It can be challenging to locate friends in-experience. The FriendsLocator developer module lets players easily find and teleport to their friends inside a place.
Module usage
Installation
To use the FriendsLocator module in an experience:
Make sure the Models sorting is selected, then click the See All button for Categories.
Locate and click the Dev Modules tile.
Locate the Friends Locator module and click it, or drag-and-drop it into the 3D view.
In the Explorer window, move the entire FriendsLocator model into ServerScriptService. Upon running the experience, the module will distribute itself to various services and begin running.
Test in Studio
To test the module in Studio, the FriendsLocator module must be run in a multi-client simulation, since no friends will be present in a solo playtest.
In StarterPlayerScripts, create a new LocalScript and rename it ConfigureFriendsLocator.
Paste the following code into the new ConfigureFriendsLocator script. The showAllPlayers setting within the configure function ensures that locators are shown for all users while testing in Studio, but not in a published place.
LocalScript - ConfigureFriendsLocatorlocal RunService = game:GetService("RunService")local ReplicatedStorage = game:GetService("ReplicatedStorage")local FriendsLocator = require(ReplicatedStorage:WaitForChild("FriendsLocator"))FriendsLocator.configure({showAllPlayers = RunService:IsStudio(), -- Allows for debugging in Studio})From the Test tab, select the following combination for Clients and Servers, then click the Start button. Three new instances of Studio will open; one simulated server and two simulated clients.
Go into either of the client Studio instances, move a distance of 100 studs away from the other character, and you should see a locator icon appear over its head.
Connect to events
The FriendsLocator module exposes events so that you can introduce custom behaviors when users interact with a locator icon.
Make sure that you've created the ConfigureFriendsLocator script outlined in testing in Studio.
Add lines 8 and 11-13 to the script:
LocalScript - ConfigureFriendsLocatorlocal RunService = game:GetService("RunService")local ReplicatedStorage = game:GetService("ReplicatedStorage")local FriendsLocator = require(ReplicatedStorage:WaitForChild("FriendsLocator"))FriendsLocator.configure({showAllPlayers = RunService:IsStudio(), -- Allows for debugging in StudioteleportToFriend = false, -- Prevent teleport on icon click/tap})FriendsLocator.clicked:Connect(function(player, playerCFrame)print("You clicked on locator icon for", player.DisplayName)end)Conduct a multi-client test and click on another character's locator icon. Notice that your character does not teleport to that location, and the event triggers to allow for custom handling of icon clicks.
Custom locator UI
If the default style does not fit your experience, you can replace the default avatar portrait UI with your own UI.
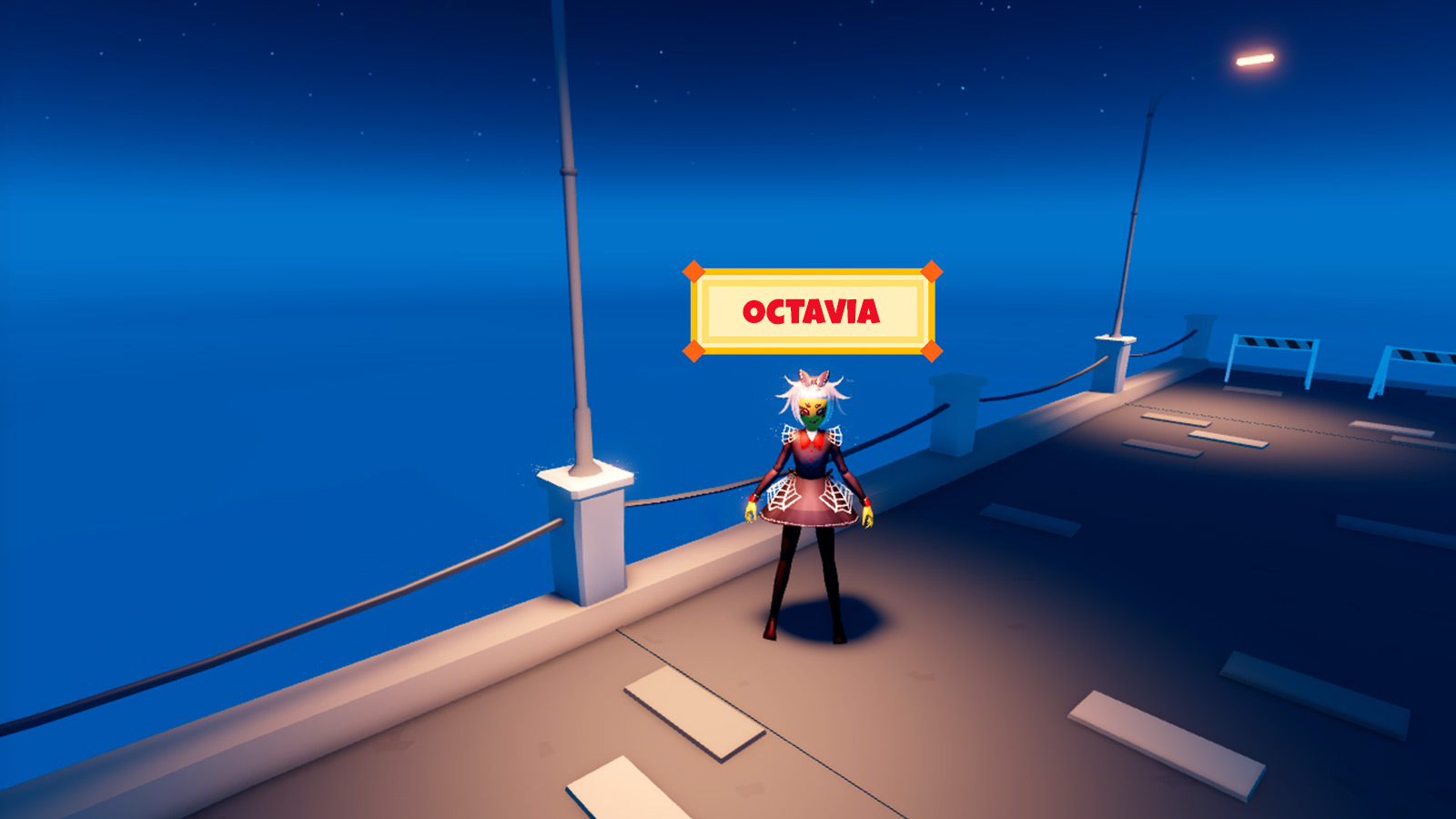
To replace the default UI:
Create a new ScreenGui instance inside the StarterGui container.
Create a Frame instance named FriendLocator as a child of the new ScreenGui, then add elements like ImageLabels, TextLabels to design your custom UI.
When finished, disable the parent ScreenGui so that the module doesn't show the custom locator UI until needed.
(Optional) If you want the friend's avatar portrait and DisplayName to show up somewhere in the custom UI, you can place the following instances inside the FriendLocator frame.
- An ImageLabel of the name Portrait.
- A TextLabel of the name DisplayName.
The module will look for these items and display the friend's avatar portrait and/or display name respectively.
API reference
Functions
configure
configure(config: table)
Overrides default configuration options through the following keys/values in the config table.
Key | Description | Default |
---|---|---|
alwaysOnTop | If true, shows locator icons on top of everything, preventing them from being blocked by 3D world objects. | true |
showAllPlayers | If true, shows locations for all players, not just friends; this can help verify the module's functionality in Studio. | false |
teleportToFriend | Teleports player character to the friend's location when their locator icon is clicked or tapped. | true |
thresholdDistance | Camera distance threshold at which locator icons appear; friends closer than this distance will not display icons. | 100 |
maxLocators | Maximum number of locator icons shown at any given time. | 10 |
LocalScript - ConfigureFriendsLocator
local ReplicatedStorage = game:GetService("ReplicatedStorage")local FriendsLocator = require(ReplicatedStorage:WaitForChild("FriendsLocator"))FriendsLocator.configure({alwaysOnTop = true,showAllPlayers = false,teleportToFriend = true,thresholdDistance = 100,maxLocators = 10})
Events
clicked
Fires when a locator icon is clicked/activated by the local player. This event can only be connected in a LocalScript.
Parameters | |
---|---|
player: Player | Player that the locator icon belongs to. |
playerCFrame: CFrame | CFrame of the player's Humanoid.RootPart that the locator icon belongs to. |
LocalScript
local ReplicatedStorage = game:GetService("ReplicatedStorage")
local Players = game:GetService("Players")
local Workspace = game:GetService("Workspace")
local FriendsLocator = require(ReplicatedStorage:WaitForChild("FriendsLocator"))
local localPlayer = Players.LocalPlayer
FriendsLocator.clicked:Connect(function(player, playerCFrame)
-- Request streaming around target location
if Workspace.StreamingEnabled then
local success, errorMessage = pcall(function()
localPlayer:RequestStreamAroundAsync(playerCFrame.Position)
end)
if not success then
print(errorMessage)
end
end
print("You clicked on locator icon for", player.DisplayName, "at position", playerCFrame.Position)
end)
visibilityChanged
Fires when a locator icon is shown/hidden on the local player's screen. This event can only be connected in a LocalScript.
Parameters | |
---|---|
player: Player | Player object that the locator icon belongs to. |
playerCFrame: CFrame | CFrame of the player's Humanoid.RootPart that the locator icon belongs to. |
isVisible: boolean | Whether the locator icon is currently visible on the local player's screen. Note that this will still be true if alwaysOnTop is false and the locator renders behind an object in the 3D world. |
LocalScript
local ReplicatedStorage = game:GetService("ReplicatedStorage")
local FriendsLocator = require(ReplicatedStorage:WaitForChild("FriendsLocator"))
FriendsLocator.visibilityChanged:Connect(function(player, playerCFrame, isVisible)
print("Visibility of locator icon for", player.DisplayName, ":", isVisible)
end)