Roblox 为每个体验提供一个秘密商店。秘密是与外部服务进行身份验证的敏感信息,例如 API 钥匙、密码和访问代币。例如,如果您想连接到第三方分析或音乐服务,您可能需要使用 API 密钥进行身份验证。
您可以将 API 钥匙复制并粘贴到脚本中或添加到数据存商店中,但这些方法带来了不必要的安全风险。更好的解决方案是使用秘密存储并使用小集安全方法访问钥匙。
添加秘密
要查看、创建 or 创作或编辑秘密,你必须是体验所有者或群组所有者。你可以拥有每个体验最多 500 个秘密。
导航到 创建者仪表板。
选择您的体验,然后选择 秘密 > 创建秘密 。
提供名称、秘密和适用域。
- 名称作为秘密的唯一标识符,因此我们建议使用描述性的东西。
- 秘密长度最多可达 1,024 个字符。API 钥匙和访问代币应来自服务提供商,但如果秘密是密码,你可能是自己创建的。
- 您可以为域使用有限匹配符语法,例如 * 对任何域 (不推荐) 或 *.example.com 对任何子域在 example.com 上。特定域更好,例如 my.example.com 。
本地秘密
为了安全原因,每个体验的秘密存储仅限于生产游戏服务器或 团队测试 环境。如果您尝试从本地测试服务器访问秘密,例如在 Studio 按下 播放 按钮后,您会收到一个 Can't find secret with given key 错误。
要为本地测试指定秘密,请在 游戏设置 中添加带有 base64 编码秘密的有效 JSON 对象。JSON 可以包含空格,但必须放在单行上。
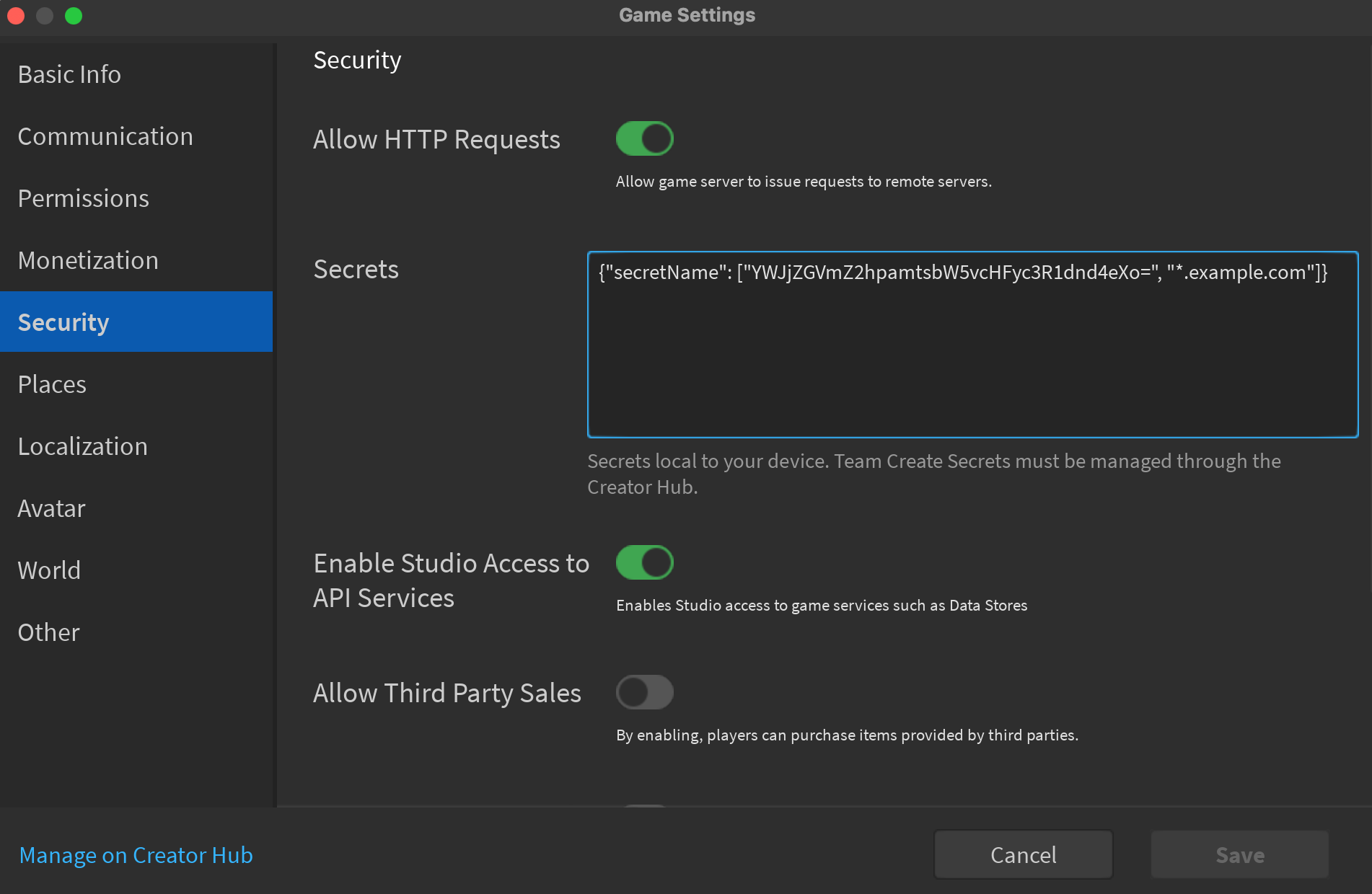
例如,以下是 base64 编码的字符串 abcdefghijklmnopqrstuvwxyz 被限制为子域在 example.com :
{"secretName": ["YWJjZGVmZ2hpamtsbW5vcHFyc3R1dnd4eXo=", "*.example.com"]}
要添加多个秘密,用逗号分开值:
{"secretName1": ["dGVzdDE=", "*.example.com"],"secretName2": ["dGVzdDI=", "*.example.com"],"secretName3": ["dGVzdDM=", "*.example.com"]}
使用秘密
在使用体验中的秘密之前,您必须在 游戏设置 的安全选项卡中启用 允许 HTTP 请求 。然后在脚本中调用 HttpService:GetSecret() :
local HttpService = game:GetService("HttpService")local testSecret = HttpService:GetSecret("test_secret")
使用秘密商店的一个优点是,你不能意外打印秘密。而不是秘密本身,以下代码输出您在创建秘密时提供的名称:
print(testSecret) --> Secret(test_secret)
您不能直接操纵字符串。相反,Secret数据类型允许您添加前缀和后缀到秘密以帮助形成URL或插入内容,如Bearer:
local HttpService = game:GetService("HttpService")local testSecret = HttpService:GetSecret("test_secret")local prefix = "https://my.example.com/endpoint?apiKey="local suffix = "&user=username"local url = testSecret:AddPrefix(prefix)url = url:AddSuffix(suffix)print(url) --> https://my.example.com/endpoint?apiKey=Secret(test_secret)&user=username
在你拥有插入了秘密的 URL 之后,你可以使用方法如 HttpService:RequestAsync() 来发出标准 HTTP 请求。当然,你也可以忽略这些方法,直接将秘密插入到头部中。