Emotes are a core component of any social experience. The EmoteBar developer module aims to provide players an accessible, customizable way to facilitate meaningful social interaction.
Module Usage
Installation
To use the EmoteBar module in an experience:
Make sure the Models sorting is selected, then click the See All button for Categories.
Locate and click the Dev Modules tile.
Locate the Emote Bar module and click it, or drag-and-drop it into the 3D view.
In the Explorer window, move the entire EmoteBar model into ServerScriptService. Upon running the experience, the module will distribute itself to various services and begin running.
Configuration
The module is preconfigured with 7 emotes and it can be easily customized with your own emotes and display options. Additionally, if the player owns any emotes from previous Roblox events such as Lil Nas X, Royal Blood, or Twenty One Pilots, those emotes will be automatically added to the list of available emotes.
In ServerScriptService, create a new Script and rename it ConfigureEmotes.
Paste the following code into the new ConfigureEmotes script. The useDefaultEmotes setting of false overrides the default emotes and lets you define custom emotes via the setEmotes function.
Script - ConfigureEmoteslocal ReplicatedStorage = game:GetService("ReplicatedStorage")local EmoteBar = require(ReplicatedStorage:WaitForChild("EmoteBar"))EmoteBar.configureServer({useDefaultEmotes = false,})EmoteBar.setEmotes({{name = "Hello",animation = "rbxassetid://3344650532",image = "rbxassetid://7719817462",defaultTempo = 1,},{name = "Applaud",animation = "rbxassetid://5915693819",image = "rbxassetid://7720292217",defaultTempo = 2,},})
Mega Emotes
A mega emote is formed when multiple players in the same area perform the same emote at the same time. As more and more players join in, the mega emote grows larger. As players stop performing the emote, the mega emote grows smaller until eventually it disappears.
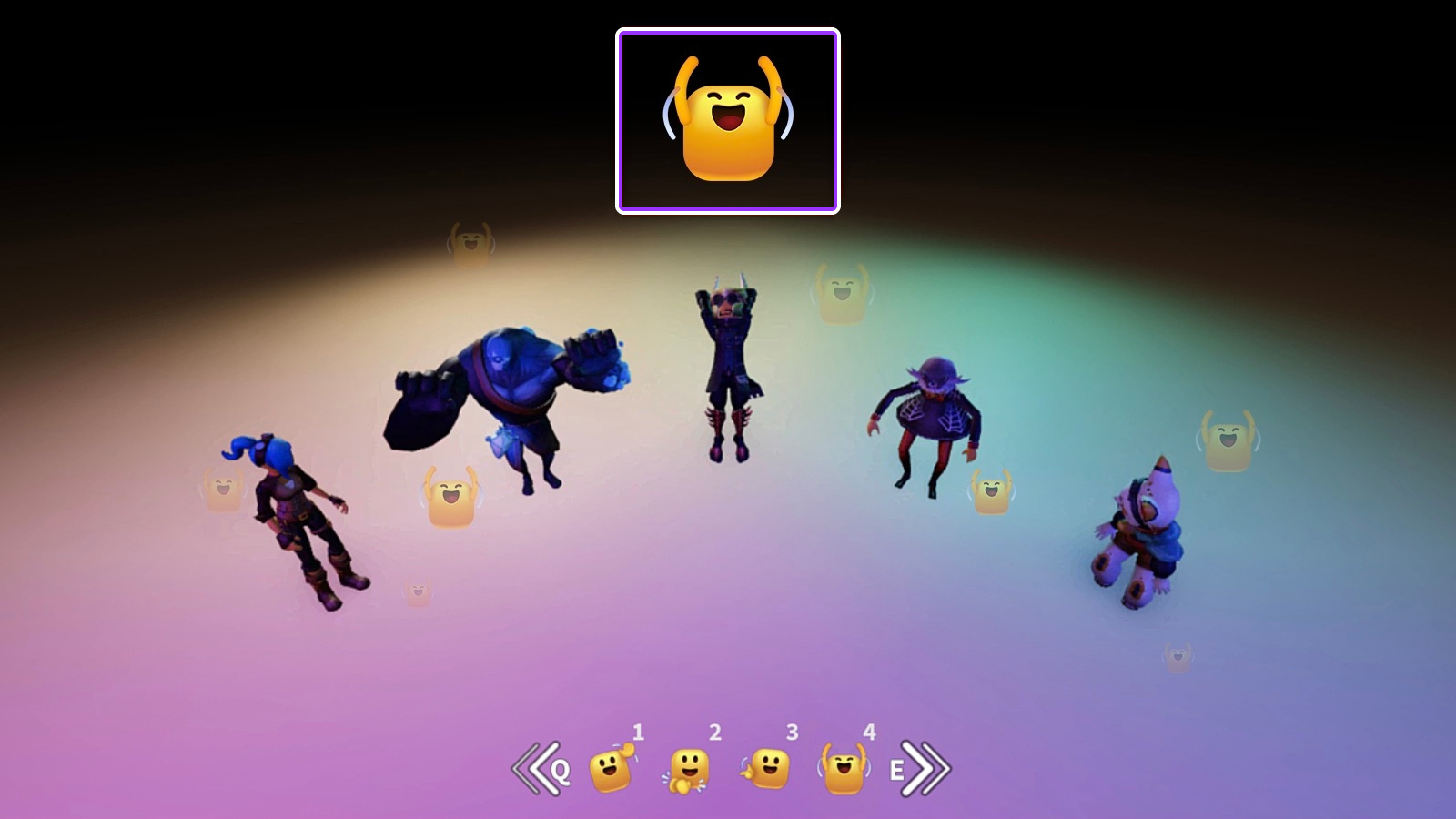
Tempo
An emote's tempo is the speed at which it plays when its button is tapped once. The default speed of an emote is determined by its defaultTempo. An emote's speed can be increased or decreased by tapping its button faster or slower.
API Reference
Types
Emote
Each emote is represented by a dictionary with the following key-value pairs:
Key | Type | Description |
---|---|---|
name | string | Emote name, for example "Shrug". |
animation | string | Asset ID for the emote's animation. |
image | string | Asset ID for the emote's image in the GUI. |
defaultTempo | number | Default speed factor at which to play the emote animation. For example, a tempo of 2 will play the animation at twice its normal speed. Must be greater than 0. |
isLocked | bool | Whether the emote is "locked" from being activated. |
Enums
EmoteBar.GuiType
Name | Summary |
---|---|
EmoteBar | Default form where emotes are displayed in a bar along the bottom of the screen, separated into individual "pages." |
EmoteWheel | Variant where emotes are displayed in a ring when a player clicks or taps on their player character. |
LocalScript
local ReplicatedStorage = game:GetService("ReplicatedStorage")local EmoteBar = require(ReplicatedStorage:WaitForChild("EmoteBar"))EmoteBar.configureClient({guiType = EmoteBar.GuiType.EmoteWheel,})
Functions
configureServer
configureServer(config: table)
Overrides default server-side configuration options through the following keys/values in the config table. This function can only be called from a Script and changes will automatically replicate to all clients.
Key | Description | Default |
---|---|---|
useDefaultEmotes | Whether the provided default emotes are included or not. | true |
useMegaEmotes | Enables or disables the mega emotes feature. | true |
emoteMinPlayers | Minimum number of players performing the same emote to contribute to a mega emote. | 3 |
emoteMaxPlayers | Maximum number of players performing the same emote to contribute to a mega emote. | 50 |
playParticles | Enables or disables the emotes players are playing as floating particles above their heads. | true |
sendContributingEmotes | Enables or disables sending a small emote icon to contribute to the mega emote. | true |
Script
local ReplicatedStorage = game:GetService("ReplicatedStorage")local EmoteBar = require(ReplicatedStorage:WaitForChild("EmoteBar"))EmoteBar.configureServer({emoteMinPlayers = 2,playParticles = false,})
configureClient
configureClient(config: table)
Overrides default client-side configuration options through the following keys/values in the config table. This function can only be called from a LocalScript. Depending on the value of guiType, options in the noted tabs also apply.
Key | Description | Default |
---|---|---|
guiType | Controls which form the GUI will take for displaying emotes (EmoteBar.GuiType). | EmoteBar |
useTempo | Enables or disables the tempo feature where users are able to control how fast or slow their emotes are played by repeatedly activating the same emote rhythmically. | true |
tempoActivationWindow | Amount of time, in seconds, the user has between sequential activations of an emote for it to count as part of the tempo. | 3 |
lockedImage | Image to display overtop locked emotes. | "rbxassetid://6905802778" |
LocalScript - Emote Bar
local ReplicatedStorage = game:GetService("ReplicatedStorage")local EmoteBar = require(ReplicatedStorage:WaitForChild("EmoteBar"))EmoteBar.configureClient({guiType = EmoteBar.GuiType.EmoteBar,maxEmotesPerPage = 6,nextPageKey = Enum.KeyCode.Z,prevPageKey = Enum.KeyCode.C,})
LocalScript - Emote Wheel
local ReplicatedStorage = game:GetService("ReplicatedStorage")local EmoteBar = require(ReplicatedStorage:WaitForChild("EmoteBar"))EmoteBar.configureClient({guiType = EmoteBar.GuiType.EmoteWheel,})
setEmotes
setEmotes(emotes: table)
Sets the custom emotes to use. These will be added to the defaults if useDefaultEmotes is true, or replace the defaults if useDefaultEmotes is false. This function can only be called from a Script and changes will automatically replicate to all clients.
See Emote for the structure of each emote passed to this function.
Script - ConfigureEmotes
local ReplicatedStorage = game:GetService("ReplicatedStorage")local EmoteBar = require(ReplicatedStorage:WaitForChild("EmoteBar"))EmoteBar.configureServer({useDefaultEmotes = false,})EmoteBar.setEmotes({{name = "Hello",animation = "rbxassetid://3344650532",image = "rbxassetid://7719817462",defaultTempo = 1,},{name = "Applaud",animation = "rbxassetid://5915693819",image = "rbxassetid://7720292217",defaultTempo = 2,},})
setGuiVisibility
setGuiVisibility(visible: boolean)
Shows or hides the emotes GUI. This function can only be called from a LocalScript on a specific client.
LocalScript
local ReplicatedStorage = game:GetService("ReplicatedStorage")local EmoteBar = require(ReplicatedStorage:WaitForChild("EmoteBar"))EmoteBar.setGuiVisibility(false)
getEmote
Gets an Emote by name. Returns nil if the emote cannot be found. This function can only be called from a LocalScript on a specific client.
LocalScript
local ReplicatedStorage = game:GetService("ReplicatedStorage")local EmoteBar = require(ReplicatedStorage:WaitForChild("EmoteBar"))local shrug = EmoteBar.getEmote("Shrug")
playEmote
playEmote(emote: Emote)
Plays the given Emote and fires the emotePlayed event on the server, if connected. This function can only be called from a LocalScript on a specific client.
LocalScript
local ReplicatedStorage = game:GetService("ReplicatedStorage")local EmoteBar = require(ReplicatedStorage:WaitForChild("EmoteBar"))local shrug = EmoteBar.getEmote("Shrug")EmoteBar.playEmote(shrug)
lockEmote
lockEmote(emoteName: string)
Locks the Emote with the given name. This function can only be called from a LocalScript on the client.
LocalScript
local ReplicatedStorage = game:GetService("ReplicatedStorage")local EmoteBar = require(ReplicatedStorage:WaitForChild("EmoteBar"))EmoteBar.lockEmote("Applaud")
unlockEmote
unlockEmote(emoteName: string)
Unlocks the Emote with the given name. This function can only be called from a LocalScript on the client.
LocalScript
local ReplicatedStorage = game:GetService("ReplicatedStorage")local EmoteBar = require(ReplicatedStorage:WaitForChild("EmoteBar"))EmoteBar.unlockEmote("Applaud")
Events
emotePlayed
Fires when any client plays an emote. This event can only be connected in a LocalScript.
LocalScript
local ReplicatedStorage = game:GetService("ReplicatedStorage")
local EmoteBar = require(ReplicatedStorage:WaitForChild("EmoteBar"))
EmoteBar.emotePlayed:Connect(function(player, emote)
print(player.Name, "played", emote.name)
end)
lockedEmoteActivated
Fires when a client clicks a locked emote. This event can only be connected in a LocalScript.
Parameters | |
---|---|
emote: Emote | Locked emote which was activated. |
LocalScript
local ReplicatedStorage = game:GetService("ReplicatedStorage")
local Players = game:GetService("Players")
local EmoteBar = require(ReplicatedStorage:WaitForChild("EmoteBar"))
EmoteBar.lockedEmoteActivated:Connect(function(emote)
print(Players.LocalPlayer, "clicked", emote.name)
end)