A collision occurs when two 3D objects come into contact within the 3D world. For customized collision handling, BasePart has a set of collision events and collision filtering techniques, so you can control which physical assemblies collide with others.
Collision events
Collision events occur when two BaseParts touch or stop touching in the 3D world. You can detect these collisions through the Touched and TouchEnded events which occur regardless of either part's CanCollide property value. When considering collision handling on parts, note the following:
- A part's CanTouch property determines whether it triggers collision events. If set to false, neither Touched nor TouchEnded will fire.
- A part's CanCollide property affects whether it will physically collide with other parts and cause forces to act upon them. Even if CanCollide is disabled for a part, you can detect touch and non‑touch through Touched and TouchEnded events.
- The Touched and TouchEnded events only fire as a result of physical movement, not from a Position or CFrame changes that cause a part to intersect or stop intersecting another part.
Touched
The Touched event fires when a BasePart comes in contact with another, or with a Terrain voxel. It only fires as a result of physical simulation and will not fire when the part's Position or CFrame is explicitly set such that it intersects another part or voxel.
The following code pattern shows how the Touched event can be connected to a custom onTouched() function. Note that the event sends the otherPart argument to the function, indicating the other part involved in the collision.
Part Collision
local Workspace = game:GetService("Workspace")
local part = Workspace.Part
local function onTouched(otherPart)
print(part.Name .. " collided with " .. otherPart.Name)
end
part.Touched:Connect(onTouched)
Note that the Touched event can fire multiple times in quick succession based on subtle physical collisions, such as when a moving object "settles" into a resting position or when a collision involves a multi‑part model. To avoid triggering more Touched events than necessary, you can implement a simple debounce system which enforces a "cooldown" period through an instance attribute.
Part Collision With Cooldown
local Workspace = game:GetService("Workspace")
local part = Workspace.Part
local COOLDOWN_TIME = 1
local function onTouched(otherPart)
if not part:GetAttribute("Touched") then
print(part.Name .. " collided with " .. otherPart.Name)
part:SetAttribute("Touched", true) -- Set attribute to true
task.wait(COOLDOWN_TIME) -- Wait for cooldown duration
part:SetAttribute("Touched", false) -- Reset attribute
end
end
part.Touched:Connect(onTouched)
TouchEnded
The TouchEnded event fires when the entire collision bounds of a BasePart exits the bounds of another BasePart or a filled Terrain voxel. It only fires as a result of physical simulation and will not fire when the part's Position or CFrame is explicitly set such that it stops intersecting another part or voxel.
The following code pattern shows how the TouchEnded event can be connected to a custom onTouchEnded() function. Like Touched, the event sends the otherPart argument to the function, indicating the other part involved.
Non-Collision Detection
local Workspace = game:GetService("Workspace")
local part = Workspace.Part
local function onTouchEnded(otherPart)
print(part.Name .. " is no longer touching " .. otherPart.Name)
end
part.TouchEnded:Connect(onTouchEnded)
Collision filtering
Collision filtering defines which physical parts collide with others. You can configure filtering for numerous objects through collision groups or you can control collisions on a part‑to‑part basis with NoCollisionConstraint instances.
Collision groups
Collision groups let you assign BaseParts to dedicated groups and specify whether or not they collide with those in other groups. Parts within non‑colliding groups pass through each other completely, even if both parts have their CanCollide property set to true.
You can easily set up collision groups through Studio's Collision Groups Editor, accessible by clicking the Collision Groups button in the toolbar's Model tab.

The editor functions in either List View which favors docking to the left or right side of Studio, or in a wider Table View, which favors docking to the top or bottom.
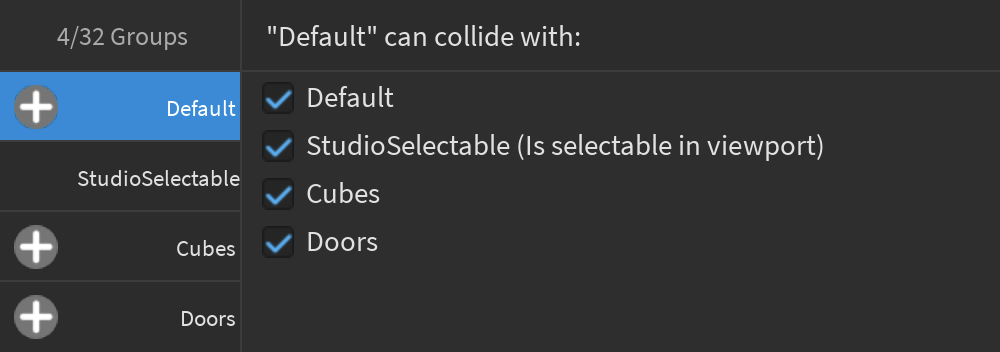
Register groups
The editor includes one Default collision group which cannot be renamed or deleted. All BaseParts automatically belong to this default group unless assigned to another group, meaning that they will collide with all other objects in the Default group.
To create a new collision group:
Click the Add Group button along the top of the editor panel, enter a new group name, and press Enter. The new group appears in both columns of list view, or in both the left column and upper row of table view.
Repeat the process if necessary, choosing a unique and descriptive name for each group. Note that you can change a group's name during development by clicking in its field, or by selecting it and clicking the rename button.
Configure group collisions
Under default configuration, objects in all groups collide with each other. To prevent objects in one group from colliding with objects in another group, uncheck the box in the respective row/column.
In the following example, objects in the Cubes group will not collide with objects in the Doors group.
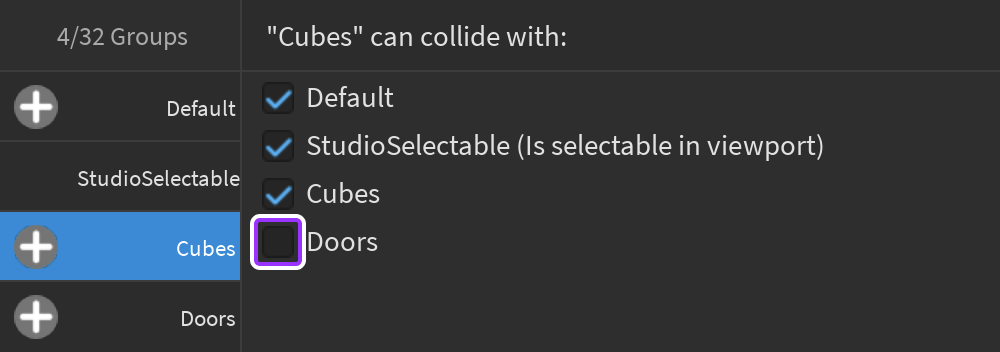
Assign objects to groups
To assign objects to groups you've registered through the Studio editor:
Select one or more BaseParts that qualify as part of a collision group.
Assign them to the group by clicking the ⊕ button for its row. Objects can belong to only one collision group at a time, so placing them in a new group removes them from their current group.
Once assigned, the new group is reflected under the object's CollisionGroup property.
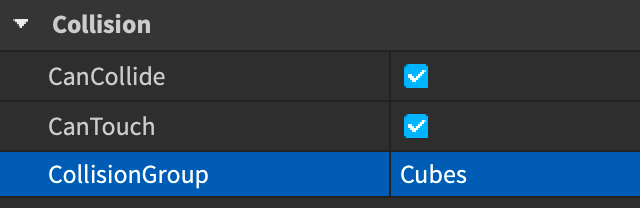
StudioSelectable collision group
Tools in Studio use the collision filtering system to determine which objects are candidates for selection when clicking in the 3D viewport. Objects whose assigned collision group does not collide with StudioSelectable will be ignored.
For example, if you have checkpoints in a racing experience whose effective areas are defined by large transparent parts, you can assign them to a Checkpoints collision group and then make that group non‑collidable with StudioSelectable so that they don't get in the way when you're editing the underlying map geometry.
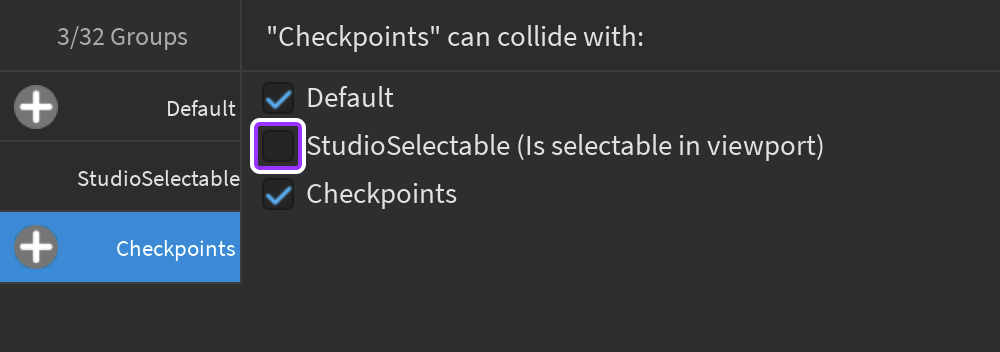
For plugin code, it's recommended that you assign "StudioSelectable" as the collision group filter of your RaycastParams when finding parts under the cursor. This allows your plugins to match the selection mechanics that creators have learned to expect from built‑in Studio tools.
Recommended Plugin Selection Raycast
local UserInputService = game:GetService("UserInputService")local Workspace = game:GetService("Workspace")local raycastParams = RaycastParams.new()raycastParams.CollisionGroup = "StudioSelectable" -- To follow the conventionraycastParams.BruteForceAllSlow = true -- So that parts with CanQuery of "false" can be selectedlocal mouseLocation = UserInputService:GetMouseLocation()local mouseRay = Workspace.CurrentCamera:ViewportPointToRay(mouseLocation.X, mouseLocation.Y)local filteredSelectionHit = Workspace:Raycast(mouseRay.Origin, mouseRay.Direction * 10000, raycastParams)
Part-to-part filtering
To prevent collisions between two specific parts without setting up collision groups, such as between a vehicle's wheel and its chassis, consider the No Collision constraint. Advantages include:
- Collision groups and/or configuration scripts are not required, so you can easily create and share models with customized collision filtering.
- Connected parts will not collide with each other, but they can still collide with other objects.
Disable character collisions
Roblox player characters collide with each other by default. This can lead to interesting but unintended gameplay, such as characters jumping on top of each other to reach specific areas. If this behavior is undesirable, you can prevent it through the following Script in ServerScriptService.
Script - Disable Character Collisions
local PhysicsService = game:GetService("PhysicsService")
local Players = game:GetService("Players")
local CollisionGroupName = "Characters"
PhysicsService:RegisterCollisionGroup(CollisionGroupName)
PhysicsService:CollisionGroupSetCollidable(CollisionGroupName, CollisionGroupName, false)
local function setCollisionGroup(model)
-- Apply collision group to all existing parts in the model
for _, descendant in model:GetDescendants() do
if descendant:IsA("BasePart") then
descendant.CollisionGroup = CollisionGroupName
end
end
end
Players.PlayerAdded:Connect(function(player)
player.CharacterAdded:Connect(function(character)
setCollisionGroup(character)
end)
-- If the player already has a character, apply the collision group immediately
if player.Character then
setCollisionGroup(player.Character)
end
end)
Model collisions
Model objects are containers for parts rather than inheriting from BasePart, so they can't directly connect to BasePart.Touched or BasePart.TouchEnded events. To determine whether a model triggers a collision events, you need to loop through its children and connect the custom onTouched() and onTouchEnded() functions to each child BasePart.
The following code sample connects all BaseParts of a multi‑part model to collision events and tracks the total number of collisions with other parts.
Model Collision
local model = script.Parent
local numTouchingParts = 0
local function onTouched(otherPart)
-- Ignore instances of the model intersecting with itself
if otherPart:IsDescendantOf(model) then return end
-- Increase count of model parts touching
numTouchingParts += 1
print(model.Name, "intersected with", otherPart.Name, "| Model parts touching:", numTouchingParts)
end
local function onTouchEnded(otherPart)
-- Ignore instances of the model un-intersecting with itself
if otherPart:IsDescendantOf(model) then return end
-- Decrease count of model parts touching
numTouchingParts -= 1
print(model.Name, "un-intersected from", otherPart.Name, "| Model parts touching:", numTouchingParts)
end
for _, child in model:GetChildren() do
if child:IsA("BasePart") then
child.Touched:Connect(onTouched)
child.TouchEnded:Connect(onTouchEnded)
end
end
Mesh and solid model collisions
MeshPart and PartOperation (parts joined by solid modeling) are subclasses of BasePart, so meshes and solid modeled parts inherit the same collision events and collision filtering options as regular parts. However, since meshes and solid modeled parts usually have more complex geometries, they have a distinctive CollisionFidelity property which determines how precisely the physical bounds align with the visual representation for collision handling.
The CollisionFidelity property has the following options, in order of fidelity and performance impact from lowest to highest:
- Box — Creates a bounding collision box, ideal for small or non‑interactive objects.
- Hull — Generates a convex hull, suitable for objects with less pronounced indentations or cavities.
- Default — Produces an approximate collision shape that supports concavity, suitable for complex objects with semi-detailed interaction needs.
- PreciseConvexDecomposition — Offers the most precise fidelity but still not a 1:1 representation of the visual. This option has the most expensive performance cost and takes longer for the engine to compute.
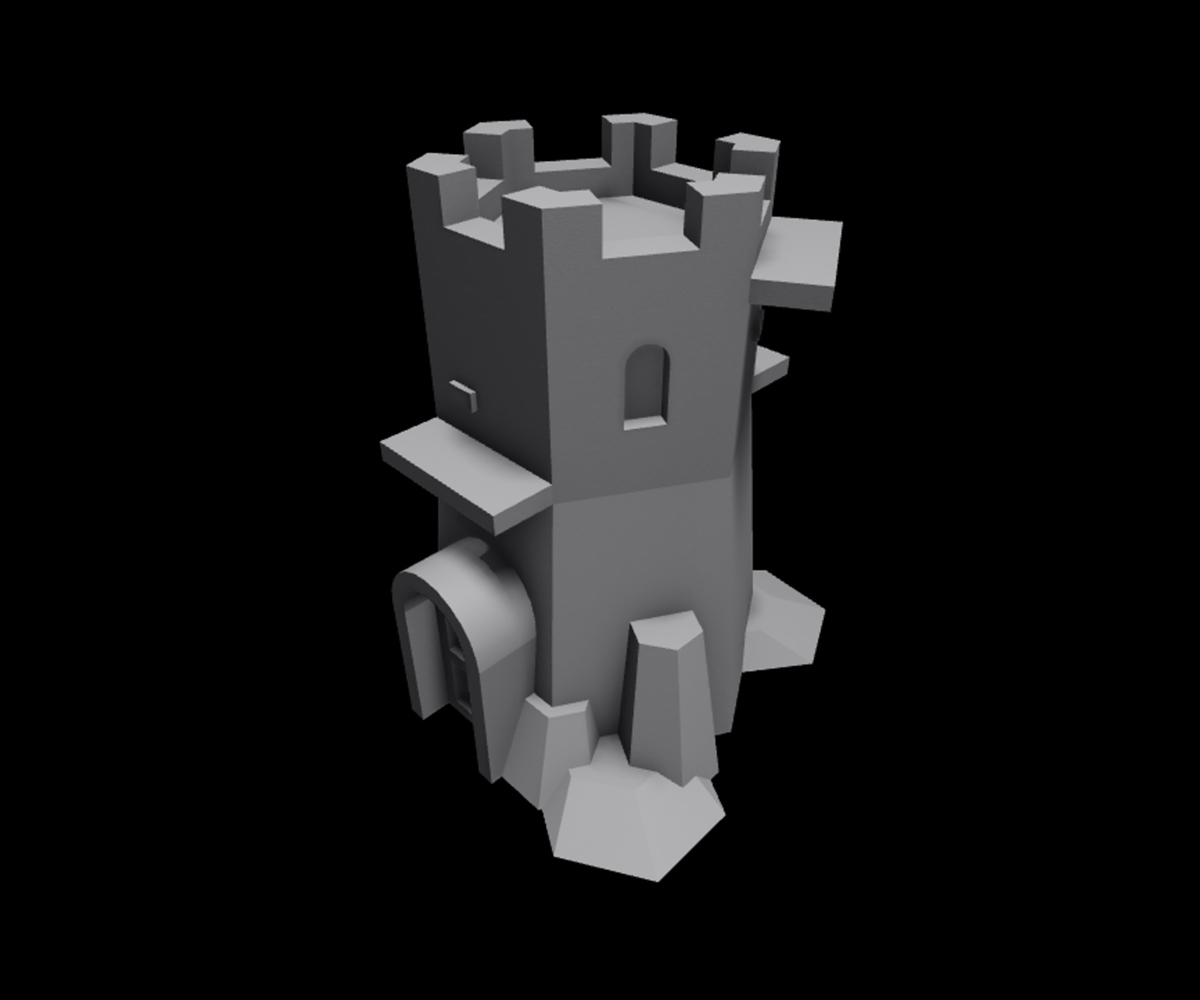
For more information on the performance impact of collision fidelity options and how to mitigate them, see Performance optimization.