With the map created, it's time to start building out the scripts. The remainder of this course will heavily focus on scripting all different elements of the game loop.
Setting up the Scripts
The battle royale will use a combination of module scripts and normal scripts. Below are the scripts and their functions.
GameManager | Script. Runs functions from the Match Manager using variables from the Game Settings |
MatchManager | Module Script. Runs functions like sending players into an arena or keeping track of time in a match. |
GameSettings | Module Script. Stores commonly used variables used by other scripts. |
GameSettings Script
Create a module script named GameSettings to store variables used by other scripts, like match and intermission duration. These variables will be used by the GameManager script later.
In ServerStorage, create a folder named ModuleScripts. In that folder, create a new module script named GameSettings.
Open GameSettings and rename the module table to match the name of the script.
local GameSettings = {}return GameSettingsIn the module table, add variables for the following uses. Take your best guess for each value, you can always change it later as you test.
- Intermission Duration - Seconds players wait before a match.
- Match Duration - Length of a match in seconds.
- Minimum Players - Smallest number of players needed to start.
- Transition Time - Time before and after a match in seconds. Makes transitioning between parts of the game loop less sudden.
local GameSettings = {}-- Game VariablesGameSettings.intermissionDuration = 5GameSettings.matchDuration = 10GameSettings.minimumPlayers = 2GameSettings.transitionTime = 5return GameSettings
MatchManager Script
The second script connected to the GameManager is the MatchManager. This script manages tasks like starting the timer or resetting players once the match ends.
Within MatchManager is a function named prepareGame() starts the game by transitioning players into the match.
In ServerStorage > ModuleScripts > add a module script named MatchManager. Rename the module table.
local MatchManager = {}return MatchManagerAdd a new module function to MatchManager named prepareGame(). Include a print statement to test the script later.
local MatchManager = {}function MatchManager.prepareGame()print("Game starting!")endreturn MatchManager
Coding the Game Loop
The main game loop will be coded in the GameManager script using the variables just created. Remember, there are three phases in the game loop: intermission, competition, and cleanup & reset.
GameManager Script
This script is a normal server script, so put it in ServerScriptService, rather than the module scripts folder. The actual game loop will be in a while true do loop.
In ServerScriptService, create a new script named GameManager.
Add a variable for the service "ServerStorage", which is where the Modulescripts are. Then add a variable for the service "Players", which will be needed to check player count during intermissions.
-- Serviceslocal ServerStorage = game:GetService("ServerStorage")local Players = game:GetService("Players")To use the previously created modules:
- Set a variable named moduleScripts to the location of the ModuleScripts folder.
- Add variables named matchManager and gameSettings. Set each variable to require their respective script.
-- Serviceslocal ServerStorage = game:GetService("ServerStorage")local Players = game:GetService("Players")-- Module Scriptslocal moduleScripts = ServerStorage:WaitForChild("ModuleScripts")local matchManager = require(moduleScripts:WaitForChild("MatchManager"))local gameSettings = require(moduleScripts:WaitForChild("GameSettings"))After the variables, add a while true do loop. All phases of the game loop will go inside to repeat indefinitely.
-- Module Scriptslocal moduleScripts = ServerStorage:WaitForChild("ModuleScripts")local matchManager = require(moduleScripts:WaitForChild("MatchManager"))local gameSettings = require(moduleScripts:WaitForChild("GameSettings"))-- Main game loopwhile true doend
Coding the Intermission
While the game loop runs indefinitely, the intermission should pause the loop and only continue when there are enough players for a match. To code this pause, include a nested repeat loop for the intermission in the while loop. That nested loop will repeat until there are enough players, pausing the main loop. Once there are enough players, it'll exit and transition players into a match.
With a repeat loop, the code in the loop will run at least once. Unlike a while loop, it doesn't check it's condition until the loop ends. This ensures players always go to the lobby before a match.
In the while true do loop, type repeat and press Enter to autocomplete with the keyword until.
while true dorepeatuntilendCheck if the current number of players (#Players:GetPlayers()) is greater or equal to the minimumPlayers variable created earlier in the GameSettings module.
while true dorepeatuntil #Players:GetPlayers() >= gameSettings.minimumPlayersendIn the repeat loop, add a print statement saying the intermission is starting. Use task.wait() to pause for the intermission using intermissionDuration from GameSettings.
while true dorepeatprint("Starting intermission")task.wait(gameSettings.intermissionDuration)until #Players:GetPlayers() >= gameSettings.minimumPlayersendPlaytest and check that the print statement "Starting intermission" is shown at least twice. Seeing the message twice proves the repeat loop didn't find enough players and ran again. You'll have to wait the length of intermission before seeing the message a second time.
Troubleshooting Tips
At this point, if you're not spawning as intended, try one of the following below.
- task.wait() should be inside the repeat loop. Without the wait, the script will run too many times in a second, overloading Roblox Studio and causing an error.
- In the Game Settings module, the variable intermissionDuration should be greater than 1. If lower, the script can repeat too often, causing slow down issues.
Ending the Intermission
Once there are enough players, have them wait a short transition time. Then, send them into the match by calling the prepareGame() function in MatchManager. Remember, that function just prints a line, but you'll add more code later.
At the end of the repeat loop, add a print statement saying the intermission is over to test your code. Then, follow it with a task.wait() using GameSetting's transitionTime variable.
while true dorepeatprint("Starting intermission")task.wait(gameSettings.intermissionDuration)until #Players:GetPlayers() >= gameSettings.minimumPlayersprint("Intermission over")task.wait(gameSettings.transitionTime)endAfter the wait, call the prepareGame() from the MatchManager module. When the code runs, this will just print text to the output window. Wait until the next section to test this code.
while true dorepeatprint("Starting intermission")task.wait(gameSettings.intermissionDuration)until #Players:GetPlayers() >= gameSettings.minimumPlayersprint("Intermission over")task.wait(gameSettings.transitionTime)matchManager.prepareGame()end
Testing Multiplayer Games
Right now, to have the code run prepareGame(), it needs to exit the repeat loop. But, to do that, there needs to be more than one player. This means if you use the playtest button, the function will never run because you're the only player in the game (unless your minimum players is one). To test this out, you'll need to simulate a multiplayer game.
Starting a Local Server
To test code requiring more than one player, create a local server. While published games are normally on Roblox servers, a local server simulates a multiplayer game on your computer with simulated players.
To start a local server, in the Test tab > Clients and Servers section > set the player dropdown to the number of players in GameSetting's variable minimumPlayers. This lesson uses 2 players.
Click Start to begin the server.
Wait a few seconds for the server to set up. Multiple windows will open in addition to your original Studio window. You may need to allow access to Roblox Studio from firewalls or other online security software.
Troubleshooting Tips
At this point, you're unable to see the test servers, try one of the following below.
- If you have any issues with the server starting, double check the article Firewall and Router Issues.
- Set the number of players to a small amount, like 2 or 3.
- If the issue doesn't resolve, try restarting Studio or restarting your computer.
Testing in the Local Server
You'll see multiple windows when the server starts. Each one represents a different part of the server/client relationship.
- Server (green border) runs the game.
- Client (blue borders) simulates a player's experience.
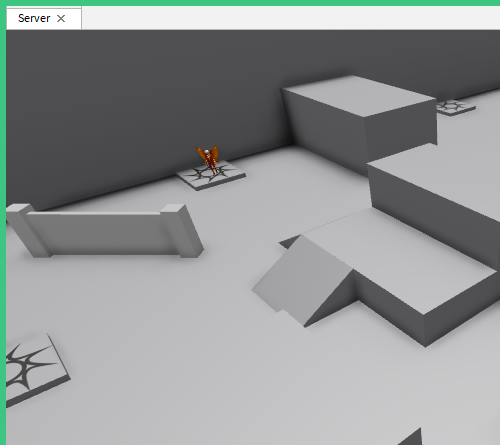
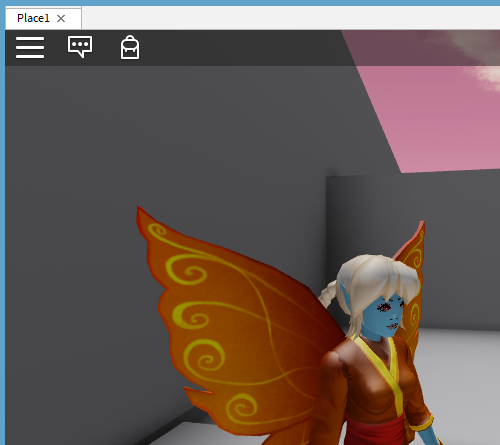
With the server up, you can check if the code worked.
Find the Server window with the green border. Check for the print statement called from the MatchManager script. Because there is a repeat loop, you'll see the same print statements repeating.
Once you're done testing, in any window, close the server by Cleanup button. This closes all Server and Client windows and takes you back to your normal Studio window.
Troubleshooting Tips
At this point, if the intended print statements didn't appear, try one of the following below.
- Check that functions like prepareGame() are in scope of the while true do loop.
- If the print from MatchManager didn't work, check some common troubleshooting with module scripts, like making sure that the MatchManager script is required in GameManager or that prepareGame() is added to that module's table.
Completed Scripts
Below are completed scripts to double check your work.
GameManager Script
-- Serviceslocal ServerStorage = game:GetService("ServerStorage")local Players = game:GetService("Players")-- Module Scriptslocal moduleScripts = ServerStorage:WaitForChild("ModuleScripts")local matchManager = require(moduleScripts:WaitForChild("MatchManager"))local gameSettings = require(moduleScripts:WaitForChild("GameSettings"))-- Main game loopwhile true dorepeatprint("Starting intermission")task.wait(gameSettings.intermissionDuration)until #Players:GetPlayers() >= gameSettings.minimumPlayersprint("Intermission over")task.wait(gameSettings.transitionTime)matchManager.prepareGame()end
MatchManager Script
local MatchManager = {}
function MatchManager.prepareGame()
print("Game starting!")
end
return MatchManager
GameSettings Script
local GameSettings = {}-- Game VariablesGameSettings.intermissionDuration = 5GameSettings.roundDuration = 10GameSettings.minimumPlayers = 2GameSettings.transitionTime = 5return GameSettings