A developer product is an item or ability that a user can purchase more than once, such as in-experience currency, ammo, or potions.
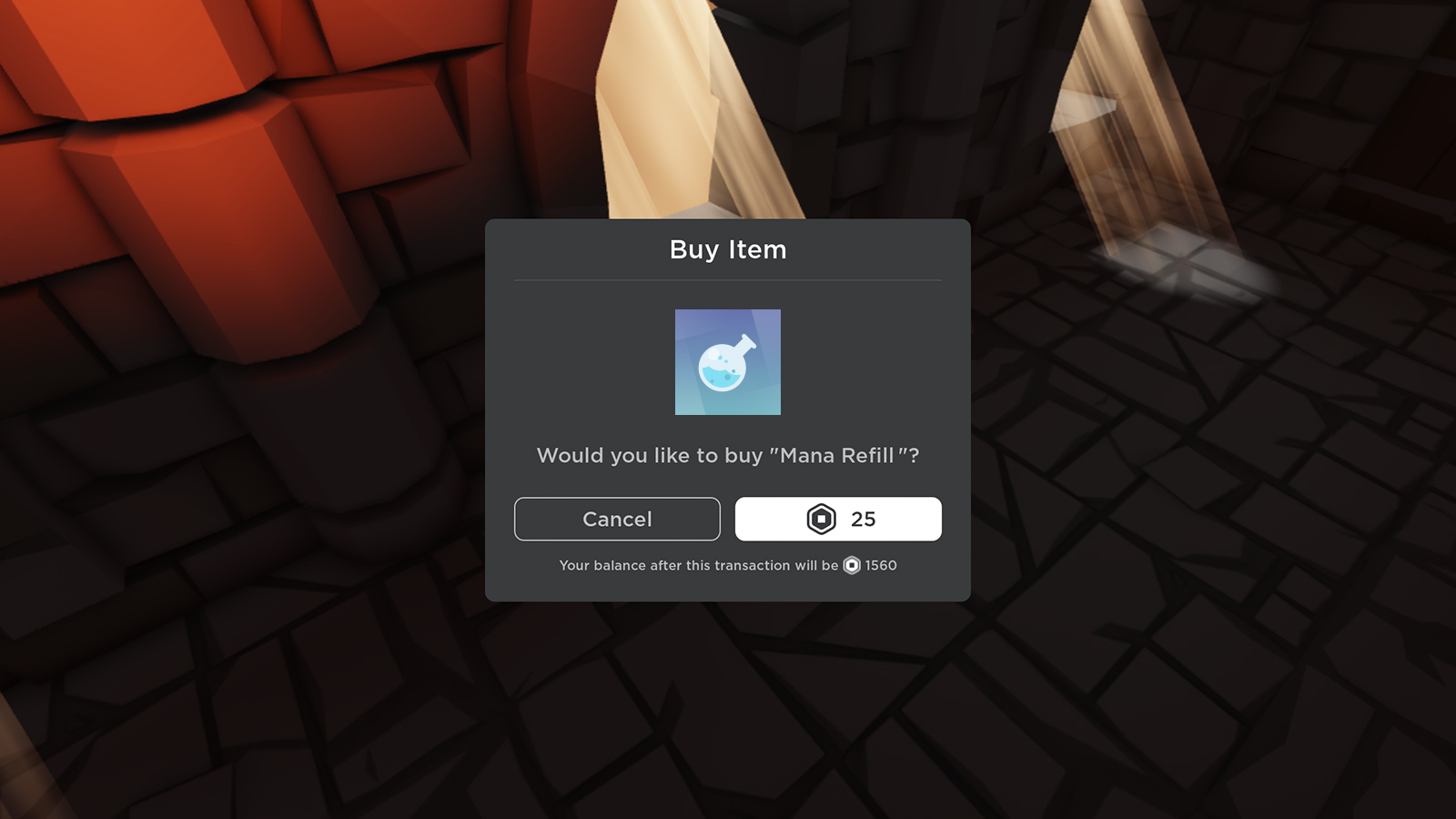
Create a developer product
To create a developer product:
- Go to Creations and select an experience.
- Go to Monetization > Developer Products.
- Click Create a Developer Product.
- Upload an image to display as the product icon. Make sure the image doesn't exceed 512x512 pixels, doesn't include important details outside of its circular boundaries, and is in .jpg, .png, or .bmp format.
- Enter a name and a description for the product.
- Set the product price in Robux. The minimum price is 1 Robux, and the maximum price is 1 billion Robux.
- Click Create Developer Product.
Get the developer product ID
To use scripting, you need a developer product ID. To get the product ID:
Go to Monetization ⟩ Developer Products.
Hover over a product's thumbnail, click the ⋯ button, and select Copy Asset ID from the context menu.
Sell a developer product
Before selling developer products, make sure you are properly processing sales receipts and granting users their purchased products. To do so, you must:
- Use the ProcessReceipt API to check purchase receipts. ProcessReceipt automatically reads and acknowledges that a user has purchased a product outside of the experience.
- Validate each receipt for User ID, Developer Product ID, and the receipt status.
- If the receipt has a status of Open, grant the user the developer items they have purchased.
- Respond to the ProcessReceipt API with a message acknowledging the receipt and validating that the purchased items were granted.
You can sell developer products in two ways:
Inside your experience
To implement and sell a developer product inside an experience, call MarketplaceService functions.
Use GetProductInfo to retrieve information about a developer product, like name and price, and then to display that product to users. You can sell the product inside your experience's marketplace, for example. For developer products, the second parameter must be Enum.InfoType.Product.
local MarketplaceService = game:GetService("MarketplaceService")
-- Replace the placeholder ID with your developer product ID
local productId = 000000
local success, productInfo = pcall(function()
return MarketplaceService:GetProductInfo(productId, Enum.InfoType.Product)
end)
if success and productInfo then
-- Display product information
-- Replace the print statements with UI code to display the product
print("Developer Product Name: " .. productInfo.Name)
print("Price in Robux: " .. productInfo.PriceInRobux)
print("Description: " .. productInfo.Description)
end
Use GetDeveloperProductsAsync to retrieve all developer products associated with your experience. This function returns a Pages object that you can inspect and filter to build things like an in-experience store or product list GUI.
local MarketplaceService = game:GetService("MarketplaceService")
local success, developerProducts = pcall(function()
return MarketplaceService:GetDeveloperProductsAsync()
end)
if success and developerProducts then
local firstPage = developerProducts:GetCurrentPage()
for _, developerProduct in firstPage do
-- Replace the print statement with UI code to display the product
print(field .. ": " .. value)
end
end
Use PromptProductPurchase to prompt product purchases inside your experience. You can call this function when a user performs actions like pressing a button or talking to a vendor NPC.
local MarketplaceService = game:GetService("MarketplaceService")
local Players = game:GetService("Players")
local player = Players.LocalPlayer
-- Replace the placeholder ID with your developer product ID
local productId = 000000
local function promptProductPurchase()
local success, errorMessage = pcall(function()
MarketplaceService:PromptProductPurchase(player, productId)
end)
if success then
print("Purchase prompt shown successfully")
end
end
You can also combine functions inside a LocalScript. For example, you can create a UI element like a button or a vendor NPC, then use GetProductInfo() to connect an existing developer product to that element, check if the product is for sale, and use PromptProductPurchase() to prompt a purchase whenever the user clicks on it.
local MarketplaceService = game:GetService("MarketplaceService")
local Players = game:GetService("Players")
local player = Players.LocalPlayer
local button = script.Parent
-- Replace the placeholder ID with your developer product ID
local productId = 000000
-- Gets product info when user clicks the UI button
button.MouseButton1Click:Connect(function()
local success, productInfo = pcall(function()
return MarketplaceService:GetProductInfo(productId, Enum.InfoType.Product)
end)
if success and productInfo then
-- Checks if product is for sale
if productInfo.IsForSale then
print("This is for sale")
-- Prompts product purchase
MarketplaceService:PromptProductPurchase(player, productId)
else
-- Notifies product isn't for sale
print("This product is not currently for sale.")
end
else
print("Error retrieving product info: " .. tostring(productInfo))
end
end)
Outside your experience
To enable developer product purchases outside your experience, you must work with the ProcessReceipt API. After a user makes a purchase in the Store tab of your experience details page, you must use ProcessReceipt to confirm their purchase and grant them their items once they enter your experience.
Test mode
The test mode feature helps you validate your purchase flow by simulating a developer product purchase outside your experience. You should use test mode to make sure that you have implemented ProcessReceipt correctly before enabling external developer product sales.
The developer products you put up for sale in test mode can only be seen by you and by members of your group. They are not visible to users.
To test your implementation:
- In the Creator Hub, go to Monetization > Developer Products.
- Click the ⋮ menu and select External Purchase Settings.
- In the External Purchase Settings page, click Enable test mode.
- Once test mode is active, return to the Developer Products page and select a product to test.
- In the Basic Settings page, select the Allow external purchases checkbox and save your changes.
- Go to the Store tab of the experience details page and purchase the product you made available for sale.
- Enter the experience and confirm that you have received the product you purchased. The receipt status of the ProcessReceipt API should update to Closed.
After you test your implemention, Roblox verifies that the test has been successfully completed and allows you to fully activate the feature to sell developer products outside your experiences.
For more information about the ProcessReceipt API and its implementation, see the ProcessReceipt page.
Enable external sales
To enable external sales:
- Go to the External Purchase Settings page.
- Turn on External Purchases.
- Return to the Developer Products page and select the products you want to sell outside of your experience.
- In the Basic Settings page, select the Allow external purchases checkbox and save your changes.
- Confirm that the products are now available for purchase in the Store tab of the experience details page.
To disable the external sale of a developer product, select the product on the Developer Products page and clear the Allow external purchases checkbox.
Limitations
- Items for sale in test mode cost actual Robux. We recommend testing low-cost developer products.
- Items for sale in test mode can only be seen by you or by members of your group.
- To be sold externally, your developer products must have a thumbnail.
- You should not sell the following outside your experience:
- Paid random items
- Items that are limited to specific quantities, time, place, or user settings and roles
Handle a developer product purchase
After a user purchases a developer product, you must handle and record the transaction. To do this, use a Script within ServerScriptService using the ProcessReceipt function.
For more information about the ProcessReceipt API and its implementation, see the ProcessReceipt page.
local MarketplaceService = game:GetService("MarketplaceService")
local Players = game:GetService("Players")
local productFunctions = {}
-- Example: product ID 123123 brings the user back to full health
productFunctions[123123] = function(receipt, player)
local character = player.Character
local humanoid = character and character:FindFirstChildWhichIsA("Humanoid")
if humanoid then
humanoid.Health = humanoid.MaxHealth
-- Indicates a successful purchase
return true
end
end
-- Example: product ID 456456 awards 100 gold coins to the user
productFunctions[456456] = function(receipt, player)
local leaderstats = player:FindFirstChild("leaderstats")
local gold = leaderstats and leaderstats:FindFirstChild("Gold")
if gold then
gold.Value += 100
return true
end
end
local function processReceipt(receiptInfo)
local userId = receiptInfo.PlayerId
local productId = receiptInfo.ProductId
local player = Players:GetPlayerByUserId(userId)
if player then
-- Gets the handler function associated with the developer product ID and attempts to run it
local handler = productFunctions[productId]
local success, result = pcall(handler, receiptInfo, player)
if success then
-- The user has received their items
-- Returns "PurchaseGranted" to confirm the transaction
return Enum.ProductPurchaseDecision.PurchaseGranted
else
warn("Failed to process receipt:", receiptInfo, result)
end
end
-- The user's items couldn't be awarded
-- Returns "NotProcessedYet" and tries again next time the user joins the experience
return Enum.ProductPurchaseDecision.NotProcessedYet
end
-- Sets the callback
-- This can only be done once by one server-side script
MarketplaceService.ProcessReceipt = processReceipt
Developer product analytics
Use developer product analytics to analyze the success of individual products, identify trends, and forecast potential future earnings.
With analytics, you can:
- View your top developer products over a selected time period.
- Showcase up to eight top-selling items on a time-series graph to analyze overall sales and net revenue.
- Monitor your catalog and sort items by sales and net revenue.
To access developer product analytics:
- Go to Creations and select an experience.
- Go to Monetization > Developer Products.
- Select the Analytics tab.
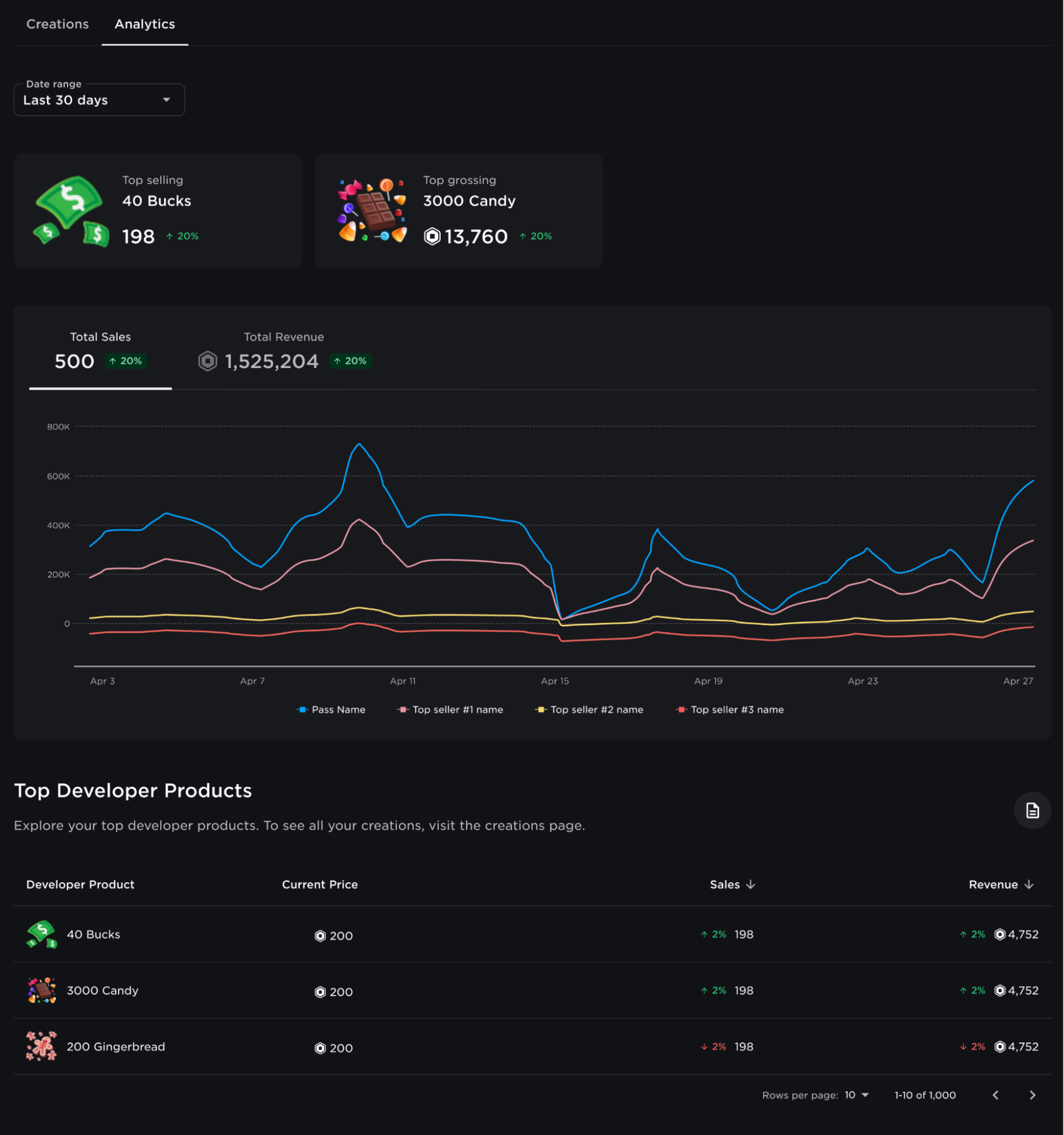