The Avatar Editor Service lets you access and make changes to a user's avatar within an experience. The Avatar Editor Service can also access a user's inventory and the Marketplace to save outfits and purchase avatar items to the user's account.
We recommend implementing the Avatar Editor Service with an in-game avatar editor for a complete character customization experience. See the Simple Avatar Editor Demo reference place for an example of this feature.
To begin using the Avatar Editor Service, you must first request access to the user's inventory. After access is successfully granted, you can perform the following actions:
- Read user's inventory to get a list of items owned by the user.
- Search the Marketplace, using a variety of properties to filter and sort.
- Equip avatar items and save outfits to the user's avatar.
- Prompt the user to purchase an Marketplace item.
Requesting Access
To begin accessing a user's inventory, you need to prompt the user to allow access through PromptAllowInventoryReadAccess(). You need to perform this request once per session.
Use the following code sample to initiate the access prompt and listen for the user response:
local AvatarEditorService = game:GetService("AvatarEditorService")AvatarEditorService:PromptAllowInventoryReadAccess()local result = AvatarEditorService.PromptAllowInventoryReadAccessCompleted:Wait()if result == Enum.AvatarPromptResult.Success then-- Access granted!end
The user receives the following prompt:
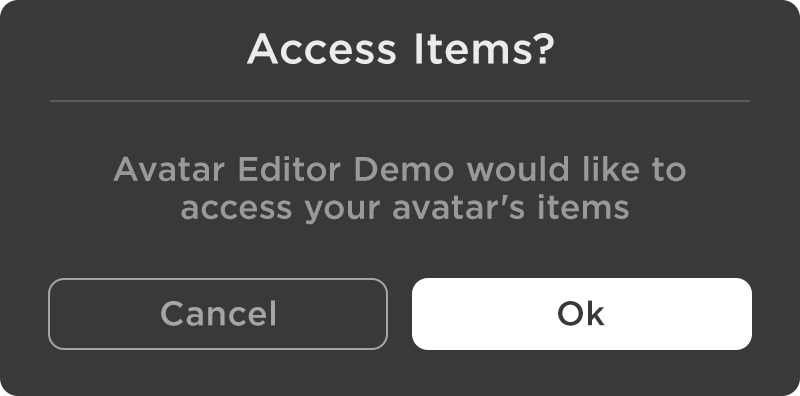
Once the user accepts the prompt, the AvatarEditorService can begin accessing the user's inventory.
Reading User Inventory
Once access is granted by the user, you can read their inventory with the GetInventory() function, supplying an array of AvatarAssetTypes to filter by. This function returns an InventoryPages object containing the user owned items.
Use the following code sample to print a list of specific accessories in a user's inventory:
local AvatarEditorService = game:GetService("AvatarEditorService")AvatarEditorService:PromptAllowInventoryReadAccess()local result = AvatarEditorService.PromptAllowInventoryReadAccessCompleted:Wait()if result == Enum.AvatarPromptResult.Success then-- Access granted!local assetTypes = {Enum.AvatarAssetType.BackAccessory,Enum.AvatarAssetType.ShoulderAccessory,Enum.AvatarAssetType.WaistAccessory}local pagesObject = AvatarEditorService:GetInventory(assetTypes)local currentPage = pagesObject:GetCurrentPage()for _, item in currentPage doprint(item)endend
Searching the Marketplace
AvatarEditorService includes functions and events which let you search the Roblox catalog. To search, supply your query with a CatalogSearchParams object that includes one or more of the following properties:
Property | Description |
---|---|
AssetTypes | An array of Enum.AvatarAssetType such as Enum.AvatarAssetType.BackAccessory. |
BundleTypes | An array of Enum.BundleType such as Enum.BundleType.BodyParts. |
CategoryFilter | A Enum.CatalogCategoryFilter describing the various catalog categories like "Featured" or "Community Creations". By default this is set to Enum.CatalogCategoryFilter.None |
MaxPrice | An integer describing the maximum price to filter. |
MinPrice | An integer describing the minimum price to filter. By default, MinPrice is 0. |
SearchKeyword | A string to query against item descriptions in the catalog. |
SortType | A Enum.CatalogSortType that describes how the results are ordered. By default this is set to Enum.CatalogSortType.Relevance. |
IncludeOffSale | A boolean describing whether the results of the search include off sale items. By default this is set to false. |
CreatorId | An integer to specify a given creator. You can use either a UserId or a GroupId. |
CreatorName | A string used to search by items created by a given creator. You can use either a User Name or a Group Name. |
The following code sample constructs a CatalogSearchParams object for Back and Shoulder asset types, and passes that through a SearchCatalog() call:
local AvatarEditorService = game:GetService("AvatarEditorService")local catalogSearchParams = CatalogSearchParams.new()local assetTypes = {Enum.AvatarAssetType.BackAccessory,Enum.AvatarAssetType.ShoulderAccessory}catalogSearchParams.AssetTypes = assetTypeslocal pagesObject =--This function returns a CatalogPages object containing the results.AvatarEditorService:SearchCatalog(catalogSearchParams)local currentPage = pagesObject:GetCurrentPage()for _, item in currentPage doprint(item)end
Saving Avatars and Outfits
When used alongside an in-game avatar editor, AvatarEditorService can save and update avatar items and outfits to the Roblox platform. Users don't receive catalog items they don't own when saving an avatar or outfit.
Any HumanoidDescription can be saved to the user's current avatar with PromptSaveAvatar(). This may include:
- Pre-defined avatar configurations that you've built using existing catalog items.
- Any configuration that the user has chosen through an in-game avatar editor.
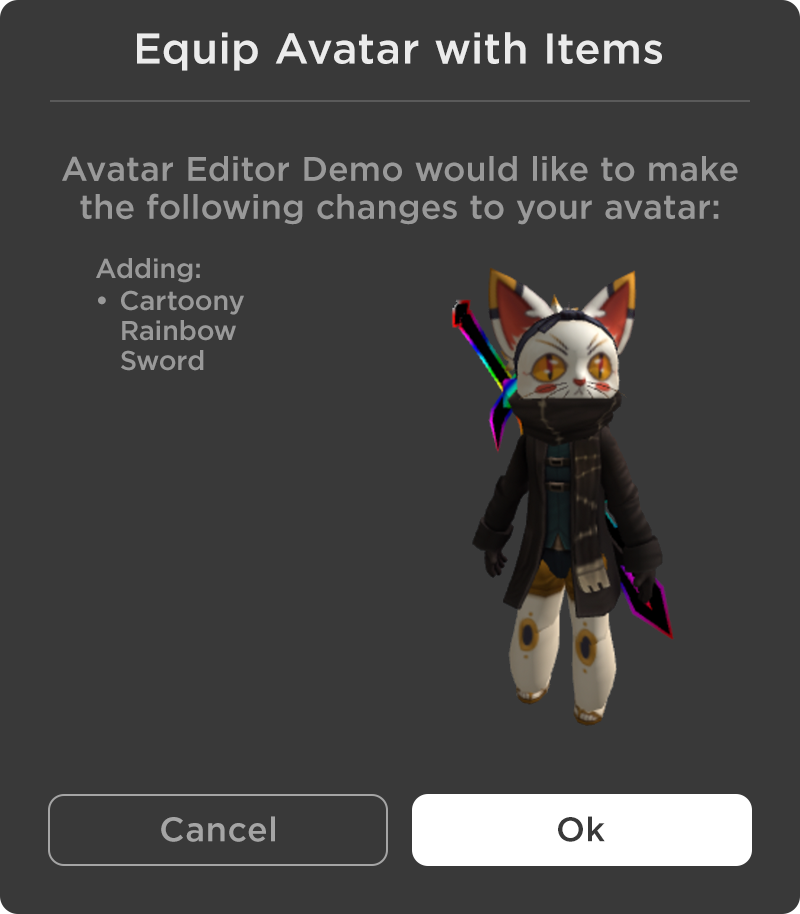
Since AvatarEditorService:PromptSaveAvatar() does not yield, you can get the result by listening to the AvatarEditorService.PromptSaveAvatarCompleted event.
The following code will save a current HumanoidDescription using PromptSaveAvatar() and checks for a successful AvatarEditorService.PromptSaveAvatarCompleted event:
local AvatarEditorService = game:GetService("AvatarEditorService")local Players = game:GetService("Players")local player = Players.LocalPlayerlocal character = player.Character or player.CharacterAdded:Wait()local humanoid = character:WaitForChild("Humanoid")local currentDescription = humanoid:GetAppliedDescription()AvatarEditorService:PromptSaveAvatar(currentDescription, humanoid.RigType)local result = AvatarEditorService.PromptSaveAvatarCompleted:Wait()if result == Enum.AvatarPromptResult.Success then-- Avatar saved!end
To save any HumanoidDescription as an outfit (without overwriting the user's current avatar), use AvatarEditorService:PromptCreateOutfit().
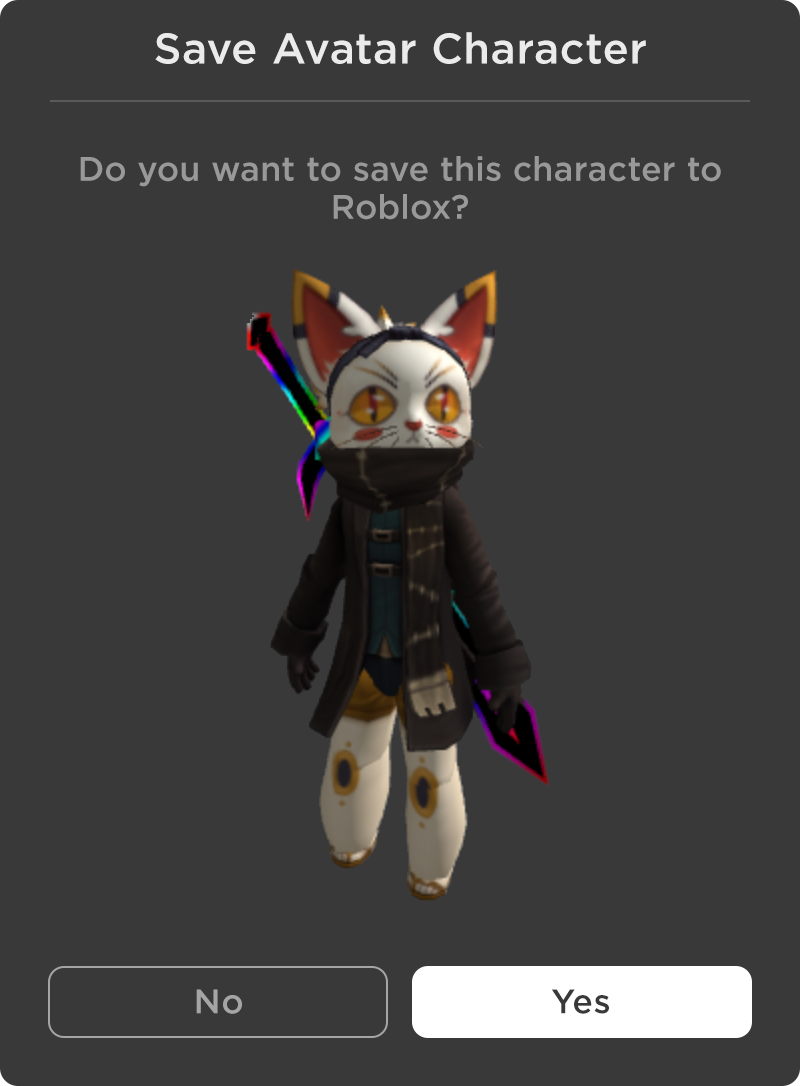
Once called, you can get the result of AvatarEditorService:PromptCreateOutfit() by listening to the AvatarEditorService.PromptCreateOutfitCompleted event.
The following code sample creates an outfit with AvatarEditorService:PromptCreateOutfit() and listens for a successful AvatarEditorService.PromptCreateOutfitCompleted event:
local AvatarEditorService = game:GetService("AvatarEditorService")local Players = game:GetService("Players")local player = Players.LocalPlayerlocal character = player.Character or player.CharacterAdded:Wait()local humanoid = character:WaitForChild("Humanoid")local currentDescription = humanoid:GetAppliedDescription()AvatarEditorService:PromptCreateOutfit(currentDescription, humanoid.RigType)local result = AvatarEditorService.PromptCreateOutfitCompleted:Wait()if result == Enum.AvatarPromptResult.Success then-- Outfit saved!end
Purchasing Items
When saving either an avatar or an outfit that uses catalog items, the user doesn't receive any items that they do not own. Before saving an avatar or outfit, check if the user owns the asset with MarketplaceService:PlayerOwnsAsset() and provide them with an option to purchase the item with MarketplaceService:PromptPurchase().
If you don't wish to implement item purchases, you can instead allow users to favorite non-owned items with AvatarEditorService:PromptSetFavorite().