Unless UI objects are under control of a layout structure or a size modifier/constraint, you have complete control over their position and size. You can also set the Z‑index layering order in which objects overlap.
Core Properties
All GuiObjects share a core set of properties to position, size, anchor, and layer them within an on‑screen or in‑experience container.
Position
The Position property is a UDim2 coordinate set that positions the object along the X and Y axes. A UDim2 is represented by both Scale and Offset values for each axis:

To edit the position of a selected GuiObject, click the Position field in the Properties window and enter a new UDim2 coordinate set.
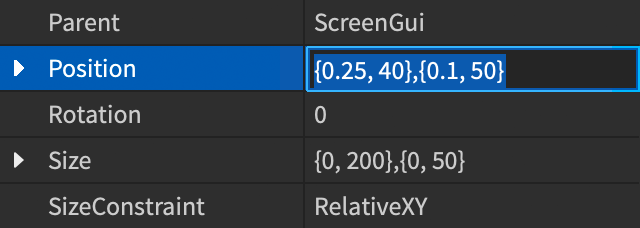

Size
The Size property is a UDim2 coordinate set that sizes the object along the X and Y axes. A UDim2 is represented by both Scale and Offset values for each axis:
- Scale — Values that represent a percentage of the container's size along the corresponding axis, additive to any Offset values.
- Offset — Values that represent the object's pixel size along the corresponding axis, additive to any Scale values.
To edit the size of a selected GuiObject, click the Size field in the Properties window and enter a new UDim2 coordinate set.
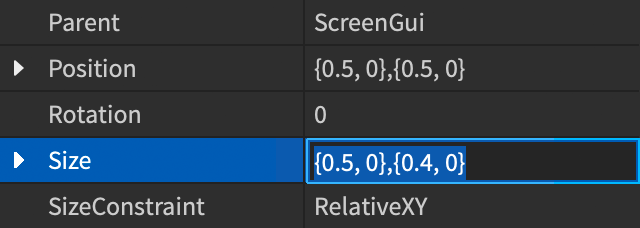

AnchorPoint
The AnchorPoint property defines the origin point from where the object changes position and size. The default AnchorPoint values are (0, 0) which places the anchor in the top‑left corner of the object.
AnchorPoint values are a fraction from 0 to 1, relative to the size of the object, meaning an object with AnchorPoint values of (0.5, 0.5) places the anchor point halfway (50%) through the object both horizontally and vertically, and any changes to its position or size both move and scale outward from this point.


To view and edit the anchor point of a selected GuiObject:
In the Properties window, click inside the AnchorPoint field.
Enter a new Vector2 coordinate and press Enter.
ZIndex
The ZIndex property defines the layer order in which GuiObjects render and overlap each other. If you want to create new rendering layers, you must set the ZIndex property to different positive or negative integer values for each object.
For UI containers like ScreenGui, the default ZIndexBehavior always renders children above their parents, and each child's ZIndex is used to decide the order in which it renders over others.
To edit an object's ZIndex, locate ZIndex in the Properties window and enter a new integer value.

Layout Structures
Layout structures let you quickly organize and display GuiObjects, for example into a horizontal or vertical list, a grid of equally‑sized tiles, a page sequence, and more. Layouts typically override or influence the position/size of objects under their control.
Layout | Description |
---|---|
List | UIListLayout positions sibling GuiObjects into horizontal rows or vertical columns within their parent container. |
Grid | UIGridLayout positions sibling GuiObjects in a grid of uniform cells of the same size within their parent container. |
Table | UITableLayout positions sibling GuiObjects and their children into table format. |
Page | UIPageLayout organizes its sibling GuiObjects into unique pages that you can transition to through scripting. |
Cross-Platform Factors
Roblox is inherently cross-platform, as players can discover and join experiences on a PC or console, then later pick up their phone and continue where they left off. You should design your Roblox experiences to be accessible and enjoyable on all platforms that you choose to support, instead of optimizing for one platform and neglecting others.
Reserved Zones
On mobile devices, the default controls occupy a portion of the bottom-left and bottom-right corners of the screen. When you design an experience's UI, avoid placing important info or virtual buttons in these zones.
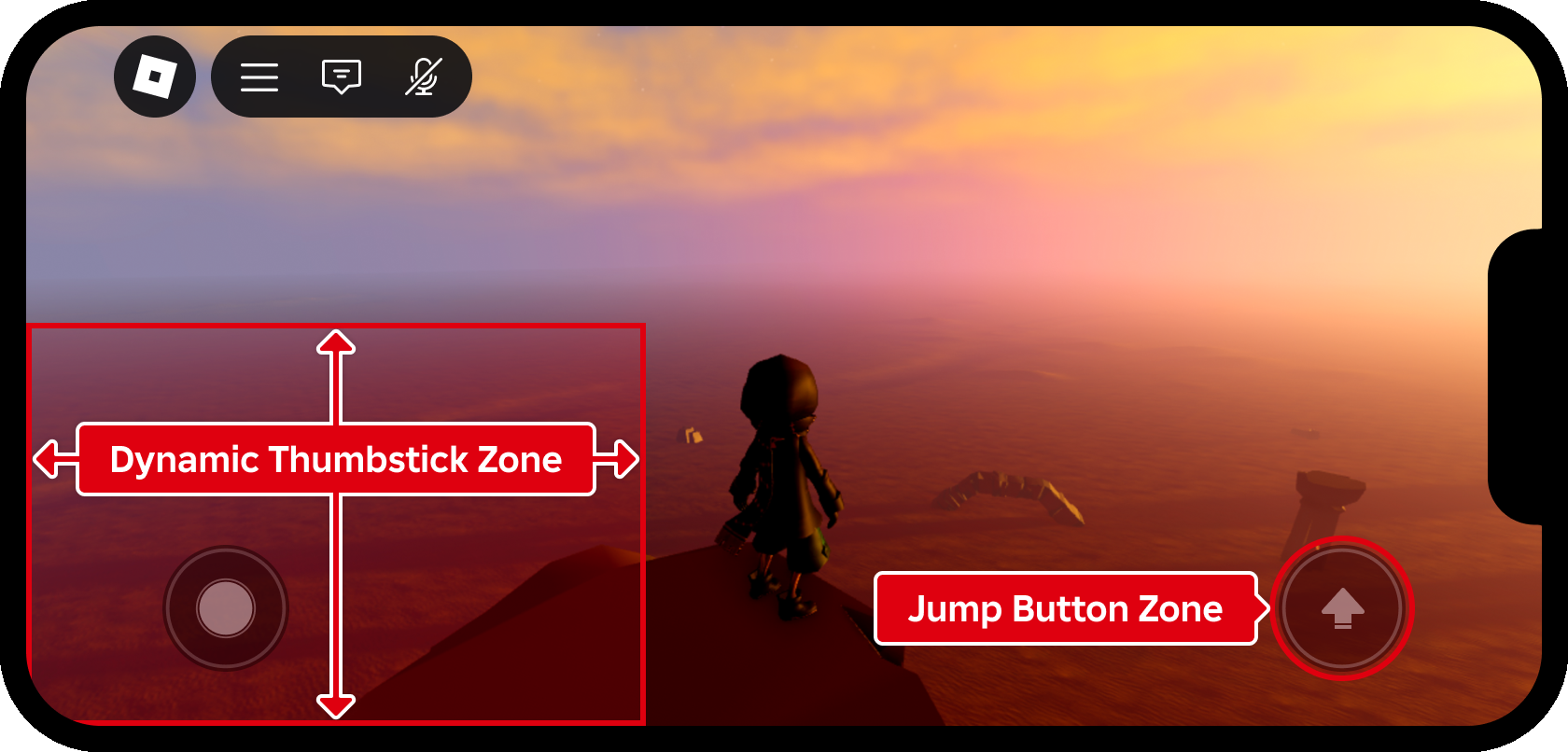
Thumb Zones
Most mobile players use two thumbs — one on the virtual thumbstick and one on the jump button. Depending on the physical size of the device and the player's hands, reaching too far from the bottom corners becomes uncomfortable or impossible, so you should avoid placing frequently‑used buttons outside of easy‑to‑reach zones.
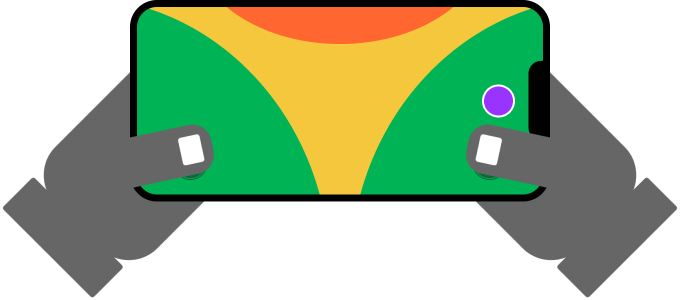
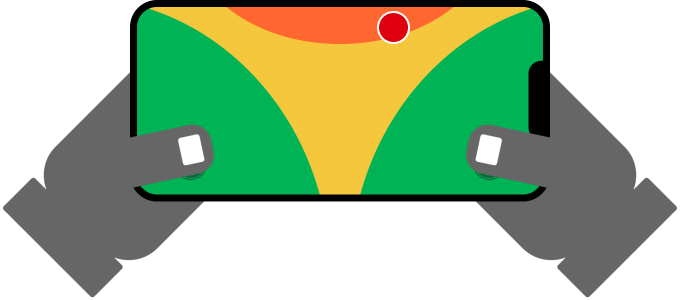
Remember that comfortable thumb zones differ between phones and tablets because tablets have a larger screen. A button placed 40% below the screen's top edge is reachable on a phone but almost unreachable on a tablet.
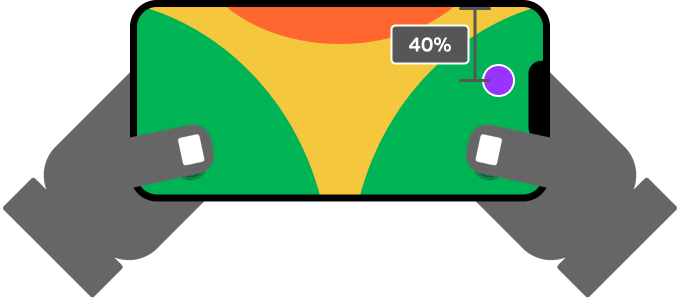
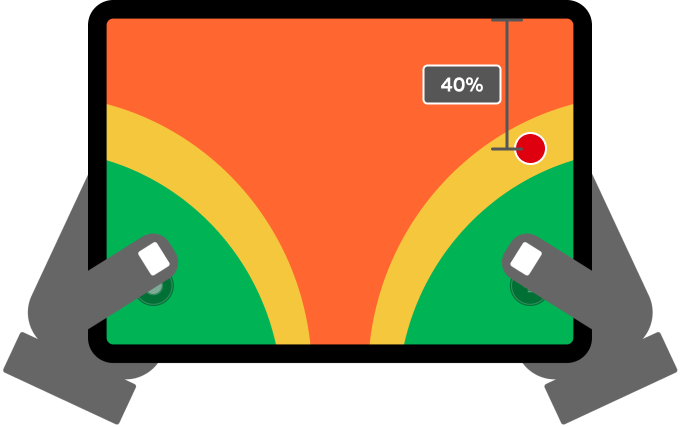
A reliable approach on both phones and tablets is relative positioning of custom buttons near frequently‑used controls like the default jump button, placing them within easy reach.
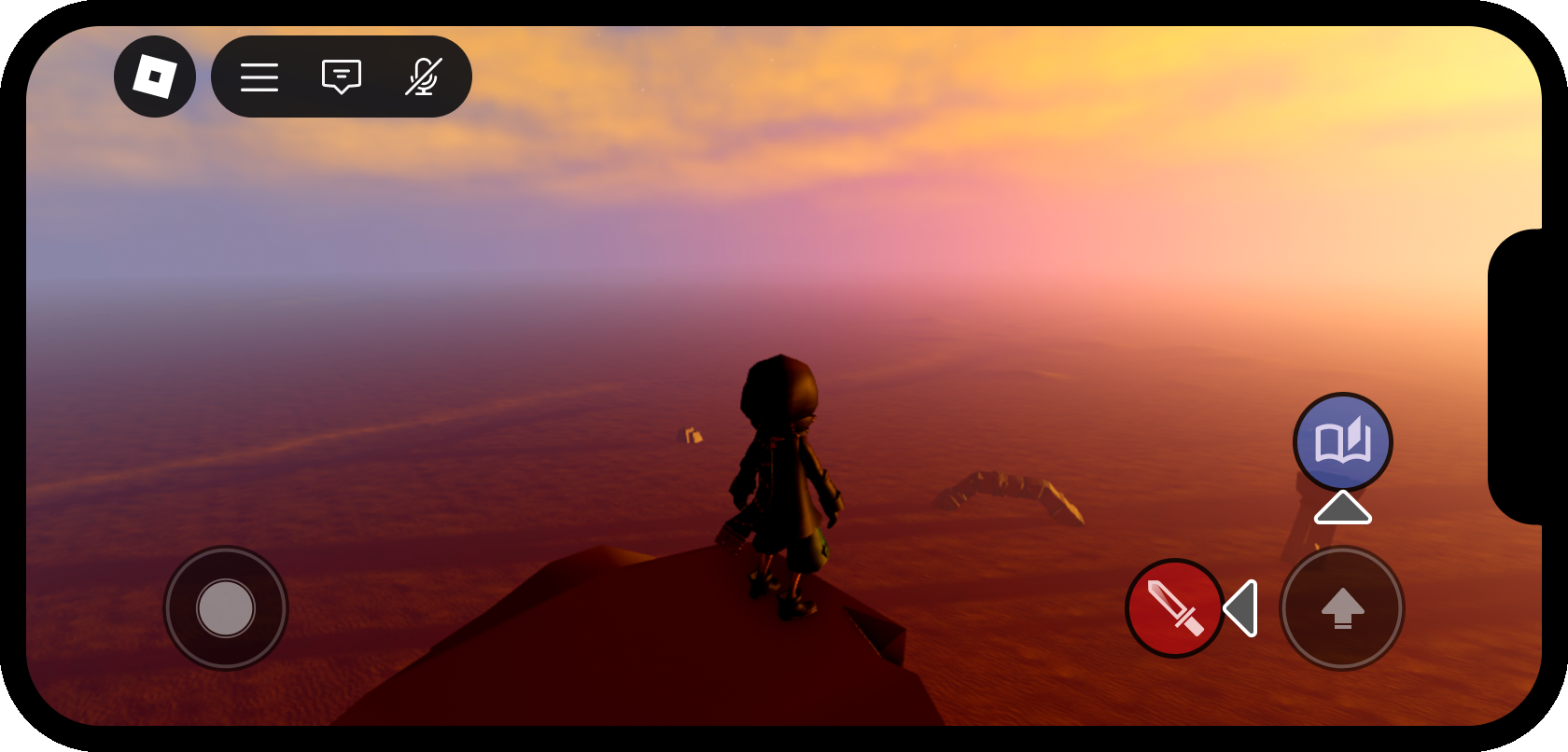
The following code, placed in a client-side script within StarterPlayerScripts, fetches the position of the jump button and creates a placeholder button 20 pixels to its left.
Client Script - Custom Button Near Jump Button
local Players = game:GetService("Players")local UserInputService = game:GetService("UserInputService")local player = Players.LocalPlayerlocal playerGui = player:WaitForChild("PlayerGui")if UserInputService.TouchEnabled then-- Wait for jump button to be fully loadedwhile not (playerGui:FindFirstChild("JumpButton", true) and playerGui:FindFirstChild("JumpButton", true).IsLoaded) dotask.wait()endlocal jumpButton = playerGui:FindFirstChild("JumpButton", true)-- Place new custom button to left of jump buttonlocal customButton = Instance.new("ImageButton")customButton.AnchorPoint = Vector2.new(1, 1)customButton.Size = UDim2.fromOffset(jumpButton.Size.X.Offset * 0.8, jumpButton.Size.Y.Offset * 0.8)customButton.Position = jumpButton.Position + UDim2.fromOffset(-20, jumpButton.Size.Y.Offset)customButton.Parent = jumpButton.Parentelsewarn("Device is not touch-enabled or Studio is not emulating a touch-enabled device!")end
Context-Based UI
Screen space is limited on mobile devices, so you should show only the most vital information during active gameplay. For example, if your experience includes a special input action to open doors and treasure chests, it doesn't make sense to constantly show an "open" button on the screen. Instead, use a proximity prompt or similar method to accept input only when the character approaches a door or chest.
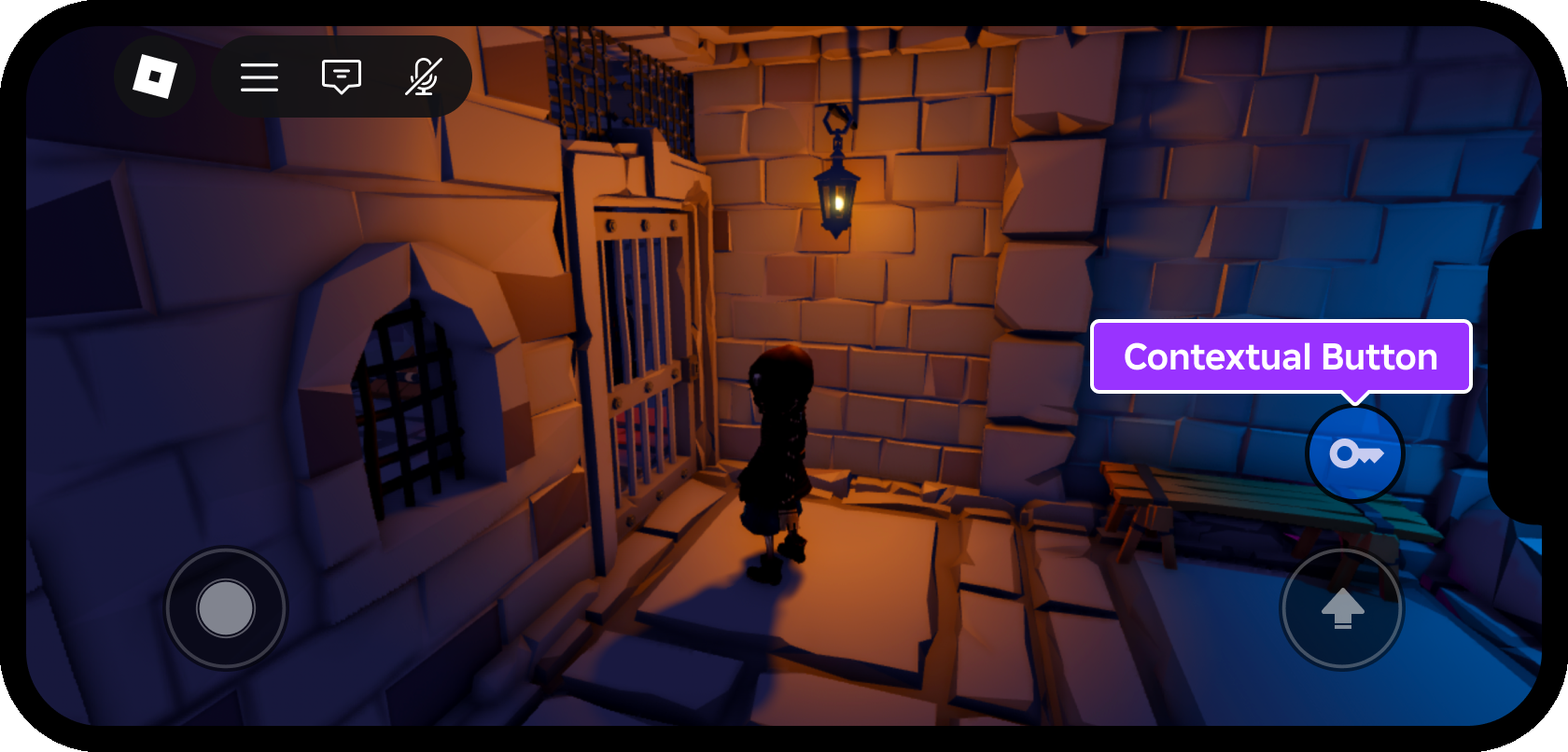