With the in-experience text chat system, you can support bubble chat to display customizable speech chat bubbles above user avatars and NPCs. Bubble chat can make your experience more visually immersive and help users easily identify messages and their speakers in a contextually relevant manner. This feature is especially useful for experiences where users need to focus on the content in the meantime communicating with others in a less obtrusive way.
Bubble Chat Configuration
To enable bubble chat in your experience:
In the Explorer window, select BubbleChatConfiguration under TextChatService.
In the Properties window, check the Enabled checkbox under the Behavior category.
Bubble Customization
After enabling bubble chat, you can customize the appearance and behavior of your chat bubbles to match your experience theme. Use the Properties window of BubbleChatConfiguration for basic changes like text color and spacing. For advanced customization, such as adding background images for bubbles, add UI objects as children of BubbleChatConfiguration and then modify these objects.
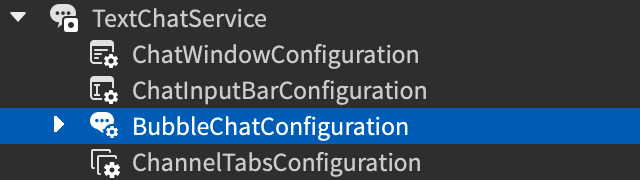
Alternatively, you can add a LocalScript in StarterPlayerScripts with all your customization settings. This allows the engine to apply your customizations during runtime, overriding the settings in Studio. It's useful for adding special effects to chat bubbles when users trigger certain events or conditions.
Basic Customization
The following table shows common bubble chat customization properties. For a full list of customization properties, see BubbleChatConfiguration.
Appearance
Property | Description | Default |
---|---|---|
BackgroundColor3 | Background color of bubbles in Color3. | [250, 250, 250] |
FontFace | Font of the bubble text. | GothamMedium |
TextColor3 | Color of bubble text in Color3. | [57, 59, 61] |
TextSize | Size of bubble text. | 16 |
Behavior
Property | Description | Default |
---|---|---|
Enabled | Indicating whether bubble chat is enabled in the experience. | true (checked) |
AdorneeName | String name of the body part or Attachment that bubbles attach to; if multiple instances of the same name exist, the system attaches to the first instance found. | HumanoidRootPart |
BubbleDuration | Time before a bubble fades out, in seconds. | 30 |
BubblesSpacing | Vertical space between stacked bubbles, in pixels. | 6 |
LocalPlayerStudsOffset | If adorned to the local player, the offset of bubbles in studs from their adornee, relative to the camera orientation (Vector3). | (0, 0, 0) |
MaxDistance | Maximum distance from the camera that bubbles are shown. | 100 |
MinimizeDistance | Distance from the camera when bubbles turn into a single bubble with an ellipsis (⋯) to indicate chatter. | 40 |
VerticalStudsOffset | Extra space between bubbles and their adornee, in studs. | 0 |
MaxBubbles | Maximum number of bubbles displayed before older bubbles disappear. | 3 |
Advanced Customization
For advanced customization of your bubble, add UI objects representing certain aspects of the bubble appearance as children under BubbleChatConfiguration, including:
- ImageLabel for background image settings.
- UIGradient for background gradient settings.
- UICorner for the corner shape of bubbles.
- UIPadding for the padding space between the text and bubble edges, relative to the parent's normal size.
To add these objects as children of BubbleChatConfiguration, you can either add a script or use the Studio user interface directly:
- Hover over BubbleChatConfiguration and click the ⊕ button.
- Select the object from list.
After adding these objects, you can modify properties of these objects applicable to chat bubbles for advanced bubble customization. The following example client-side LocalScript adds a background image and sharp corners to bubbles:
Advanced Bubble Customization
local BubbleChatConfiguration = game:GetService("TextChatService").BubbleChatConfigurationBubbleChatConfiguration.TailVisible = falseBubbleChatConfiguration.TextSize = 24BubbleChatConfiguration.TextColor3 = Color3.fromRGB(220, 50, 50)BubbleChatConfiguration.FontFace = Font.fromEnum(Enum.Font.LuckiestGuy)local UICorner = BubbleChatConfiguration:FindFirstChildOfClass("UICorner")if not UICorner thenUICorner = Instance.new("UICorner")UICorner.Parent = BubbleChatConfigurationendUICorner.CornerRadius = UDim.new(0, 0)local ImageLabel = BubbleChatConfiguration:FindFirstChildOfClass("ImageLabel")if not ImageLabel thenImageLabel = Instance.new("ImageLabel")ImageLabel.Parent = BubbleChatConfigurationendImageLabel.Image = "rbxassetid://6733332557"ImageLabel.ScaleType = Enum.ScaleType.SliceImageLabel.SliceCenter = Rect.new(40, 40, 360, 160)ImageLabel.SliceScale = 0.5
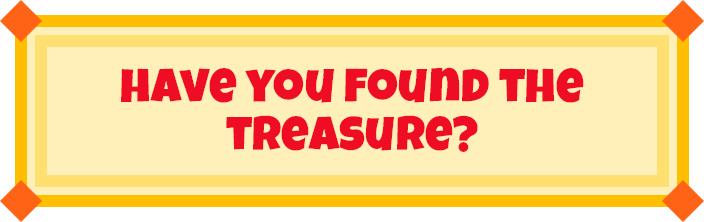
The following tables include all available properties for customization:
ImageLabel
Property | Description | Default |
---|---|---|
Image | Asset ID of the bubble background image. | |
ImageColor3 | Color tint of the bubble background image in Color3. | [255, 255, 255] |
ImageRectOffset | Offset of the image area to be displayed from the top-left in pixels. | (0, 0) |
ImageRectSize | Size of the image area to be displayed in pixels. To display the entire image, set either dimension to 0. | (0, 0) |
ScaleType | The scale type for rendering the image when its size is different from the absolute size of the bubble. | Stretch |
SliceCenter | Slice boundaries of the image if the image is a 9-sliced image. Only applicable when you set ScaleType as Slice. | (0, 0, 0, 0) |
SliceScale | Scale ratio of slice edges if the image is a 9-sliced image. Only applicable when you set ScaleType as Slice. | 1 |
TileSize | Tiling size of the image. Only applicable when you set ScaleType as Tile. | (1, 0, 1, 0) |
UIGradient
Property | Description | Default |
---|---|---|
Enabled | Indicating whether the bubble background gradient is enabled. | false (unchecked) |
Color | Color of the background gradient. | [250, 250, 250] |
Offset | Scalar translation of the gradient from the center of the bubble. | (0, 0) |
Rotation | Clockwise rotation, in degrees, of the gradient starts from left to right. | 0 |
Transparency | Transparency of the background gradient. | (1, 0) |
UICorner
Property | Description | Default |
---|---|---|
CornerRadius | Radius of the bubble corner shape in pixels. | (0, 12) |
UIGradient
Property | Description | Default |
---|---|---|
PaddingBottom | Padding on the bottom. | UDim.new(0,8) |
PaddingLeft | >Padding on the left. | UDim.new(0,8) |
PaddingRight | Padding on the right. | UDim.new(0,8) |
PaddingTop | Padding on the top. | UDim.new(0,8) |
Per-Bubble Customization
You can individually style and modify chat bubble behaviors based on specific conditions that overrides your general settings. For example, you can use chat bubbles to differentiate NPCs and users, highlight critical health status, and apply special effects to messages with pre-defined keywords.
To set per-bubble customization, add a client-side LocalScript using BubbleChatMessageProperties, which overrides matching properties of BubbleChatConfiguration, and the TextChatService.OnBubbleAdded callback to specify how to customize each bubble. The callback supplies you with the TextChatMessage property as well as the adornee, so you can apply the customization based on attributes associated with users, the chat text content, user character properties, and any special conditions you want to define.
The following example adds special appearance to VIP users' chat bubbles by checking if a chat message sender has the IsVIP attribute:
VIP Bubbles
local TextChatService = game:GetService("TextChatService")
local Players = game:GetService("Players")
-- Event handler for when a new chat bubble is added to the experience
TextChatService.OnBubbleAdded = function(message: TextChatMessage, adornee: Instance)
-- Check if the chat message has a TextSource (sender) associated with it
if message.TextSource then
-- Create a new BubbleChatMessageProperties instance to customize the chat bubble
local bubbleProperties = Instance.new("BubbleChatMessageProperties")
-- Get the user who sent the chat message based on their UserId
local player = Players:GetPlayerByUserId(message.TextSource.UserId)
if player:GetAttribute("IsVIP") then
-- If the player is a VIP, customize the chat bubble properties
bubbleProperties.TextColor3 = Color3.fromHex("#F5CD30")
bubbleProperties.BackgroundColor3 = Color3.fromRGB(25, 27, 29)
bubbleProperties.FontFace = Font.fromEnum(Enum.Font.PermanentMarker)
end
return bubbleProperties
end
end
Available Options
The following basic customization properties are available for per-bubble customization:
Property | Description | Default |
---|---|---|
BackgroundColor3 | Background color of bubbles in Color3. | (250, 250, 250) |
BackgroundTransparency | Background transparency of bubbles. | 0.1 |
FontFace | Font of the bubble text. | GothamMedium |
TextColor3 | Color of bubble text in Color3. | [57, 59, 61] |
TextSize | Size of bubble text. | 16 |
All advanced customization options are available for per-bubble customization. Similar to advanced customization for general bubbles, add instances that you want to customize as children of BubbleChatMessageProperties. The following example adds a special gradient effect along with other properties to chat bubbles of users with low health status by checking the Humanoid.Health property of chat message senders' characters:
Low Health Bubbles
local TextChatService = game:GetService("TextChatService")
local Players = game:GetService("Players")
-- Event handler for when a new chat bubble is added to the experience
TextChatService.OnBubbleAdded = function(message: TextChatMessage, adornee: Instance)
-- Check if the chat message has a TextSource (sender) associated with it
if message.TextSource then
-- Get the user who sent the chat message by using their UserId
local player = Players:GetPlayerByUserId(message.TextSource.UserId)
-- Find the humanoid in the user's character
local humanoid = player.Character:FindFirstChild("Humanoid")
if humanoid and humanoid.Health < 25 then
-- Create a new BubbleChatMessageProperties instance to customize the chat bubble
local bubbleProperties :BubbleChatMessageProperties = Instance.new("BubbleChatMessageProperties")
-- Customize the chat bubble properties for low health condition
bubbleProperties.BackgroundColor3 = Color3.fromRGB(245, 245, 245)
bubbleProperties.TextColor3 = Color3.fromRGB(234, 51, 96)
bubbleProperties.TextSize = 20
bubbleProperties.FontFace = Font.fromEnum(Enum.Font.DenkOne)
-- Add a UIGradient as a child to customize the gradient
local uiGradient : UIGradient = Instance.new("UIGradient")
uiGradient.Color = ColorSequence.new(Color3.fromRGB(110, 4, 0), Color3.fromRGB(0, 0, 0))
uiGradient.Parent = bubbleProperties
uiGradient.Rotation = 90
return bubbleProperties
end
end
end
NPC Bubbles
You can display chat bubbles for non-player characters (NPCs) by calling TextChatService:DisplayBubble(), with the NPC character and the message as parameters. These bubbles are customizable using the TextChatService.OnBubbleAdded callback just like any other chat bubble.
TextChatService:DisplayBubble() only works on client-side scripts, so be sure to use a LocalScript in an appropriate container, such as StarterPlayerScripts. If you attach a ProximityPrompt to an NPC, a script for displaying a chat bubble might look like this:
local TextChatService = game:GetService("TextChatService")
local prompt = workspace.SomeNPC.ProximityPrompt
local head = prompt.Parent:WaitForChild("Head")
prompt.Triggered:Connect(function()
TextChatService:DisplayBubble(head, "Hello world!")
end)