bit32
This library provides functions to perform bitwise operations.
Number Limitations
This library treats numbers as unsigned 32-bit integers; numbers will be converted to this before being used (see image below). Numbers with decimal numbers are rounded to the nearest whole number.
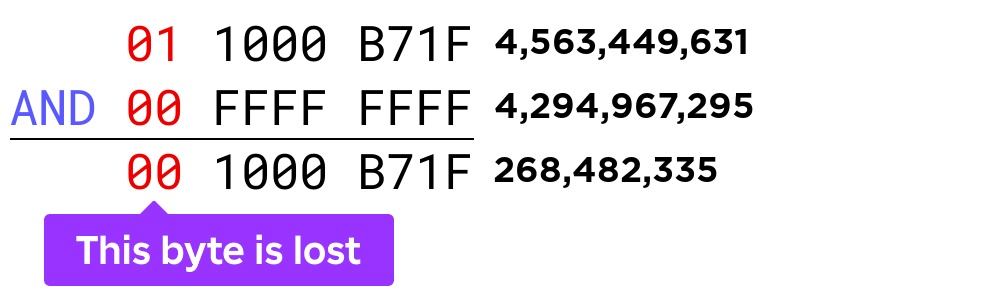
Summary
Functions
Returns a number after its bits have been arithmetically shifted to the right by a given displacement.
Returns the bitwise AND of all provided numbers.
Returns the bitwise negation of a given number.
Returns the bitwise OR of all provided numbers.
Returns a boolean describing whether the bitwise and of its operands is different from zero.
Returns the bitwise XOR of all provided numbers.
Returns the given number with the order of the bytes swapped.
Returns the number of consecutive zero bits in the 32-bit representation of the provided number starting from the left-most (most significant) bit.
Returns the number of consecutive zero bits in the 32-bit representation of the provided number starting from the right-most (least significant) bit.
Extract a range of bits from a number and return them as an unsigned number.
Return a copy of a number with a range of bits replaced by a given value.
Returns a number after its bits have been rotated to the left by a given number of times.
Returns a number whose bits have been logically shifted to the left by a given displacement.
Returns a number after its bits have been rotated to the right by a given number of times.
Returns a number whose bits have been logically shifted to the right by a given displacement.
Functions
arshift
Returns the number x shifted disp bits to the right. The number disp may be any representable integer. Negative displacements shift to the left.
This shift operation is what is called arithmetic shift. Vacant bits on the left are filled with copies of the higher bit of x; vacant bits on the right are filled with zeros. In particular, displacements with absolute values higher than 31 result in zero or 0xFFFFFFFF (all original bits are shifted out).
Parameters
Returns
extract
Returns the unsigned number formed by the bits field to field + width - 1 from n. Bits are numbered from 0 (least significant) to 31 (most significant). All accessed bits must be in the range [0, 31]. The default for width is 1.
Returns
lrotate
Returns the number x rotated disp bits to the left. The number disp may be any representable integer. For any valid displacement, the following identity holds:
assert(bit32.lrotate(x, disp) == bit32.lrotate(x, disp % 32))
In particular, negative displacements rotate to the right.
Returns
lshift
Returns the number x shifted disp bits to the left. The number disp may be any representable integer. Negative displacements shift to the right. In any direction, vacant bits are filled with zeros. In particular, displacements with absolute values higher than 31 result in zero (all bits are shifted out).
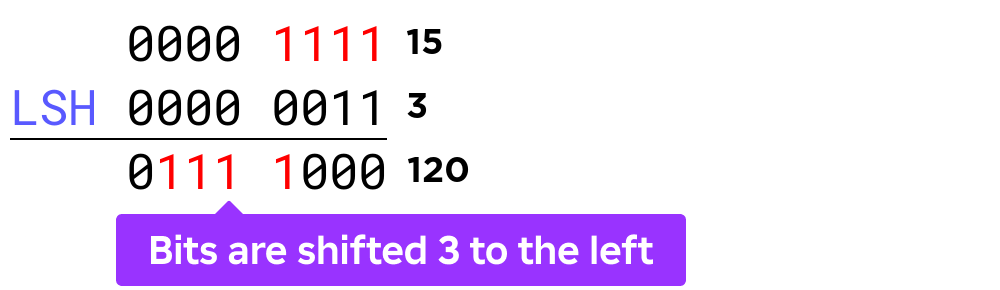
For positive displacements, the following equality holds:
assert(bit32.lshift(b, disp) == (b * 2^disp) % 2^32)
Returns
rrotate
Returns the number x rotated disp bits to the right. The number disp may be any representable integer.
For any valid displacement, the following identity holds:
assert(bit32.rrotate(x, disp) == bit32.rrotate(x , disp % 32))
In particular, negative displacements rotate to the left.
Returns
rshift
Returns the number x shifted disp bits to the right. The number disp may be any representable integer. Negative displacements shift to the left. In any direction, vacant bits are filled with zeros. In particular, displacements with absolute values higher than 31 result in zero (all bits are shifted out).
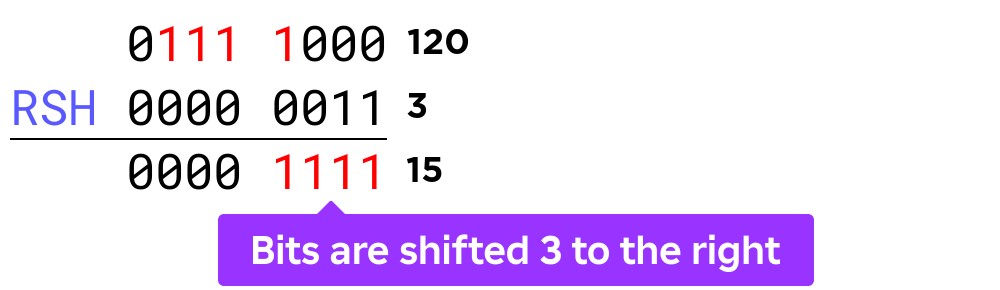
For positive displacements, the following equality holds:
assert(bit32.rshift(b, disp) == (b % 2^32 / 2^disp) // 1)
This shift operation is what is called logical shift.