控制結構可以有多於一個條件。關鍵字 else 和 elseif 可以創建在多種條件下應發生的額外情況。語法看起來像:
顯示 Creek和其他的語法
if isGreen thenprint("Go")elseif isYellow thenprint("Slow")elseif isPedestrians thenprint("Wait")elseprint("stop")end
在這個項目中, else 和 elseif 用於編寫一個公園賽道,賽手根據完成時間獲得獎勵。
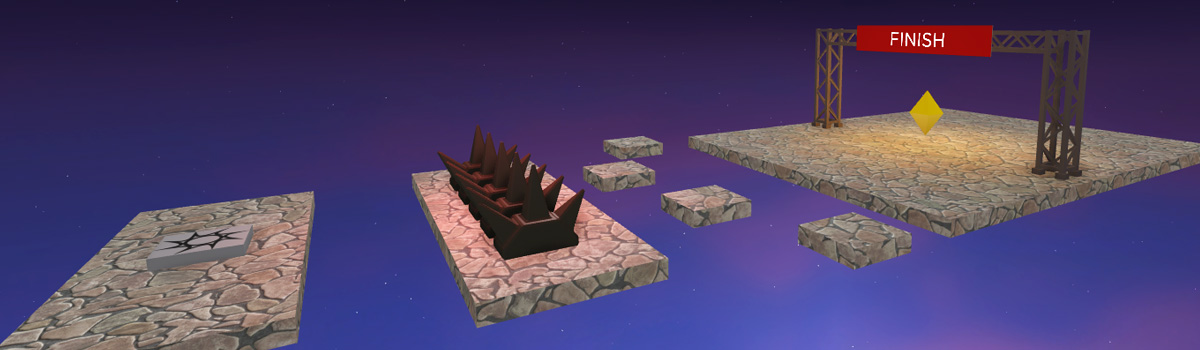
代碼多種條件
對於這個項目,您可以為每個獎牌寫一個獨特的 if 聲明來獎勵玩家,但這需要花很多時間。舉例來說,下面的想像代碼。
所有 if 聲明
if finishTime < 5 then-- 獲得一枚金牌endif finishTime >= 5 and <= 10 then-- 獲得銀牌endif finishTime > 10 and <= 15 then-- 獲得銅牌end
一種更容易閱讀和高效的方法來編寫此代碼是使用單個 if 聲明並使用關鍵字 elseif 來提供替代條件來測試以前的條件不是真實的。
使用 elseif 和 else
if finishTime < 5 then-- 獲得一枚金牌elseif finishTime >= 5 and <= 10 then-- 獲得銀牌elseif finishTime > 10 and <= 15 then-- 獲得銅牌end
當 if/然後文件運行時,它會從頂部開始運行,只運行找到的第一個 真實條件 的代碼。
設置競賽場地
首先放置課程的起點和終點,然後創建一個腳本來計時玩家並授予不同的獎牌。
創建一個名為「完成線」的錨定零件。為了加快測試速度,將開始和結束放在一起。您可以在完成腳指令碼後移動完成線。
完成零件 整個課程 在完成線中,插入名為 RaceManager 的腳本。然後,添加一個變量來儲存比賽開始時間已過多少秒以及一個第二變量來參考完成線。
local finishLine = script.Parentlocal timePassed = 0創建名為 finish() 的功能。
local timePassed = 0local finishLine = script.Parentlocal function finish()end自擁有的:
- 編寫名為 partTouched() 的功能,當玩家觸碰它時運行 finish() 。
- 將完成線零件連接到 partTouched() 。
- 將您的代碼與下面的範例進行比較。
local function finish()print("touched the finish line")endlocal function partTouched(otherPart)local character = otherPart.Parentlocal humanoid = character:FindFirstChildWhichIsA("Humanoid")if humanoid thenfinish()endendfinishLine.Touched:Connect(partTouched)測試並檢查 finish() 當您觸碰完成線時會被呼叫。
防止 finish() 重複
目前,每當玩家觸碰終點線,finish() 將持續呼叫,直到玩家觸碰零件。
使用 二元操作符 ,一個儲存真實或虛假的變量,以確保 finish() 只被呼叫一次。
創建一個新變量名為 raceActive 並將其設為 true 。
local timePassed = 0local finishLine = script.Parentlocal raceActive = true-- 每當玩家觸碰完成線部分時運行local function finish()將第二個條件添加到 if 聲明,以檢查賽車Active是否真實,然後才能呼叫 finish() 。
local function partTouched(otherPart)local character = otherPart.Parentlocal humanoid = character:FindFirstChildWhichIsA("Humanoid")if humanoid and raceActive == true thenfinish()endend若要停止 finish() 再次被呼叫,將 raceActive 設為 false 。
local function finish()print("touched the finish line")raceActive = falseend測試你的遊戲,確認你只看到你的測試列印聲明一次。
追蹤時間
像 if 聲明一樣,while 循環也可以使用條件來確定是否應該運執行。要時間玩家,使用 while true 循環創建一個計時器,只在 raceActive 否定符是真實的時運行。
在腳指令碼底部輸入 while raceActive == true do 。按一下 Enter 來自動完成並添加 end 。
finishLine.Touched:Connect(partTouched)while raceActive == true doend為了時間玩家,在循環中,每秒增加 1 到 timePassed 變量。包括打印聲明來測試你的工作。
finishLine.Touched:Connect(partTouched)while raceActive == true dotask.wait(1)timePassed += 1print(timePassed)end玩遊戲,並檢查您是否看到每秒鐘在輸出窗口中顯示。
授予玩家獎牌
為了完成,使用多個條件的 if 聲明來獎勵玩家不同的獎牌,根據他們的履約。使用一個 if 聲明和兩個 elseif 聲明來檢查玩家的完成時間並授予他們正確的獎牌。
要確認每個獎牌可以授予,請使用印刷聲明。
local function finish()raceActive = falseprint("You finished in " .. timePassed)end要授予一枚金牌,編寫一個 if 聲明,比較 timePassed 與所需的完成時間。這個例子檢查玩家的時間是否少於或等於 10 秒。
local function finish()raceActive = falseprint("You finished in " .. timePassed)if timePassed <= 10 thenprint("You get a gold medal!")endend測試並確認黃金獎牌可以授予。
添加額外條件
現在您已經測試了黃金獎牌,使用 elseif 關鍵字測試其他獎牌的代碼條件。
對於銀牌,使用 elseif 和下一個期望時間範圍。在這個例子中,範圍大於 10 秒,但小於或等於 20 秒。
local function finish()raceActive = falseprint("You finished in " .. timePassed)if timePassed <= 10 thenprint("You get a gold medal!")elseif timePassed > 10 and timePassed <= 20 thenprint("You get a silver medal!")endend使用相同的模式來獲得銅牌。請與以下示例檢查您的代碼。
local function finish()raceActive = falseprint("You finished in " .. timePassed)if timePassed <= 10 thenprint("You get a gold medal!")elseif timePassed > 10 and timePassed <= 20 thenprint("You get a silver medal!")elseif timePassed > 20 and timePassed <= 30 thenprint("You get a bronze medal!")endend為銀和銅牌進行測試。
排除故障提示
如果你沒有看到銀和銅金出現,試試以下之一。
- 每個 elseif 應該有一個然後在其條件之後。
- 在 partTouched() 中,確保 if 聲明的第二個條件使用 == , 如在 raceActive == true 中。
- 檢查每個 elseif 是否在範圍內。每個 elseif 條件必須位於 if/then 聲明的第一行和最後 end 之間。
添加其他條件
如果玩家沒有獲得任何獎牌,你應該鼓勵他們再試一次。在這種情況下,您可以使用 else 聲明,如果 沒有其他條件 是真實的,將顯示給他們一個訊息。
在最後的 elseif 和上面的 end 之下,開始新行並輸入 else 。 不要 在此添加。在其他情況下,使用印刷聲明來提示他們再試一次。
local function finish()raceActive = falseprint("You finished in " .. timePassed)if timePassed <= 10 thenprint("You get a gold medal!")elseif timePassed > 10 and timePassed <= 20 thenprint("You get a silver medal!")elseif timePassed > 20 and timePassed <= 30 thenprint("You get a bronze medal!")elseprint("Try again!")endend
2。播放測試以查看其他訊息。
總結
控制結構可以有多於一個情況。使用 if 聲明來設置初始聲明進行檢查,然後添加所需的 elseif 條件。最後,使用 else 來說明所有指定條件都是假的時應發生的事情。
從頂部開始,所有條件都會被檢查,只有第一個真實條件才會執行代碼。剩下的條件不會被檢查,也不會執行他們的代碼。
完成項目後,您可以擴展腳本以在幾種額外方式新增新元素。
- 添加代碼,讓玩家在完成時觸碰開始線即可重複比賽。
- 設計一種方式來在比賽期間顯示時間。您可以使用 Surface GUI 顯示零件上的時間,例如在 創建時間橋教學 中。
Completed script
local timePassed = 0
local finishLine = script.Parent
-- 用於在賽車結束時防止 finish() 和 timer 重複使用
local raceActive = true
-- 當玩家觸碰完成線時執行,並向他們展示獎品
local function finish()
raceActive = false
print("You finished in " .. timePassed)
if timePassed <= 10 then
print("You get a gold medal!")
elseif timePassed > 10 and timePassed <= 20 then
print("You get a silver medal!")
elseif timePassed > 20 and timePassed <= 30 then
print("You get a bronze medal!")
else
print("Try again!")
end
end
-- 檢查玩家是否在比賽激活時觸碰零件
local function partTouched(otherPart)
local character = otherPart.Parent
local humanoid = character:FindFirstChildWhichIsA("Humanoid")
if humanoid and raceActive == true then
finish()
end
end
finishLine.Touched:Connect(partTouched)
-- 在比賽活動期間跟蹤比賽時間。需要位於腳本底部。
while raceActive == true do
task.wait(1)
timePassed += 1
print(timePassed)
end