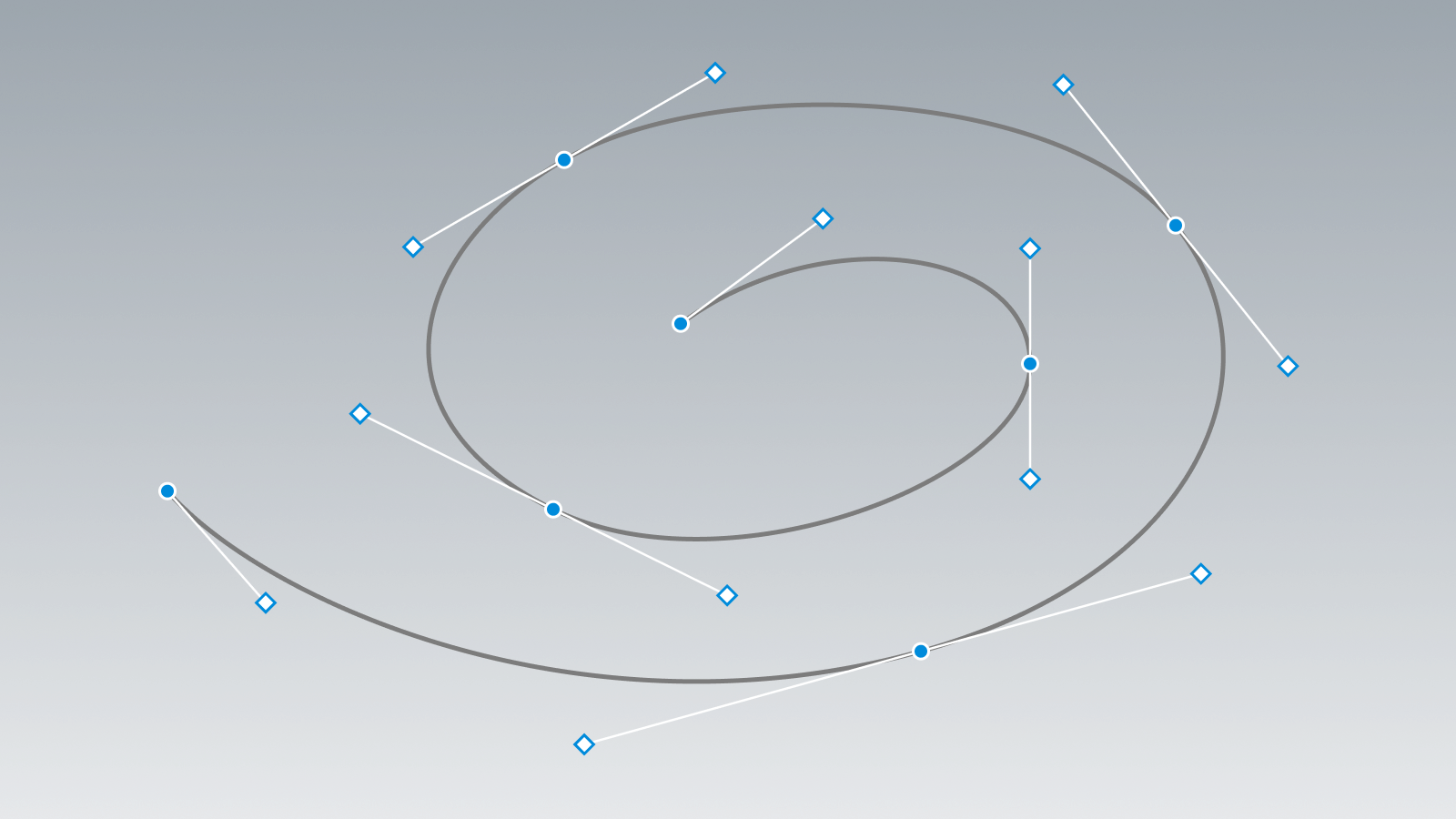
円錐を追加する
すでにタンジェントがありません:
ツーリングウィジェットで タンジェントを追加 ツールを有効にします。
必要なコントロールポイントにカーソルを置き、クリックして 2つの ミラー のタンジェントを追加します (クリック後にドラッグして新しいタンジェントを調整)。
切角を調整中
個々のコントロールポイントの既存の接線を調整するには:
Select ツール ( V ) を有効化します。
コントロールポイントではなく、タンジェントマーカーにカーソルを置き、クリックして新しい位置にドラッグします。タンジェントがミラーされている場合は、ペアのタンジェントポイントが同じ位置に移動します。
タンジェントの特定の UDim2 位置を手動で設定するには:
Select ツール ( V ) を有効にし、コントロールポイントを選択します。In the プロパティ window, expand the 1> Class.Path2D.SelectedControlPointData|SelectedControlPointData1> field to expose the 4> Datatype.Path2
位置を設定してください LeftTangent と/or RightTangent。これは、ミラードビーの動作を破壊する のタンジェントのミラービーの動作を破壊する。
タンジェントを削除する
コントロールポイントの両方のタンジェントを削除するには、そのポイントの上にマウスポイントを置き、コンテキストポップアップメニューから タンジェントのクリア を選択します。
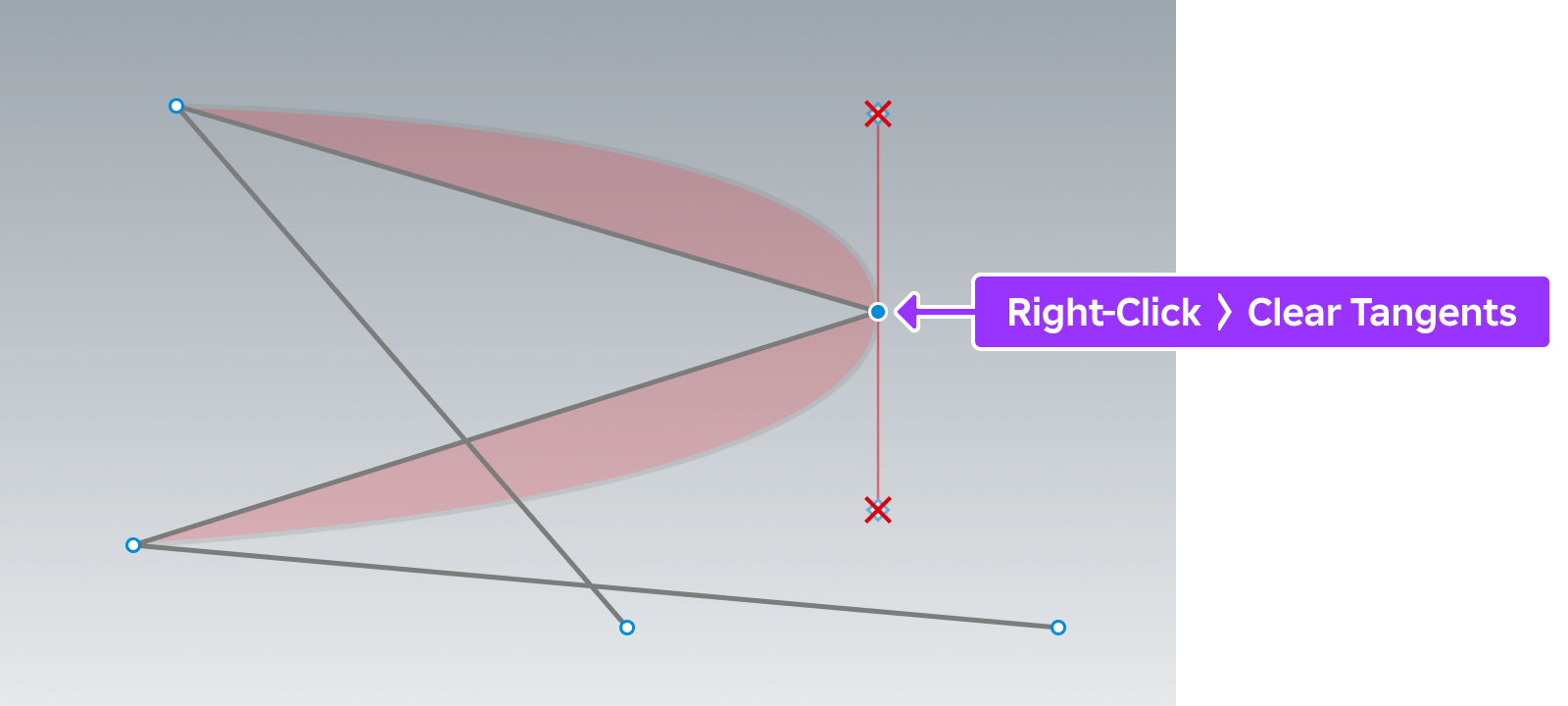
タンジェントの 1 つの削除には、左または右のタンジェントマーカーをホバーし、 タンジェントを削除 を選択します。
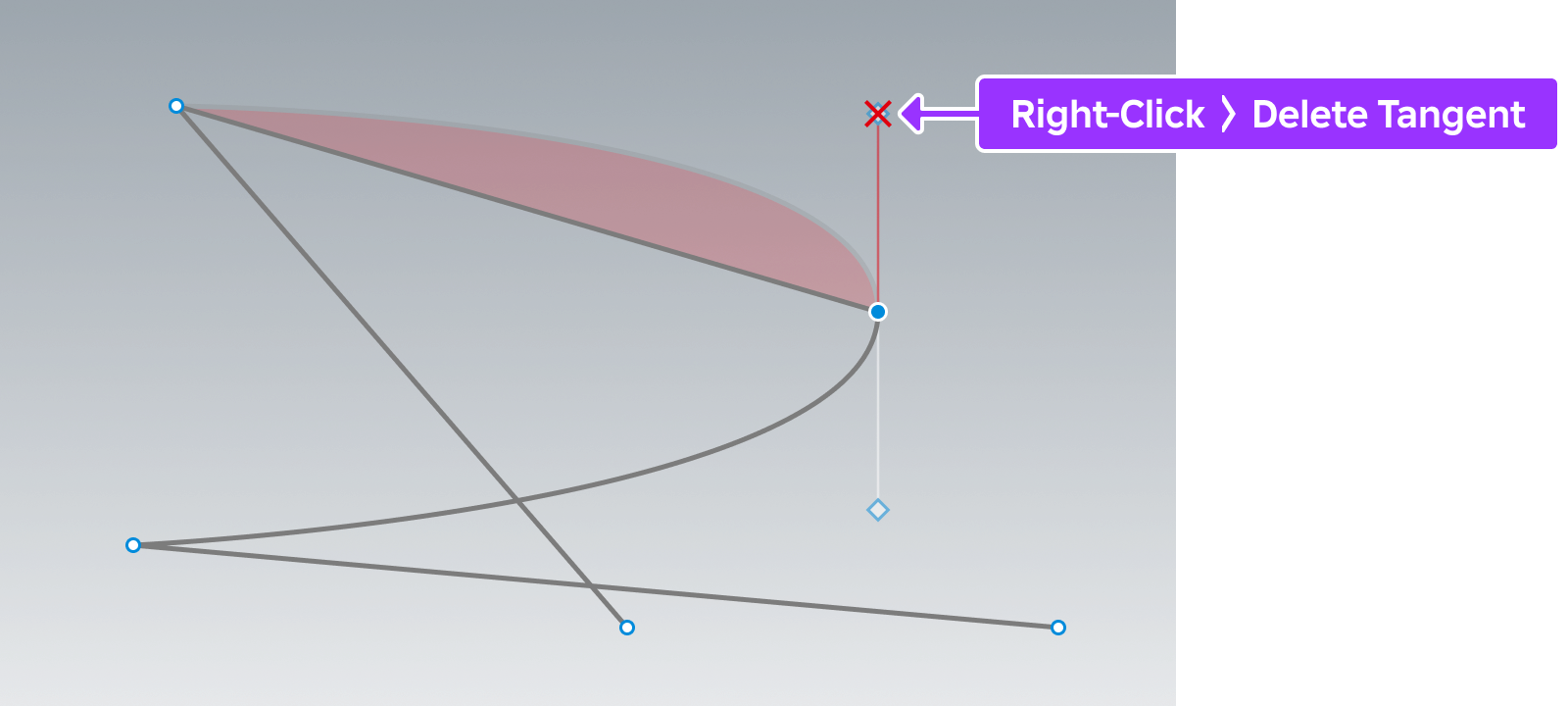
破壊とミラーリング
デフォルトでは、タンジェントは互いにミラーです。ドラッグして調整 1つのタンジェントマーカーをドラッグすると、ペアのタンジェントポイントが互いに移動します。
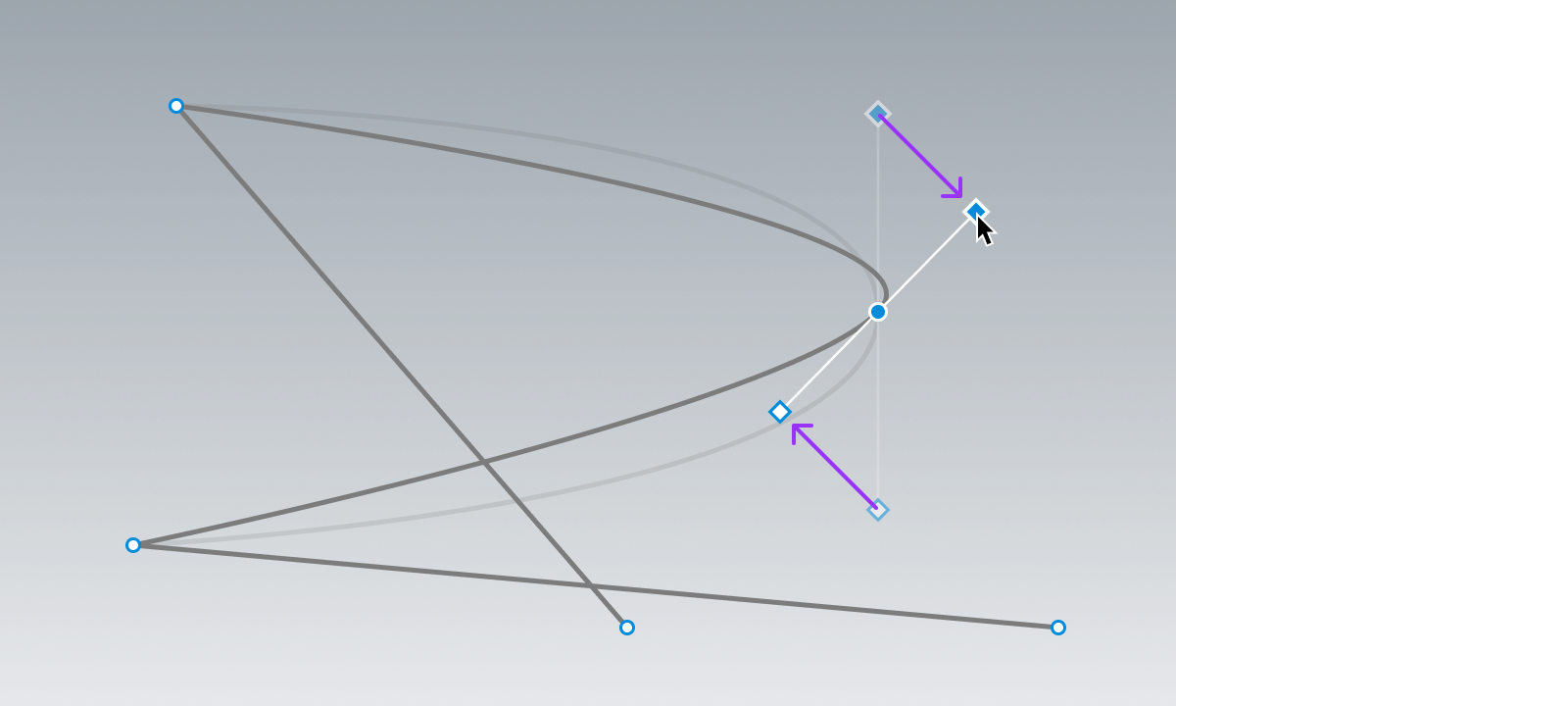
各人が他の人と独立して動くことができるように、ジャンジェントを「壊す」ために、関連するコントロールポイントをホバーして右クリックし、コンテキストメニューから ジャンジェントを壊す を選択します。壊れたジャンジェントマーカーは、他の人に影響を与えることなく、各人のマーカーを独立して動かすことができます。
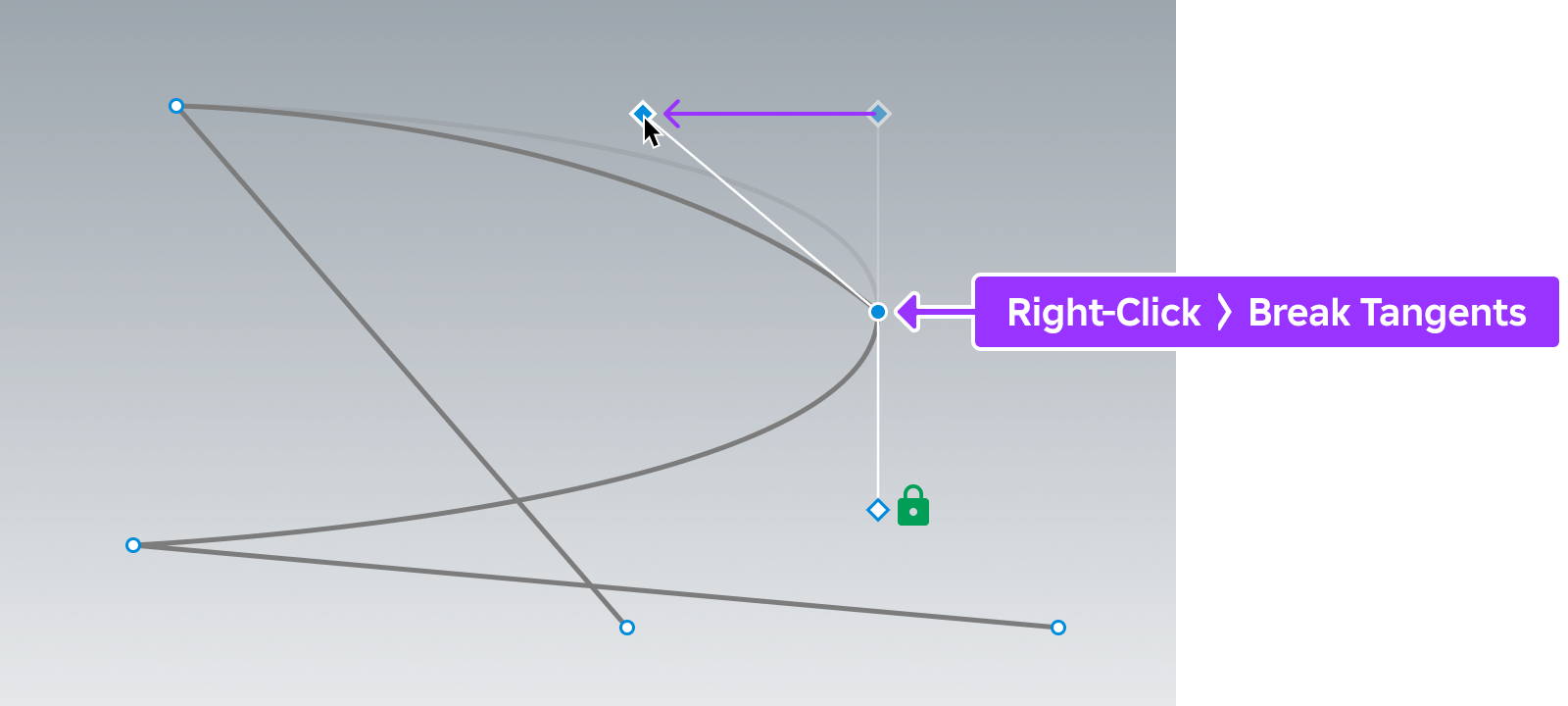
パスビジュアルプロパティ
次のプロパティで、Path2D のビジュアルアパートをカスタマイズできます:
プロパティ | 説明 |
---|---|
Color3 | パスラインの色を設定します。 |
Thickness | パスラインのサイズをピクセル単位で設定します。 |
Visible | パスを編集およびランタイムの両方で表示または表示しないようにします。 |
ZIndex | 他の GUI に対してパスがレンダリングされる順序を指定します。 |
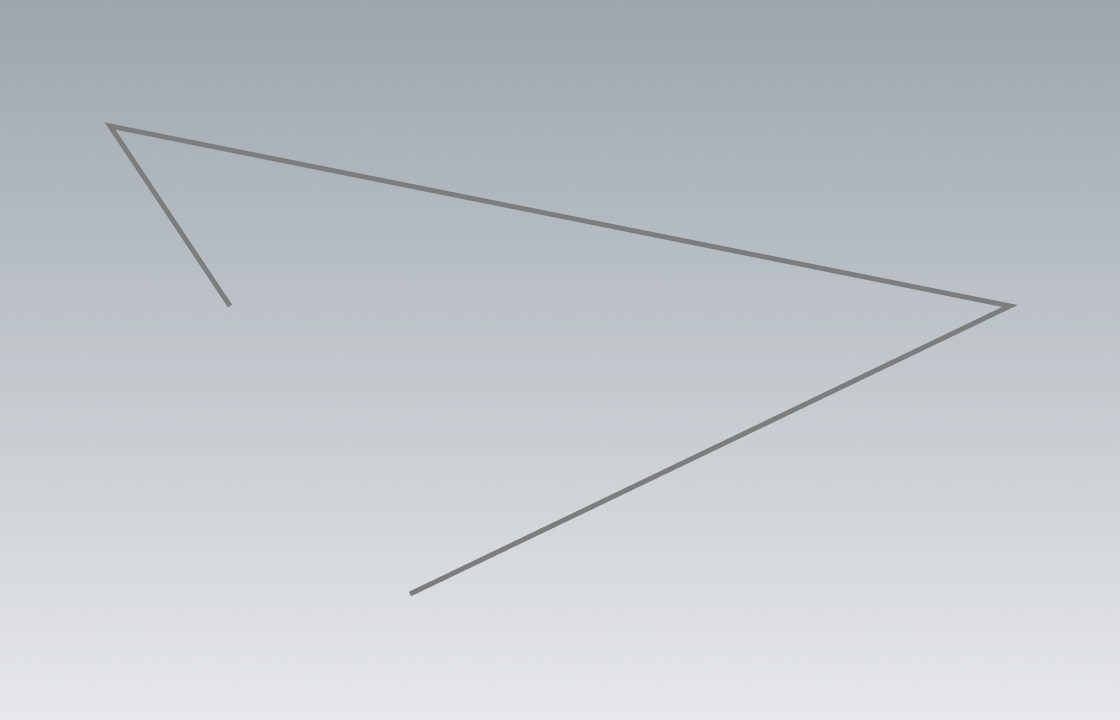
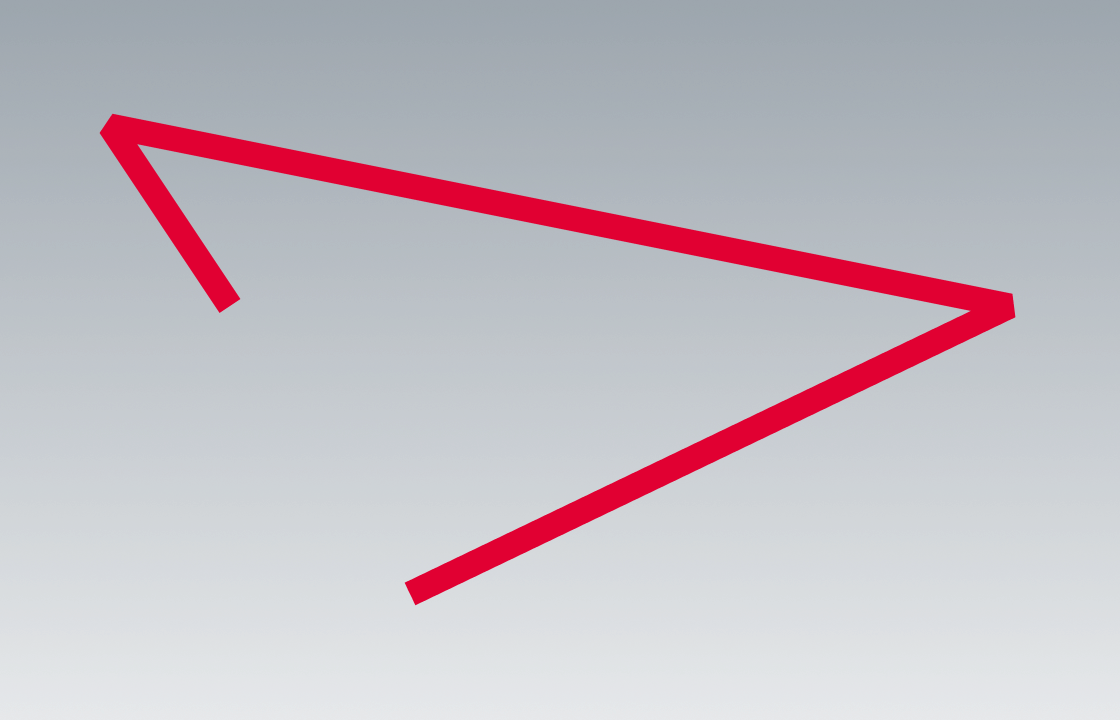
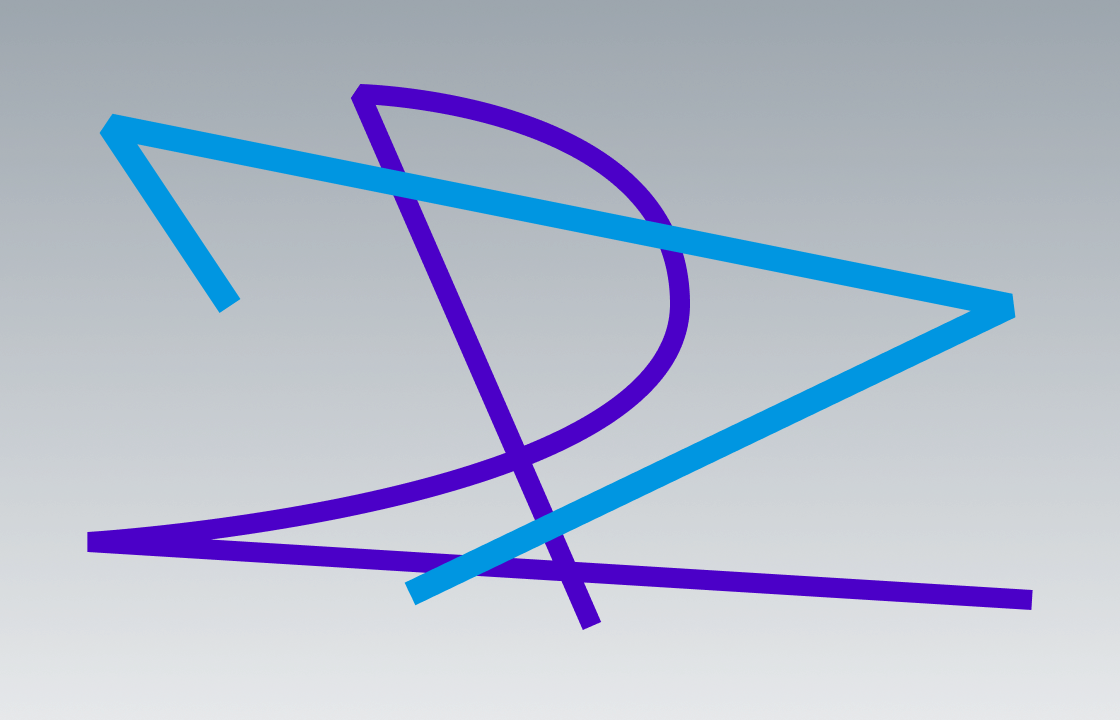
パススクリプト
スクリプトは、複数のパス関連ワークフローに便利です。次の例では、GetControlPoints() などのメソッドを使用して、 Datatype.Path2
パス間で UI オブジェクトを整列
local parent = script.Parent
local path = parent:FindFirstChildWhichIsA("Path2D")
local function arrangeChildren()
local segmentCount = #path:GetControlPoints()
local objectsToArrange = {}
for _, child in parent:GetChildren() do
if child:IsA("GuiObject") then
table.insert(objectsToArrange, child)
end
end
for idx, child in objectsToArrange do
local t = idx / (#objectsToArrange + 1)
child.Position = path:GetPositionOnCurveArcLength(t)
end
end
-- 最初に子 UI オブジェクトをパスで整理
arrangeChildren()
-- 配列を調整するために子供を追加/削除します
parent.ChildAdded:Connect(arrangeChildren)
parent.ChildRemoved:Connect(arrangeChildren)
パス中の UI オブジェクトをアニメート
local Tweenservice = game:GetService("TweenService")
local parent = script.Parent
local path = parent:FindFirstChildWhichIsA("Path2D")
local objectToAnimate = parent:FindFirstChildWhichIsA("GuiObject")
local TWEEN_DURATION = 4
local TWEEN_EASING_STYLE = Enum.EasingStyle.Cubic
local TWEEN_EASING_DIRECTION = Enum.EasingDirection.InOut
local pathSampleValue = Instance.new("NumberValue")
local tweenInfo = TweenInfo.new(TWEEN_DURATION, TWEEN_EASING_STYLE, TWEEN_EASING_DIRECTION, 0, true, 2)
local tween = Tweenservice:Create(pathSampleValue, tweenInfo, {Value = 1})
local function onSampleValueChanged()
objectToAnimate.Position = path:GetPositionOnCurveArcLength(pathSampleValue.Value)
end
pathSampleValue.Changed:Connect(onSampleValueChanged)
tween:Play()