There are different ways to make code run over and over. If you want the code to only run a certain amount of times, use a for loop. This article will cover the logic behind for loops and demonstrate some practical examples, such as coding a countdown.
How For Loops Work
For loops use three values to control how many times they run: a control variable, an end value, and an increment value. Starting from the value of the control variable, the for loops will either count up or down each time it runs code inside the loop until it passes the end value. Positive increment values count up, and negative increment values count down.
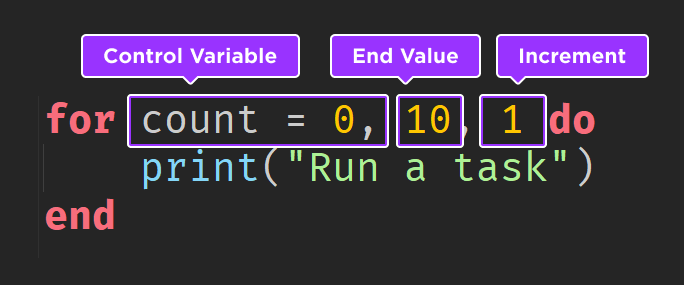
Steps in a For Loop
To understand for loops, it helps to see a flow chart diagram showing the logic of how they progress.
First, the for loop compares the control variable with the end value.
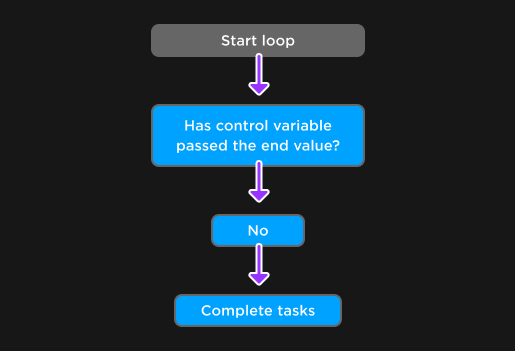
After running the code, the increment value is added to the control variable. The loop then checks the control variable and starts over.
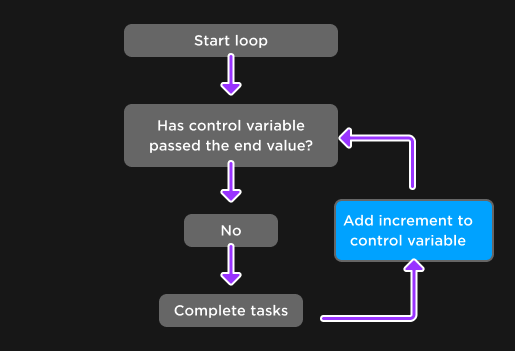
Once the control variable passes the end value, the loop will stop. For example, if a loop has an end value of 10, once the control variable has passed 10, the for loop will stop.
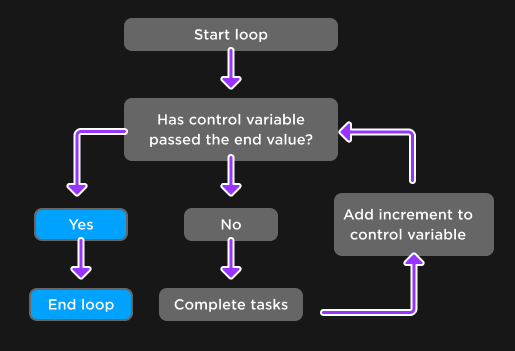
Code a Countdown
To see how a for loop works, use these steps to code a for loop that starts at 10 and counts down to 0, one number at a time. Every time the loop runs, it'll print the current value inside the control variable.
In ServerScriptService, create a new script named PracticeLoop. In the script, start by typing the keyword for.
forCreate a control variable named count and set a starting value of 10.
for count = 10Set the end value to 0, by typing , 0. Make sure to include a comma to separate the values.
for count = 10, 0Create an increment value of -1 by typing , -1. After the loop has finished its action, it'll add the increment value to the control variable, count. Because the increment is negative, it'll be subtracted when added to the control variable.
for count = 10, 0, -1To finish the for loop, type do and press Enter to add end. Any code typed between do and end will run each time the loop repeats.
for count = 10, 0, -1 doendInside the loop, create a countdown by printing the value of the control variable, count, and then delay the script with a wait function.
for count = 10, 0, -1 do-- Prints the current number the for loop is onprint(count)-- Wait 1 secondtask.wait(1)endRun the project and watch the Output Window to see the for loop.
Notice that the loop will print out the current value of count each time it goes through an iteration. An iteration is the complete process of checking the control value, running code, and updating the increment value. Because the control variable starts at 0 and has to go pass 10, the loop will go through 11 iterations before stopping.
Troubleshooting Tips
At this point, if the loop doesn't work as intended, try one of the following below.
- Check that you have two commas separating the numbers in your for loop. Having extra or missing commas will make the loop not start.
- If the for loop prints all at once, make sure that there is a wait function that uses at least 1 second.
Different For Loop Examples
Changing the three values of a for loop will change how the loop functions. Below are different examples of for loops with different start, end, and increment values. Try putting them into scripts and see what happens.
Counting Up By One
for count = 0, 5, 1 doprint(count)task.wait(1)end
Counting Up in Even Numbers
for count = 0, 10, 2 doprint(count)task.wait(1)end
If For Loops Don't Run At All
If the control variable starts out beyond the end value, like in the example below, the for loop won't run at all.
for count = 10, 0, 1 doprint(count)task.wait(1)end
In this case, the for loop is counting up and checking if count is greater than 0. When the for loop does it's first check, it sees that 10 is greater than 0, and so it'll stop the loop without printing anything.
Summary
A for loop is a common type of loop that's used when a set of instructions should repeat a specific amount of times. To create a for loop, use three variables with the syntax below:
for count = 0, 10, 1 doprint(count)end
In the example above, the loop will start at 0. For each loop, it will print the count variable, add 1 to count, and finally finish looping when count is equal to 10.