Puoi utilizzare le API di localizzazione per compiti di traduzione specializzati che non sono gestiti automaticamente dal aggiungere le traduzioni alla tabella di localizzazione. Roblox fornisce un LocalizationService per gestire tutte le esigenze di script di localizzazione. Usa il LocalizationService per le seguenti attività:
Se usi API di localizzazione quando traduci la tua esperienza, ascolta per qualsiasi cambiamento nel LocaleID dell'utente per reagire agli utenti che cambiano la lingua mentre in un'esperienza.
Quando si riutilizza il codice di traduzione, si dovrebbe utilizzare un TranslationHelperModuleScript per gestire gli errori e le traduzioni mancanti.
Localizzazione delle immagini e dei suoni
Aggiungi localizzazione oltre il testo nella tua esperienza fornendo uniche immagini e suoni in base alla posizione dell'utente. Per localizzare le risorse, prima aggiungi il 源 e target ID delle risorse all'esperienza's tabella di localizzazione e quindi usa l'API di localizzazione per ottenere le diverse risorse.
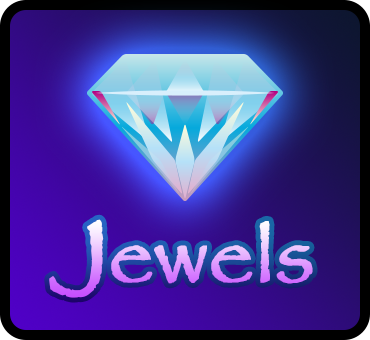
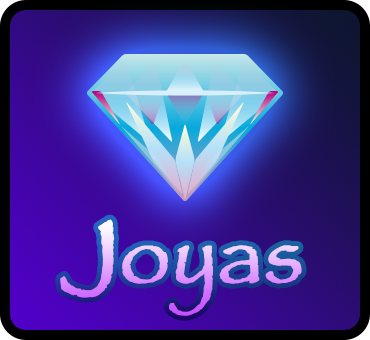
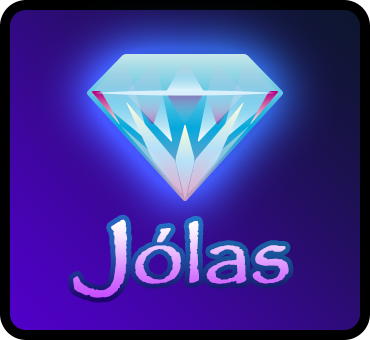
Per iniziare a localizzare immagini e suoni, aggiungi i tuoi 源 e target alla tua tavola di localizzazione. Gli ID risorsa e target sulla tavola di localizzazione devono includere un 0> Key0> come identificatore per essere chiamato dall'API.
Il seguente è un esempio di entry on a localization table using asset IDs:
Chiave | Fonte | es | pt |
---|---|---|---|
Key_JewelsImage | 2957093606 | 2957093671 | 2957093727 |
Il seguente codice sostituirà l'ID dell'asset di un ImageLabel con l'ID dell'asset fornito nella tabella di localizzazione:
local ReplicatedStorage = game:GetService("ReplicatedStorage")
local LocalizationService = game:GetService("LocalizationService")
-- Variabili locali
local localizedImageID
local localizedImage = Instance.new("ImageLabel")
-- Carica il traduttore per "es". Wrap la funzione all'interno di un pcall() per proteggere contro i guasti.
local res, translator = pcall(function()
return LocalizationService:GetTranslatorForLocaleAsync("es")
end)
if res then
-- Ottieni l'ID dell'asset dalla tabella di localizzazione facendo riferimento alla chiave
localizedImageID = translator:FormatByKey("Key_JewelsImage")
-- Imposta l'immagine
localizedImage.Image = "rbxassetid://" .. localizedImageID
else
print('GetTranslatorForPlayerAsync failed: ' .. translator)
end
Traduzione delle singole corde
In alcune situazioni, potresti voler target individuali per la traduzione. Translator:Translate() può recuperare singoli elementi sulla tabella di localizzazione in base alla Stringadi origine.
Nel prossimo esempio, viene utilizzata la seguente impostazione di localizzazione:
Fonte | es | es | pt |
---|---|---|---|
Schermo | Schermo | 295093671 | 2957093727 |
Il seguente script stamperà la traduzione spagnola di "Screen" nella finestra Output :
local ReplicatedStorage = game:GetService("ReplicatedStorage")
local LocalizationService = game:GetService("LocalizationService")
-- Carica il traduttore per "es". Wrap la funzione all'interno di un pcall() per proteggere contro i guasti.
local res, translator = pcall(function()
return LocalizationService:GetTranslatorForLocaleAsync("es")
end)
if res then
-- Usa la funzione traduci , fornendo contesto oggetto e Stringa
local sourceTranslation = translator:Translate(game, "Screen")
print(sourceTranslation) -- Output previsto: "Pantalla"
else
print('GetTranslatorForPlayerAsync failed: ' .. translator)
end
Uso di Context Overrides
Ci sono alcuni casi in cui la stessa stringa può avere più significati. Ad esempio, la parola "Screen" può indicare sia uno schermo del computer che uno schermo della finestra, ma le traduzioni spagnole sono completamente diverse.
La colonna Contesto della tabella di localizzazione è per specificare le traduzioni attraverso gli overrid del contesto.Specify the in-game object on your localization table come in the following example:
Contesto | Fonte | es |
---|---|---|
workspace.WindowScreen.SurfaceGui.TextLabel | Schermo | Mosquito |
Schermo | Schermo |
Il seguente script usa un'覆盖 del contesto per priorizzare una traduzione specifica:
local ReplicatedStorage = game:GetService("ReplicatedStorage")
local LocalizationService = game:GetService("LocalizationService")
-- Carica il traduttore per "es". Wrap la funzione all'interno di un pcall() per proteggere contro i guasti.
local res, translator = pcall(function()
return LocalizationService:GetTranslatorForLocaleAsync("es")
end)
if res then
-- utilizzare la funzione Traduci , fornendo contesto dell'oggetto e Stringa
local sourceTranslation = translator:Translate( workspace.WindowScreen.SurfaceGui.TextLabel, "Screen")
print(sourceTranslation) -- Output previsto: Mosquitero
else
print('GetTranslatorForPlayerAsync failed: ' .. translator)
end
Più contesti
Nel caso di più contesti, il servizio di localizzazione confronta le relazioni degli oggetti nel campo Context da destra a sinistra , utilizzando la Corrispondepiù vicina.
Ad esempio, una tabella di localizzazione nella tua esperienza potrebbe avere le seguenti entrade condivise delle stringhe di origine:
Contesto | Fonte | es |
---|---|---|
workspace.WindowScreen.SurfaceGui.TextLabel | Schermo | Mosquito |
playerGui.ScreenGui.TextButton | Schermo | Schermo |
Se la stringa "Screen" viene aggiunta a un oggetto playerGui.ScreenGui.TextLabel nella tua esperienza, il servizio di localizzazione mostra "Mosquitero" come traduzione spagnola come il contesto Corrispondevicino.
Sostituzione dei parametri
Quando si utilizzano i parametri per tradurre contenuto dinamico, imposta i valori in una tabella e passa la tabella come argomento attraverso l'API.
In questo esempio, l'esperienza ha una tabella di localizzazione con le seguenti entrade:
Chiave | Fonte | es |
---|---|---|
Premio_1 | gemme 1:int | gioie 1:int |
Premio_2 | $AmountCash:fixed contanti e NumJewels:int gioielli | $AmountCash:fixed denaro e NumJewels:int gioielli |
Usa il seguente esempio di codice per tradurre queste stringhe con valori di parametro:
local ReplicatedStorage = game:GetService("ReplicatedStorage")
local LocalizationService = game:GetService("LocalizationService")
-- Carica il traduttore per "es". Wrap la funzione all'interno di un pcall() per proteggere contro i guasti.
local res, translator = pcall(function()
return LocalizationService:GetTranslatorForLocaleAsync("es")
end)
if res then
-- Imposta il valore del parametro in "Key_Prize_1" su 100
local keyTranslation1 = translator:FormatByKey("Key_Prize_1", {100})
print(keyTranslation1) -- Output previsto: 100 joyas
-- Imposta più parametri su 500 e 100 per nome
local keyTranslation2 = translator:FormatByKey("Key_Prize_2", {AmountCash=500, NumJewels=100})
print(keyTranslation2) -- Ricompensa prevista: $ 500.00 denaro e 100 gioielli
else
print('GetTranslatorForPlayerAsync failed: ' .. translator)
end
Cambio di lingue
In alcuni casi, potresti voler visualizzare le traduzioni di altre lingue nella tua esperienza. Puoi impostare un nuovo traduttore con un codice di paese diverso usando LocalizationService:GetTranslatorForLocaleAsync() .
Il seguente codice di esempio imposta un traduttore con un codice paese manuale e un traduttore aggiuntivo basato sulle impostazioni globali dell'utente:
local ReplicatedStorage = game:GetService("ReplicatedStorage")
local LocalizationService = game:GetService("LocalizationService")
local Players = game:GetService("Players")
-- Variabili locali
local player = Players.LocalPlayer
-- Carica il traduttore per "pt". Wrap le funzioni del traduttore in un pcall() per proteggere contro i guasti.
local res1, translator = pcall(function()
return LocalizationService:GetTranslatorForLocaleAsync("pt")
end)
-- Carica il secondo traduttore con il linguaggio del giocatore, in questo esempio "es"
local res2, fallbackTranslator = pcall(function()
return LocalizationService:GetTranslatorForPlayerAsync(player)
end)
-- Usa la funzione Traduci con il primo traduttore
if res1 then
local translate1 = translator:Translate(game, "jewels")
print(translate1) -- Output previsto in pt: joyas
else
print('GetTranslatorForPlayerAsync failed: ' .. translator)
end
-- Usa la funzione di traduzione con il secondo traduttore
if res2 then
local translate2 = fallbackTranslator:Translate(game, "jewels")
print(translate2) -- Output Esperto in if utente è impostato su 'es': jóias
else
print('GetTranslatorForPlayerAsync failed: ' .. fallbackTranslator)
end
Reattare agli utenti che cambiano lingue
Gli utenti possono modificare le impostazioni linguistiche in qualsiasi momento usando il loro menu Impostazioni in-experience . Questa impostazione utente cambia automaticamente le risorse di localizzazione non scriptate, come le stringhe gestite dal traduzione automatico, ma potrebbe non aggiornare le modifiche alle localizzazioni scriptate che sono già state generate, come le immagini GUI o i suoni.
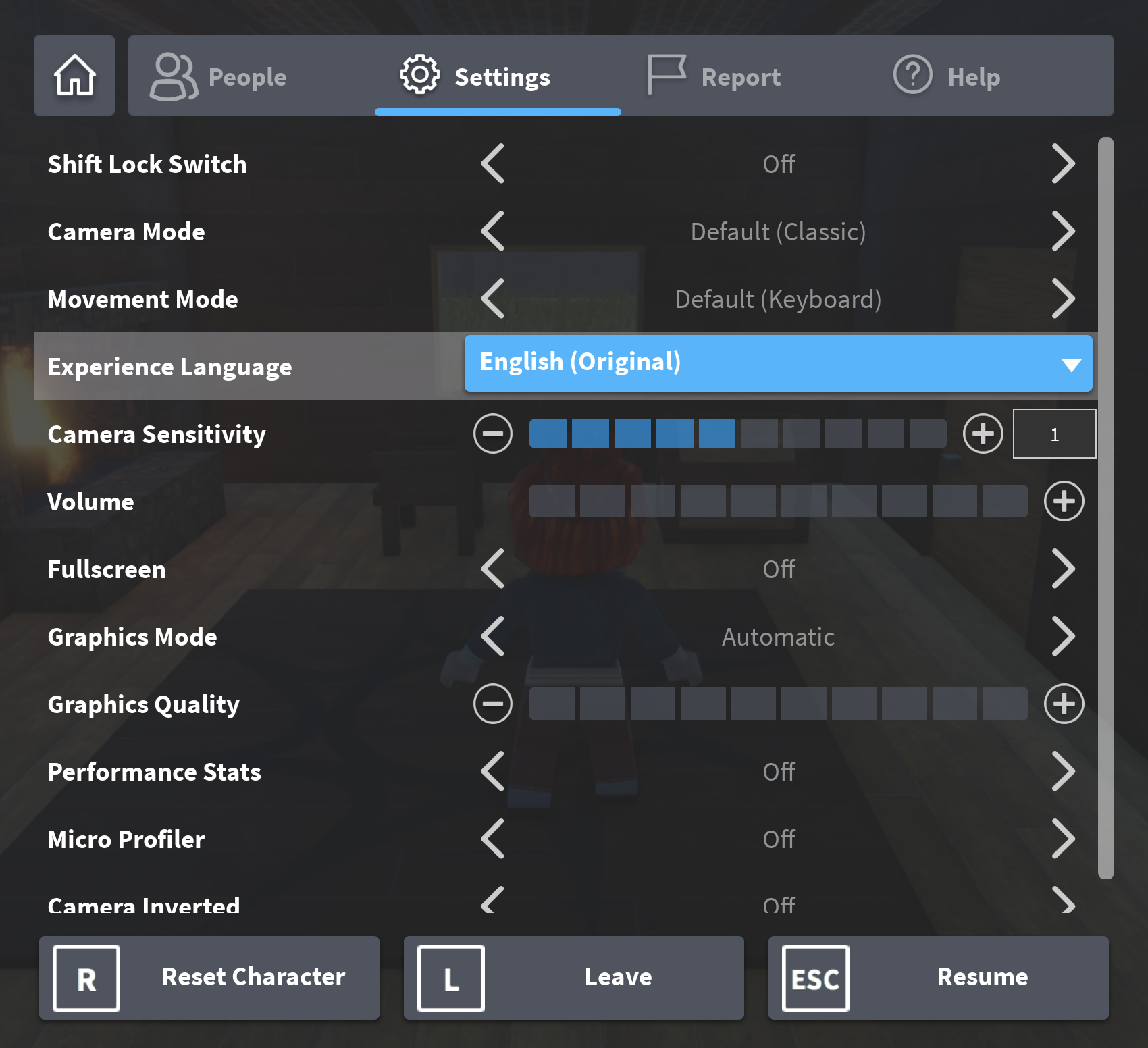
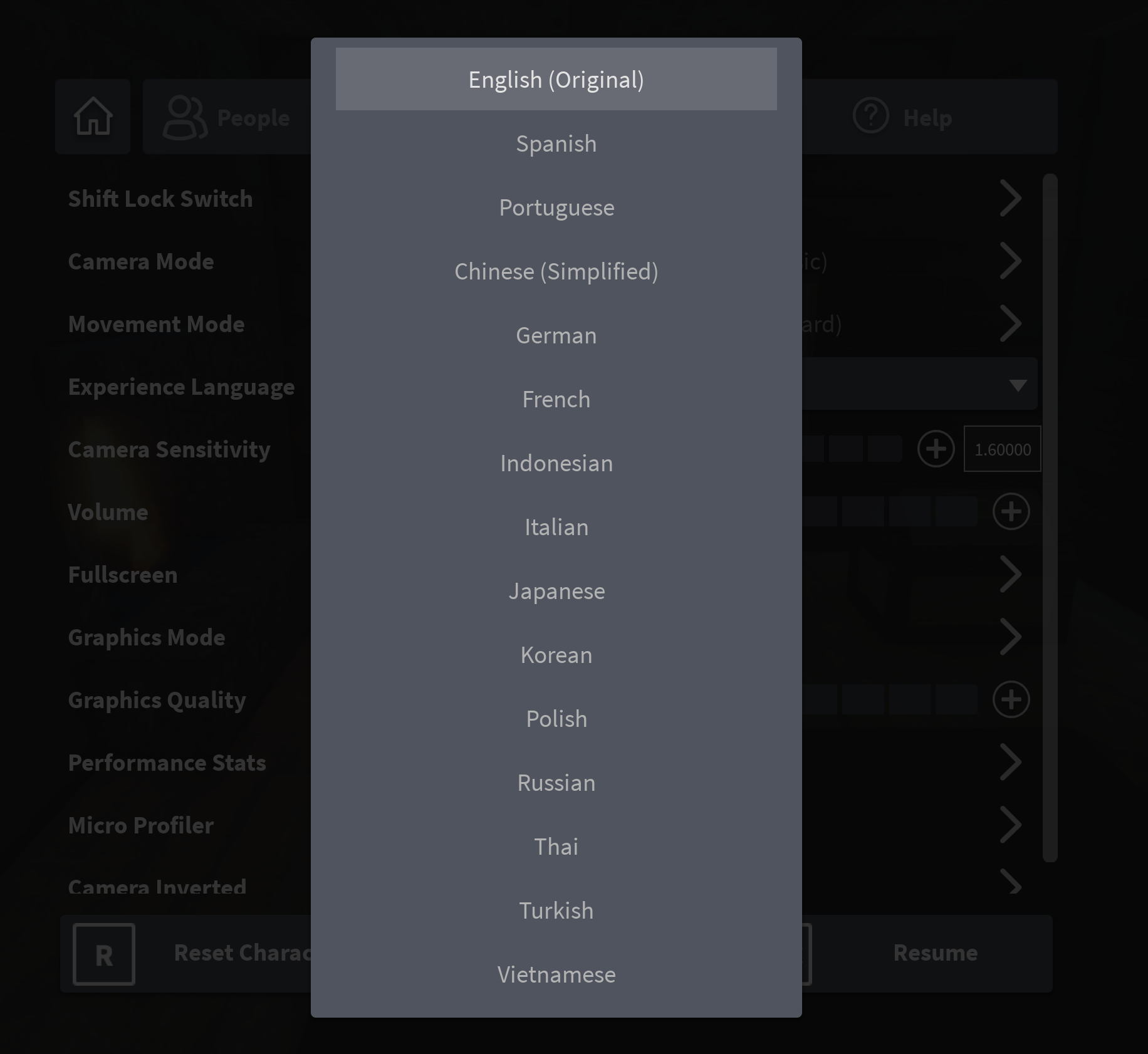
Per assicurarti che le tue risorse scriptate localizzate si aggiornino correttamente, ascolta l'evento GetPropertyChangedSignal per le modifiche nella proprietà LocaleID della istanza Translator restituita da
Il seguente esempio di codice stampa l'ID Locale dell'utente e l'ID Locale dell'istanza Translator per l'utente quando l'utente cambia lingue:
local LocalizationService = game:GetService("LocalizationService")
local Players = game:GetService("Players")
local player = Players.LocalPlayer
-- Se GetTranslatorForPlayerAsync ha successo, restituirà un traduttore per la lingua corrente del Giocatore
local res, translator = pcall(function()
return LocalizationService:GetTranslatorForPlayerAsync(player)
end)
-- Funzione che viene chiamata quando viene rilevato l'ID della lingua del Giocatore
local function OnLocaleIdChanged()
print("Translator has changed to: " .. translator.LocaleId)
-- Dovresti ri-tradurre qualsiasi risorsa tradotta con API di localizzazione nella nuova lingua del Giocatorequi
end
-- Controlla se GetTranslatorForPlayerAsync è riuscito
if res then
-- Se ci riesci, traduci le risorse qui usando il traduttore
-- Ascolta per un cambiamento nell'ID della lingua del Giocatore
translator:GetPropertyChangedSignal("LocaleId"):Connect(OnLocaleIdChanged)
else
print('GetTranslatorForPlayerAsync failed: ' .. translator)
end
Creazione di un Modulo di Traduzione
Quando carichi i traduttori in base alla lingua predefinita del Giocatore, potresti riutilizzare il codice. Per riutilizzare il codice, configura un aiutante ModuleScript che carica in sicurezza i traduttori in base alla lingua predefinita del Giocatoree include funzioni per fornire traduzioni specifiche e per passare da una lingua all'altra.
Il seguente codice di esempio implementa un TranslationHelper che puoi copiare nel tuo progetto come ModuleScript in ReplicatedStorage :
local TranslationHelper = {}
local LocalizationService = game:GetService("LocalizationService")
local Players = game:GetService("Players")
-- Variabili locali
local player = Players.LocalPlayer
local sourceLanguageCode = "en"
-- Ottieni traduttori
local playerTranslator, fallbackTranslator
local foundPlayerTranslator = pcall(function()
playerTranslator = LocalizationService:GetTranslatorForPlayerAsync(player)
end)
local foundFallbackTranslator = pcall(function()
fallbackTranslator = LocalizationService:GetTranslatorForLocaleAsync(sourceLanguageCode)
end)
-- Crea un metodo TranslationHelper.setLanguage per caricare una nuova traduzione per il TranslationHelper
function TranslationHelper.setLanguage(newLanguageCode)
if sourceLanguageCode ~= newLanguageCode then
local success, newPlayerTranslator = pcall(function()
return LocalizationService:GetTranslatorForLocaleAsync(newLanguageCode)
end)
--Sovrascrivi solo il playerTranslator attuale se il nuovo è valido (fallbackTranslator rimane come la lingua di origine dell'esperienza)
if success and newPlayerTranslator then
playerTranslator = newPlayerTranslator
return true
end
end
return false
end
-- Crea una funzione di traduzione che usa un traduttore di fallback se il primo non carica o non torna con successo. Puoi anche impostare l'oggetto di riferimento su un oggetto di gioco generico
function TranslationHelper.translate(text, object)
if not object then
object = game
end
if foundPlayerTranslator then
return playerTranslator:Translate(object, text)
end
if foundFallbackTranslator then
return fallbackTranslator:Translate(object, text)
end
return false
end
-- Crea una funzione di nome Fallback che usa un traduttore di fallback se il primo non carica o non ritorna con successo
function TranslationHelper.translateByKey(key, arguments)
local translation = ""
local foundTranslation = false
-- Prima cerca di tradurre per la lingua del Giocatore(se un traduttore è stato trovato)
if foundPlayerTranslator then
foundTranslation = pcall(function()
translation = playerTranslator:FormatByKey(key, arguments)
end)
end
if foundFallbackTranslator and not foundTranslation then
foundTranslation = pcall(function()
translation = fallbackTranslator:FormatByKey(key, arguments)
end)
end
if foundTranslation then
return translation
else
return false
end
end
return TranslationHelper
Una volta che il modulo è in ReplicatedStorage , richiedilo da un LocalScript per chiamare le funzioni del modulo. Il seguente codice usa la funzione di aiuto del modulo per tradurre una singola Stringa:
local ReplicatedStorage = game:GetService("ReplicatedStorage")-- Requires translation modulelocal TranslationHelper = require(ReplicatedStorage:WaitForChild("TranslationHelper"))-- Usa le funzioni fornite in TranslationHelperTranslationHelper.setLanguage("es")local sourceTranslation = TranslationHelper.translate("Screen")print(sourceTranslation) -- Expected Output in 'es': "Pantalla"