A デバウンス パターンは、機能が複数回実行されたり、入力が複数回トリガーされたりするのを防ぐコード技術です。次のスクリプトのシナリオは、最良の実践として debounce を示しています。
衝突を検出
触れると 10 ダメージを与える危険なトラップパーツを作成したいとします。最初の実装は、基本の BasePart.Touched 接続とこのような damagePlayer 機能を使用する可能性があります:
スクリプト - ダメージプレイヤー
local part = script.Parent
local function damagePlayer(otherPart)
print(part.Name .. " collided with " .. otherPart.Name)
local humanoid = otherPart.Parent:FindFirstChildWhichIsA("Humanoid")
if humanoid then
humanoid.Health -= 10 -- プレイヤーの体力を減少する
end
end
part.Touched:Connect(damagePlayer)
最初は論理的に見えても、テストでは Touched イベントが、微細な物理的衝突に基づいて迅速に連続して複数回発動することを示します。
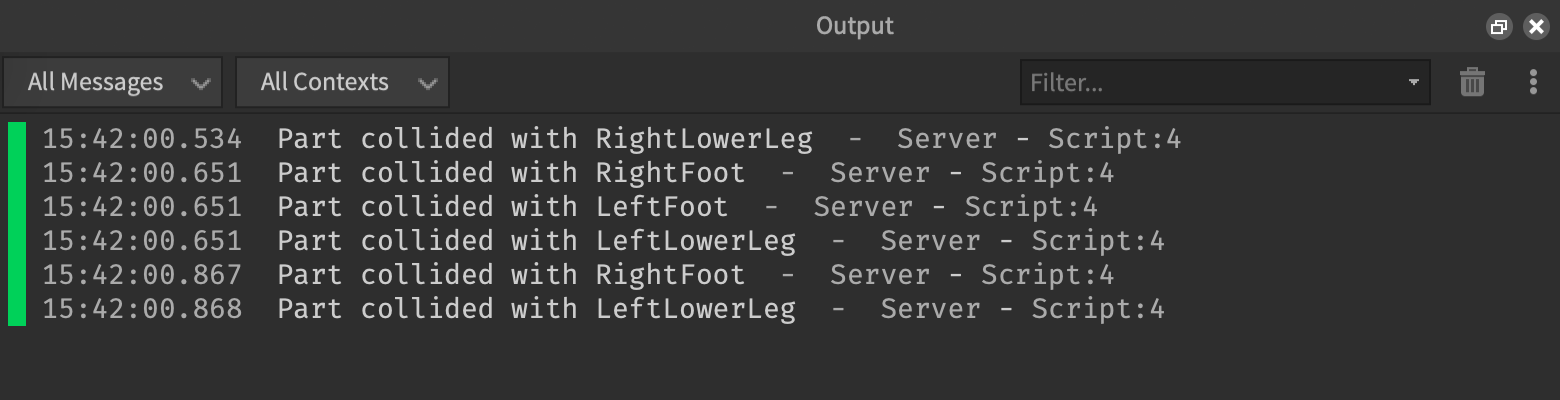
初期接連絡で過剰なダメージを避けるために、インスタンス属性 を通じてダメージにクールダウン期間を課すデバウンスシステムを追加できます。
スクリプト - Debounce を使用してプレイヤーにダメージを与える
local part = script.Parent
local RESET_TIME = 1
local function damagePlayer(otherPart)
print(part.Name .. " collided with " .. otherPart.Name)
local humanoid = otherPart.Parent:FindFirstChildWhichIsA("Humanoid")
if humanoid then
if not part:GetAttribute("Touched") then
part:SetAttribute("Touched", true) -- 属性を真に設定
humanoid.Health -= 10 -- プレイヤーの体力を減少する
task.wait(RESET_TIME) -- リセット時間を待つ
part:SetAttribute("Touched", false) -- 属性をリセット
end
end
end
part.Touched:Connect(damagePlayer)
トリガーサウンド
デバウンスは、2つのパーツが衝突するときにサウンドを再生する(Touched)、またはユーザーが画面上のボタンと対話するときにサウンドを再生するActivatedイベントでも有用です。両方の場合、Sound:Play() を呼び出すと、トラックの最初から再生が開始され、デバウンスシステムなしでは、サウンドが素早く連続して複数回再生する可能性があります。
サウンドの重複を防ぐには、IsPlaying オブジェクトの Sound プロパティを使用してデバウンスできます:
スクリプト - Debounce を使用してコリジョンサウンドを再生
local projectile = script.Parent
local function playSound()
-- パーツで子音を見つける
local sound = projectile:FindFirstChild("Impact")
-- すでに再生されていない場合にのみサウンドを再生する
if sound and not sound.IsPlaying then
sound:Play()
end
end
projectile.Touched:Connect(playSound)
スクリプト - debounce を使用したプレイボタンクリック
local button = script.Parent
local function onButtonActivated()
-- ボタンで子音を見つける
local sound = button:FindFirstChild("Click")
-- すでに再生されていない場合にのみサウンドを再生する
if sound and not sound.IsPlaying then
sound:Play()
end
end
button.Activated:Connect(onButtonActivated)
ピックアップ効果
エクスペリエンスには、医療キット、弾薬パックなどの 3D 世界のコレクションがしばしば含まれています。これらのピックアップを、プレイヤーが再び再び掴むために世界に残すように設計する場合、ピックアップのリフレッシュと再起動の前に「クールダウン」時間が追加される必要があります。
衝突を検出する と同様に、インスタンス属性 でデバウンス状態を管理し、パーツの Transparency を変更してクールダウン期間を視覚化できます。
スクリプト - Debounce を使用したヘルスピックアップ
local part = script.Parent
part.Anchored = true
part.CanCollide = false
local COOLDOWN_TIME = 5
local function healPlayer(otherPart)
local humanoid = otherPart.Parent:FindFirstChildWhichIsA("Humanoid")
if humanoid then
if not part:GetAttribute("CoolingDown") then
part:SetAttribute("CoolingDown", true) -- 属性を真に設定
humanoid.Health += 25 -- プレイヤーの健康を増やす
part.Transparency = 0.75 -- クールダウン状態を示すためにパーツを半透明にする
task.wait(COOLDOWN_TIME) -- クールダウン期間を待つ
part.Transparency = 0 -- パーツを完全に不透明にリセット
part:SetAttribute("CoolingDown", false) -- 属性をリセット
end
end
end
part.Touched:Connect(healPlayer)