Most experiences let players use their own Roblox avatar, although some implement an in-experience customization system like the Merch Booth module. Other experiences make limited modifications to player avatars such as helmets, wings, or accessories that match the genre.
To create a unique experience that alters the appearance of your users, you can customize the default character properties with the following:
- Configure the Avatar Game Settings to set basic global avatar appearance defaults for all users.
- Use HumanoidDescription at any point to apply a wide-range of specific character customizations to one or more users in your experience.
Game Settings
The Avatar section in Game Settings menu allows you to quickly set several global character properties in your experience. When editing the Avatar Game Settings, your avatar displays in the workspace as a visual preview.
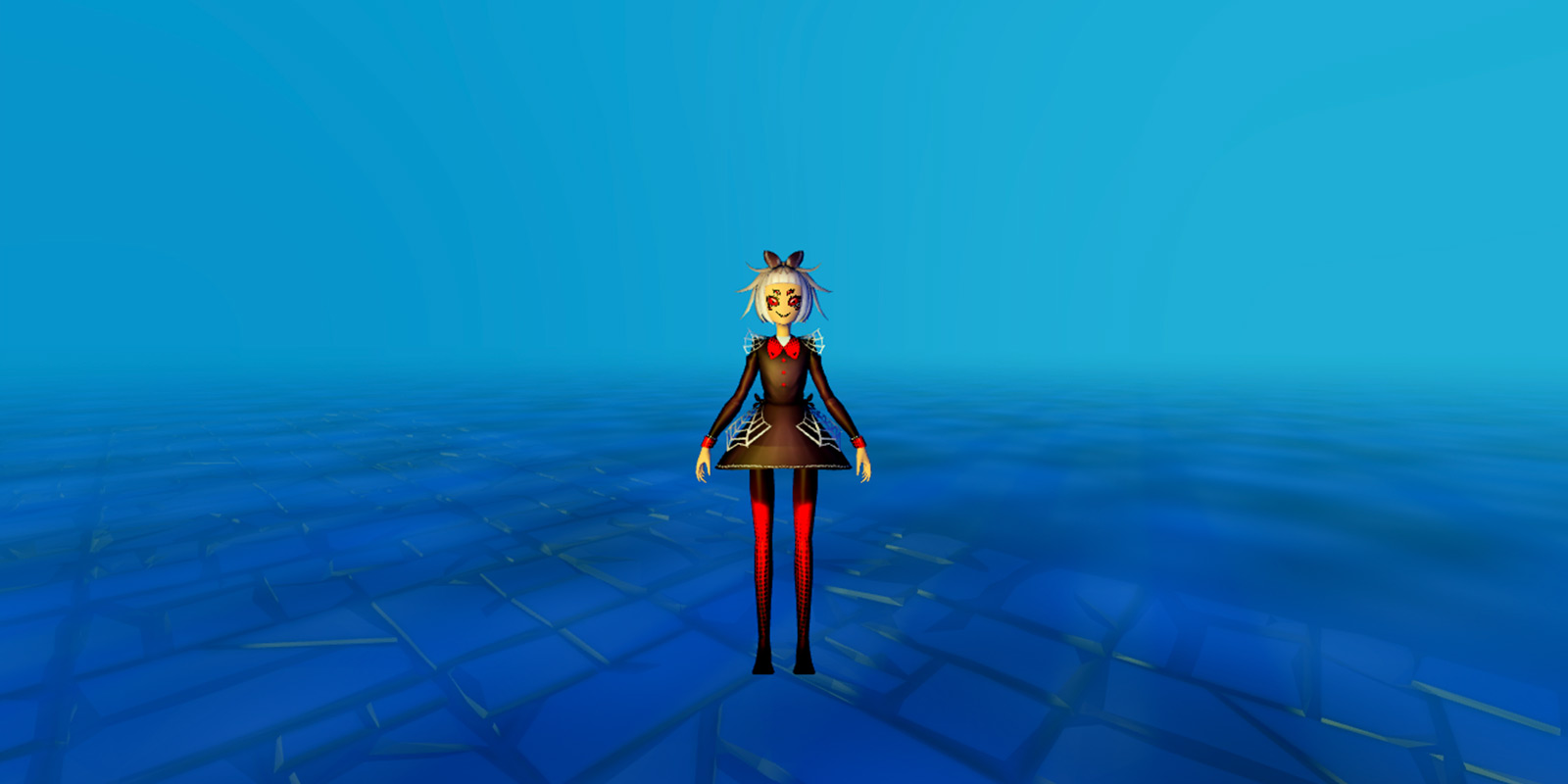
You can adjust the following character properties in your experience using the Avatar Game Settings:
Setting | Description |
---|---|
Presets | Applies a common set of BodyTypeScale and ProportionScale combinations. You can further adjust these properties with HumanoidDescription after selecting a preset. |
Avatar Type | Sets the default avatar type to either R15 or R6. |
Animation | The set of Animations a user has access to. |
Collision | Sets the collision boundaries for characters in the experience. |
Body Parts | The Asset IDs of the Face, Head, Torso, RightArm, LeftArm, RightLeg and LeftLeg parts of a character. |
Clothing | The Asset IDs of the classic Shirt, Pants, and ShirtGraphic image textures that you can apply to the character. |
Avatar Types
The Avatar Type setting sets your experience to only load R15 or R6 character models.
R15 is the default modern avatar with 15 limbs. This avatar allows for more flexible customization, accessory options, and animations.
R6 is a classic simple avatar with 6 limbs. This avatar type provides a retro feel but is limited in animations and additional customization. Changes to the body scale property do not affect R6 characters.
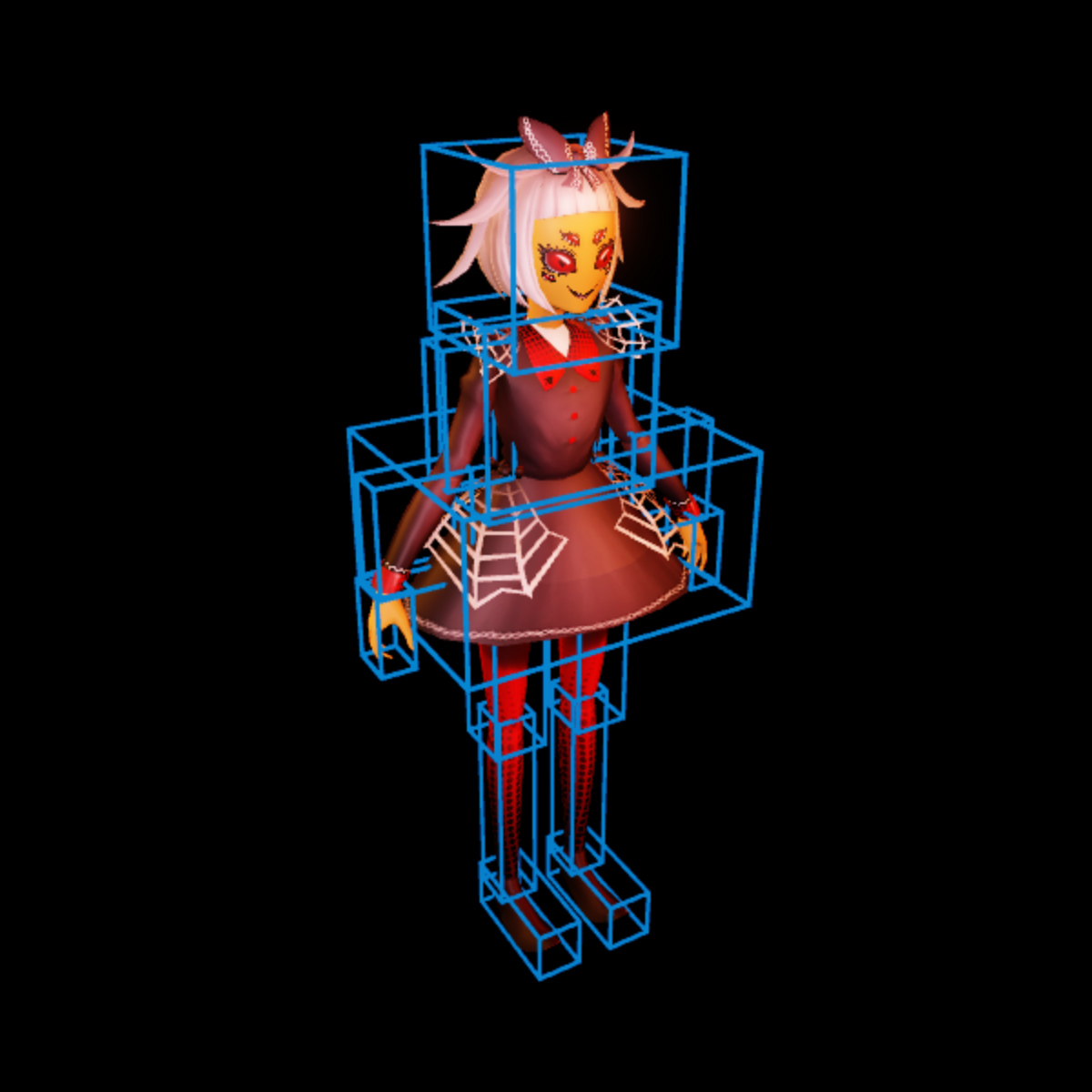
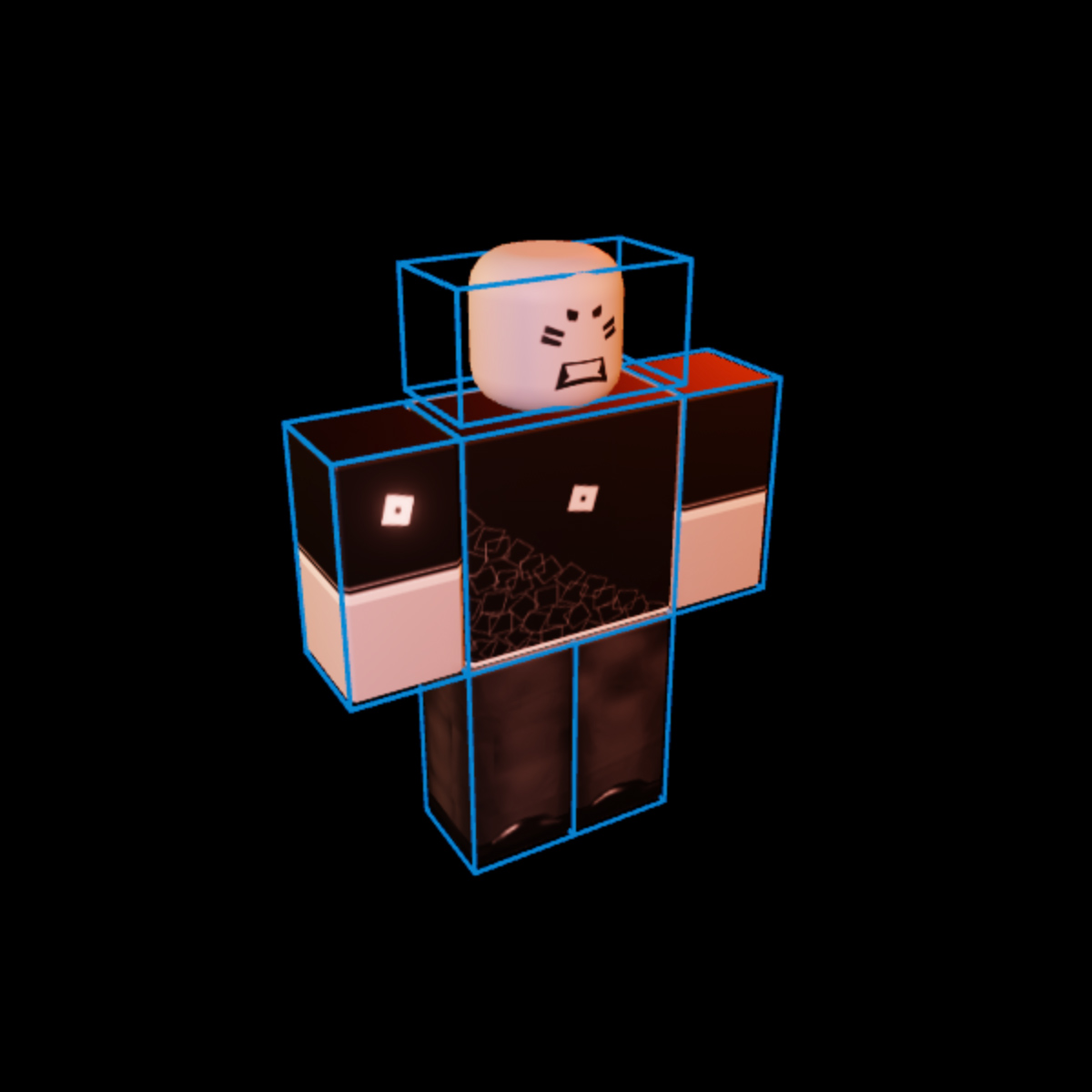
Collision Boundaries
The Collision setting sets the collision boundaries for characters in the experience. This doesn't impact the physical appearance of the characters in your experience.
Setting this option to Outer Box dynamically sizes the collision boxes of characters based on their individual models. This is the default and recommended setting for most experiences.
Setting this option to Inner Box provides fixed collision boundaries for all characters in your experience.
HumanoidDescription
Playable character models contain a Humanoid object that allows the model special characteristics, such as walking, jumping, equipping items, and interacting with the environment. To customize a Humanoid character's appearance, you can apply a new HumanoidDescription to change character properties.
You can adjust the following character properties in your experience using HumanoidDescription:
Character Property | Description |
---|---|
Scale | Number values for physical traits height, width, head, body type and proportion. This doesn't affect R6 body types. |
Accessories | The Asset IDs of accessories equipped by a character. |
Classic Clothing | The Asset IDs of the Shirt, Pants, and ShirtGraphic image textures that you can apply to the character. |
Body Part | The Asset IDs of the Face, Head, Torso, RightArm, LeftArm, RightLeg and LeftLeg parts of a character. |
Body Colors | The BodyColors of the character's individual parts. |
Animations | The Asset IDs of Animations you can use on a character. |
You can customize a character with HumanoidDescription using the following steps:
- Create a description from the user's character, a specific Outfit ID, or from a specific User ID.
- Modify the description to customize the properties that you want to apply to the Humanoid character.
- Apply the description on either a single character, all player characters, or even on all spawning characters.
Creating HumanoidDescription
You can create a new HumanoidDescription instance directly within the Explorer hierarchy or within a Script with the following code:
local humanoidDescription = Instance.new("HumanoidDescription")
In most cases, you should use an existing HumanoidDescription instead of a default new HumanoidDescription by referencing an existing player character, avatar outfit, or user ID.
From the Player Character
Use the following code sample to create a new HumanoidDescription based on the player character's current properties:
local humanoid = player.Character and player.Character:FindFirstChildWhichIsA("Humanoid")local humanoidDescription = Instance.new("HumanoidDescription")if humanoid thenhumanoidDescription = humanoid:GetAppliedDescription()end
From an Existing Outfit
Use the following sample code to create a HumanoidDescription from an outfit ID using Players.GetHumanoidDescriptionFromOutfitID:
local Players = game:GetService("Players")local outfitId = 480059254local humanoidDescriptionFromOutfit = Players:GetHumanoidDescriptionFromOutfitId(outfitId)
From a Specific User
Use the following sample code to create a HumanoidDescription from a user ID using Players:GetHumanoidDescriptionFromUserId():
local Players = game:GetService("Players")local userId = 491243243local humanoidDescriptionFromUser = Players:GetHumanoidDescriptionFromUserId(userId)
Modifying HumanoidDescription
To customize HumanoidDescription properties, set them directly on the HumanoidDescription or use a specified method before applying the HumanoidDescription to a character.
The following code sample provides examples of setting the different types of HumanoidDescription properties:
local humanoidDescription = Instance.new("HumanoidDescription")humanoidDescription.HatAccessory = "2551510151,2535600138"humanoidDescription.BodyTypeScale = 0.1humanoidDescription.ClimbAnimation = 619521311humanoidDescription.Face = 86487700humanoidDescription.GraphicTShirt = 1711661humanoidDescription.HeadColor = Color3.new(0, 1, 0)
Setting Multiple Accessories
For layered or bulk accessory changes, you can use HumanoidDescription:SetAccessories() to make accessory related updates. The following code sample adds a layered sweater and jacket in that order to a HumanoidDescription:
local humanoidDescription = Instance.new("HumanoidDescription")local accessoryTable = {{Order = 1,AssetId = 6984769289,AccessoryType = Enum.AccessoryType.Sweater},{Order = 2,AssetId = 6984767443,AccessoryType = Enum.AccessoryType.Jacket}}humanoidDescription:SetAccessories(accessoryTable, false)
Applying HumanoidDescription
Apply HumanoidDescription to specific Humanoid characters in your experience with Humanoid:ApplyDescription() or Humanoid.LoadCharacterWithHumanoidDescription.
On a Single Character
ApplyDescription() can target any Humanoid. Use the following code to add a new pair of sunglasses and a new torso to the player character:
local humanoid = player.Character and player.Character:FindFirstChildWhichIsA("Humanoid")if humanoid thenlocal descriptionClone = humanoid:GetAppliedDescription()descriptionClone.Torso = 86500008-- Multiple face accessory assets are allowed in a comma-separated stringdescriptionClone.FaceAccessory = descriptionClone.FaceAccessory .. ",2535420239"-- Apply modified "descriptionClone" to humanoidhumanoid:ApplyDescription(descriptionClone)end
On All Player Characters
Use the following sample code to apply a HumanoidDescription to all current players in the game:
local Players = game:GetService("Players")for _, player in Players:GetPlayers() dolocal humanoid = player.Character and player.Character:FindFirstChildWhichIsA("Humanoid")if humanoid then-- Create a HumanoidDescriptionlocal humanoidDescription = Instance.new("HumanoidDescription")humanoidDescription.HatAccessory = "2551510151,2535600138"humanoidDescription.BodyTypeScale = 0.1humanoidDescription.ClimbAnimation = 619521311humanoidDescription.Face = 86487700humanoidDescription.GraphicTShirt = 1711661humanoidDescription.HeadColor = Color3.new(0, 1, 0)humanoid:ApplyDescription(humanoidDescription)endend
On All Spawning Characters
Use the following sample code to set a specific HumanoidDescription for all spawning player characters:
local Players = game:GetService("Players")
-- Stop automatic spawning so it can be done in the "PlayerAdded" callback
Players.CharacterAutoLoads = false
local function onPlayerAdded(player)
-- Create a HumanoidDescription
local humanoidDescription = Instance.new("HumanoidDescription")
humanoidDescription.HatAccessory = "2551510151,2535600138"
humanoidDescription.BodyTypeScale = 0.1
humanoidDescription.ClimbAnimation = 619521311
humanoidDescription.Face = 86487700
humanoidDescription.GraphicTShirt = 1711661
humanoidDescription.HeadColor = Color3.new(0, 1, 0)
-- Spawn character with the HumanoidDescription
player:LoadCharacterWithHumanoidDescription(humanoidDescription)
end
-- Connect "PlayerAdded" event to "onPlayerAdded()" function
Players.PlayerAdded:Connect(onPlayerAdded)
If the HumanoidDescription instance was created in the Explorer and parented to the workspace, use the following sample code in a Script to access the workspace instance:
local Players = game:GetService("Players")
-- Stop automatic spawning so it can be done in the "PlayerAdded" callback
Players.CharacterAutoLoads = false
local function onPlayerAdded(player)
-- Spawn character with "workspace.StudioHumanoidDescription"
player:LoadCharacterWithHumanoidDescription(workspace.StudioHumanoidDescription)
end
-- Connect "PlayerAdded" event to "onPlayerAdded()" function
Players.PlayerAdded:Connect(onPlayerAdded)