By utilizing appearance modifiers, you can further customize the appearance of your GuiObjects.
- Apply a gradient to the background of an object.
- Apply a stroke to text or a border.
- Set rounded corners for an object.
- Increase padding between the borders of an object.
Gradient
The UIGradient object applies a color and transparency gradient to its parent GuiObject.
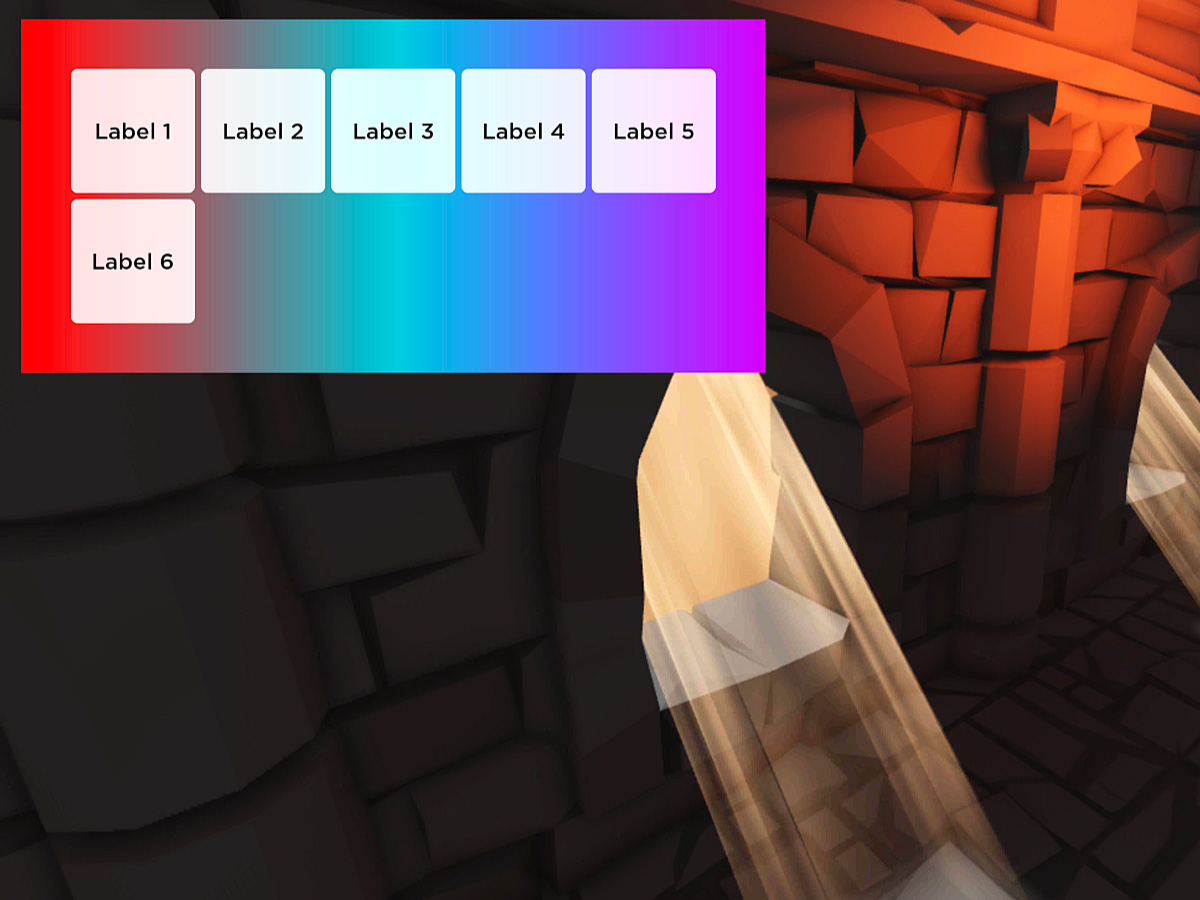
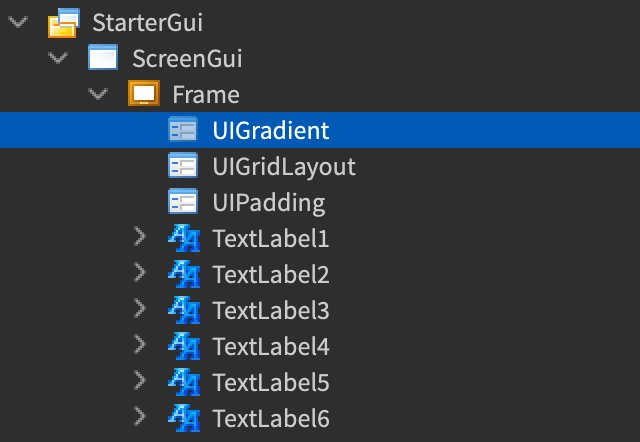
You can configure the gradient by:
- Choosing the gradient's starting point (inside or outside the parent's bounds) through the Offset property.
- Choosing the gradient's angle through the Rotation property.
Color sequence
To set a gradient's color sequence:
In the Explorer window, select the UIGradient.
In the Properties window, click inside the Color property field, then click the … button to the right of the input box. A color sequence pop-up displays.
Each triangle on the bottom axis of the color sequence is a keypoint that determines the color value at that point.
Click a keypoint in the color sequence, then click the small square next to Color to open the Colors pop-up window.
Select the desired color for the keypoint.
If needed, you can:
- Add another keypoint by clicking anywhere on the graph.
- Drag an existing keypoint to a new position, or select a keypoint and enter a specific time value through the Time input.
- Delete a keypoint by selecting it and clicking the Delete button.
- Reset the sequence by clicking the Reset button.
Transparency
To adjust a gradient's transparency across its range:
In the Explorer window, select the UIGradient.
In the Properties window, click inside the Transparency property field, then click the … button to the right of the input box. A number sequence pop-up displays.
Each square across the number sequence graph is a keypoint that determines the transparency value at that point.
Click and drag any keypoint around, or select a keypoint and enter a specific time/value combination through the Time and Value inputs.
If needed, you can:
- Add another keypoint by clicking anywhere on the graph.
- Delete a keypoint by selecting it and clicking the Delete button.
- Reset the sequence by clicking the Reset button.
Offset and rotation
The Offset and Rotation properties let you adjust the gradient's control points and its angle. As illustrated in the following diagrams, Offset is based on a percentage of the parent's width or height, and both positive or negative values are valid.
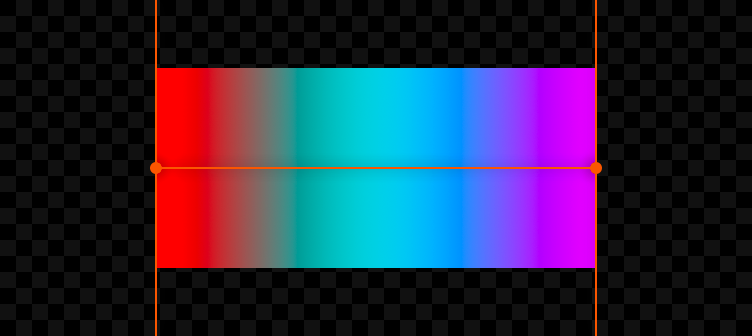
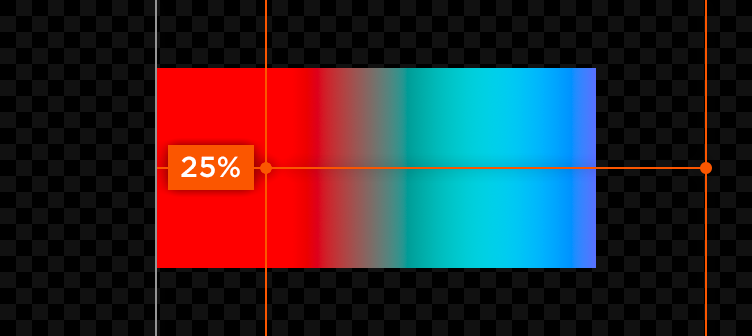
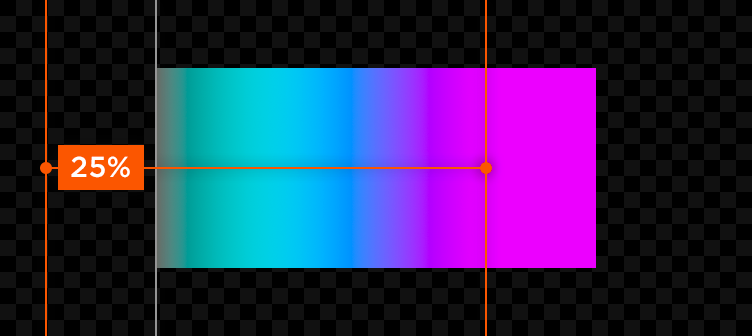
Similarly, when you rotate the gradient, the control points also rotate.
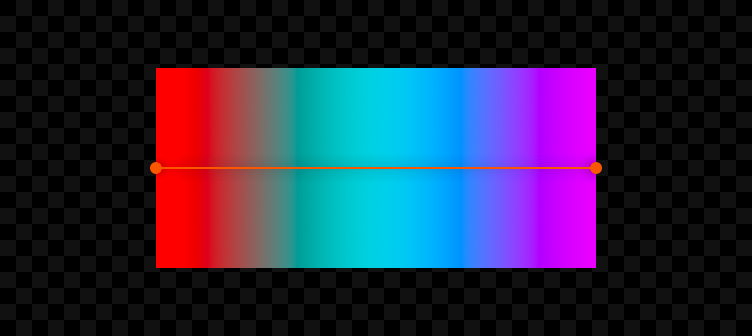
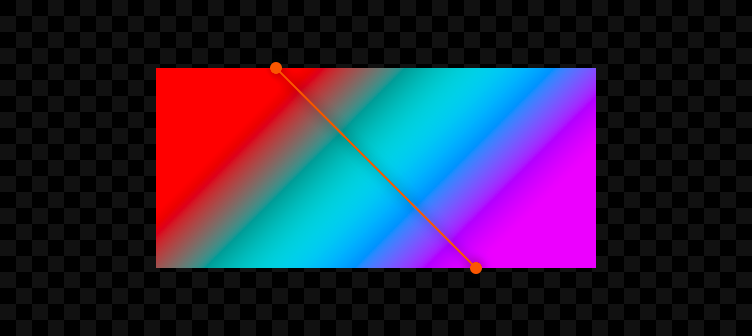
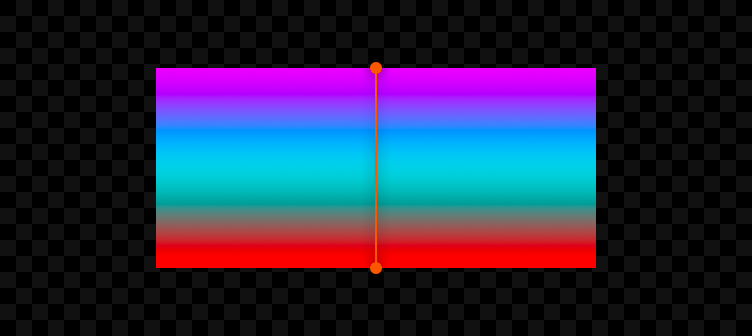
Stroke
The UIStroke instance applies an outline to text or a border. Key features include:
- Ability to set the stroke transparency independently of the parent's transparency.
- Three corner styles (round, bevel, or miter).
- Stroke gradient support through the UIGradient instance.
Text outline / border
Depending on its parent, UIStroke operates as either a text outline or as a border. When you parent UIStroke to a text object, it applies to the text's outline; when you parent UIStroke to other GuiObjects, it applies to the border.
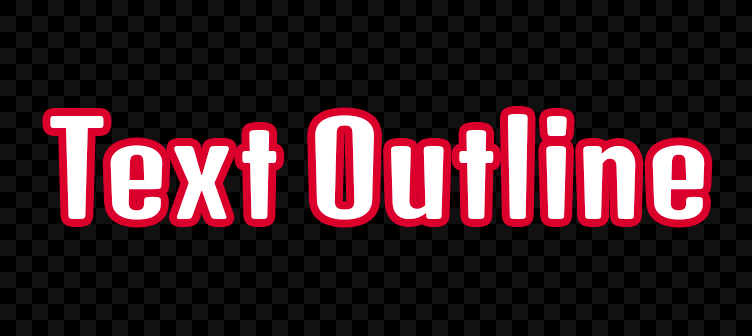
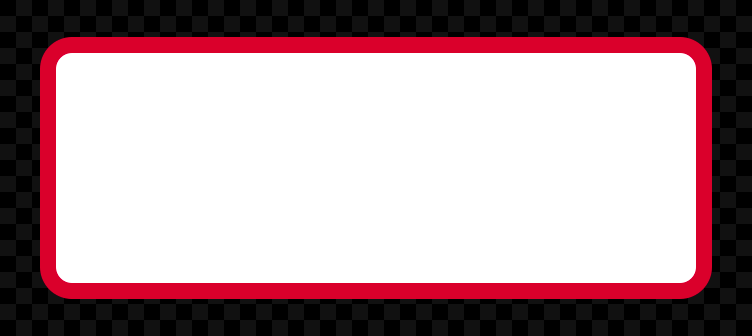
When applied to a text object, you can override the default stroke behavior by the ApplyStrokeMode property, letting you apply the stroke to the object's bounds instead of the text itself. You can even control the text outline and border independently by parenting two UIStroke instances to a text object, one set to Contextual and the other to Border.
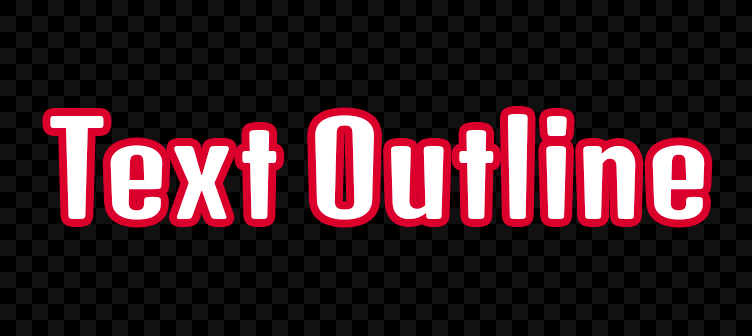
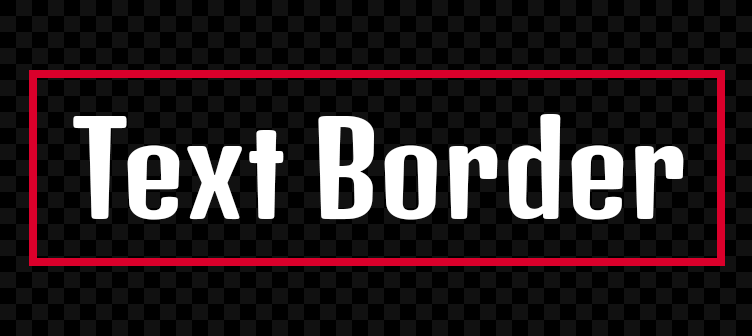
Thickness
You can set the stroke width through the Thickness property which is measured in pixels from the parent's outer edges.
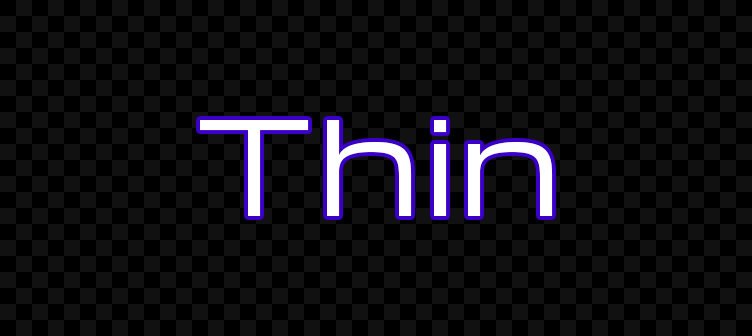
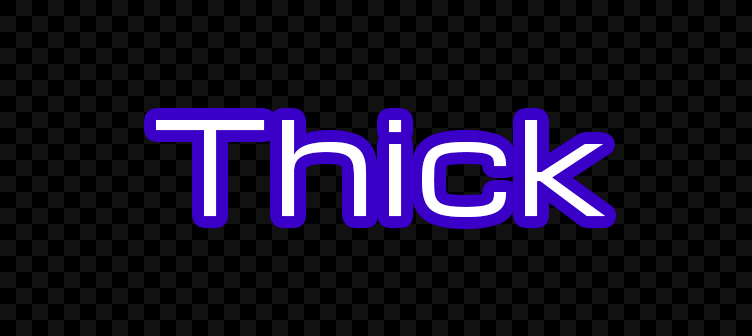
Color / gradient
You can set the stroke color through the Color property, as well as insert a child UIGradient instance to create gradient strokes.
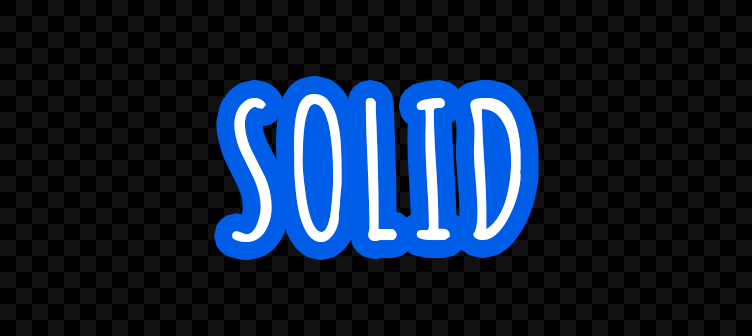
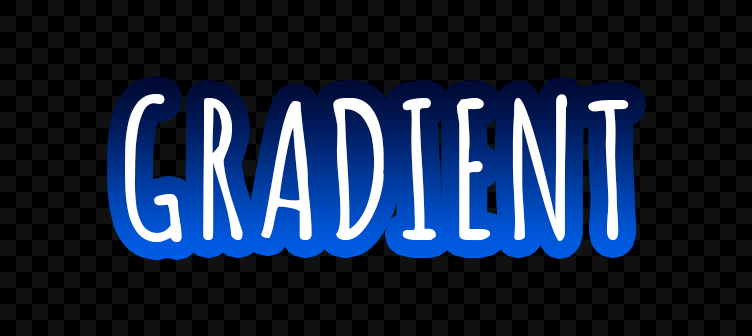
Transparency
The Transparency property sets the stroke transparency independently of the parent object's BackgroundTransparency or TextTransparency. This allows you to render text and borders that are "hollow" (consisting of only an outline).
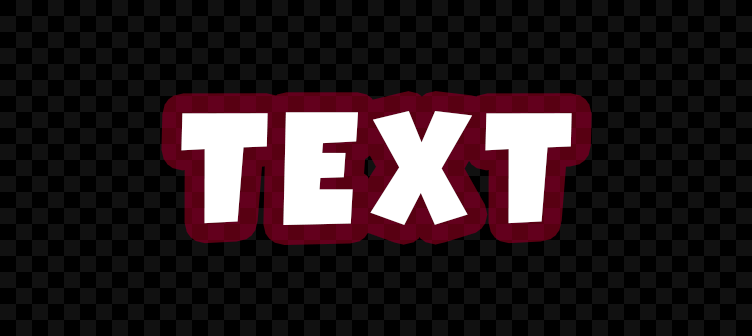
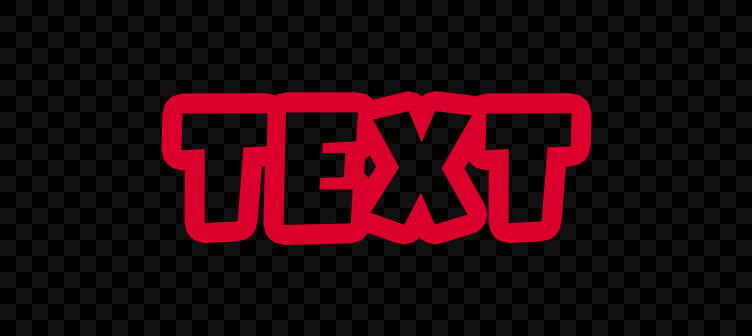
Corner style
The LineJoinMode property lets you control how corners are interpreted. It accepts a value of either Round, Bevel, or Miter.
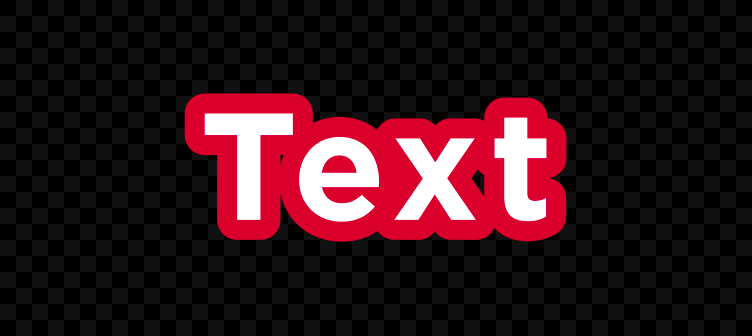
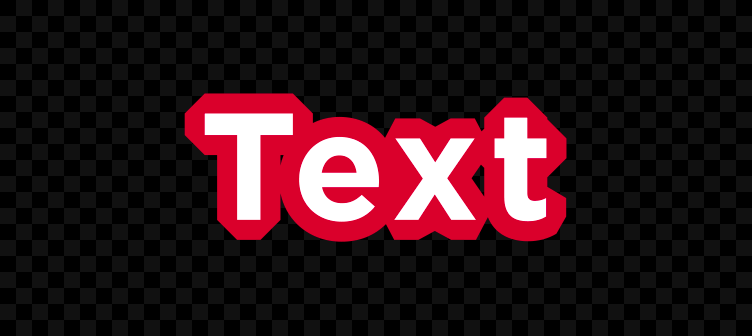
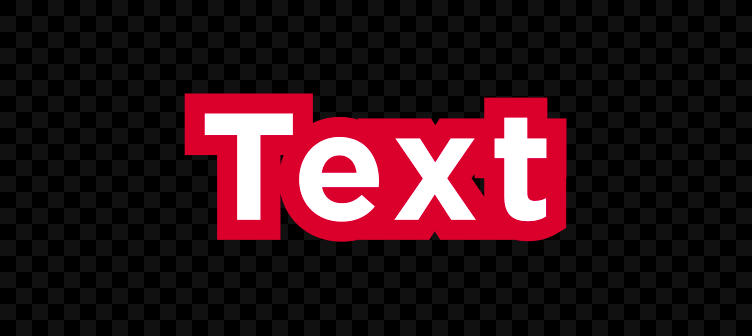
Corners
The UICorner instance applies deformation to all four corners of its parent GuiObject. You can control the applied radius through the CornerRadius property using either Scale or Offset.
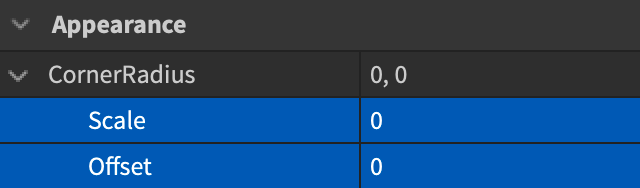
Scale rounds the corners to a percentage based on the total length of the shortest edge of the parent, meaning that a scale of 0.5 or higher deforms the parent into a "pill" shape, regardless of its width or height. Offset rounds the corners to a specific number of pixels, regardless of the width/height of the parent.
Padding
A UIPadding object applies top, bottom, left, and/or right padding to the contents of the parent GuiObject.
For example, you can move the text inside a text button downward or upward by applying an offset to the bottom of the button.