參與獎勵 功能包是一個可定制的框架,用於為特定玩家活動提供遊戲內獎勵,包括每日登錄連續日和遊戲會話時間。除了客戶端和伺服器邏輯外,包裝還包括用於查看獎勵進度、獎勵狀態和領取獎勵的預設介面。
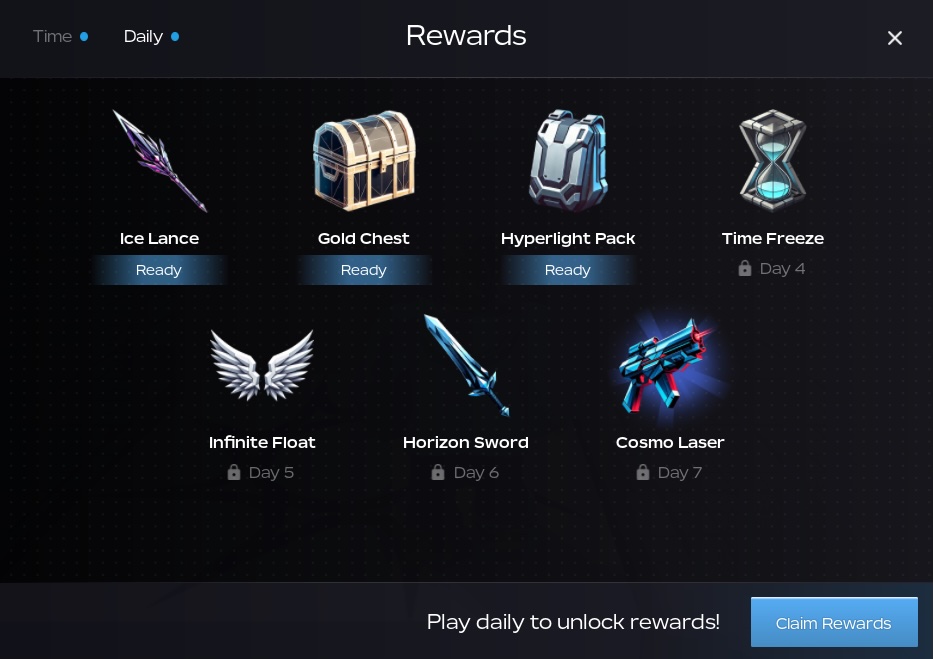
取得包裹
在工作室中,選擇 視圖 標籤。
點擊 工具箱 。
在 工具箱 窗口中,單擊 庫存 標籤。
按一下 功能包核心 磚塊,然後按一下 參與獎勵功能包 磚塊。兩個包夾都會顯示在 資料檢視器 視窗中。
將文件夾拖入 ReplicatedStorage 。
啟用包裝
將包裹移動到 ReplicatedStorage 並測試您的體驗執行 EngagementRewardsExample 腳本在 ReplicatedStorage.EngagementRewards.Server.Examples 中。
這個腳本顯示如何初始化包以供體驗使用,這包括需要多個模組腳本並定義一個 rewardClaimedHandlerFunction() 函數來最終向玩家提供獎勵(s)。
此功能必須返回布林。在示例腳指令碼中,您可以看到它沒有給玩家任何獎勵;它只打印玩家、獎勵和要領取的數量。
參與獎勵示例
local ReplicatedStorage = game:GetService("ReplicatedStorage")
local EngagementRewardsConfig = require(ReplicatedStorage.EngagementRewards.Configs.EngagementRewardsConfig)
local EngagementRewards = require(ReplicatedStorage.EngagementRewards.Server.EngagementRewards)
local EngagementRewardsUtils = require(ReplicatedStorage.EngagementRewards.Utils.EngagementRewardsUtils)
local Types = require(ReplicatedStorage.EngagementRewards.Configs.Types)
-- 將此處理器功能替換為您自己領取的獎勵處理器功能
-- 此功能應該處理傳送給獎勵ID的獎勵領取事件
local function rewardClaimedHandlerFunction(player: Player, rewardId: RewardId, quantity: number): (boolean, string?)
print(`Reward {rewardId} claimed by {player} with quantity {quantity}`)
return true
end
-- more
您可以直接修改此腳本或將其移動到 伺服器腳本服務 如果那是您喜歡的伺服器代碼位置。狀態為「原樣」,腳本只有測試用途。
給予玩家獎勵的方式會因經驗而異。在某些體驗中,您可能會授予體驗提升或只是增加玩家的黃金數量。在其他體驗中,您可能會擁有自定義庫存系統,而其他人可能會將物品放入玩家背包。但在所有情況下,您必須用自己的函數來替換 rewardClaimedHandlerFunction() 。
添加獎勵和條件
大多數獎勵自訂發生在 ReplicatedStorage.EngagementRewards.Configs.EngagementRewardsConfig 內。此模組腳本定義了獎勵和解鎖它的要求。
您可以在 ReplicatedStorage.EngagementRewards.Configs.Types 中查看完整類型宣言(或添加新的類型),但您可能只需要與 EngagementRewardsConfig 工作。功能包包括兩種獎勵類型:Time和Daily。
local engagementRewardsConfig: Types.EngagementRewardsConfig = {[Types.RewardType.Time] = {tabDisplayName = "Time",tabOrder = 1,description = "Keep playing to unlock rewards!",rewards = {MinutesPlayed1 = {icon = 116913478160966,displayName = "Ice Lance",requiredSecondsInGame = 1 * 5,},-- 更多[Types.RewardType.Daily] = {tabDisplayName = "Daily",tabOrder = 2,description = "Play daily to unlock rewards!",rewards = {DailyStreak1 = {icon = 116913478160966,displayName = "Ice Lance",requiredDaysVisitedStreak = 1,effect = Types.RewardEffect.Valuable,},-- more
兩種獎勵類型都需要一個圖示,這是 Roblox 圖像資產 ID( 不是 一個貼花)。你也可能想要一個用於介面使用的顯示名稱。為 1 以外的值指定 quantity。您也可選擇將獎勵指定為具有價值()來在介面上給予不同的背景框架。
變數 | 類型 | 說明 | 預設 | 需要 :--- | :--- | :--- | :--- | :--- 圖示 | 數字 | Roblox 介面圖示的資產ID。| N/A | 是 displayName | 字串 | 在介面上使用的獎勵名稱。| RewardId | 沒有數量 | 數字 | 要獎勵的物品數量。| 1 | 沒有效果 | 獎勵效果 | UI中使用的視覺效果。請參閱自訂介面。| Types.RewardEffect.Default | No
时间奖励
Time自訂這些數字以滿足您的需求。你可能會使用小數字進行簡單測試,在發布體驗中,在 10 分鐘後授予獎勵、在 30 分鐘後再授予獎勵、在一小時後再授予獎勵等等。
乘法使數字在秒鐘內更容易讓人類使用,因此在兩小時內,你可能會選擇指定 2 * 60 * 60 而不是 7200 。
變數 | 類型 | 說明 | 預設 | 需求 :--- | :--- | :--- | :--- | :--- 需要秒數在遊戲中花費來獲得獎勵。| N/A | 是
每日獎勵
Daily 獎勵在連續登入多天後解鎖。例如,當玩家第一次登入時,您可能會給他們三種藥水或肉桂捲。之後的日子裡,你可能會獎勵更有價值的消耗品,然後跟隨一件耐用的物道具,例如新的釣魚竿,在七天內。
變數 | 類型 | 說明 | 預設 | 需求 :--- | :--- | :--- | :--- | :--- requiredDaysVisitedStreak | 數字 | 玩家必須連接到體驗以獲得獎勵的連續日數。| N/A | 是
額外的每日獎勵配置選項還有在 DailyRewardTabConfig 。
[Types.RewardType.Daily] = {tabDisplayName = "Daily",tabOrder = 2,description = "Play daily to unlock rewards!",isHiddenOnJoin = true,isAlignedToStreakResetTime = true,rewards = {DailyStreak1 = {icon = 116913478160966,displayName = "Ice Lance",requiredDaysVisitedStreak = 1,},-- more
變數 | 類型 | 說明 | 預設 | 需求 :--- | :--- | :--- | :--- | :--- 隱藏在加入體驗時不會自動顯示獎勵。當為 false 時,玩家加入遊戲時會自動出現獎勵,如果玩家有一個新的每日獎勵可領取。| 否 | 沒有配置到連續重置時間 | 布林 | 當真實時,第二天的獎勵可以在初始第一天領取時間後的 24 小時領取。當為 false 時,第 2 天獎勵可在第 1 天可領取時間後的第一個午夜領取。例如,如果玩家於晚上11:00(23:00)登入並領取第一天獎勵,真正意味著他們下一天11:00之前無法領取第二天獎勵。假意味著他們可以在上午 12:00 (00:00) 領取第 2 天獎勵。第 3 天及以後的獎勵總是在前一天可領取時間後的 24 小時內提供。| 否 | 否
自訂介面使用者介面
在前一節中,您可能已注意到 tabDisplayName 、 tabOrder 和 description 欄位,這些欄位可用於基本自訂使用者介面。你也可以在 ReplicatedStorage.FeaturePackagesCore.Configs.TranslationStrings 中找到一些 UI 字串。
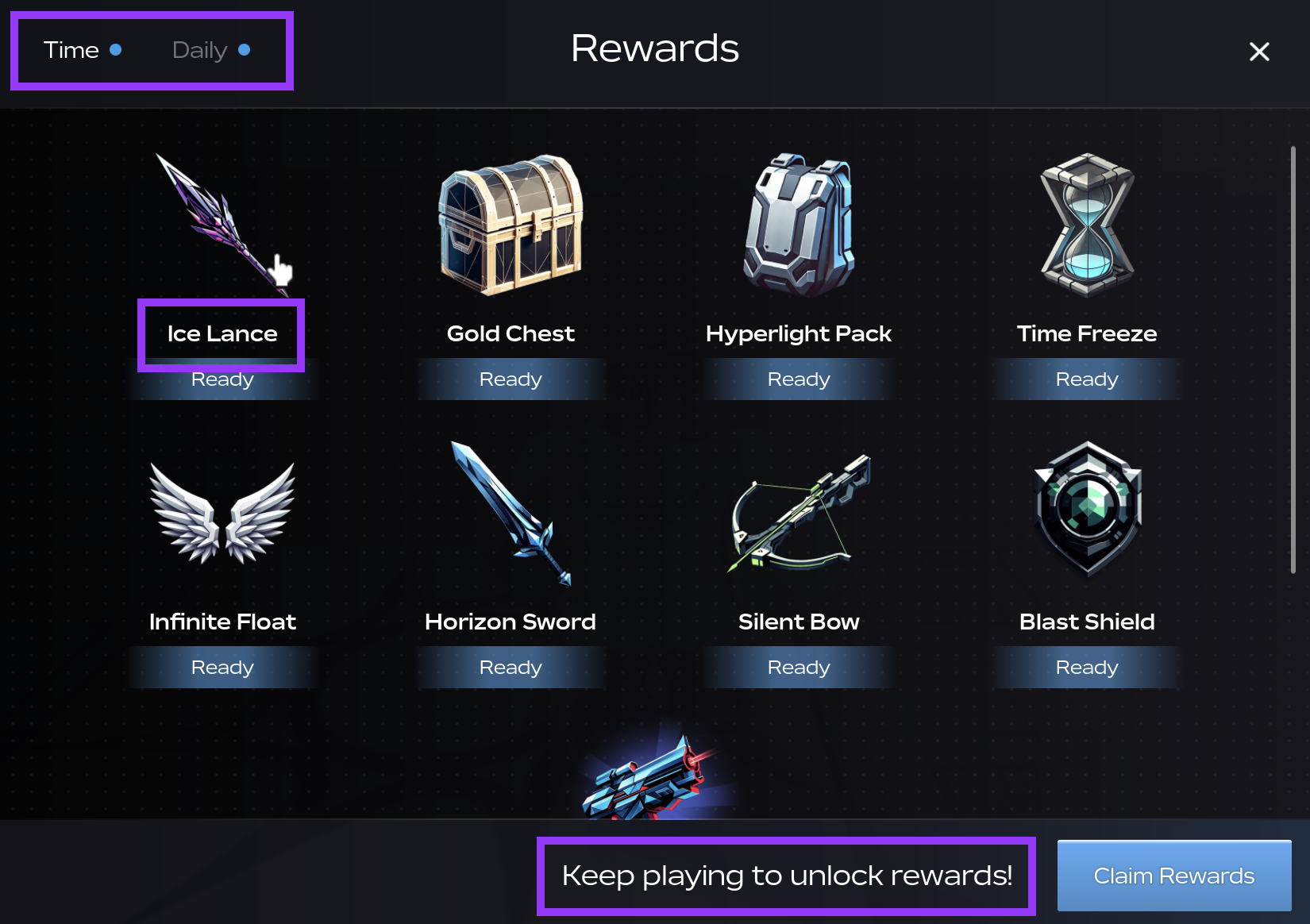
要使用 effect 變量指定新的獎勵背景,請在 ReplicatedStorage.EngagementRewards.Objects.RewardItemFrames 添加一個框。然後將框架名稱添加到 Types.RewardEffect 表中的 ReplicatedStorage.EngagementRewards.Configs.Types 表中。
若要更完整地自訂,請修改 ReplicatedStorage.EngagementRewards.Objects 中的對象。例如,您可能會修改 BackgroundColor3 框架的 RewardsHudButton.Background 或 Color 的 FooterContentFrame.ClaimableUIGradient 。
用戶介面的入口是 ReplicatedStorage.EngagementRewards.Client.UIController 指令碼本,它會獲得必要的對象並初始化用戶介面。如果您添加或重命名最高級別對象(與只添加子對象或修改屬性不同),您可能需要在此文件夾中更新代碼來處理它們。