Right now, much of the game information is currently in the Output Window, invisible to players. So players can be informed of what's happening in the game, you'll create a graphical user interface (GUI) and code it.
Displaying Information with a GUI
For this game a text label will display the current game status as well as the remaining player count and time.
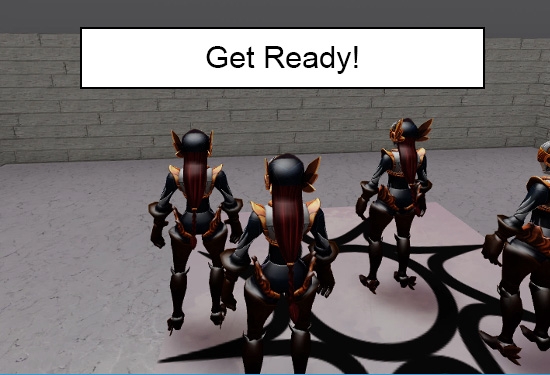
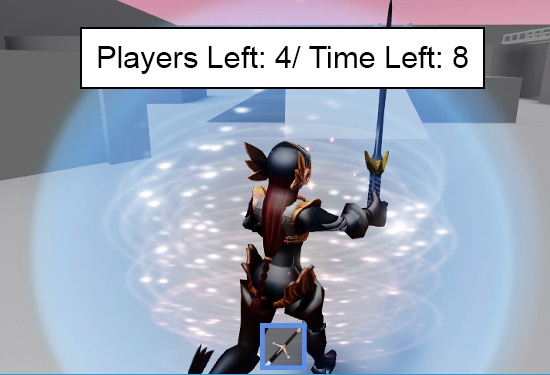
Setting up the GUI
First, create a Screen GUI object to hold the different text elements. When the player moves the camera, the screen GUI stays in the same place on their screen.
To ensure all players see the same display, place the GUI in the StarterGUI folder. At game startup, this folder is copied to all players.
In the StarterGUI folder, create a new ScreenGUI. Then in ScreenGUI, add a new TextLabel named StatusText.
To move the label, in the Explorer, select StatusText. Then, in the game view, drag the label where you would like it. Your numbers may differ from the video. The label can also be resized using the anchor points on the corners.
Scripting the GUI
To reflect changes in the game, scripts will need to update the GUI elements. For instance, the game status, whether it's an intermission or active round, will be stored in a StringValue and updated using local scripts.
Setting up the Script
The StatusDisplay script will be used to update the player's GUI whenever the game state changes.
In ReplicatedStorage, create a folder named DisplayValues. In that folder, add a StringValue named Status. To test the value later, give it a temporary value, like "Welcome to the Battle!".
In StarterGUI > ScreenGUI > Status, add a new local script named StatusDisplay. Scripts that affect the GUI are often parented to that GUI element.
Open StatusDisplay and define the following variables for the follow:
ReplicatedStorage service
DisplayValues folder
Status StringValue
TextLabel - use script.Parent.
local ReplicatedStorage = game:GetService("ReplicatedStorage")local displayValues = ReplicatedStorage:WaitForChild("DisplayValues")local status = displayValues:WaitForChild("Status")local textLabel = script.Parent
Changing the Text Label
To change the text in the label, use a Changed event so whenever the Status string is changed by another script, the text label will be updated.
Code a new function named updateText(). In that function, set the Text property of textLabel to status.Value.
local textLabel = script.Parentlocal function updateText()textLabel.Text = status.ValueendConnect the function to the Changed event.
local function updateText()textLabel.Text = status.Valueendstatus.Changed:Connect(updateText)So players see the most up to date status when starting the game, run updateText() at the end of the script.
local function updateText()textLabel.Text = status.Valueendstatus.Changed:Connect(updateText)updateText()Run the game and confirm that you see the temporary value in the display.
Creating the Display Manager
During a game, the text label will need to get information from GameManager, MatchManager, and possibly other scripts. So these different scripts can update the text label when needed, create a module script named DisplayManager.
Setting up the Script
Because DisplayManager needs to communicate with other scripts, it'll be a module script.
In ServerStorage > ModuleScripts, create a new module script named DisplayManager. Rename the module table to match the script name.
Add local variables for the following: Replicated Storage, DisplayValues folder, Status.
local DisplayManager = {}-- Serviceslocal ReplicatedStorage = game:GetService("ReplicatedStorage")-- Display Values used to update Player GUIlocal displayValues = ReplicatedStorage:WaitForChild("DisplayValues")local status = displayValues:WaitForChild("Status")-- Local Functions-- Module Functionsreturn DisplayManagerCreate a new module function named updateStatus() that updates the string in the Status value. Other scripts will be able to call this function.
-- Local Functions-- Module Functionsfunction DisplayManager.updateStatus(newStatus)status.Value = newStatusend
Updating the Text Status
With the Display Manager set up, it can be used in other scripts to update the GUI text label. As the first message in the GUI, show the start and end of the intermission through the GameManager script.
In ServerScriptService > GameManager, create a variable named displayManager and require the DisplayManager module in ServerStorage.
-- Serviceslocal ReplicatedStorage = game:GetService("ReplicatedStorage")local ServerStorage = game:GetService("ServerStorage")local Players = game:GetService("Players")-- Module Scriptslocal moduleScripts = ServerStorage:WaitForChild("ModuleScripts")local roundManager = require(moduleScripts:WaitForChild("RoundManager"))local gameSettings = require(moduleScripts:WaitForChild("GameSettings"))local displayManager = require(moduleScripts:WaitForChild("DisplayManager"))As the first line after the while true do statement, call displayManager > updateStatus() and pass in a message about waiting for players.
-- Eventslocal events = ServerStorage:WaitForChild("Events")local matchEnd = events:WaitForChild("MatchEnd")while true dodisplayManager.updateStatus("Waiting for Players")repeatprint("Starting intermission")task.wait(gameSettings.intermissionDuration)until #Players:GetPlayers() >= gameSettings.minimumPlayerstask.wait(gameSettings.transitionTime)matchManager.prepareGame()matchEnd.Event:Wait()endAfter the end of the repeat loop for the intermission, call updateStatus() and pass in a string announcing the match is starting. Since you'll be testing with the GUI, delete the two print statements for noting the start and end of the intermission.
while true dodisplayManager.updateStatus("Waiting for Players")repeattask.wait(gameSettings.intermissionDuration)until #Players:GetPlayers() >= gameSettings.minimumPlayersdisplayManager.updateStatus("Get ready!")task.wait(gameSettings.transitionTime)matchManager.prepareGame()matchEnd.Event:Wait()endTest the game with and without your minimum players. The message should read the following:
- Without the minimum players: "Waiting for Players".
- With the minimum players: "Get ready".
Troubleshooting Tips
At this point, if the text label doesn't display the first message, or still displays "Label", try one of the following below.
- Make sure in the StatusDisplay local script that updateText() is called at the bottom of the script. This ensures that the player gets the most up to date message.
- Check that the Status StringValue is in ReplicatedStorage. Due to the unique nature of client-server relations, if it's in ServerStorage, a local script won't be able to find it.
Displaying Match Status
During a match, the GUI will display two numbers: remaining player count and time. As these numbers change, the text label will change as well.
Setting up Values and Functions
IntValues will be used to store the player count and time left.
In ReplicatedStorage > DisplayValues, create two IntValues named PlayersLeft and TimeLeft.
In DisplayManager, add variables to store the players left and time left values.
local DisplayManager = {}-- Serviceslocal ReplicatedStorage = game:GetService("ReplicatedStorage")-- Display Values used to update Player GUIlocal displayValues = ReplicatedStorage:WaitForChild("DisplayValues")local status = displayValues:WaitForChild("Status")local playersLeft = displayValues:WaitForChild("PlayersLeft")local timeLeft = displayValues:WaitForChild("TimeLeft")Create a local function named updateMatchStatus(). Then, set the value of status to display the number of players left and the remaining time.
local displayValues = ReplicatedStorage:WaitForChild("DisplayValues")local status = displayValues:WaitForChild("Status")local playersLeft = displayValues:WaitForChild("PlayersLeft")local timeLeft = displayValues:WaitForChild("TimeLeft")-- Local Functionslocal function updateRoundStatus()status.Value = "Players Left: " .. playersLeft.Value .. " / Time Left: " .. timeLeft.ValueendFor both IntValue variables, connect updateRoundStatus() to the Changed event.
-- Module Functionsfunction DisplayManager.updateStatus(newStatus)status.Value = newStatusendplayersLeft.Changed:Connect(updateRoundStatus)timeLeft.Changed:Connect(updateRoundStatus)return DisplayManager
Displaying Players
Next, add the code for displaying the number of players at the start of a game. Later lessons will update the PlayersLeft value as players are eliminated from the game.
In PlayerManager, add local variables for ReplicatedStorage service, DisplayValues folder and PlayersLeft IntValue.
local PlayerManager = {}-- Serviceslocal Players = game:GetService("Players")local ServerStorage = game:GetService("ServerStorage")local ReplicatedStorage = game:GetService("ReplicatedStorage")-- Map Variableslocal lobbySpawn = workspace.Lobby.StartSpawnlocal arenaMap = workspace.Arenalocal spawnLocations = arenaMap.SpawnLocations-- Valueslocal displayValues = ReplicatedStorage:WaitForChild("DisplayValues")local playersLeft = displayValues:WaitForChild("PlayersLeft")Show the starting player count by setting the value of playersLeft to the size of the active players array.
Then, in sendPlayersToMatch(), under the for loop, type: playersLeft.Value = #activePlayers
function PlayerManager.sendPlayersToMatch()local availableSpawnPoints = spawnLocations:GetChildren()for playerKey, whichPlayer in Players:GetPlayers() dotable.insert(activePlayers,whichPlayer)local spawnLocation = availableSpawnPoints[1]table.remove(availableSpawnPoints, 1)preparePlayer(whichPlayer, spawnLocation)endplayersLeft.Value = #activePlayersend
Displaying the Timer
Remember that module scripts are used to centralize similar code. Since the timer is tracked in MatchManager, update the TimeLeft value using functions from the Timer script. The display manager will listen for changes to the TimeLeft, and update to match the new value.
In MatchManager, create variables to store the ReplicatedStorage service, DisplayValues folder and TimeLeft value.
local MatchManager = {}-- Serviceslocal ServerStorage = game:GetService("ServerStorage")local ReplicatedStorage = game:GetService("ReplicatedStorage")-- Module Scriptslocal moduleScripts = ServerStorage:WaitForChild("ModuleScripts")local playerManager = require(moduleScripts:WaitForChild("PlayerManager"))local gameSettings = require(moduleScripts:WaitForChild("GameSettings"))local timer = require(moduleScripts:WaitForChild("Timer"))-- Eventslocal events = ServerStorage:WaitForChild("Events")local matchStart = events:WaitForChild("MatchStart")-- Valueslocal displayValues = ReplicatedStorage:WaitForChild("DisplayValues")local timeLeft = displayValues:WaitForChild("TimeLeft")local myTimer = timer.new()Find the startTimer() function. After the timer's Finished event, copy and paste the whole, highlighted while loop below. The code runs a loop to update the timeLeft value as long as the timer is still active.
while myTimer:isRunning() do-- Adding +1 makes sure the timer display ends at 1 instead of 0.timeLeft.Value = (math.floor(myTimer:getTimeLeft() + 1))-- By not setting the time for wait, it offers more accurate loopingtask.wait()endWhen added in, the code should look like the sample below.
local function startTimer()print("Timer started")myTimer:start(gameSettings.matchDuration)myTimer.finished:Connect(timeUp)while myTimer:isRunning() do-- Adding +1 makes sure the timer display ends at 1 instead of 0.timeLeft.Value = (math.floor(myTimer:getTimeLeft() + 1))-- By not setting the time for wait, it offers more accurate loopingtask.wait()endendRun the game with the minimum players. Check that the status text displays:
- The correct amount of starting players. Remember, this number won't change until additional code is added in a future lesson.
- Time decreases each second until it stops at 1.
Completed Scripts
Below are completed scripts to double check your work.
GameManager Script
-- Serviceslocal ServerStorage = game:GetService("ServerStorage")local Players = game:GetService("Players")-- Module Scriptslocal moduleScripts = ServerStorage:WaitForChild("ModuleScripts")local matchManager = require(moduleScripts:WaitForChild("MatchManager"))local gameSettings = require(moduleScripts:WaitForChild("GameSettings"))local displayManager = require(moduleScripts:WaitForChild("DisplayManager"))-- Eventslocal events = ServerStorage:WaitForChild("Events")local matchEnd = events:WaitForChild("MatchEnd")while true dodisplayManager.updateStatus("Waiting for Players")repeattask.wait(gameSettings.intermissionDuration)until #Players:GetPlayers() >= gameSettings.minimumPlayersdisplayManager.updateStatus("Get ready!")task.wait(gameSettings.transitionTime)matchManager.prepareGame()matchEnd.Event:Wait()end
DisplayManager Script
local DisplayManager = {}
-- Services
local ReplicatedStorage = game:GetService("ReplicatedStorage")
-- Display Values used to update Player GUI
local displayValues = ReplicatedStorage:WaitForChild("DisplayValues")
local status = displayValues:WaitForChild("Status")
local playersLeft = displayValues:WaitForChild("PlayersLeft")
local timeLeft = displayValues:WaitForChild("TimeLeft")
-- Local Functions
local function updateRoundStatus()
status.Value = "Players Left: " .. playersLeft.Value .. " / Time Left: " .. timeLeft.Value
end
-- Module Functions
function DisplayManager.updateStatus(newStatus)
status.Value = newStatus
end
playersLeft.Changed:Connect(updateRoundStatus)
timeLeft.Changed:Connect(updateRoundStatus)
return DisplayManager
MatchManager Script
local ReplicatedStorage = game:GetService("ReplicatedStorage")
-- Module Scripts
local moduleScripts = ServerStorage:WaitForChild("ModuleScripts")
local playerManager = require(moduleScripts:WaitForChild("PlayerManager"))
local gameSettings = require(moduleScripts:WaitForChild("GameSettings"))
local timer = require(moduleScripts:WaitForChild("Timer"))
-- Events
local events = ServerStorage:WaitForChild("Events")
local matchStart = events:WaitForChild("MatchStart")
local matchEnd = events:WaitForChild("MatchEnd")
-- Values
local displayValues = ReplicatedStorage:WaitForChild("DisplayValues")
local timeLeft = displayValues:WaitForChild("TimeLeft")
local myTimer = timer.new()
-- Local Functions
local function timeUp()
print("Time is up!")
end
local function startTimer()
print("Timer started")
myTimer:start(gameSettings.matchDuration)
myTimer.finished:Connect(timeUp)
while myTimer:isRunning() do
-- Adding +1 makes sure the timer display ends at 1 instead of 0.
timeLeft.Value = (math.floor(myTimer:getTimeLeft() + 1))
-- By not setting the time for wait, it offers more accurate looping
task.wait()
end
end
-- Module Functions
function MatchManager.prepareGame()
playerManager.sendPlayersToMatch()
matchStart:Fire()
end
matchStart.Event:Connect(startTimer)
return MatchManager
StatusDisplay Script
local ReplicatedStorage = game:GetService("ReplicatedStorage")
local displayValues = ReplicatedStorage:WaitForChild("DisplayValues")
local status = displayValues:WaitForChild("Status")
local textLabel = script.Parent
local function updateText()
textLabel.Text = status.Value
end
status.Changed:Connect(updateText)
updateText()