Roblox는 Xbox 및 PlayStation 컨트롤러와 같은 USB 게임 패드의 입력을 허용합니다.게임 패드는 다양한 종류로 제공되므로 플레이어의 게임 패드 입력이 경험에서 사용 가능한지 확인하기 위해 추가 설정을 따라야 합니다.
게임패드 입력을 설정하려면 또는 를 사용하여 플레이어의 기기연결된 게임패드를 검색하고, Roblox와 호환되는 지원되는 입력을 확인하고, 입력을 받으며 등등을 수행할 수 있습니다.
게임패드 입력을 바인딩할 때, 일반 컨트롤 스키마를 참조하여 플레이어에게 일관된 게임패드 경험을 제공합니다.입력이 설정된 후에는 지원되는 컨트롤러에 촉각 피드백을 포함하여 플레이어의 경험을 향상시킬 수 있습니다.
게임패드 감지
플레이어의 장치에 현재 게임패드가 활성화되어 있는지 여부를 검색할 수 있습니다 UserInputService.GamepadEnabled 속성을 사용하여.
게임패드 감지
local UserInputService = game:GetService("UserInputService")if UserInputService.GamepadEnabled thenprint("Player has gamepad enabled...")end
UserInputService.GamepadConnected 및 UserInputService.GamepadDisconnected 이벤트를 통해 연결된 게임패드를 확인할 수 있습니다.이 이벤트는 장치가 연결되거나 연결 해제될 때 발생하며, 두 이벤트 모두 연결된 함수에 Enum.UserInputType를 전달하여 이벤트를 발생시킨 게임패드를 나타냅니다.대부분의 경우, 연결된 게임패드는 Gamepad1입니다.
연결 및 연결 해제 확인
local UserInputService = game:GetService("UserInputService")
UserInputService.GamepadConnected:Connect(function(gamepad)
print("User has connected controller: " .. tostring(gamepad))
end)
UserInputService.GamepadDisconnected:Connect(function(gamepad)
print("User has disconnected controller: " .. tostring(gamepad))
end)
특정 컨트롤러가 UserInputService:GetGamepadConnected()을 사용하여 연결되었는지 쿼리할 수도 있습니다.이는 인수로 를 사용하고 오직 을 통해 값을 허용합니다.
특정 게임패드 연결 쿼리
local UserInputService = game:GetService("UserInputService")if UserInputService:GetGamepadConnected(Enum.UserInputType.Gamepad1) thenprint("Gamepad1 is connected")elseif UserInputService:GetGamepadConnected(Enum.UserInputType.Gamepad2) thenprint("Gamepad2 is connected")end
지원되는 입력 확인
게임 패드에는 다양한 입력 세트가 있을 수 있으므로 UserInputService:GetSupportedGamepadKeyCodes()로 지원되는 입력이 무엇인지 확인해야 합니다.이 메서드는 인수로 Enum.UserInputType 를 사용하고 지정된 컨트롤러에 대한 모든 입력 목록이 있는 테이블을 반환합니다.
지원되는 입력 확인
local UserInputService = game:GetService("UserInputService")local availableInputs = UserInputService:GetSupportedGamepadKeyCodes(Enum.UserInputType.Gamepad2)print("This controller supports the following controls:")for _, control in availableInputs doprint(control)end
입력 받기
는 게임패드와 마우스 및 키보드 입력이나 모바일 터치스크린 버튼과 같은 다른 입력 소스에 컨트롤을 바인딩하거나, 여러 기능을 단일 버튼 입력에 바인딩하는 데 유용합니다.예를 들어, 다음 코드 샘플은 게임패드의 OpenSpellBook 버튼과 키보드의 ButtonR2 키에 액션 B 을 바인딩합니다.
컨텍스트 액션 서비스 바인드 작업
local ContextActionService = game:GetService("ContextActionService")
local function openSpellBook(actionName, inputState, inputObject)
if inputState == Enum.UserInputState.Begin then
-- 스펠 북 열기
end
end
ContextActionService:BindAction("OpenSpellBook", openSpellBook, false, Enum.KeyCode.ButtonR2, Enum.KeyCode.B)
또는 게임패드에서 직접 컨트롤을 바인딩하기 위해 UserInputService를 사용할 수 있습니다.이 서비스를 통해 게임패드 이벤트를 감지할 때 InputBegan 이벤트를 사용하여 버튼이 처음 누르어졌을 때를 감지하고 InputEnded 이벤트를 사용하여 버튼이 릴리스될 때를 감지합니다.처리 함수에서 InputObject.UserInputType 속성은 어떤 게임패드가 이벤트를 발사했는지 나타내고 InputObject.KeyCode 특정 버튼이나 스틱이 발사한 것을 나타냅니다.
사용자 입력 서비스 버튼 누르기 감지
local UserInputService = game:GetService("UserInputService")
UserInputService.InputBegan:Connect(function(input)
if input.UserInputType == Enum.UserInputType.Gamepad1 then
if input.KeyCode == Enum.KeyCode.ButtonA then
print("Button A pressed on Gamepad1")
end
end
end)
게임패드 상태
UserInputService:GetGamepadState() 메서드를 사용하여 게임패드에 있는 모든 버튼과 스틱의 현재 상태를 감지할 수 있습니다.특정 이벤트가 경험에서 발생할 때 현재 게임패드 입력을 확인해야 하는 경우, 예를 들어 캐릭터가 물체에 닿았을 때 특정 버튼이 누르는지 확인하는 경우 유용합니다.
게임패드 입력 상태 확인
local Players = game:GetService("Players")
local UserInputService = game:GetService("UserInputService")
local player = Players.LocalPlayer
local character = player.Character or player.CharacterAdded:Wait()
local leftFoot = character:WaitForChild("LeftFoot")
-- 왼쪽 발이 무언가와 접촉할 때, 게임패드 입력 상태를 확인하십시오
leftFoot.Touched:Connect(function(hit)
local state = UserInputService:GetGamepadState(Enum.UserInputType.Gamepad1)
for _, input in state do
-- ButtonR2가 현재 유지되고 있는 경우 메시지를 출력합니다
if input.KeyCode == Enum.KeyCode.ButtonR2 and input.UserInputState == Enum.UserInputState.Begin then
print("Character's left foot touched something while holding right trigger")
end
end
end)
압력 트리거
입력 트리거의 Position.Z을 확인하여 게임패드 트리거에 얼마나 많은 압력이 가해지고 있는지 감지할 수 있습니다.
테스트 트리거 압력 확인
local UserInputService = game:GetService("UserInputService")
UserInputService.InputChanged:Connect(function(input)
if input.UserInputType == Enum.UserInputType.Gamepad1 then
if input.KeyCode == Enum.KeyCode.ButtonL2 then
print("Pressure on left trigger has changed:", input.Position.Z)
elseif input.KeyCode == Enum.KeyCode.ButtonR2 then
print("Pressure on right trigger has changed:", input.Position.Z)
end
end
end)
일반 제어 스키마
게임패드는 다양한 모양과 크기로 제공됩니다.플레이어 입력의 모든 방법과 마찬가지로, 다른 게임과 경험 간에 일관성을 만드는 것이 가장 좋습니다.
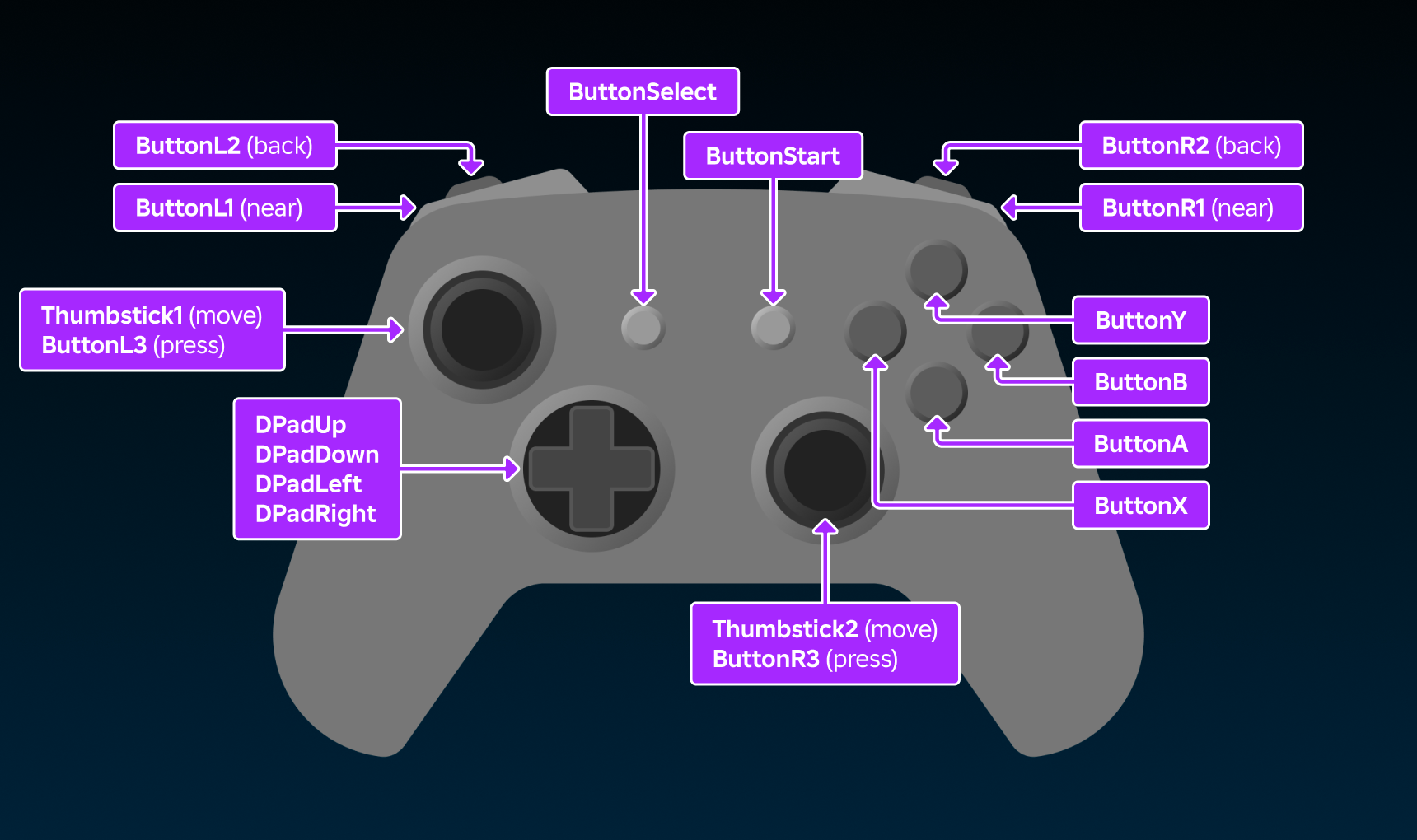
다음은 플레이어가 게임패드 제어즉시 익숙하고 편안하게 느끼도록 도울 일반적인 입력 바인드입니다:
입력 | 일반 사용 사례 |
---|---|
ButtonA | 플레이어 프롬프트나 GUI 선택을 수락합니다. 점프와 같은 주요 작업에 대해 대체로 사용됩니다. |
ButtonB | 플레이어 프롬프트나 GUI 선택을 취소합니다. 대체로 회피, 롤 또는 질주와 같은 보조 작업에 사용됩니다. |
Thumbstick1 | 일반적으로 문자 이동과 연관되어 있습니다. |
Thumbstick2 | 일반적으로 카메라 이동과 연관되어 있습니다. |
ButtonL2 , ButtonR2 | 일반적으로 발사와 같은 주요 작업에 사용됩니다. |
ButtonL1 , ButtonR1 , ButtonX , ButtonY | 재장전, 타겟팅 또는 인벤토리 또는 미니맵에 액세스와 같은 보조 작업. |
햅틱 피드백
많은 게임패드 컨트롤러에는 촉각 피드백을 제공하기 위해 모터가 내장되어 있습니다.쿠릉과 진동을 추가하면 플레이어의 경험을 크게 향상시킬 수 있으며 시각이나 오디오 이상의 미묘한 피드백을 제공할 수 있습니다.당신은 HapticService 를 사용하여 진동 지원을 확인할 수 있습니다 전에 모터를 켜기 전에 .
진동 지원
모든 컨트롤러에 모터가 있는 것은 아니므로 햅틱 모터를 사용하기 전에 지원을 확인하는 것이 중요합니다.지정된 컨트롤러에 진동 지원이 있는지 쿼리하려면 HapticService:IsVibrationSupported()를 호출하십시오.
진동 지원 확인
local HapticService = game:GetService("HapticService")local gamepad = Enum.UserInputType.Gamepad1local isVibrationSupported = HapticService:IsVibrationSupported(gamepad)
일부 컨트롤러에는 다양한 진동 규모에 대한 여러 모터가 있습니다.게임패드가 진동을 지원하는지 확인했으면, HapticService:IsMotorSupported()를 통해 사용하려는 모터를 지원하는지도 확인해야 합니다.
지원되는 모터 확인
local HapticService = game:GetService("HapticService")local gamepad = Enum.UserInputType.Gamepad1local isVibrationSupported = HapticService:IsVibrationSupported(gamepad)if isVibrationSupported thenlocal largeMotor = HapticService:IsMotorSupported(gamepad, Enum.VibrationMotor.Large)local smallMotor = HapticService:IsMotorSupported(gamepad, Enum.VibrationMotor.Small)local leftTriggerMotor = HapticService:IsMotorSupported(gamepad, Enum.VibrationMotor.LeftTrigger)local rightTriggerMotor = HapticService:IsMotorSupported(gamepad, Enum.VibrationMotor.RightTrigger)local leftHandMotor = HapticService:IsMotorSupported(gamepad, Enum.VibrationMotor.LeftHand)local rightHandMotor = HapticService:IsMotorSupported(gamepad, Enum.VibrationMotor.RightHand)end
크기 또는 위치 | 설명 |
---|---|
Large | 일반적인 움직임에 유용한 더 큰 모터. |
Small | 더 미묘한 진동, 예를 들어 타이어가 미끄러지거나 전기 충격과 같은 더 미묘한 진동에 유용한 더 작은 모터 |
LeftTrigger | 왼쪽 트리거 아래에. |
RightTrigger | 오른쪽 트리거 아래에. |
LeftHand | 컨트롤러의 왼쪽에. |
RightHand | 컨트롤러의 오른쪽. |
모터 활성화
플레이어의 게임패드가 진동을 지원하는지 확인했으면, HapticService:SetMotor()로 특정 모터를 켜십시오.이 메서드는 게임패드와 진동의 강도를 인수로 사용합니다.주파수는 0과 1 사이의 모든 값일 수 있습니다.
모터 활성화
local HapticService = game:GetService("HapticService")local gamepad = Enum.UserInputType.Gamepad1local isVibrationSupported = HapticService:IsVibrationSupported(gamepad)if isVibrationSupported thenlocal largeMotor = HapticService:IsMotorSupported(gamepad, Enum.VibrationMotor.Large)if largeMotor thenHapticService:SetMotor(gamepad, Enum.VibrationMotor.Large, 0.5)endend
컨트롤러 모델링
컨트롤러 에뮬레이터 에서 스튜디오에서 게임패드 입력을 정확하게 에뮬레이션할 수 있습니다.기본 컨트롤러는 일반적인 게임패드이지만, 왼쪽 상단의 선택 메뉴에서 PlayStation, Xbox 및 Quest 장치에 대한 대체 방법을 선택할 수 있습니다.

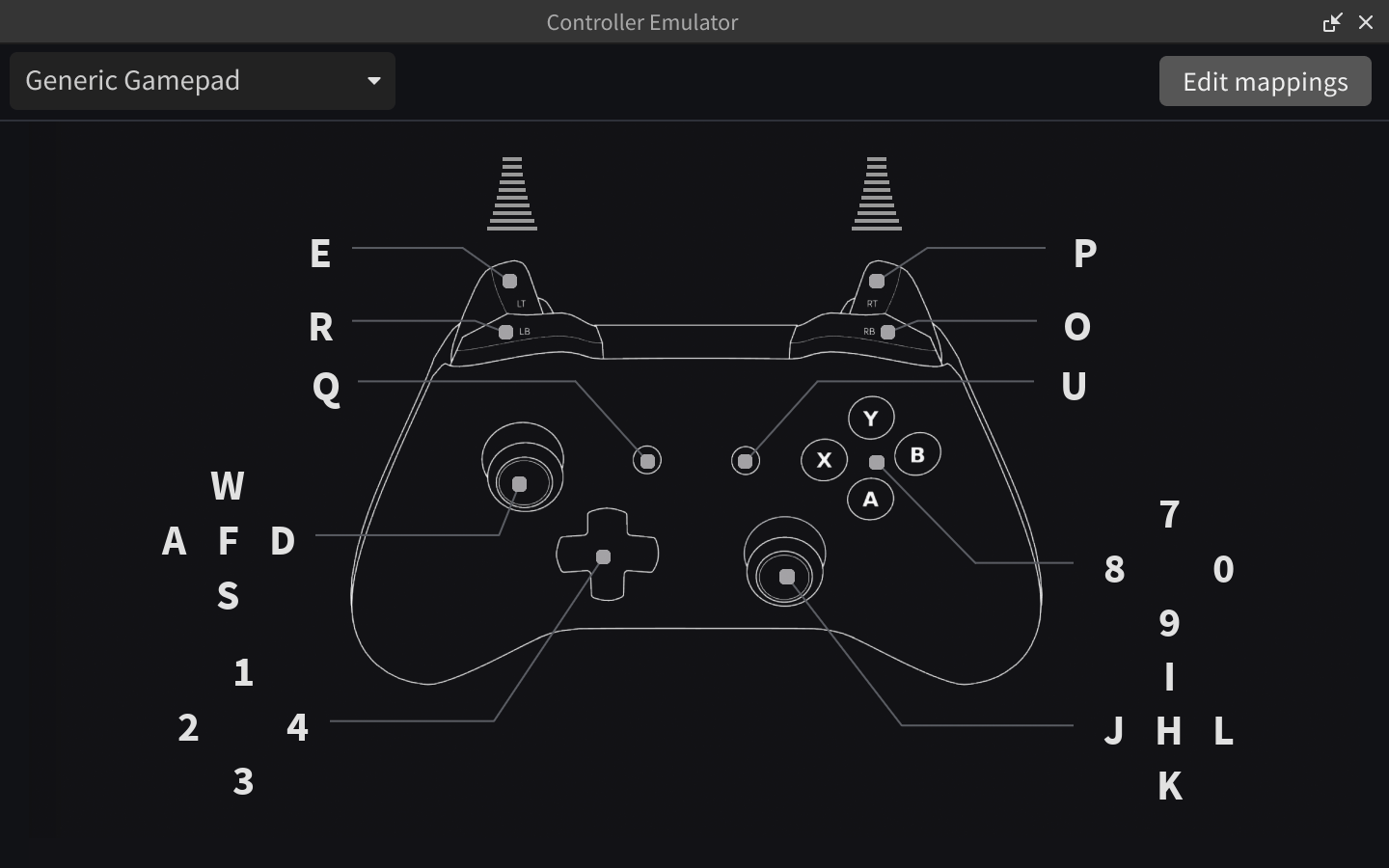
플레이테스트 중에는 마우스를 사용하여 가상 컨트롤러로 경험을 제어할 수 있습니다.
또한 오른쪽 상단 모서리에 있는 매핑 편집 을 클릭하여 가상 컨트롤러의 키 매핑을 보고 편집할 수 있습니다(예: E에서 ButtonL2 또는 9에서 ButtonA).이러한 매핑은 다른 Studio 설정과 마찬가지로 저장되며(컨트롤러당, 사용자당, 컴퓨터당) 에뮬레이터 창과 3D 뷰포트의 게임패드 이벤트로 번역됩니다.