Properties control how objects look and function. Each object in Roblox Studio has its own set of properties. For example, a part object has color, size, and shape properties. Properties can be changed in the Properties window or through code.
To learn about properties, you'll explore common properties found in parts and then write a script to change part colors.
The Properties Window
The Properties window can be used to learn about an object's properties. Use it to take a look at a part's properties.
Select a part.
In the Properties Window on the bottom-right, look at the different properties that can be changed, like color, size, material and transparency. You can also change most properties in this window from within a script.
If you don't see the Properties window, go to the View tab and click the Properties button.
Adding Comments to Scripts
Comments are special lines starting with -- that help coders remember what parts of scripts do. Unlike other code, comments don't run; they're just there so you can leave notes to yourself and other programmers. This script will change a part's Color property at the start of the game.
Select an existing part or create a new one. Rename the part PracticePart.
In ServerScriptService, create a new script named ChangeColor.
In the script, delete the default code. Then, write a comment by typing -- and a brief description of what the script will do.
Describes what the script does-- Changes the color of a part
Locating the Part
To make changes to a part, you must be able to describe the part's location. The Explorer is an excellent tool for referencing locations. In this case, PracticePart is under Workspace.
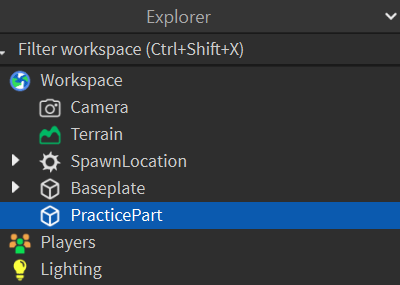
Now that you know where the part is, the part's location needs to be translated into something a script can understand.
Under the comment, type workspace.PracticePart.
References PracticePart in Workspace-- Changes the color of a partworkspace.PracticePart
Changing a Property with Code
You'll use an RGB value to change the part's color. Computers use RGB values, the combination of red, green, and blue, to create all the colors on your screen.
RGB values use three numbers from 0 to 255, separated by commas. For example, black is (0, 0, 0) while white is (255, 255, 255).
For the part, the script will change its Color property to a new Color3, a data type that stores colors.
After PracticePart, type .Color to access the Color property.
Accesses the Color property-- Changes the color of a partworkspace.PracticePart.ColorNext, type = Color3.fromRGB() This code will allow you to assign a new color.
Uses Color3.fromRGB()-- Changes the color of a partworkspace.PracticePart.Color = Color3.fromRGB()RGB color values can be manually typed inside the parentheses, but using the color picker is easier. Click inside the parentheses, and then click the color wheel. Follow the popup to create a color.
Your code should look similar to the code below.
Updates the color of PracticePart-- Changes the color of a partworkspace.PracticePart.Color = Color3.fromRGB(255, 230, 50)Press Play to test that your part changes color.
Summary
All objects have properties. Parts have properties like color and transparency. At the same time, other object types have their unique properties.
To change the color of a part, you need to be able to describe where to find it. If the part is in Workspace, use the keyword workspace. Then use dot operators to state the desired part and access its properties.