友達紹介システムは、既存のプレイヤーがあなたのエクスペリエンスに新しいプレイヤーを紹介することを促し、プレイヤーの継続と全体的なエンゲージメントを増加させます。プレイヤーは、player invite prompts から直接、またはデフォルトのインエクスペリエンス招待メニューから、紹介リンクをアクセスして共有できます。
開発者として、これらの共有可能なリファレンスリンクを使用して以下を行うことができます:
- 他のプレイヤーをエクスペリエンスに成功して招待したプレイヤーを追跡します。
- 他のプレイヤーからの紹介リンクの招待を使用して、どのプレイヤーがあなたのエクスペリエンスに参加したかを追跡します。
- 招待者と招待者の両方に報酬を作成し、配信する。
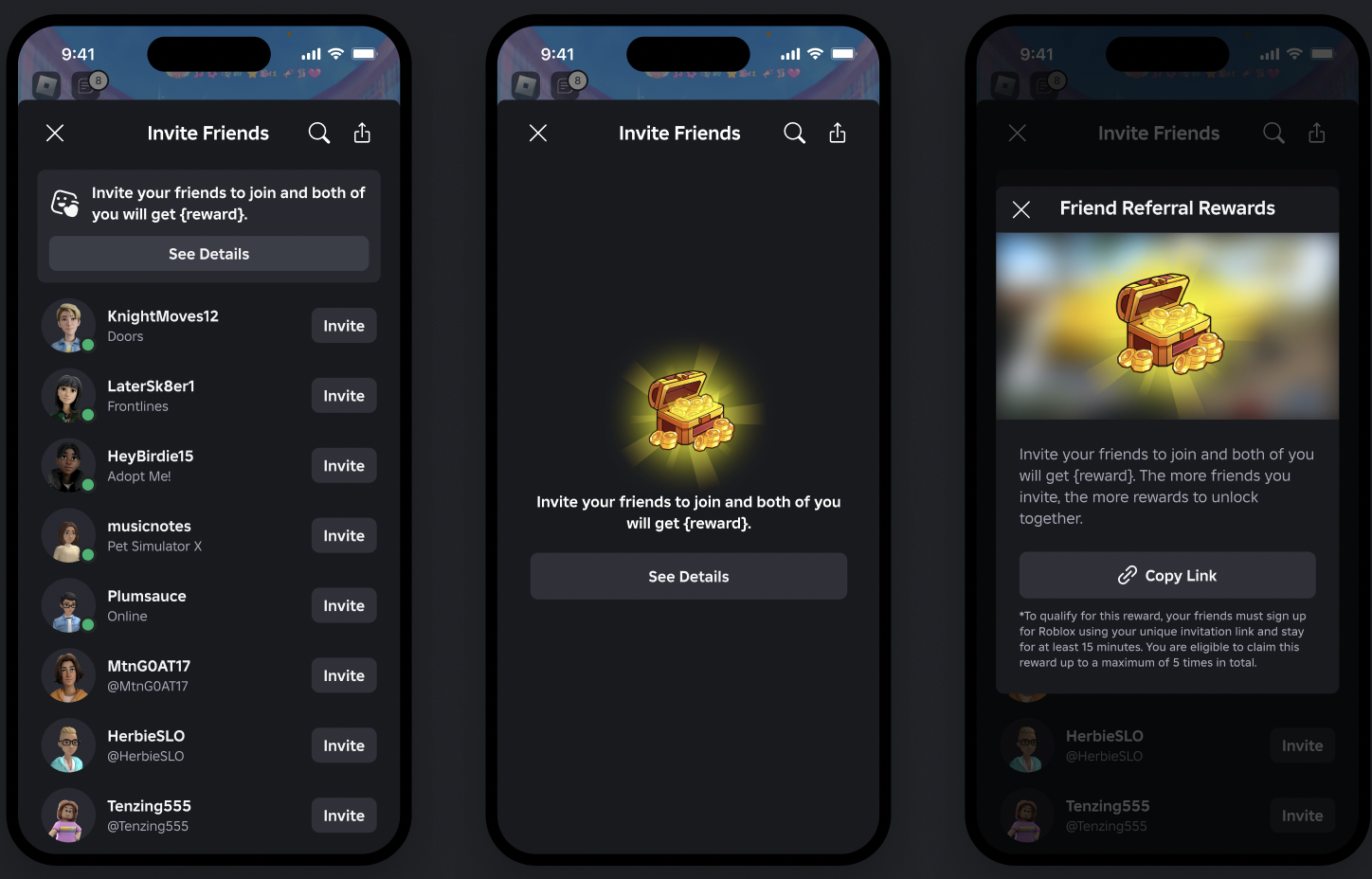
友達紹介システムを実装するには、紹介イベントを設定し、紹介報酬を作成します。ReferredByPlayerId
function onPlayerAdded(player)
local referredByPlayerId = player:GetJoinData().ReferredByPlayerId
local referrerEvent: RemoteEvent = ReplicatedStorage:FindFirstChild("ReferralReceivedEvent")
referrerEvent:FireClient(player, referredByPlayerId)
end
Players.PlayerAdded:Connect(onPlayerAdded)
紹介イベントを設定する
紹介イベントを設定するには:
- ReplicatedStorage に ReplicatedStorage を設定して、クライアントに紹介を受信すると、リモートイベントを通信するためにクライアントと接続する RemoteEvent を作成します。
- ReferredByPlayerId を使用して、Players.PlayerAdded イベント中のサーバー側スクリプトでプレイヤーが参加し、ReferredByPlayerId ロジックを処理するために、参照されたサーバー側スクリプトでプレイヤーのユーザー ID を取得します。
local Players = game:GetService("Players")
local ReplicatedStorage = game:GetService("ReplicatedStorage")
-- 紹介を処理するためにリモートイベントを作成または取得
local referrerEvent: RemoteEvent = ReplicatedStorage:FindFirstChild("ReferralReceivedEvent")
-- プレイヤーが参加するときにトリガーする機能
function onPlayerAdded(player)
local joinData = player:GetJoinData()
local referredByPlayerId = joinData.ReferredByPlayerId
-- 紹介を通じてプレイヤーが招待されているかどうかをチェックします
if referredByPlayerId then
-- クライアントに紹介イベントを発動し、招待者のID を通過
referrerEvent:FireClient(player, referredByPlayerId)
-- 招待者と招待者を報酬付与する追加のロジックはここに追加できます
-- e.g.、rewardReferrer(referredByPlayerId)
-- e.g.、rewardInvitee(playeプレイヤー)
end
end
-- PlayerAdded イベントに関数を接続する
Players.PlayerAdded:Connect(onPlayerAdded)
グラントリファラル報酬
参加を促進するために、招待者と招待者の両方に報酬を与えます。たとえば、友達がエクスペリエンスに参加すると、招待者にバッジやエクスペリエンス中の通貨を与えることができます。また、紹介リンクを通じてエクスペリエンスに参加すると、招待者にウェルカム報酬を提供することもできます。
local Players = game:GetService("Players")
local ReplicatedStorage = game:GetService("ReplicatedStorage")
-- 紹介を処理するためにリモートイベントを作成または取得
local referrerEvent: RemoteEvent = ReplicatedStorage:FindFirstChild("ReferralReceivedEvent")
-- プレイヤーが参加するときにトリガーする機能
function onPlayerAdded(player)
local joinData = player:GetJoinData()
local referredByPlayerId = joinData.ReferredByPlayerId
-- 紹介を通じてプレイヤーが招待されているかどうかをチェックします
if referredByPlayerId then
-- クライアントに紹介イベントを発動し、招待者のID を通過
referrerEvent:FireClient(player, referredByPlayerId)
-- 招待者に報酬を与える
function rewardReferrer(referrerId)
local referrerPlayer = Players:GetPlayerByUserId(referrerId)
if referrerPlayer then
-- 招待者に報酬を与える
-- 例: referrerPlayer.leaderstats.Coins.Value + 100
end
end
-- 招待者に報酬を与える
function rewardInvitee(player)
-- 招待者に報酬を与える
-- 例: プレイヤーlayer.leaderstats.WelcomeBonus.Value + 50
end
end
end
-- PlayerAdded イベントに関数を接続する
Players.PlayerAdded:Connect(onPlayerAdded)
不正行為の防止を管理
友達紹介システムを悪用するプレイヤーを防止するために、安全対策を実装できます。
- 一度きりの報酬を提供して、招待者を追跡し、1回だけ報酬を受け取ることを確認してください。
- 招待者が別の参照を送信する前に、クールダウン期間を紹介してください。
- 異常なアクティビティを監視し、ユーザーを禁止するなど、正常化メジャーを実装します。
-- 既に紹介されたプレイヤーを追跡するテーブル
local referredPlayers = {}
function onPlayerAdded(player)
local joinData = player:GetJoinData()
local referredByPlayerId = joinData.ReferredByPlayerId
-- プレイヤーが招待されているかどうか、またはすでに参照を使用していないかをチェックします
if referredByPlayerId and not referredPlayers[player.UserId] then
-- 参照したプレイヤーをマーク
referredPlayers[player.UserId] = true
-- 報酬の招待と招待者
rewardReferrer(referredByPlayerId)
rewardInvitee(player)
end
end