A 開發者產品 是一種用戶可以多次購買的物品或能力,例如經驗貨幣、彈藥或藥水。
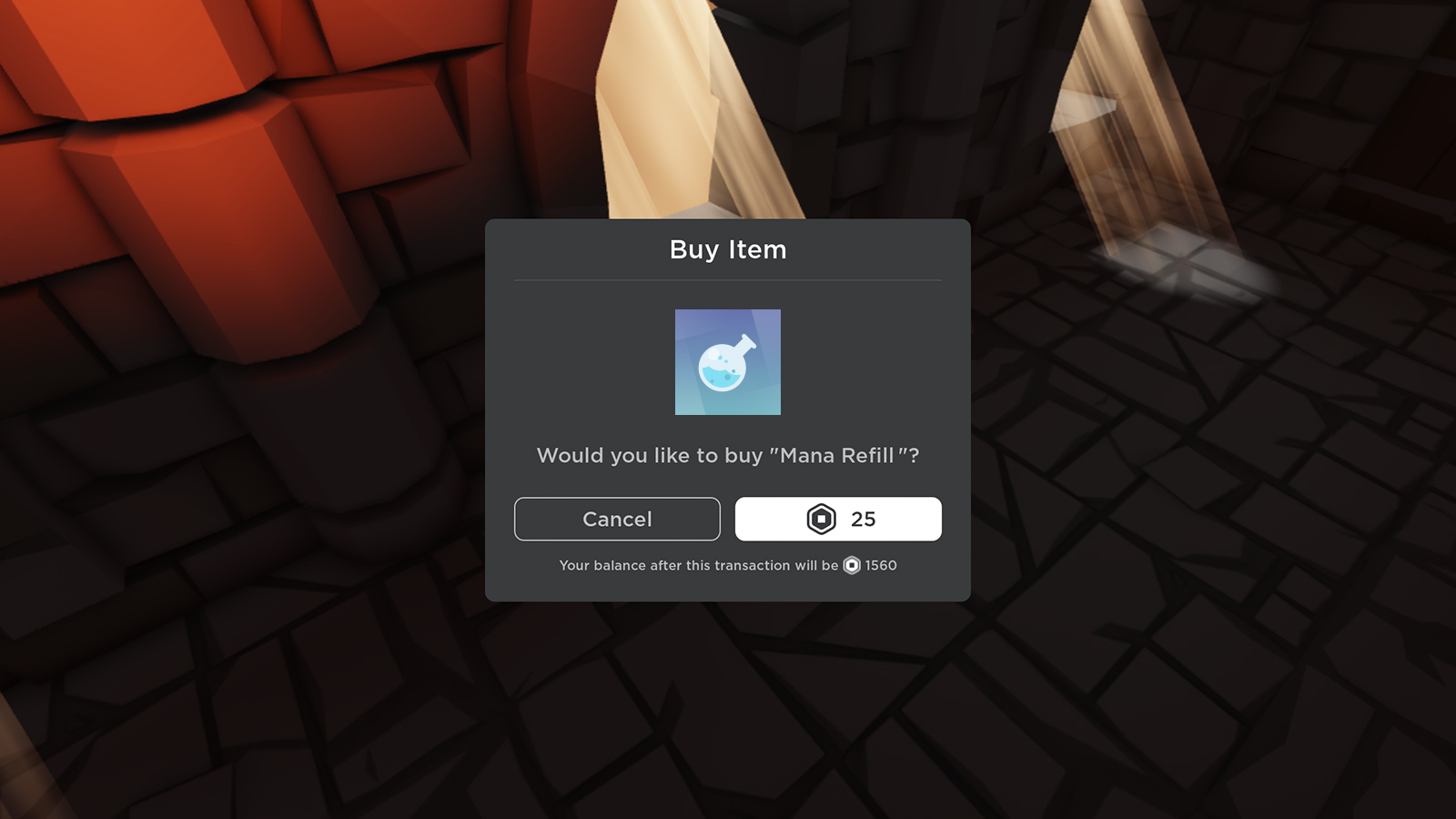
創建一個開發者產品
要創建開發者產品:
- 前往 創作 並選擇一個體驗。
- 前往 盈利化 > 開發者產品 。
- 點擊 創建開發者產品 。
- 上傳圖像以顯示為產品圖示。請確保圖像不超過 512x512 像素、不包含外部圓形邊界之外的重要細節,並使用 .jpg、.png 或 .bmp 格式。
- 輸入產品的名稱和說明。
- 將產品價格設為 Robux。最低價格為 1 Robux,最高價格為 10 億 Robux。
- 點擊 創建開發者產品 。
取得開發者產品 ID
若要使用指令碼化,您需要一個開發者產品 ID。要取得產品 ID:
前往 盈利 > 開發者產品 。
將鼠標懸停在產品的縮略圖上,點擊 ⋯ 按鈕,然後從上下文選單中選擇 複製資產ID 。
出售開發者產品
在出售開發者產品之前,請確保您正確處理銷售收據並向用戶授予他們購買的產品。要這麼做,您必須:
- 使用 ProcessReceipt API 來檢查購買收據。ProcessReceipt
- 驗證每個收據的 User ID、Developer Product ID 和收據狀態。
- 如果收據的狀態為 開啟 ,則授予用戶他們購買的開發者物品。
- 使用 ProcessReceipt API 回應以認可收悉和驗證購買的物品已獲得。
您可以以兩種方式出售開發者產品:
在你的體驗中
要在體驗中實裝並銷售開發者產品,請呼叫 MarketplaceService 功能。
使用 GetProductInfo 來恢復開發者產品的資訊,例如名稱和價格,然後將該產品顯示給使用者。您可以在體驗市場內出售產品,例如。對於開發者產品,第二個參數必須為 Enum.InfoType.Product 。
local MarketplaceService = game:GetService("MarketplaceService")
-- 將暫時器 ID 替換為開發者產品 ID
local productId = 000000
local success, productInfo = pcall(function()
return MarketplaceService:GetProductInfo(productId, Enum.InfoType.Product)
end)
if success and productInfo then
-- 顯示產品資訊
-- 將列印聲明替換為使用者介面代碼來顯示產品
print("Developer Product Name: " .. productInfo.Name)
print("Price in Robux: " .. productInfo.PriceInRobux)
print("Description: " .. productInfo.Description)
end
使用 GetDeveloperProductsAsync 來恢復與您體驗相關的所有開發產品。此功能返回一個 Pages 對象,您可以檢查和過濾以構建物品,例如體驗商店或產品列表 GUI。
local MarketplaceService = game:GetService("MarketplaceService")
local success, developerProducts = pcall(function()
return MarketplaceService:GetDeveloperProductsAsync()
end)
if success and developerProducts then
local firstPage = developerProducts:GetCurrentPage()
for _, developerProduct in firstPage do
-- 將列印聲明替換為使用者介面代碼來顯示產品
print(field .. ": " .. value)
end
end
使用 PromptProductPurchase 來提示體驗內的產品購買。當使用者執行按下按鈕或與供應商 NPC 交談等動作時,您可以呼叫此功能。
local MarketplaceService = game:GetService("MarketplaceService")
local Players = game:GetService("Players")
local player = Players.LocalPlayer
-- 將暫時器 ID 替換為開發者產品 ID
local productId = 000000
local function promptProductPurchase()
local success, errorMessage = pcall(function()
MarketplaceService:PromptProductPurchase(player, productId)
end)
if success then
print("Purchase prompt shown successfully")
end
end
您也可以在 LocalScript 內結合功能。例如,您可以創建像按鈕或供應商 NPC 一樣的 UI 元素,然後使用 GetProductInfo() 將現有開發者產品連接到該元素,檢查產品是否待買賣,並使用 PromptProductPurchase() 每當使用者單擊它時提示購買。
local MarketplaceService = game:GetService("MarketplaceService")
local Players = game:GetService("Players")
local player = Players.LocalPlayer
local button = script.Parent
-- 將暫時器 ID 替換為開發者產品 ID
local productId = 000000
-- 當使用者單擊介面按鈕時,獲得產品資訊
button.MouseButton1Click:Connect(function()
local success, productInfo = pcall(function()
return MarketplaceService:GetProductInfo(productId, Enum.InfoType.Product)
end)
if success and productInfo then
-- 檢查產品是否待買賣
if productInfo.IsForSale then
print("This is for sale")
-- 提示產品購買
MarketplaceService:PromptProductPurchase(player, productId)
else
-- 通知產品不待買賣
print("This product is not currently for sale.")
end
else
print("Error retrieving product info: " .. tostring(productInfo))
end
end)
超出你的體驗
若要啟用開發者產品在你的體驗之外的購買,你必須使用 ProcessReceipt API。在使用者在體驗詳情頁面的 商店 標籤進行購買後,您必須使用 ProcessReceipt 來確認購買並在他們進入您的體驗時授予他們物品。
測試模式
測試模式 功能 幫助您通過模擬開發者產品在體驗之外購買來驗證您的購物流程。您應該使用測試模式來確保在啟用外部開發者產品銷售之前,您已正確實裝 ProcessReceipt 。
你在測試模式下放置的開發者產品只能由你和群組成員查看。它們對使用者不可見。
要測試您的實現:
- 在 創作者中心 ,前往 盈利 > 開發者產品 。
- 點擊 ⋮ 菜單,然後選擇 外部購買設定 。
- 在 外部購買設定 頁面中,單擊 啟用測試模式 。
- 一旦測試模式啟用,返回到 開發者產品 頁面,選擇一個產品進行測試。
- 在 基本設定 頁面中,選擇 允許外部購買 檢查框並保存您的變更。
- 前往體驗詳情頁面的 商店 標籤,購買你提供出待售的產品。
- 進入體驗並確認您已收到購買的產品。ProcessReceipt的收據狀態應更新為 關閉 。
在你測試你的實現之後,Roblox 會驗證測試已成功完成,並允許你完全啟用功能來在體驗之外出售開發者產品。
有關ProcessReceipt和其實現的更多信息,請參閱ProcessReceipt。
啟用外部銷售
要啟用外部銷售:
- 前往 外部購買設定頁 。
- 開啟 外部購買 。
- 返回到 開發者產品 頁面,選擇你想在體驗之外銷售的產品。
- 在 基本設定 頁面中,選擇 允許外部購買 檢查框並保存您的變更。
- 確認產品現在可以在體驗詳情頁面的 商店 標籤購買。
若要停用開發者產品的外部銷售,請在 開發者產品 頁面選擇產品,並清除 允許外部購買 檢查框。
限制
- 在測試模式中出售的物品需要實際 Robux 費用。我們建議測試低成本開發者產品。
- 測試模式下出售的物品只能由你或你的群組成員查看。
- 要外部銷售,您的開發者產品 必須 擁有縮圖。
- 你不應該在體驗之外出售以下物品:
- 付費隨機物品
- 限於特定數量、時間、空間點或使用者設定和角色的物品
處理開發者產品購買
當使用者購買開發者產品後,您必須處理和記錄交易。要執行此操作,請使用 Script 內的 ServerScriptService 使用 ProcessReceipt 功能。
有關ProcessReceipt和其實現的更多信息,請參閱ProcessReceipt。
local MarketplaceService = game:GetService("MarketplaceService")
local Players = game:GetService("Players")
local productFunctions = {}
-- 例如:產品ID 123123帶回用戶至完全健康
productFunctions[123123] = function(receipt, player)
local character = player.Character
local humanoid = character and character:FindFirstChildWhichIsA("Humanoid")
if humanoid then
humanoid.Health = humanoid.MaxHealth
-- 指示成功購買
return true
end
end
-- 例如:產品 ID 456456 獎勵用戶 100 枚金幣
productFunctions[456456] = function(receipt, player)
local leaderstats = player:FindFirstChild("leaderstats")
local gold = leaderstats and leaderstats:FindFirstChild("Gold")
if gold then
gold.Value += 100
return true
end
end
local function processReceipt(receiptInfo)
local userId = receiptInfo.PlayerId
local productId = receiptInfo.ProductId
local player = Players:GetPlayerByUserId(userId)
if player then
-- 獲得與開發者產品ID相關的處理器功能,並嘗試運行它
local handler = productFunctions[productId]
local success, result = pcall(handler, receiptInfo, player)
if success then
-- 使用者已收到他們的物品
-- 返回「購買已獲得」以確認交易
return Enum.ProductPurchaseDecision.PurchaseGranted
else
warn("Failed to process receipt:", receiptInfo, result)
end
end
-- 使用者的物品無法獲得獎勵
-- 返回「尚未處理」,下次使用者加入體驗時再試一次
return Enum.ProductPurchaseDecision.NotProcessedYet
end
-- 設置回調
-- 此操作只能由一個服務器側的指令碼執行一次
MarketplaceService.ProcessReceipt = processReceipt
開發者產品分析
使用開發者產品分析來分析個人產品的成功,識別趨勢,並預測潛在未來收入。
使用分析,您可以:
- 查看您在指定時間期間的最佳開發者產品。
- 在時間序列圖上展示最多八個最熱門的產品,分析總銷售額和淨收入。
- 監控您的目錄並依銷售額和淨收入排序項目。
要存取開發者產品分析:
- 前往 創作 並選擇一個體驗。
- 前往 盈利化 > 開發者產品 。
- 選擇 分析 標籤。
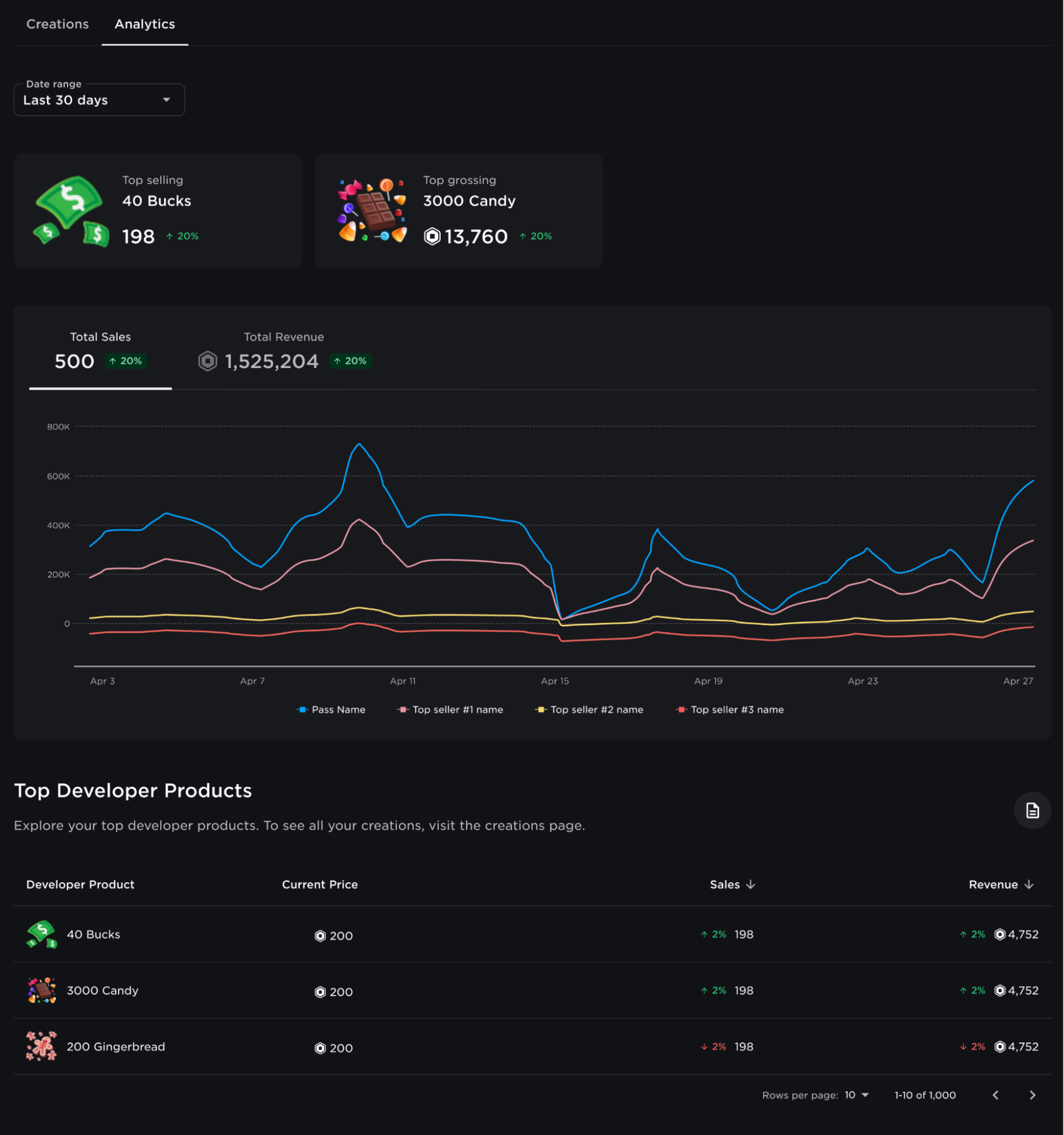