Passes allow you to charge a one-time Robux fee in order for players to access special privileges within an experience, such as entry to a restricted area, an in-experience avatar item, or a permanent power-up.
Creating Passes
When you're creating an image to use for your Pass, consider the following requirements:
- Use a template of 512×512 pixels.
- Save the image in either .jpg, .png, or .bmp format.
- Don't include important details outside of the circular boundaries because the upload process trims and crops the final badge into a circular image.
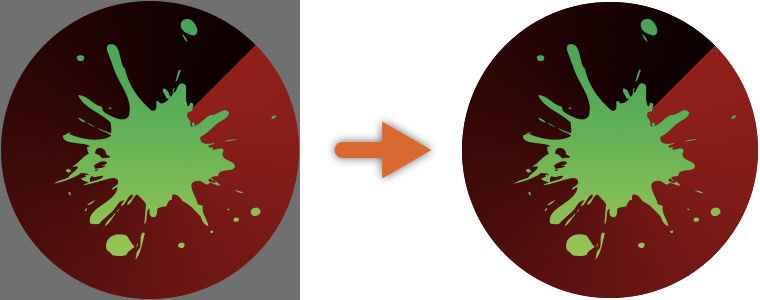
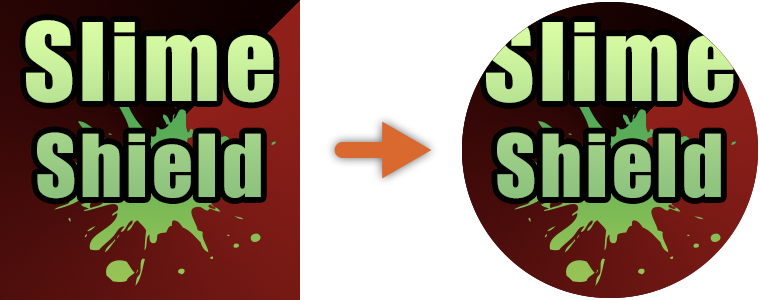
To create a new Pass:
Navigate to your Creations page on Creator Dashboard and select your experience.
In the Monetization menu, select Passes. All passes for that experience display.
Click the Create a Pass button.
Uploading an image for your pass is optional. If desired, press the Change button to change the default image and upload your own.
Fill in the following fields:
- Name: A title for your Pass.
- Description: A description what a player should expect when they purchase the Pass.
Click the Create Pass button. The Pass displays within the Passes section of the Creations page.
Locating Pass IDs
A Pass ID is the unique identifier of a Pass. You need this ID to assign the Pass a special privilege.
To locate a Pass ID:
Navigate to the Passes section of an experience's Monetization menu.
Hover over a Pass thumbnail and click the ⋯ button. A contextual menu displays.
Select Copy Asset ID. The Pass ID copies to your clipboard.
Monetizing Passes
After you create a Pass, you can configure its settings to monetize it.
To monetize a Pass:
Navigate to the Passes section of an experience's Monetization menu.
Hover over a Pass thumbnail and click the ⋯ button. A contextual menu displays.
Select Open in New Tab. The Configure Pass page displays.
In the left-hand navigation, select Sales.
Enable the Item for Sale toggle.
In the Price field, enter the amount of Robux you want to charge players for the Pass. The price you enter affects the amount of Robux you earn per sale.
Click the Save Changes button.
Assigning Pass Privileges
Once a player purchases a Pass, they'll expect to receive the associated special privilege when they play your experience. This does not happen automatically, so you must check which players own the Pass and assign the special privilege to them.
The following script checks when any player enters the experience, then verifies if that player owns the Pass with the matching ID set in the variable passID. Place this code in a Script within ServerScriptService so the server can handle the special privilege given to the player.
local MarketplaceService = game:GetService("MarketplaceService")
local Players = game:GetService("Players")
local passID = 0000000 -- Change this to your Pass ID
local function onPlayerAdded(player)
local hasPass = false
-- Check if the player already owns the Pass
local success, message = pcall(function()
hasPass = MarketplaceService:UserOwnsGamePassAsync(player.UserId, passID)
end)
-- If there's an error, issue a warning and exit the function
if not success then
warn("Error while checking if player has pass: " .. tostring(message))
return
end
if hasPass then
print(player.Name .. " owns the Pass with ID " .. passID)
-- Assign this player the ability or bonus related to the Pass
end
end
-- Connect "PlayerAdded" events to the function
Players.PlayerAdded:Connect(onPlayerAdded)
Prompting In-Experience Purchases
While players can purchase Passes directly from your experience's main page, you can also offer in-experience purchases to players through a shop or vendor NPC within the experience. Reference the example server-side and client-side scripts for a basic model to prompt players to purchase Passes.
Example Server-Side Script
Place this code in a Script object within ServerScriptService so the server can handle the special privilege given to the player.
local MarketplaceService = game:GetService("MarketplaceService")
local passID = 0000000 -- Change this to your Pass ID
-- Function to handle a completed prompt and purchase
local function onPromptPurchaseFinished(player, purchasedPassID, purchaseSuccess)
if purchaseSuccess and purchasedPassID == passID then
print(player.Name .. " purchased the Pass with ID " .. passID)
-- Assign this player the ability or bonus related to the Pass
end
end
-- Connect "PromptGamePassPurchaseFinished" events to the function
MarketplaceService.PromptGamePassPurchaseFinished:Connect(onPromptPurchaseFinished)
Example Client-Side Script
The following code implements a promptPurchase() function which safely checks if a player has a Pass and prompts them to purchase it if they don't already have it. Place this code in a LocalScript and call promptPurchase() in situations such as when the player clicks a button or when their character touches a part.
local MarketplaceService = game:GetService("MarketplaceService")
local Players = game:GetService("Players")
local passID = 0000000 -- Change this to your Pass ID
-- Function to prompt purchase of the Pass
local function promptPurchase()
local player = Players.LocalPlayer
local hasPass = false
local success, message = pcall(function()
hasPass = MarketplaceService:UserOwnsGamePassAsync(player.UserId, passID)
end)
if not success then
warn("Error while checking if player has pass: " .. tostring(message))
return
end
if hasPass then
-- Player already owns the Pass; tell them somehow
else
-- Player does NOT own the Pass; prompt them to purchase
MarketplaceService:PromptGamePassPurchase(player, passID)
end
end
Passes Analytics
Passes Analytics help you gauge the success of individual Passes, identify trends, and forecast potential future earnings.
To access Passes analytics:
Navigate to your Creations page on Creator Dashboard and select your experience.
Navigate to Monetization > Passes and select the Analytics tab.
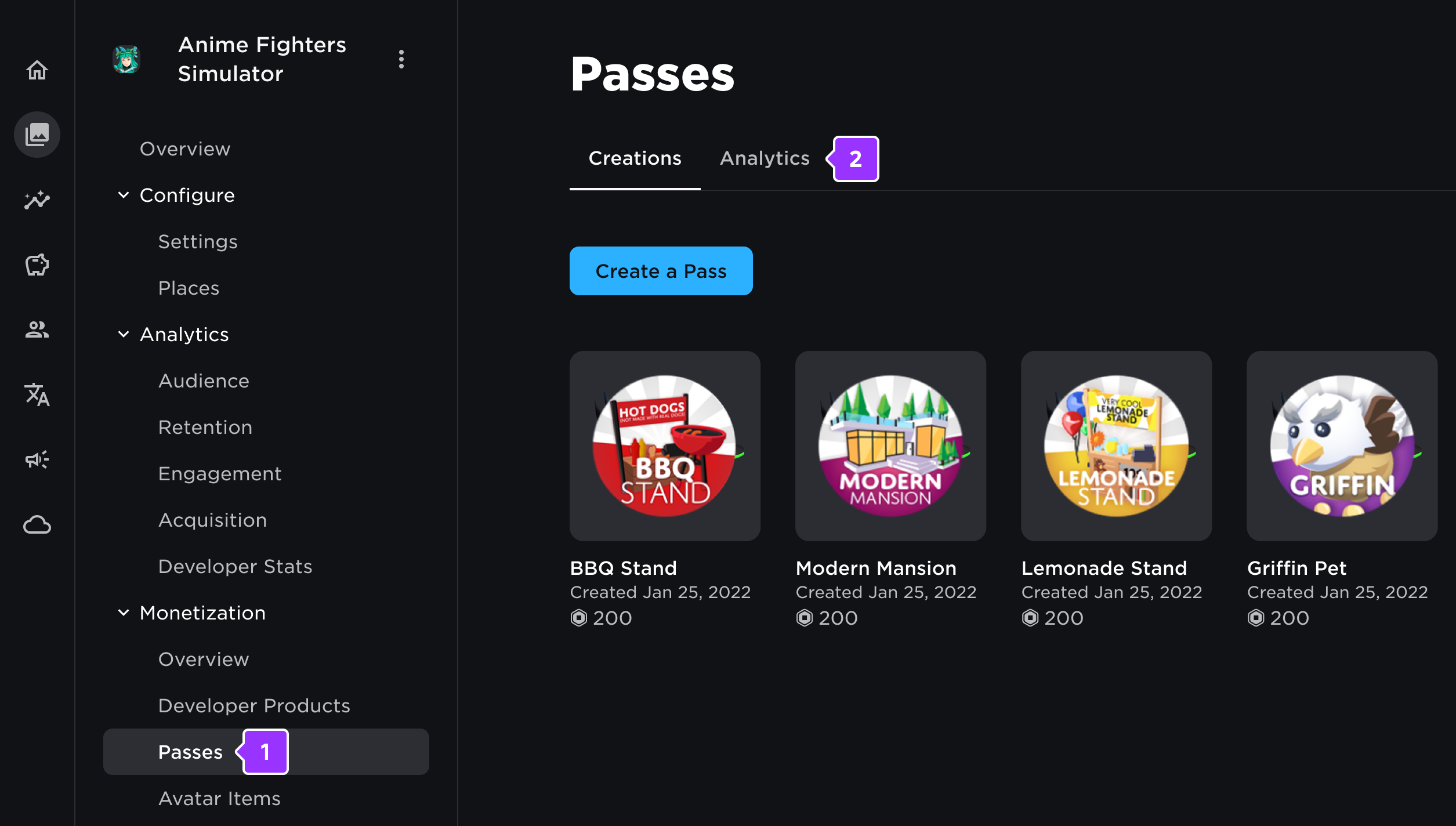
The analytics tab enables you to:
- View top performing items: See your top selling and top grossing Passes over a selected time period.
- Analyze overall sales and net revenue: Showcase up to eight top items on a time-series graph.
- Monitor your catalog: Examine a table with up to 400 items, sortable by sales and net revenue.
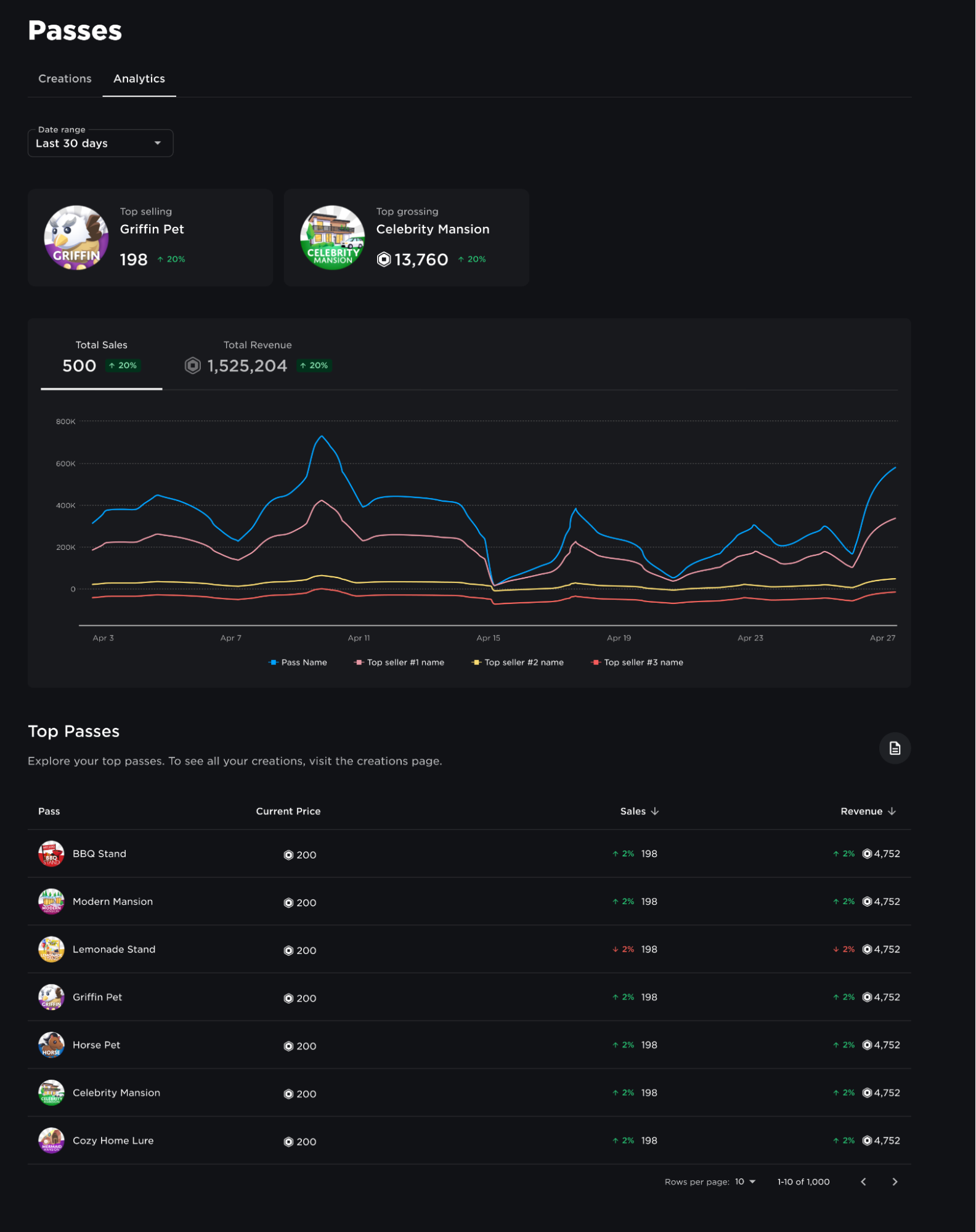