ユーザーが 1 回以上購入することができるアイテムまたは能力は、 開発者製品 です。これには、エクスペリエンス中の通貨、弾薬、またはポーションなどが含まれます。
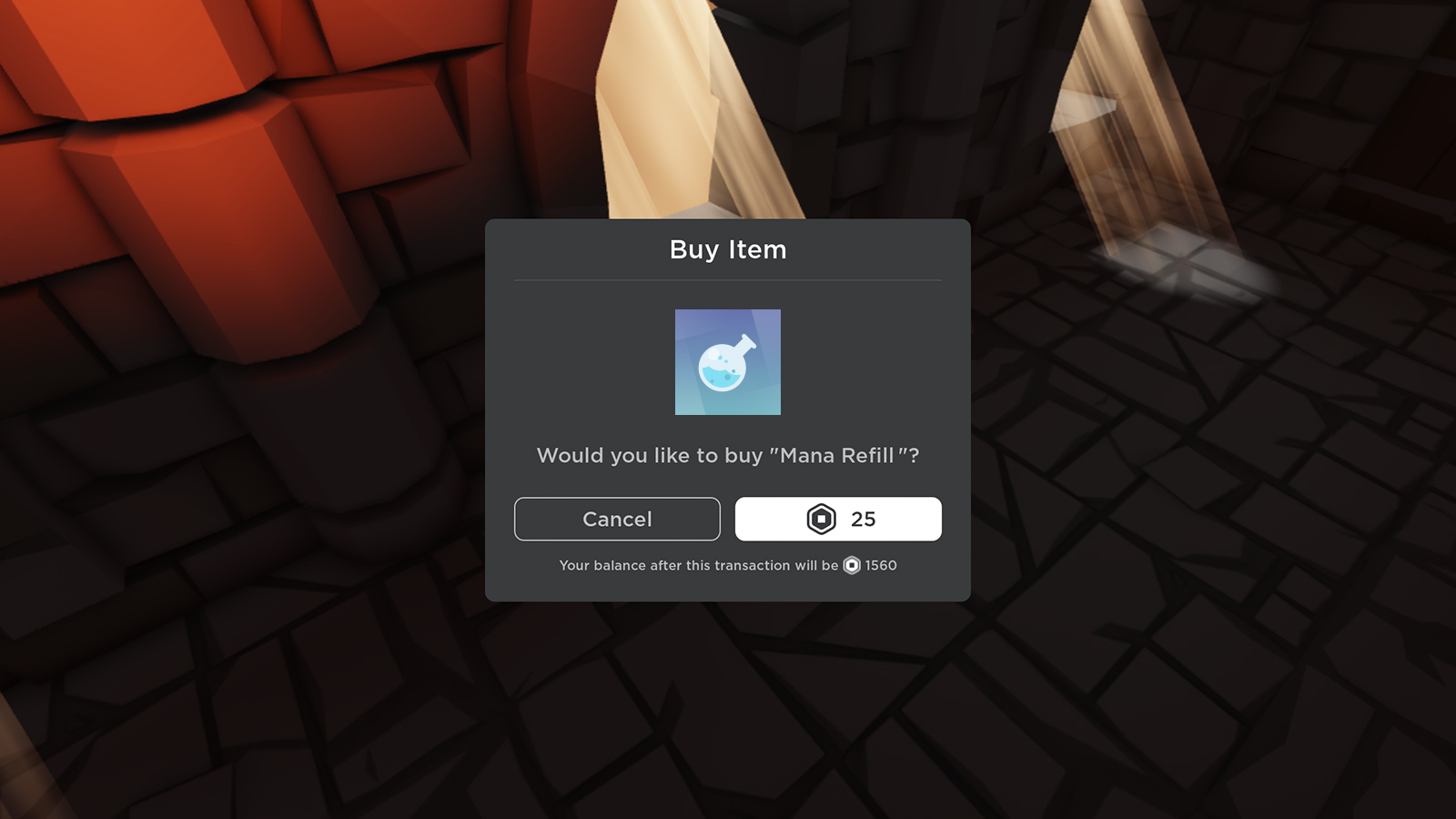
開発者製品の作成
開発者製品を作成するには:
- クリエーション に移動し、エクスペリエンスを選択します。
- Go to 収益化 > 開発者製品 .
- クリックします 開発者製品を作成 。
- 画像をアップロードして製品アイコンとして表示します。画像が 512x512 ピクセルを超えていないことを確認し、.jpg、 .jpg 、1>.png1> 、または 4>.bmp4> 形式の画像をアップロードしています。
- 製品に名前と説明を入力します。
- Robux の製品価格を設定します。最小価格は 1 Robux、最大価格は 10億 Robuxです。
- クリックします 開発者製品を作成 。
開発者製品ID を取得する
スクリプトを使用するには、開スクリプト作成製品 ID が必要です。製品 ID を取得するには:
Go to 収益化 > 開発者製品 .
製品の上にマウスポインタを置き、 ⋯ メニューをクリックします。
クリックして、 アセット ID をコピー をクリップボードにコピーします。
開発者製品の販売
エクスペリエンス内で開発者製品を実装および販売するには、MarketplaceService 機能を呼び出します。
Class.MarketplaceService:GetProductInfo()|GetProductInfo() を使用して、開発者製品の情報を取得するために、名前と価格などのデベロッパー製品の情報を取得します。Enum.InfoType.Product を使用すると、エクスペリエンスのマーケットプレイス内で製品を販売できます。たとえ
local MarketplaceService = game:GetService("MarketplaceService")
-- ディスプレースホルダー ID を開発者製品 ID に交換
local productId = 000000
local success, productInfo = pcall(function()
return MarketplaceService:GetProductInfo(productId, Enum.InfoType.Product)
end)
if success and productInfo then
-- 製品情報を表示
-- プリントステートメントを UI コードに置き換えて、製品を表示します
print("Developer Product Name: " .. productInfo.Name)
print("Price in Robux: " .. productInfo.PriceInRobux)
print("Description: " .. productInfo.Description)
end
Use GetDeveloperProductsAsync() を使用して、エクスペリエンスに関連付けられたすべての開発者製品を取得します。この関数は、Pages オブジェクトを返しますが、エクスペリエンスストアや製品リスト GUI などのビルドを構築するためにインス
local MarketplaceService = game:GetService("MarketplaceService")
local success, developerProducts = pcall(function()
return MarketplaceService:GetDeveloperProductsAsync()
end)
if success and developerProducts then
local firstPage = developerProducts:GetCurrentPage()
for _, developerProduct in firstPage do
-- プリントステートメントを UI コードに置き換えて、製品を表示します
print(field .. ": " .. value)
end
end
Class.MarketplaceService:PromptProductPurchase()|PromptProductPurchase() を使用して、エクスペリエンス内で製品購入を促すために。この関数は、ユーザーがボタンを押すなどのアクションを実行したときに呼び出されます。
local MarketplaceService = game:GetService("MarketplaceService")
local Players = game:GetService("Players")
local player = Players.LocalPlayer
-- ディスプレースホルダー ID を開発者製品 ID に交換
local productId = 000000
local function promptProductPurchase()
local success, errorMessage = pcall(function()
MarketplaceService:PromptProductPurchase(player, productId)
end)
if success then
print("Purchase prompt shown successfully")
end
end
Class.LocalScript|LocalScript 内の関数を組み合わせることもできます。たとえば、ボタンやベンダー NPC のような UI エレメントを作成し、GetProductInfo() を使用して、既存の開発者
local MarketplaceService = game:GetService("MarketplaceService")
local player = game.Players.LocalPlayer
local button = script.Parent
-- ディスプレースホルダー ID を開発者製品 ID に交換
local productId = 000000
-- ユーザーが UI ボタンをクリックしたときに製品情報を取得します
button.MouseButton1Click:Connect(function()
local success, productInfo = pcall(function()
return MarketplaceService:GetProductInfo(productId, Enum.InfoType.Product)
end)
if success and productInfo then
-- 製品が販売中されているかどうかをチェック
if productInfo.IsForSale then
print("This is for sale")
-- プロンプト製品購入
MarketplaceService:PromptProductPurchase(player, productId)
else
-- 製品が販売中されていないことを通知
print("This product is not currently for sale.")
end
else
print("Error retrieving product info: " .. tostring(productInfo))
end
end)
ハンドリング開発者製品購入
ユーザーが開発者製品を購入した後、あなたは取引を処理し、記録する必要があります。これをするには、Script 内の ServerScriptService を使用しています。ProcessReceipt() 機能を使用しています。
local MarketplaceService = game:GetService("MarketplaceService")
local Players = game:GetService("Players")
local productFunctions = {}
-- 例: product ID 123123 は、ユーザーを完全な健康に戻します
productFunctions[123123] = function(receipt, player)
local character = player.Character
local humanoid = character and character:FindFirstChildWhichIsA("Humanoid")
if humanoid then
humanoid.Health = humanoid.MaxHealth
-- 購入の成功を示します
return true
end
end
-- 例: 製品 ID 456456 は、ユーザーに 100 ゴールドコインを授与します
productFunctions[456456] = function(receipt, player)
local leaderstats = player:FindFirstChild("leaderstats")
local gold = leaderstats and leaderstats:FindFirstChild("Gold")
if gold then
gold.Value += 100
return true
end
end
local function processReceipt(receiptInfo)
local userId = receiptInfo.PlayerId
local productId = receiptInfo.ProductId
local player = Players:GetPlayerByUserId(userId)
if player then
-- 開発者製品 ID に関連するハンドラー機能を取得し、実行しようとします
local handler = productFunctions[productId]
local success, result = pcall(handler, receiptInfo, player)
if success then
-- ユーザーはアイテムを受け取りました
-- 「PurchaseGranted」を返して取引を確認します
return Enum.ProductPurchaseDecision.PurchaseGranted
else
warn("Failed to process receipt:", receiptInfo, result)
end
end
-- ユーザーのアイテムは獲得できませんでした
-- 「NotProcessedYet」が返され、次回ユーザーがエクスペリエンスに参加するときに再試行されます
return Enum.ProductPurchaseDecision.NotProcessedYet
end
-- コールバックを設定します
-- これは 1つのサーバー側スクリプトでのみできます
MarketplaceService.ProcessReceipt = processReceipt
開発者製品アナリティクス
開発者製品分析を使用して、個々の製品の成功を分析し、トレンドを識別し、将来の潜在的な収益を予測します。
アナリティクスを使用すると、次のことができます:
- 選択した期間のトップ開発者製品を表示します。
- 時系列グラフに最大 8つのトップセラーアイテムを表示し、全体的な収益と売上を分析します。
- カタログを監視し、売上と純収益でアイテムをソートします。
開発者製解析アナリティクスにアクセスするには:
- クリエーション に移動し、エクスペリエンスを選択します。
- Go to 収益化 > 開発者製品 .
- Select the アナリティクス tab.
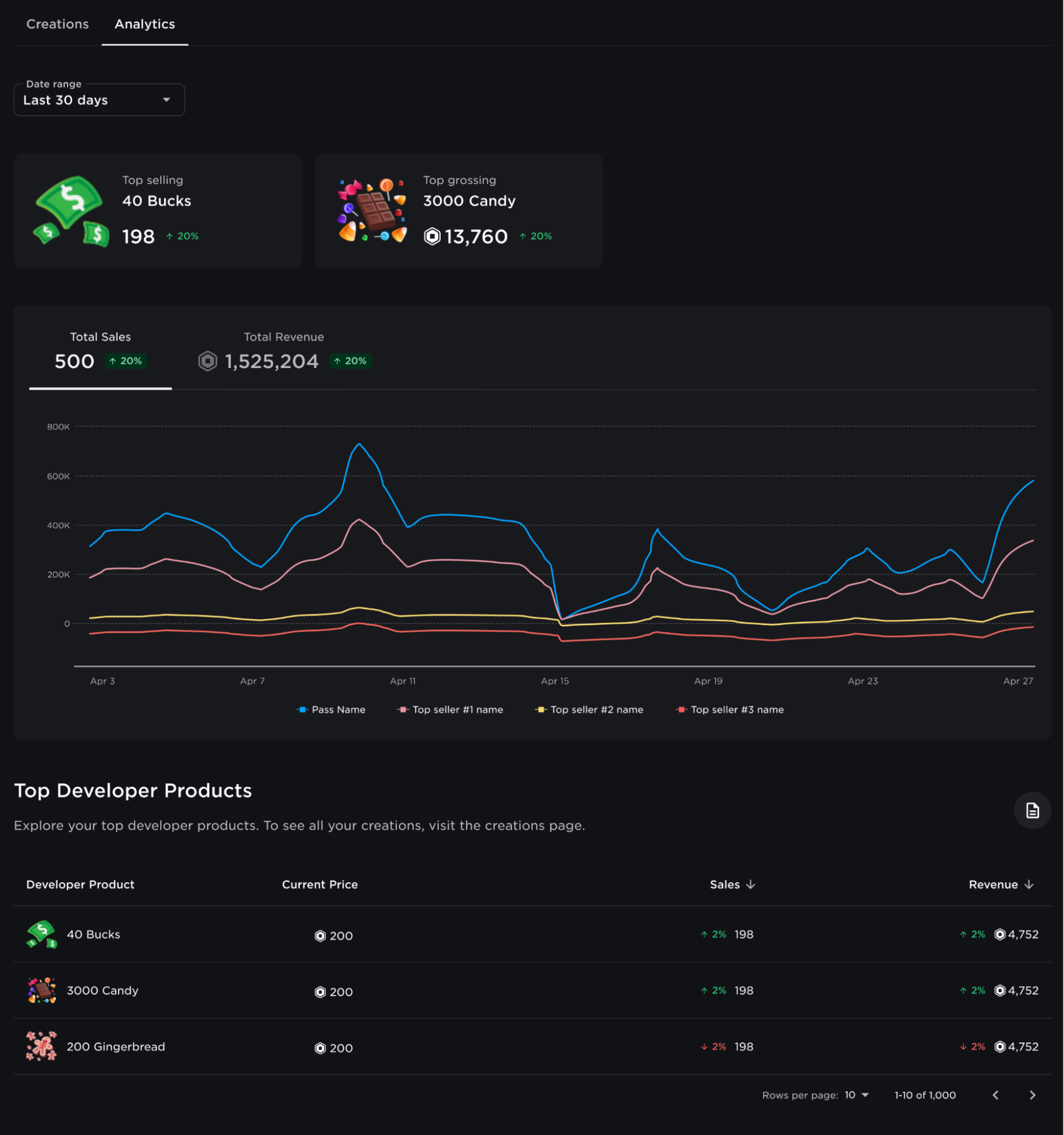