The Path2D instance, along with its API methods and properties, lets you implement 2D splines and 2D curved lines, useful for UI effects like path‑based animations and graph editors.
Create a 2D path
To add a Path2D to the screen or an in-experience object:
In the Explorer window, insert a Path2D instance under a visible ScreenGui or SurfaceGui (it does not need to be a direct child).
Select the new Path2D to reveal the in-viewport tooling widget. By default, the Add Point tool is selected.
Begin clicking on the screen to add a series of control points to form a path. The initial path will likely be imprecise but you can fine‑tune the position of any control point later.
When finished, click the widget's Done button or press Enter.
Modify control points
With a Path2D selected in the Explorer hierarchy, you can modify its individual control points as well as their tangents.
Move points
To move an individual control point on a path, enable the Select tool (V) and then click‑and‑drag it to a new position.

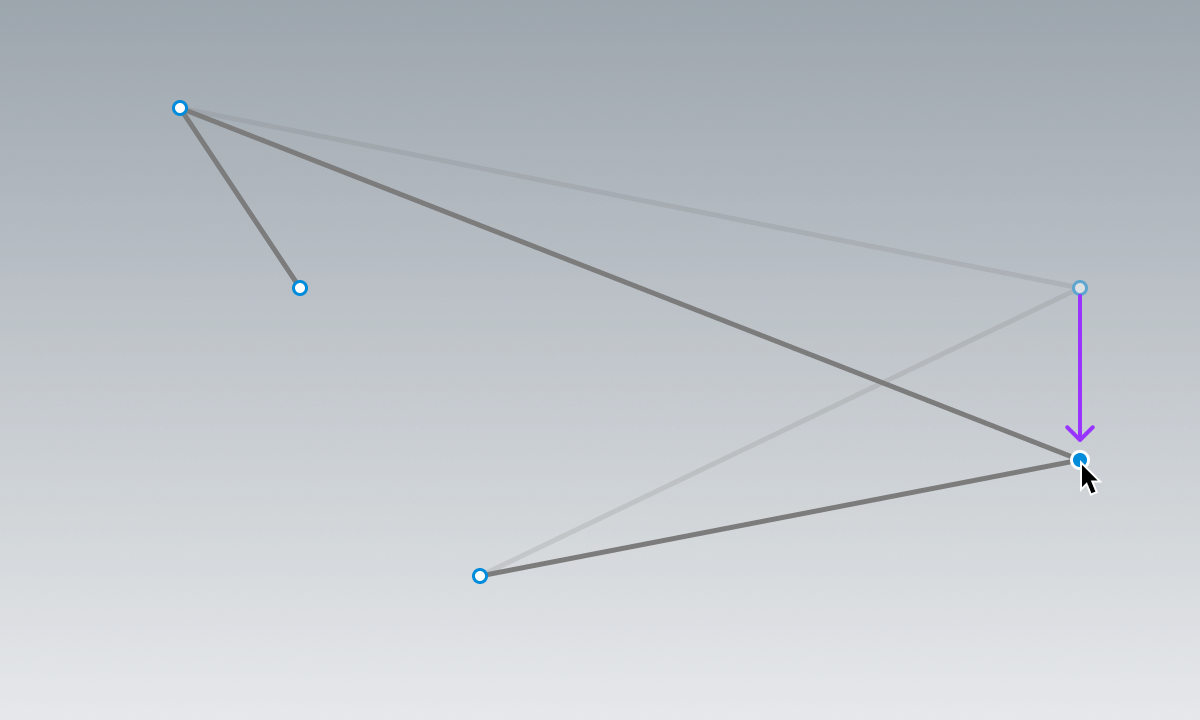
For very specific positioning, select the control point and then, in the Properties window, set a new position for the point's SelectedControlPointData property (UDim2).
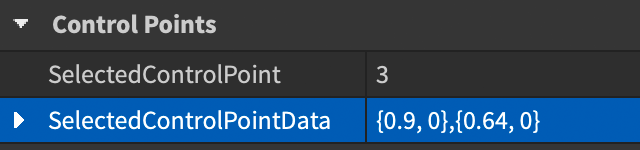
Note that a point's position is not absolute, but rather relative to the path's parent container. For example, compare a control point 30% from left and 20% from top for a path inside a ScreenGui, versus an identical path placed inside a Frame located in the upper‑right corner of the ScreenGui.
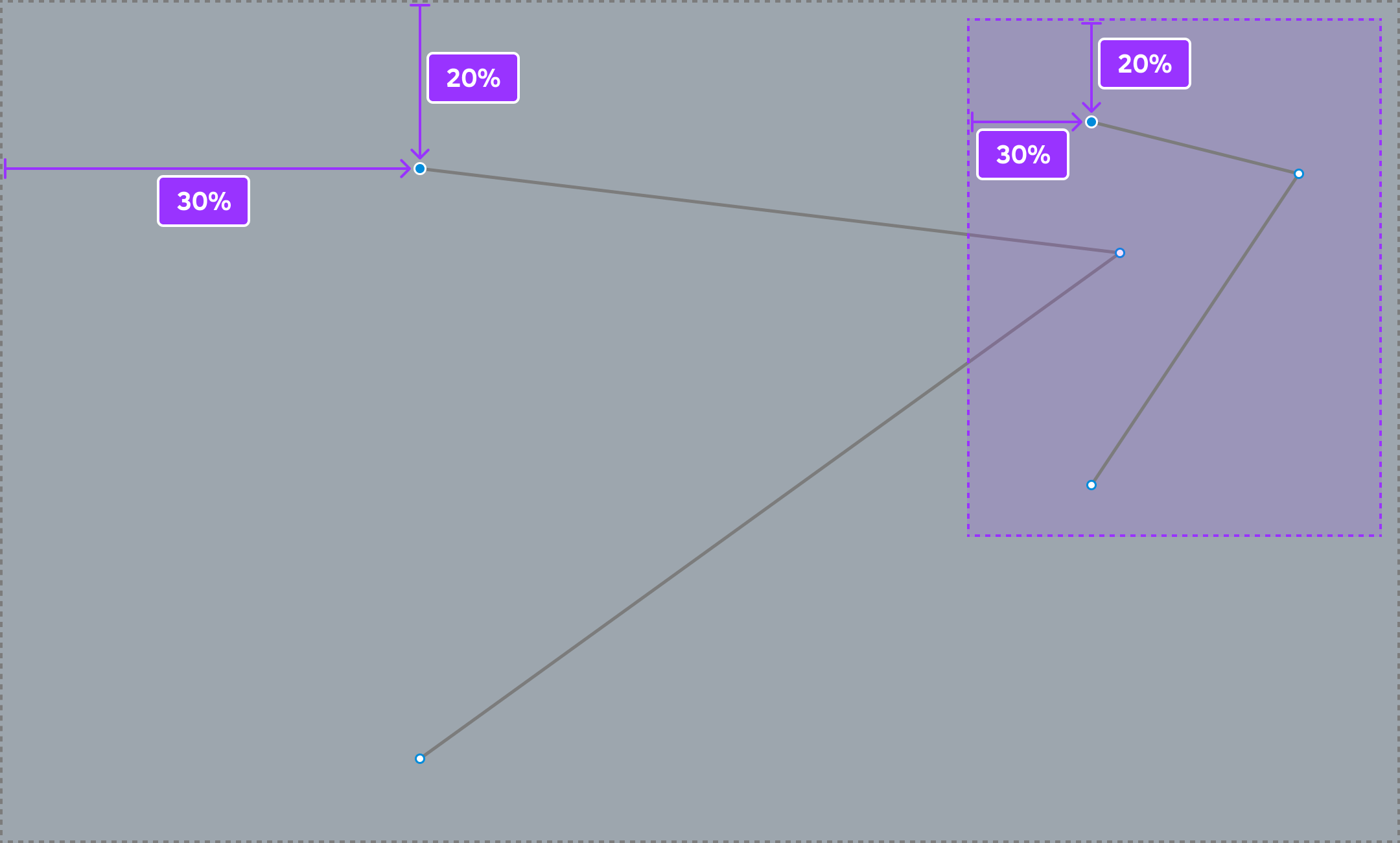
Add points
New control points can be added to a Path2D either between two existing points or from either end point using the Add Point tool (P).

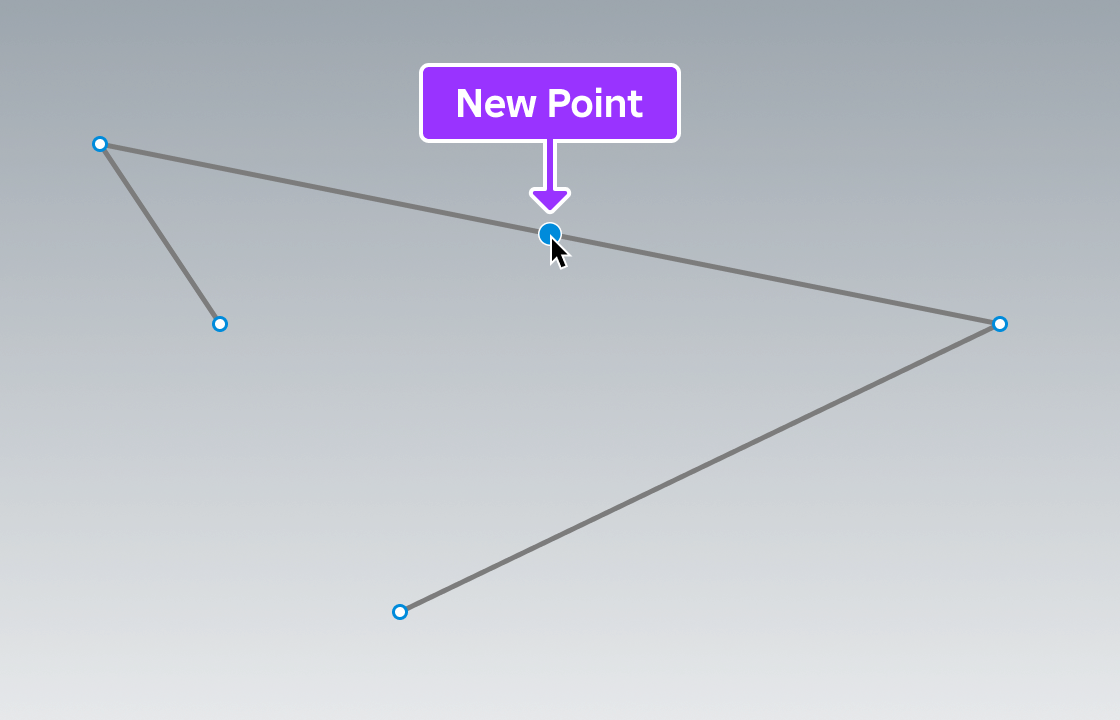
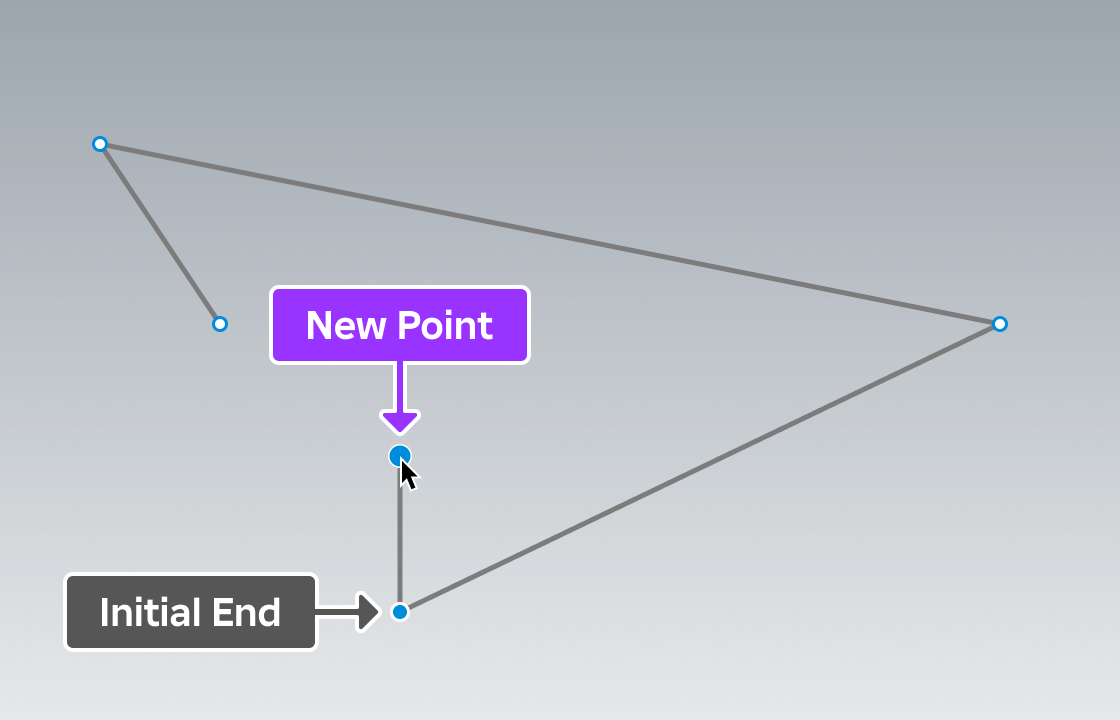
Delete points
To delete a control point, hover over and right‑click it, then select Delete Point from the contextual popup menu.
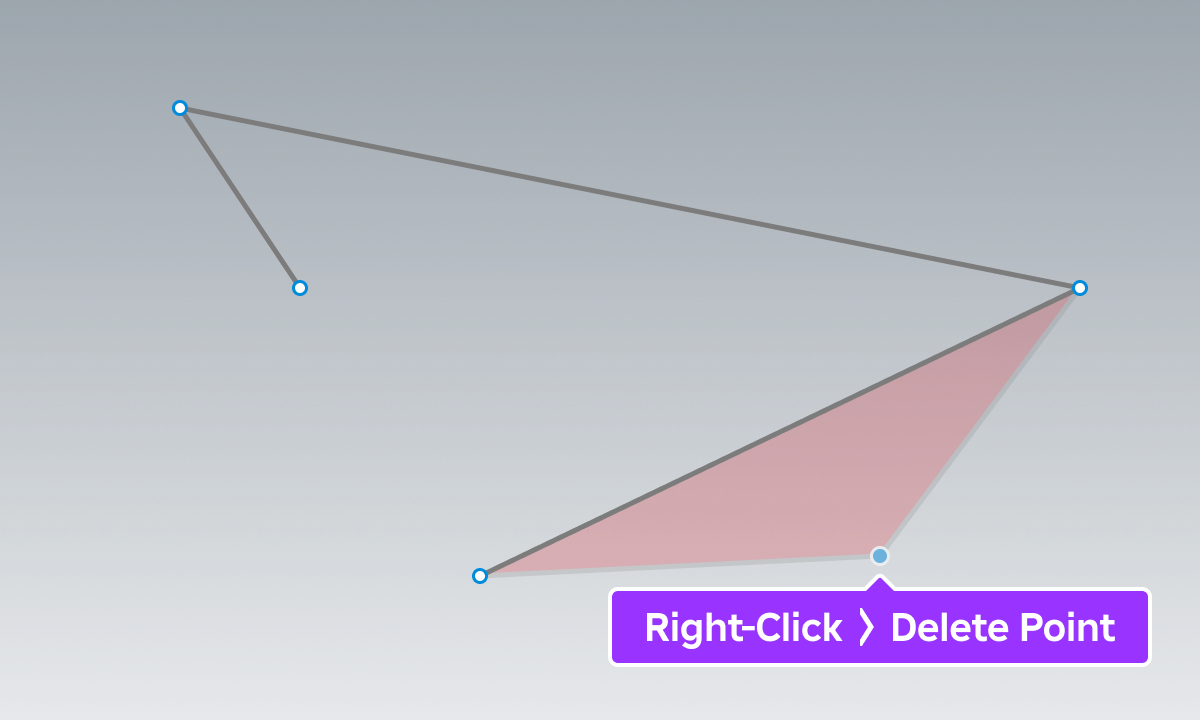
Control point tangents
Control point tangents let you create and adjust curves on a path.
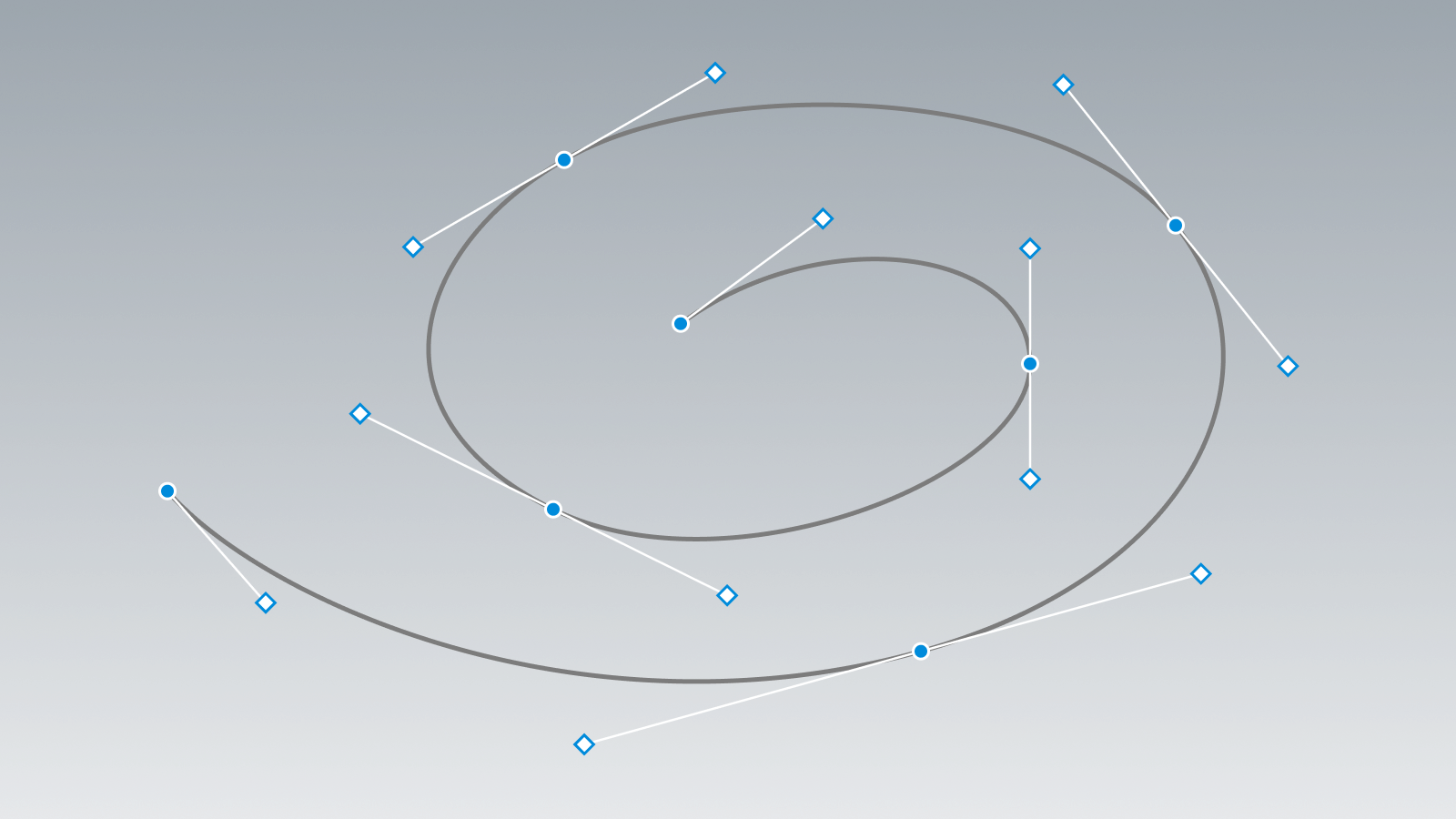
Add tangents
To add tangents to any control point that doesn't already have tangents:
Enable the Add Tangent tool in the tooling widget.
Adjust tangents
To adjust existing tangents for an individual control point:
Enable the Select tool (V).
Hover over a tangent marker (not the control point), then click‑and‑drag it to a new position. If the tangents are mirrored, the paired tangent point will move in unison.
To manually set a specific UDim2 position for a tangent:
Enable the Select tool (V) and select the control point. In the Properties window, expand the SelectedControlPointData field to expose the LeftTangent and RightTangent properties.
Set the position for LeftTangent and/or RightTangent. Note that this will break the mirrored behavior of the tangents.
Delete tangents
To delete both tangents from a control point, hover over and right-click that point, then select Clear Tangents from the contextual popup menu.
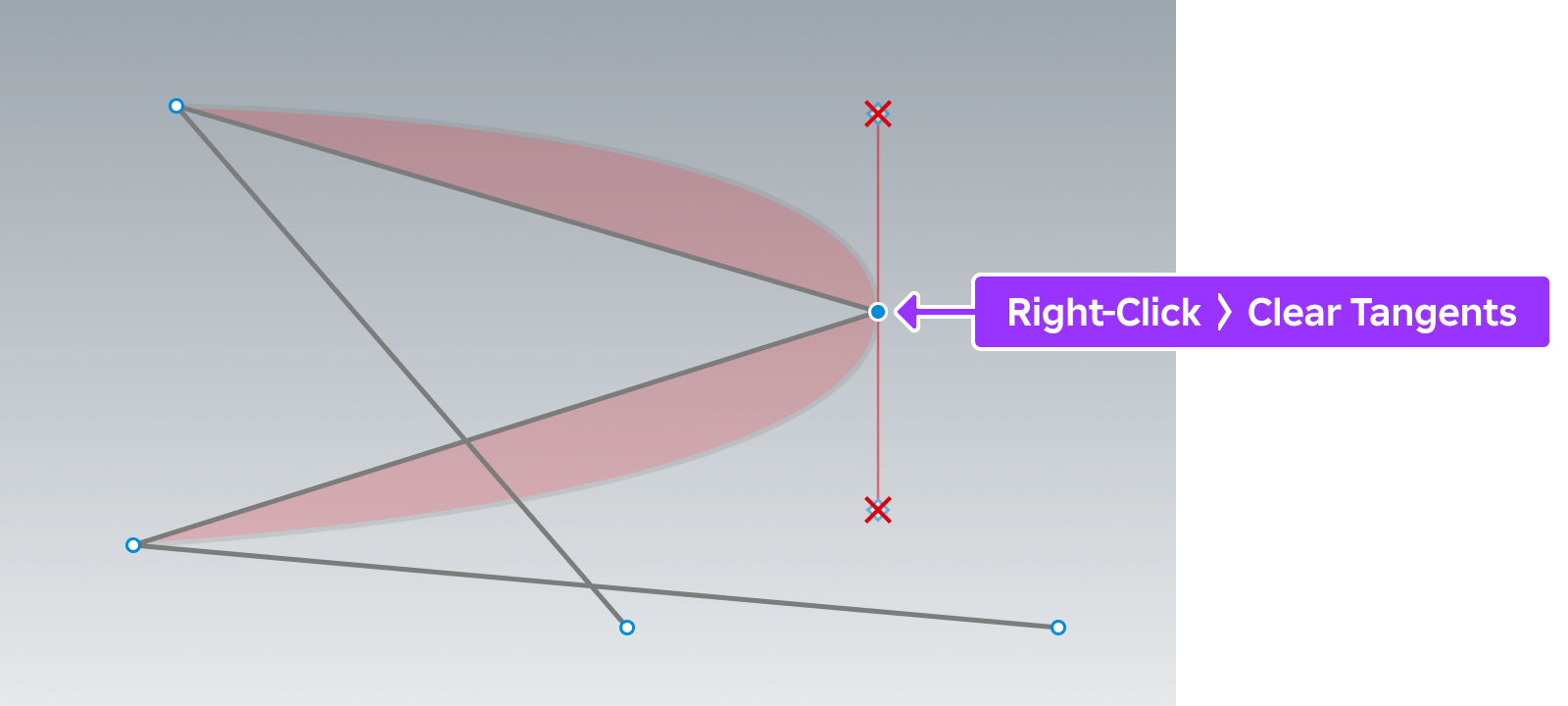
To delete just one of the tangents (left or right), hover over and right‑click that tangent's marker, then select Delete Tangent.
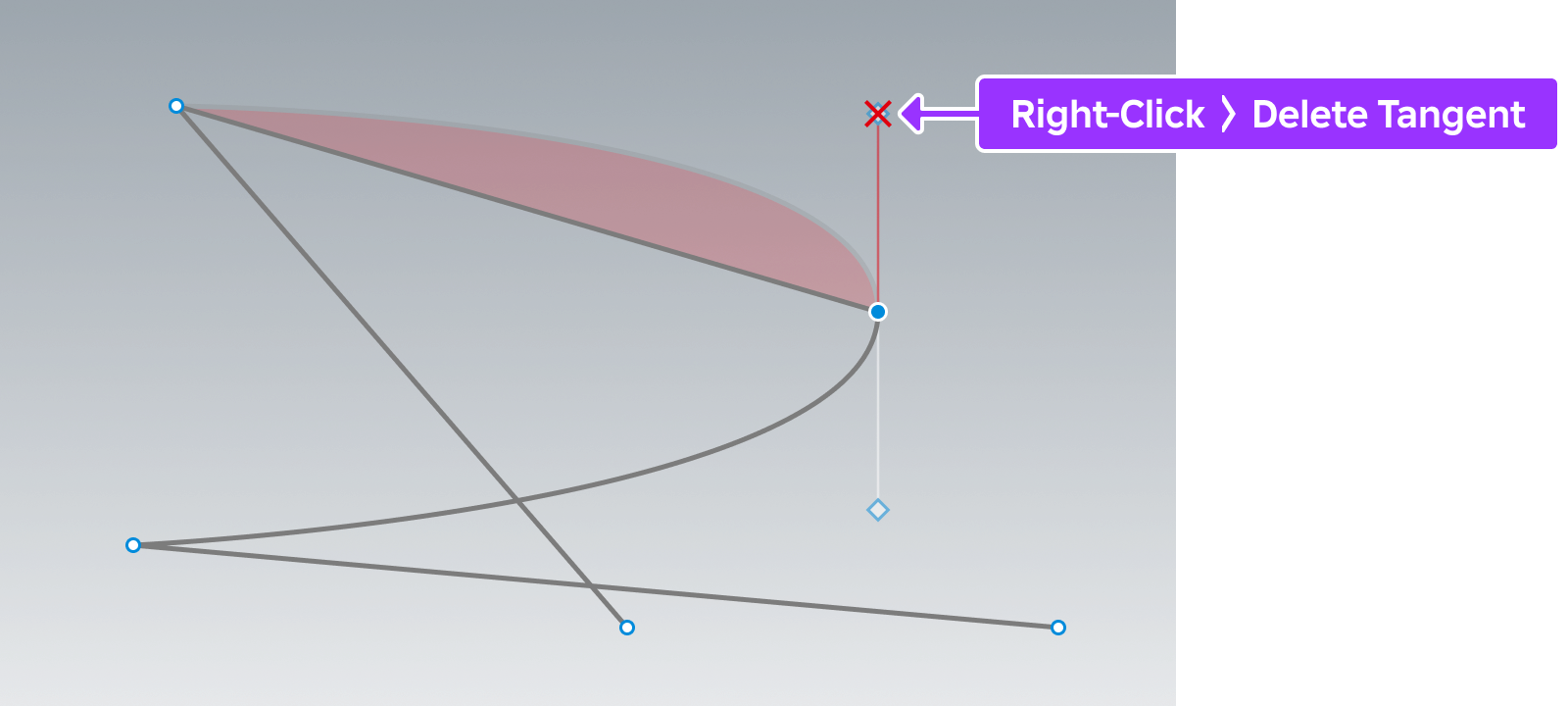
Break and mirror
By default, tangents mirror each other. When you drag to adjust one tangent marker, the paired tangent point will move in unison.
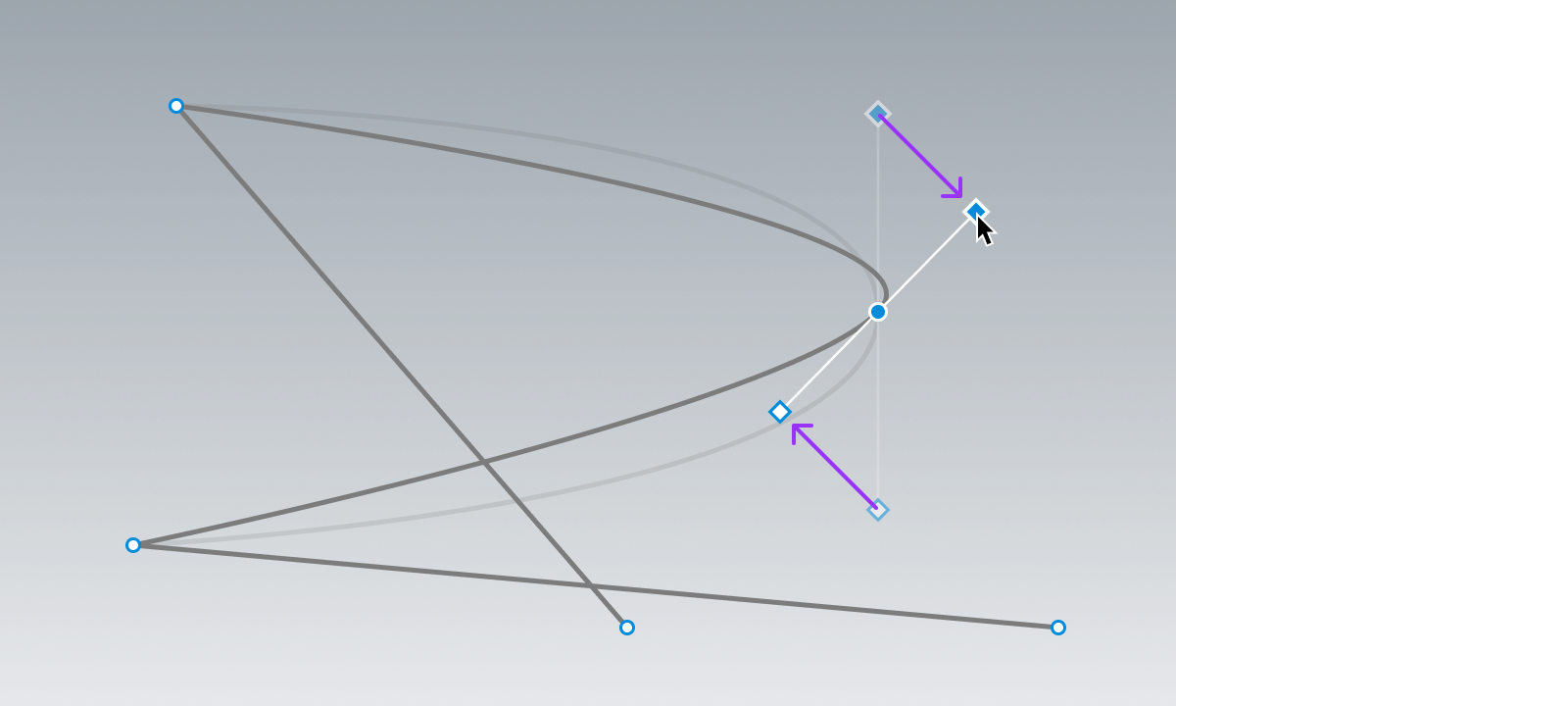
To "break" the tangents so that each can be moved independently of the other, hover over and right‑click the associated control point, then select Break Tangents from the contextual menu. Once broken, you can move each tangent marker without affecting the other.
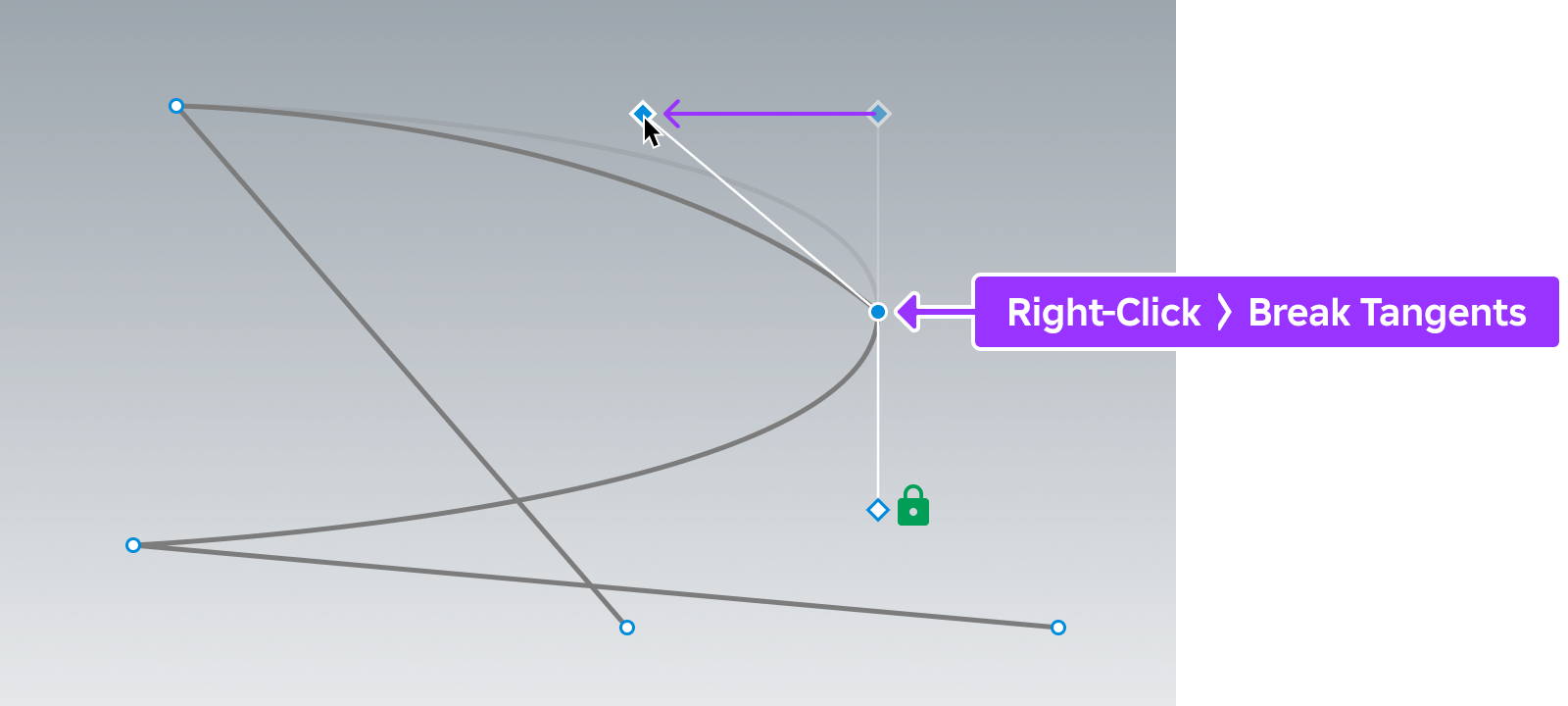
Path visual properties
You can customize the visual appearance of a Path2D with the following properties:
Property | Description |
---|---|
Color3 | Sets the color of the path line. |
Thickness | Sets the thickness of the path line, in pixels. |
Visible | Make the path visible or not in both edit and runtime. |
ZIndex | Order in which a path renders relative to other GUIs. |
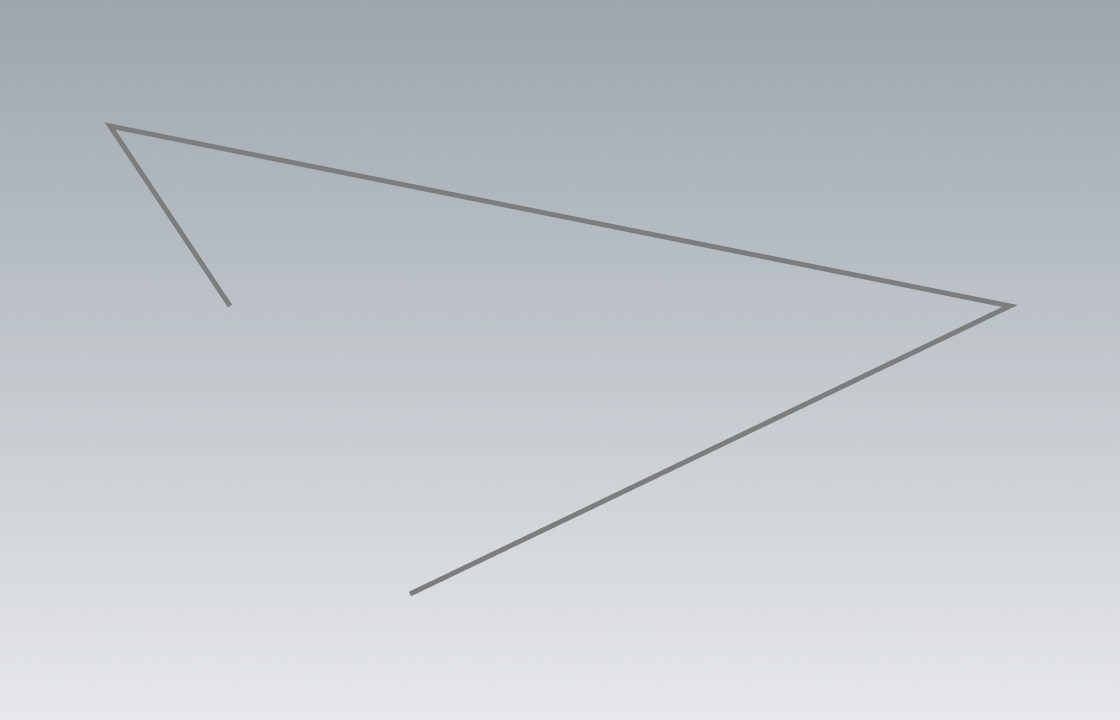
Color3 = (125, 125, 125)
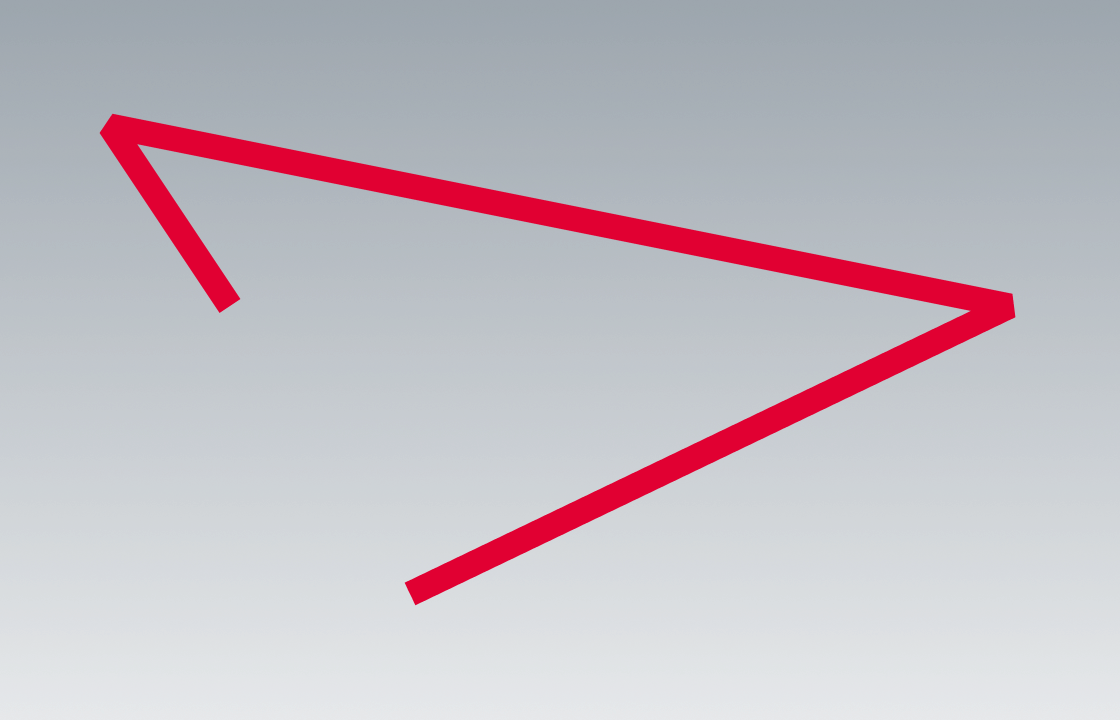
Color3 = (225, 0, 50)
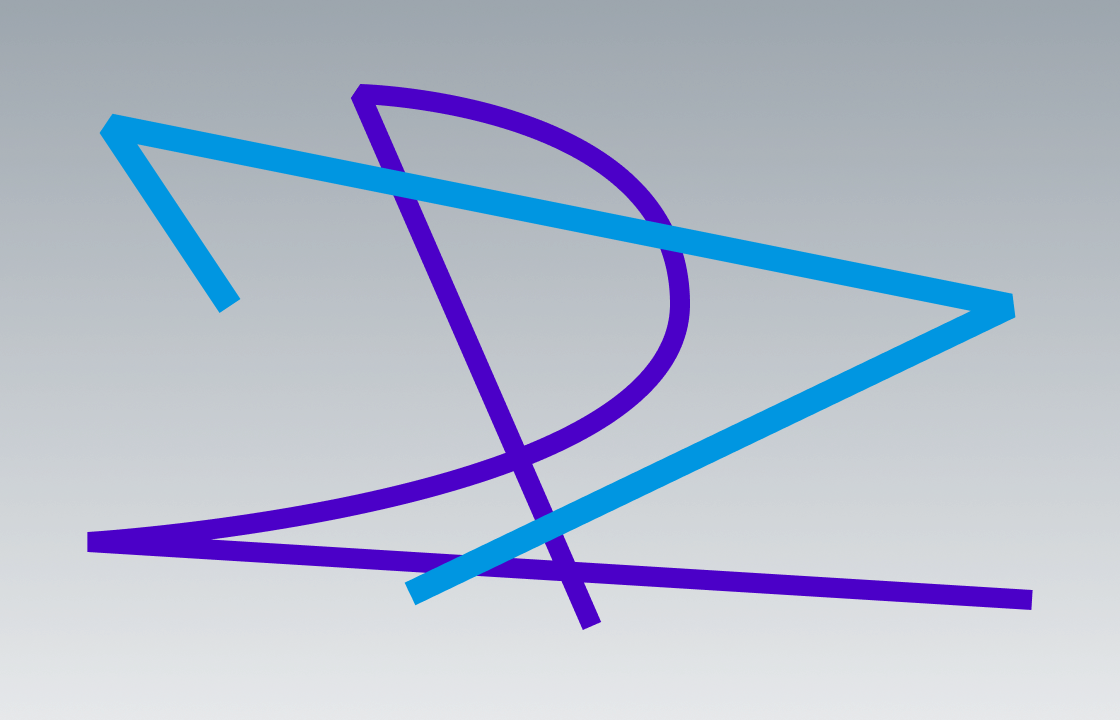
Path scripting
Scripting is useful for several path-related workflows. The following examples use methods such as GetControlPoints() which returns a table of Path2DControlPoints and GetPositionOnCurveArcLength() which returns the UDim2 position at a given t value along the spline.
Arrange UI Objects Across Path
local parent = script.Parent
local path = parent:FindFirstChildWhichIsA("Path2D")
local function arrangeChildren()
local segmentCount = #path:GetControlPoints()
local objectsToArrange = {}
for _, child in parent:GetChildren() do
if child:IsA("GuiObject") then
table.insert(objectsToArrange, child)
end
end
for idx, child in objectsToArrange do
local t = idx / (#objectsToArrange + 1)
child.Position = path:GetPositionOnCurveArcLength(t)
end
end
-- Initially arrange child UI objects across path
arrangeChildren()
-- Listen for children being added/removed to adjust arrangement
parent.ChildAdded:Connect(arrangeChildren)
parent.ChildRemoved:Connect(arrangeChildren)
Animate UI Object Along Path
local Tweenservice = game:GetService("TweenService")
local parent = script.Parent
local path = parent:FindFirstChildWhichIsA("Path2D")
local objectToAnimate = parent:FindFirstChildWhichIsA("GuiObject")
local TWEEN_DURATION = 4
local TWEEN_EASING_STYLE = Enum.EasingStyle.Cubic
local TWEEN_EASING_DIRECTION = Enum.EasingDirection.InOut
local pathSampleValue = Instance.new("NumberValue")
local tweenInfo = TweenInfo.new(TWEEN_DURATION, TWEEN_EASING_STYLE, TWEEN_EASING_DIRECTION, 0, true, 2)
local tween = Tweenservice:Create(pathSampleValue, tweenInfo, {Value = 1})
local function onSampleValueChanged()
objectToAnimate.Position = path:GetPositionOnCurveArcLength(pathSampleValue.Value)
end
pathSampleValue.Changed:Connect(onSampleValueChanged)
tween:Play()