개발자 제품은 사용자가 한 번 이상 구매할 수 있는 아이템이나 능력, 예를 들어 경험 내 통화, 탄약 또는 물약과 같이.
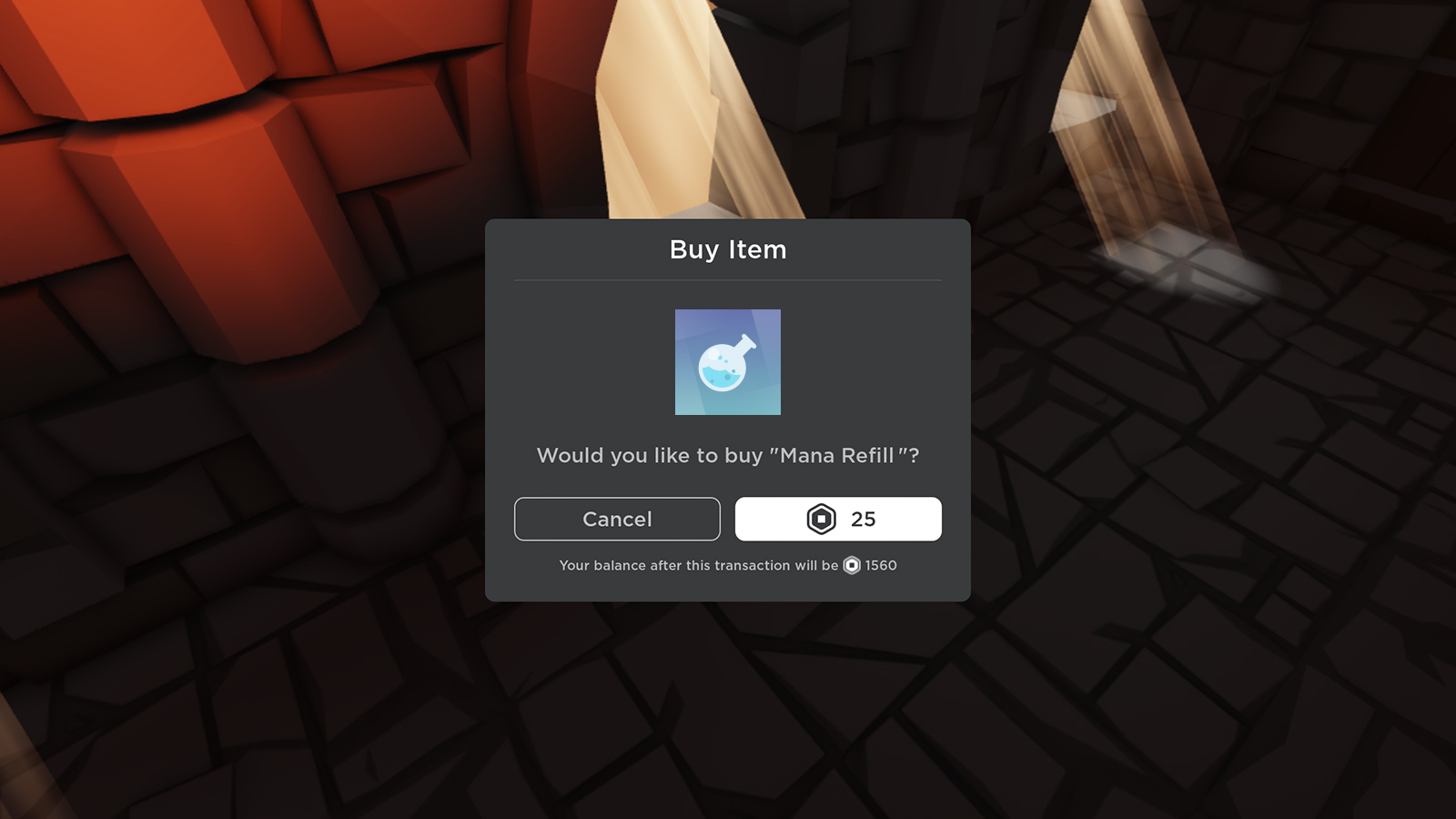
개발자 제품 생성
개발자 제품을 생성하려면:
- 크리처 탭으로 이동하고 경험을 선택하세요.
- 수익 창출 으로 이동합니다 개발자 제품 > 개발자 제품 으로.
- 클릭하십시오 개발자 제품 생성 .
- 제품 아이콘으로 업로드하려는 이미지를 업로드하십시오. 이미지가 512x512 픽셀을 초과하지 않도록 하고, 원형 경계 밖에 중요한 세부 정보가 포함되지 않도록 하며, .jpg, .png, 또는 .bmp 형식입니다.
- 제품에 대한 이름과 설명을 입력합니다.
- Robux로 제품 가격을 설정하십시오. 최소 가격은 1 Robux이고 최대 가격은 10억 Robux입니다.
- 클릭하십시오 개발자 제품 생성 .
개발자 제품 ID 取得
스크립팅사용하려면 개발자 제품 ID가 필요합니다. 제품 ID를 얻으려면:
수익 창출 으로 이동합니다 개발자 제품 > 개발자 제품 으로.
제품 위로 마우스를 이동하고 ⋯ 메뉴를 클릭하십시오.
클립보드에 ID를 복사하려면 자산 ID 복사 를 클릭하십시오.
개발자 상품 판매
경험 내에서 개발자 제품을 구현하고 판매하려면 MarketplaceService 함수를 호출하십시오.
Class.MarketplaceService:GetProductInfo()|GetProductInfo() 를 사용하여 개발자 제품, 이름 및 가격과 같은 정보를 검색한 다음 사용자에게 제품을 표시하십시오. 제품을 경험 내에서 판매할 수 있습니다. 개발자 제품의 경우 두 번째 매개 변수는 Enum.InfoType.Product
local MarketplaceService = game:GetService("MarketplaceService")
-- 대체 ID를 개발자 제품 ID로 교체
local productId = 000000
local success, productInfo = pcall(function()
return MarketplaceService:GetProductInfo(productId, Enum.InfoType.Product)
end)
if success and productInfo then
-- 제품 정보 표시
-- 인쇄 문을 UI 코드로 대체하여 제품을 표시하십시오
print("Developer Product Name: " .. productInfo.Name)
print("Price in Robux: " .. productInfo.PriceInRobux)
print("Description: " .. productInfo.Description)
end
Use GetDeveloperProductsAsync() to retrieve all developer products associating with your experience. This function returns a Pages object that you can inspect and filter to build things like an in-experience store or product list GUI.
local MarketplaceService = game:GetService("MarketplaceService")
local success, developerProducts = pcall(function()
return MarketplaceService:GetDeveloperProductsAsync()
end)
if success and developerProducts then
local firstPage = developerProducts:GetCurrentPage()
for _, developerProduct in firstPage do
-- 인쇄 문을 UI 코드로 대체하여 제품을 표시하십시오
print(field .. ": " .. value)
end
end
Class.MarketplaceService:PromptProductPurchase()|PromptProductPurchase() 를 사용하여 경험 내에서 제품 구매를 촉진합니다. 사용자가 버튼을 누르거나 판매자 NPC에게 이야기하는 등의 작업을 수행할 때 이 함수를 호출할 수 있습니다.
local MarketplaceService = game:GetService("MarketplaceService")
local Players = game:GetService("Players")
local player = Players.LocalPlayer
-- 대체 ID를 개발자 제품 ID로 교체
local productId = 000000
local function promptProductPurchase()
local success, errorMessage = pcall(function()
MarketplaceService:PromptProductPurchase(player, productId)
end)
if success then
print("Purchase prompt shown successfully")
end
end
Class.LocalScript|LocalScript 내에 함수를 조합하여 기능을 결합할 수도 있습니다. 예를 들어, 버튼이나 공급 업체 NPC와 같은 UI 요소를 생성한 다음 GetProductInfo()
local MarketplaceService = game:GetService("MarketplaceService")
local player = game.Players.LocalPlayer
local button = script.Parent
-- 대체 ID를 개발자 제품 ID로 교체
local productId = 000000
-- 사용자가 UI 버튼을 클릭할 때 제품 정보를 가져옵니다.
button.MouseButton1Click:Connect(function()
local success, productInfo = pcall(function()
return MarketplaceService:GetProductInfo(productId, Enum.InfoType.Product)
end)
if success and productInfo then
-- 제품이 판매 중인지 여부를 확인합니다.
if productInfo.IsForSale then
print("This is for sale")
-- 제품 구매 촉진
MarketplaceService:PromptProductPurchase(player, productId)
else
-- 제품이 판매되지 않음을 알립니다
print("This product is not currently for sale.")
end
else
print("Error retrieving product info: " .. tostring(productInfo))
end
end)
처리 개발자 상품 구매
사용자가 개발자 제품을 구매하면 트랜잭션을 처리하고 기록해야 합니다. 이를 수행하려면 Script 내에 있는 ServerScriptService 를 사용하십시오. ProcessReceipt() 함수를 사용하여.
local MarketplaceService = game:GetService("MarketplaceService")
local Players = game:GetService("Players")
local productFunctions = {}
-- 예: product ID 123123은 사용자를 완전한 체력으로 다시 가져옵니다.
productFunctions[123123] = function(receipt, player)
local character = player.Character
local humanoid = character and character:FindFirstChildWhichIsA("Humanoid")
if humanoid then
humanoid.Health = humanoid.MaxHealth
-- 성공적인 구매를 나타냅니다
return true
end
end
-- 예: product ID 456456은 사용자에게 100개의 금 코인을 보상으로 지급합니다.
productFunctions[456456] = function(receipt, player)
local leaderstats = player:FindFirstChild("leaderstats")
local gold = leaderstats and leaderstats:FindFirstChild("Gold")
if gold then
gold.Value += 100
return true
end
end
local function processReceipt(receiptInfo)
local userId = receiptInfo.PlayerId
local productId = receiptInfo.ProductId
local player = Players:GetPlayerByUserId(userId)
if player then
-- 개발자 제품 ID와 관련된 처리기 함수를 가져와 실행하려고 시도합니다.
local handler = productFunctions[productId]
local success, result = pcall(handler, receiptInfo, player)
if success then
-- 사용자는 자신의 아이템을 받았습니다
-- 거래를 확인하려면 "PurchaseGranted"를 반환합니다
return Enum.ProductPurchaseDecision.PurchaseGranted
else
warn("Failed to process receipt:", receiptInfo, result)
end
end
-- 사용자의 아이템은 수여할 수 없습니다.
-- 다시 사용자가 경험에 참여할 때 "NotProcessedYet"이 표시되고 다음에 다시 시도됩니다.
return Enum.ProductPurchaseDecision.NotProcessedYet
end
-- 콜백 설정
-- 이는 한 서버 사이드 스크립트에서만 수행할 수 있습니다.
MarketplaceService.ProcessReceipt = processReceipt
개발자 제품 분석
개발자 제품 분석을 사용하여 개별 제품의 성공을 분석하고, 동향을 확인하고, 미래의 잠재적 수입을 예측합니다.
애널리틱스사용하면 다음을 할 수 있습니다.
- 선택한 기간 동안 최고 개발자 제품을 보세요.
- 시계열 그래프에 최고 판매 아이템을 최대 8개까지 표시하여 전체 판매 및 순수익을 분석합니다.
- 카탈로그를 모니터링하고 판매량과 순수익으로 항목을 정렬합니다.
개발자 애널리틱스액세스하려면:
- 크리처 탭으로 이동하고 경험을 선택하세요.
- 수익 창출 으로 이동합니다 개발자 제품 > 개발자 제품 으로.
- 선택합니다 분석 탭.
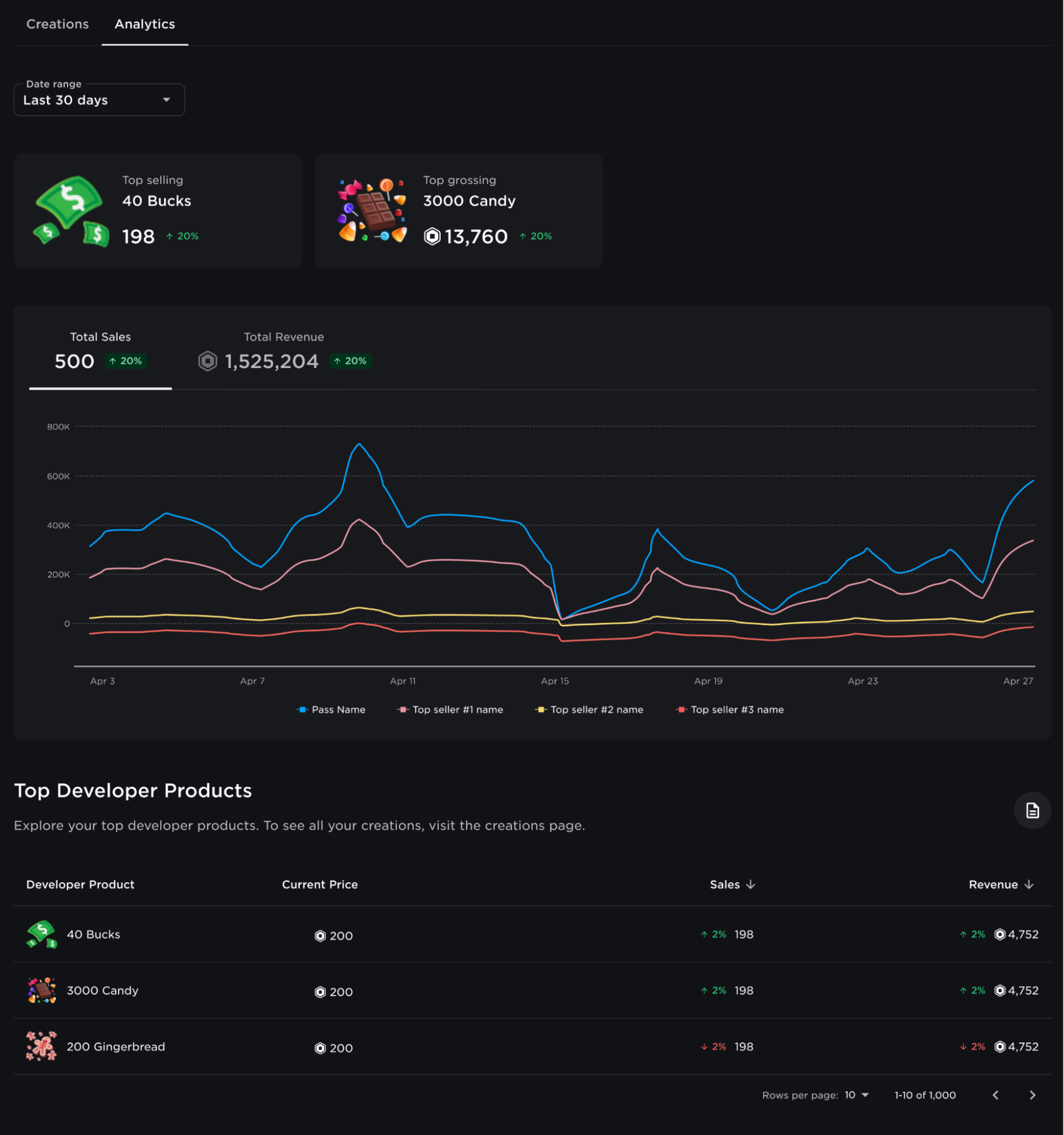